How to Use Git Branches to Manage Your Multiple Personalities
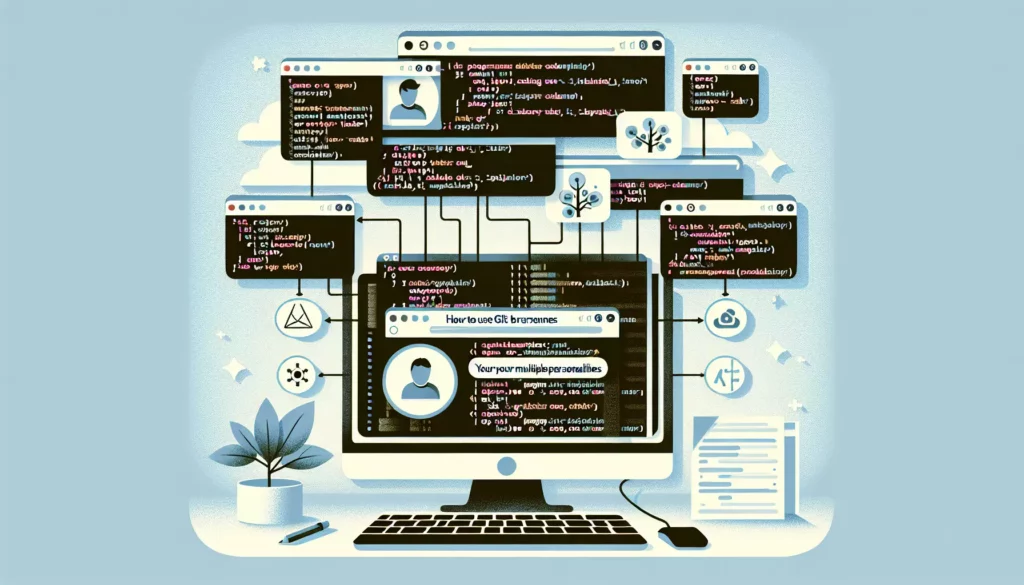
As developers, we often find ourselves juggling multiple tasks, features, and even personalities within a single project. Enter Git branches – the superhero tool that allows us to manage these various aspects of our coding life with ease and grace. In this comprehensive guide, we’ll dive deep into the world of Git branches, exploring how they can help you organize your work, collaborate effectively, and maybe even keep your sanity intact.
Table of Contents
- Understanding Git Branches
- Creating and Switching Branches
- Managing Multiple Personalities with Branches
- Merging Branches
- Resolving Conflicts
- Best Practices for Branch Management
- Advanced Branch Techniques
- Introduction to Git Flow
- Tools and Integrations for Branch Management
- Conclusion
1. Understanding Git Branches
Before we dive into the nitty-gritty of managing your multiple personalities with Git branches, let’s start with the basics. What exactly is a Git branch?
In Git, a branch is essentially a lightweight movable pointer to a commit. It represents an independent line of development, allowing you to work on different features, bug fixes, or experiments without affecting the main codebase. Think of branches as parallel universes where you can explore different ideas and implementations without fear of breaking the main timeline.
The default branch in Git is usually called “master” or “main”. When you create a new branch, you’re creating a new pointer to the current commit you’re on. As you make new commits on this branch, it will diverge from the original branch, creating a separate line of development.
2. Creating and Switching Branches
Now that we understand what branches are, let’s look at how to create and switch between them. Here are the basic commands you’ll need:
Creating a new branch:
git branch <branch-name>
This command creates a new branch but doesn’t switch to it.
Switching to a branch:
git checkout <branch-name>
This command switches to the specified branch.
Creating and switching to a new branch in one command:
git checkout -b <new-branch-name>
This command creates a new branch and immediately switches to it.
Listing all branches:
git branch
This command shows a list of all local branches, with an asterisk next to the currently checked-out branch.
3. Managing Multiple Personalities with Branches
Now, let’s get to the heart of our topic: using Git branches to manage your multiple coding personalities. Here are some scenarios where branches can help you organize your work and mindset:
The Feature Developer
When you’re working on a new feature, create a feature branch:
git checkout -b feature/awesome-new-feature
This allows you to focus solely on developing the new feature without worrying about other parts of the project.
The Bug Fixer
When you need to fix a critical bug, create a hotfix branch:
git checkout -b hotfix/critical-bug-fix
This enables you to quickly address the issue without disrupting ongoing feature development.
The Experimenter
When you want to try out a radical new idea, create an experimental branch:
git checkout -b experiment/crazy-idea
This gives you the freedom to explore without the pressure of producing production-ready code.
The Refactorer
When you’re improving existing code, create a refactor branch:
git checkout -b refactor/optimize-algorithm
This allows you to focus on improving code quality without introducing new features or fixing bugs.
By using branches in this way, you can easily switch between different tasks and mindsets, keeping your work organized and your code clean.
4. Merging Branches
Once you’ve completed work on a branch, you’ll want to integrate those changes back into the main codebase. This is where merging comes in. Here’s how to merge a branch:
- First, switch to the branch you want to merge into (usually main or master):
git checkout main
- Then, merge the feature branch into the current branch:
git merge feature/awesome-new-feature
Git will attempt to automatically merge the changes. If successful, it will create a new “merge commit” that combines the histories of both branches.
5. Resolving Conflicts
Sometimes, when merging branches, Git may encounter conflicts. This happens when the same part of a file has been modified differently in the two branches being merged. When this occurs, Git will pause the merge process and mark the conflicting areas in the affected files.
To resolve conflicts:
- Open the conflicting files and look for the conflict markers (<<<<<<<, =======, and >>>>>>>).
- Manually edit the files to resolve the conflicts, removing the conflict markers in the process.
- After resolving all conflicts, stage the changed files:
git add <resolved-file>
- Complete the merge by creating a merge commit:
git commit -m "Merge feature/awesome-new-feature into main"
6. Best Practices for Branch Management
To effectively manage your multiple personalities with Git branches, consider these best practices:
- Use descriptive branch names: Name your branches in a way that clearly indicates their purpose, e.g., “feature/user-authentication” or “bugfix/login-error”.
- Keep branches short-lived: Try to complete and merge feature branches quickly to avoid long-running branches that become difficult to merge.
- Regularly update your feature branches: Periodically merge or rebase the main branch into your feature branches to stay up-to-date and reduce merge conflicts.
- Delete merged branches: After successfully merging a branch, delete it to keep your repository clean:
git branch -d feature/completed-feature
- Use a consistent branching strategy: Adopt a branching model like GitFlow or GitHub Flow across your team to maintain consistency.
7. Advanced Branch Techniques
As you become more comfortable with basic branching, you can explore some advanced techniques:
Rebasing
Rebasing is an alternative to merging that can create a cleaner project history. Instead of creating a merge commit, rebasing moves the entire feature branch to begin on the tip of the main branch, effectively incorporating all of the new commits in main. Here’s how to rebase:
git checkout feature/awesome-new-feature
git rebase main
Cherry-picking
Cherry-picking allows you to pick specific commits from one branch and apply them to another. This can be useful when you want to apply a bug fix to multiple branches:
git cherry-pick <commit-hash>
Interactive Rebasing
Interactive rebasing allows you to modify commits in a branch before moving them to another branch. This is great for cleaning up your commit history:
git rebase -i HEAD~3
This command will open an interactive rebase for the last 3 commits.
8. Introduction to Git Flow
Git Flow is a popular branching model that provides a robust framework for managing larger projects. It defines a strict branching model designed around project releases, providing a structure for feature development, release preparation, and maintenance.
The main branches in Git Flow are:
- master: Always reflects a production-ready state.
- develop: Serves as an integration branch for features.
- feature/*: Used to develop new features.
- release/*: Prepare for a new production release.
- hotfix/*: Used to quickly patch production releases.
While Git Flow can be complex for smaller projects, it provides a solid structure for managing multiple aspects of development simultaneously.
9. Tools and Integrations for Branch Management
Several tools can help you manage your Git branches more effectively:
- GitKraken: A powerful Git GUI client that provides a visual representation of your branches and commit history.
- SourceTree: Another popular Git GUI that offers a user-friendly interface for managing branches.
- GitHub Desktop: A simplified Git client that integrates well with GitHub repositories.
- IDE Integrations: Many IDEs like Visual Studio Code, IntelliJ IDEA, and others offer built-in Git functionality, including branch management.
These tools can provide a more intuitive interface for managing branches, especially for visual learners or those new to Git.
10. Conclusion
Git branches are a powerful tool for managing the multiple aspects of your development work. By effectively using branches, you can compartmentalize different tasks, experiment freely, and collaborate more efficiently with your team. Whether you’re fixing bugs, developing new features, or refactoring code, branches allow you to switch between these different “personalities” seamlessly.
Remember, the key to successful branch management is to keep your branches focused, short-lived, and well-organized. With practice, you’ll find that branching becomes second nature, allowing you to juggle multiple tasks and perspectives with ease.
So go ahead, embrace your multiple coding personalities, and let Git branches help you become a more organized and efficient developer. Happy coding!