How to Use Git Bisect to Find Bugs in Your Code
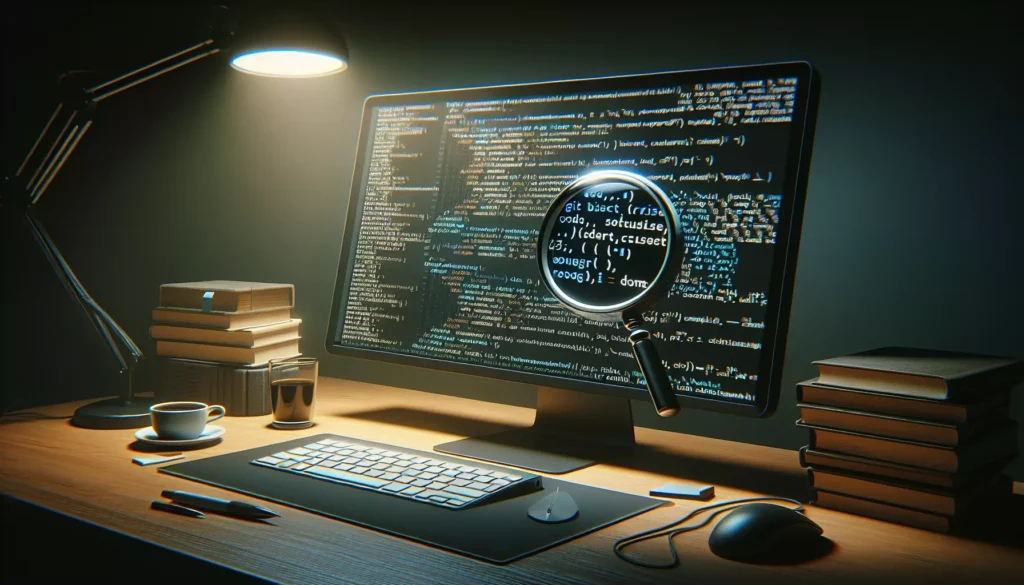
As developers, we’ve all been there: you’re working on a project, and suddenly you realize that something’s not quite right. A bug has crept into your code, but you’re not sure when or where it was introduced. This is where Git bisect comes to the rescue. In this comprehensive guide, we’ll explore how to use Git bisect to efficiently track down bugs in your codebase, saving you time and frustration in the debugging process.
What is Git Bisect?
Git bisect is a powerful debugging tool that helps you find the commit that introduced a bug in your project. It uses a binary search algorithm to efficiently narrow down the problematic commit, allowing you to pinpoint the exact change that caused the issue.
The term “bisect” refers to the process of dividing something into two equal parts. In the context of Git, it means systematically dividing your commit history to locate the source of a bug.
When to Use Git Bisect
Git bisect is particularly useful in the following scenarios:
- You’ve discovered a bug in your code, but you’re not sure when it was introduced.
- The bug is in a part of the codebase that has undergone many changes recently.
- You’re working on a large project with a long commit history.
- You need to identify which specific change caused a test to start failing.
Prerequisites
Before we dive into using Git bisect, make sure you have the following:
- Git installed on your system
- A Git repository with a commit history
- A way to reproduce the bug or test for its presence
The Git Bisect Process
Using Git bisect involves the following steps:
- Start the bisect process
- Mark a known good commit
- Mark a known bad commit
- Test the current commit and mark it as good or bad
- Repeat step 4 until Git identifies the first bad commit
- End the bisect process
Now, let’s go through each step in detail.
1. Starting the Bisect Process
To begin using Git bisect, navigate to your project’s root directory in the terminal and run:
git bisect start
This command initializes the bisect process and prepares Git to start searching for the problematic commit.
2. Marking a Known Good Commit
Next, you need to tell Git about a commit where you know the bug didn’t exist. This is typically an older commit where everything was working correctly. You can specify this commit using its hash or a branch name:
git bisect good <commit-hash-or-branch-name>
For example:
git bisect good 1a2b3c4
Or, if you know the bug didn’t exist in the master branch:
git bisect good master
3. Marking a Known Bad Commit
Now, you need to specify a commit where the bug is present. This is typically a more recent commit, often the current HEAD of your branch:
git bisect bad <commit-hash-or-branch-name>
For example:
git bisect bad HEAD
After marking the good and bad commits, Git will automatically check out a commit halfway between the two points you specified.
4. Testing and Marking Commits
At this point, Git has checked out a commit for you to test. Your job is to determine whether this commit is “good” (bug-free) or “bad” (contains the bug). Run your tests or manually check for the bug’s presence.
If the bug is present in this commit, mark it as bad:
git bisect bad
If the bug is not present, mark it as good:
git bisect good
After you mark the commit, Git will automatically check out the next commit for you to test, continuously narrowing down the search range.
5. Repeating the Process
Continue testing and marking commits as good or bad. Git will use this information to perform a binary search, efficiently narrowing down the range of commits where the bug was introduced.
6. Identifying the First Bad Commit
Once Git has enough information to identify the first bad commit, it will display a message like this:
b1a2c3d4 is the first bad commit
commit b1a2c3d4e5f6g7h8i9j0k1l2m3n4o5p6q7r8s9
Author: John Doe <john.doe@example.com>
Date: Mon Apr 10 09:00:00 2023 -0700
Implement new feature X
This output shows you the commit hash, author, date, and commit message of the first bad commit that introduced the bug.
7. Ending the Bisect Process
Once you’ve found the problematic commit, you can end the bisect process by running:
git bisect reset
This command will return you to the branch you were on when you started the bisect process.
Advanced Git Bisect Techniques
While the basic Git bisect process is powerful on its own, there are some advanced techniques you can use to make it even more efficient:
Automated Testing with Git Bisect Run
If you have an automated test that can detect the presence of the bug, you can use the git bisect run
command to automate the entire process. Here’s how it works:
- Create a script that exits with code 0 if the test passes (good) or non-zero if it fails (bad).
- Start the bisect process and specify the good and bad commits as before.
- Run the automated bisect process with your script:
git bisect run ./your-test-script.sh
Git will automatically run through the commits, using your script to determine if each commit is good or bad, until it finds the first bad commit.
Bisecting a Specific File or Directory
If you know that the bug is related to changes in a specific file or directory, you can use the -- <path>
option to limit the bisect process to that area of your codebase:
git bisect start -- path/to/file/or/directory
This can significantly speed up the process by focusing only on relevant commits.
Visualizing the Bisect Process
To get a visual representation of the bisect process, you can use the git bisect visualize
command:
git bisect visualize
This will open your configured Git visualization tool (such as gitk) to show you the commit graph and help you understand where you are in the bisect process.
Skipping Commits
Sometimes, you may encounter a commit that you can’t test (e.g., it doesn’t compile). In such cases, you can skip the commit using:
git bisect skip
Git will move on to the next commit without using the skipped commit to narrow down the search.
Best Practices for Using Git Bisect
To make the most of Git bisect, consider the following best practices:
- Commit frequently: Smaller, more frequent commits make it easier to pinpoint the exact change that introduced a bug.
- Write good commit messages: Clear and descriptive commit messages help you understand what changes were made in each commit during the bisect process.
- Use automated tests: Having a reliable suite of automated tests makes it much easier to determine if a commit is good or bad.
- Keep your repository clean: Regularly merging feature branches and avoiding long-lived branches can help simplify the bisect process.
- Document your findings: After identifying the bug-introducing commit, consider adding a note to your issue tracker or creating a fix commit that references the bisect process.
Limitations and Considerations
While Git bisect is a powerful tool, it’s important to be aware of its limitations:
- Non-linear bugs: Git bisect assumes that the bug was introduced in a single commit. If the bug is the result of multiple commits or complex interactions between changes, bisect may not be as effective.
- Intermittent bugs: If the bug doesn’t consistently reproduce, it can be challenging to accurately mark commits as good or bad.
- Time-consuming process: For large repositories with many commits, the bisect process can take a significant amount of time, especially if manual testing is required.
- Dependency on reproducibility: Git bisect relies on your ability to consistently reproduce the bug across different commits.
Alternatives to Git Bisect
While Git bisect is an excellent tool for finding bugs, there are other debugging techniques and tools you might consider:
- Git blame: Use
git blame
to see who last modified each line of a file, which can be helpful for tracking down recent changes. - Git log: Examine the commit history using
git log
to manually review recent changes. - Debugging tools: Use language-specific debugging tools and IDEs to step through code and identify issues.
- Code review: Implement thorough code review processes to catch bugs before they make it into the main branch.
- Continuous Integration (CI): Set up CI pipelines to automatically run tests on each commit, helping to identify bugs early.
Conclusion
Git bisect is a powerful and efficient tool for tracking down bugs in your codebase. By systematically narrowing down the range of commits where a bug was introduced, it can save you hours of manual debugging and help you pinpoint the exact change that caused an issue.
As you become more comfortable with Git bisect, you’ll find that it becomes an indispensable part of your debugging toolkit. Remember to combine it with other debugging techniques and best practices to maintain a healthy, bug-free codebase.
By mastering Git bisect and other advanced Git features, you’ll be better equipped to handle complex debugging scenarios and contribute more effectively to large-scale software projects. Happy bug hunting!