How to Use Fenwick Trees and Segment Trees in Coding Challenges
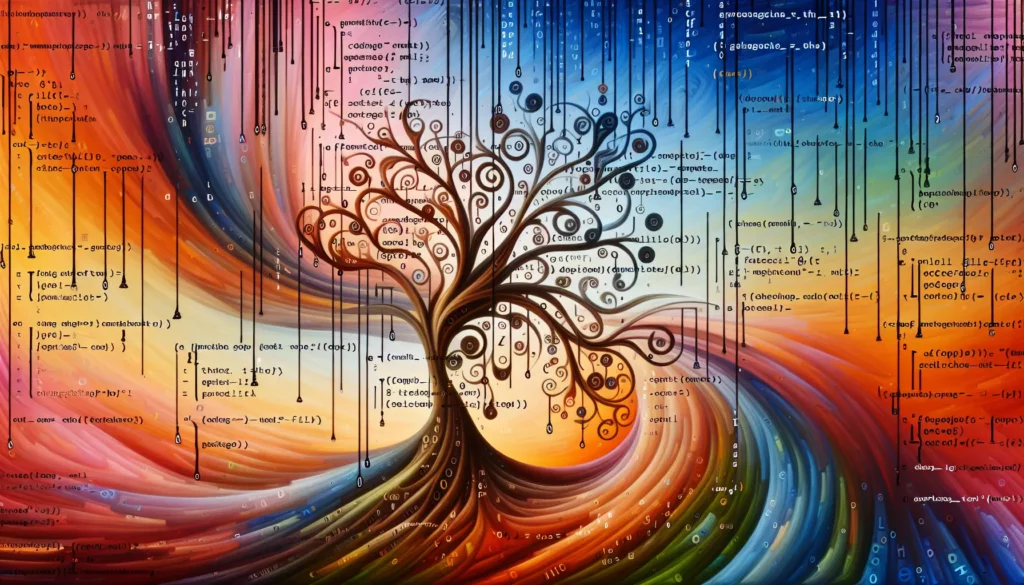
In the world of competitive programming and coding interviews, efficient data structures play a crucial role in solving complex problems. Two such powerful data structures that often come in handy are Fenwick Trees (also known as Binary Indexed Trees) and Segment Trees. These structures are particularly useful for handling range queries and updates on arrays in logarithmic time complexity. In this comprehensive guide, we’ll explore how to use Fenwick Trees and Segment Trees in coding challenges, their implementations, and real-world applications.
Understanding Fenwick Trees
Fenwick Trees, introduced by Peter Fenwick in 1994, are a clever data structure designed to efficiently handle cumulative frequency tables. They’re particularly useful for calculating prefix sums and updating elements in an array.
Key Features of Fenwick Trees:
- Space-efficient: Requires O(n) space
- Fast updates: O(log n) time complexity
- Quick prefix sum calculations: O(log n) time complexity
- Relatively simple implementation compared to Segment Trees
Basic Operations of Fenwick Trees:
- Update: Modify a single element in the array
- Query: Calculate the sum of elements from index 1 to a given index
Implementation of Fenwick Tree in C++:
#include <vector>
class FenwickTree {
private:
std::vector<int> tree;
public:
FenwickTree(int n) : tree(n + 1, 0) {}
void update(int i, int delta) {
while (i < tree.size()) {
tree[i] += delta;
i += i & (-i);
}
}
int query(int i) {
int sum = 0;
while (i > 0) {
sum += tree[i];
i -= i & (-i);
}
return sum;
}
};
In this implementation, we use a vector to represent the Fenwick Tree. The update
function adds a value to an element, while the query
function calculates the prefix sum up to a given index.
Using Fenwick Trees in Coding Challenges
Fenwick Trees are particularly useful in problems involving range sum queries and point updates. Here’s an example problem:
Problem: Given an array of n integers, perform m operations. Each operation is either:
- Update: Change the value at index i to x
- Query: Find the sum of elements from index l to r (inclusive)
Here’s how we can solve this using a Fenwick Tree:
#include <iostream>
#include <vector>
class FenwickTree {
// ... (implementation as above)
};
int main() {
int n, m;
std::cin >> n >> m;
std::vector<int> arr(n + 1, 0);
FenwickTree ft(n);
// Initialize the Fenwick Tree
for (int i = 1; i <= n; i++) {
std::cin >> arr[i];
ft.update(i, arr[i]);
}
// Process queries
for (int i = 0; i < m; i++) {
char type;
std::cin >> type;
if (type == 'U') {
int idx, val;
std::cin >> idx >> val;
int delta = val - arr[idx];
arr[idx] = val;
ft.update(idx, delta);
} else if (type == 'Q') {
int l, r;
std::cin >> l >> r;
std::cout << ft.query(r) - ft.query(l - 1) << std::endl;
}
}
return 0;
}
This solution efficiently handles both update and query operations in O(log n) time complexity.
Understanding Segment Trees
Segment Trees are versatile data structures that allow for efficient range queries and updates on arrays. They’re particularly useful when dealing with more complex operations beyond simple sum queries.
Key Features of Segment Trees:
- Flexible: Can handle various types of range queries (sum, min, max, GCD, etc.)
- Efficient updates: O(log n) time complexity
- Fast range queries: O(log n) time complexity
- More memory-intensive: Requires O(4n) space
Basic Operations of Segment Trees:
- Build: Construct the segment tree from the input array
- Update: Modify a single element in the array
- Query: Perform a range query (e.g., find the sum, minimum, or maximum in a given range)
Implementation of Segment Tree in C++:
#include <vector>
#include <algorithm>
class SegmentTree {
private:
std::vector<int> tree;
int n;
void build(const std::vector<int>& arr, int v, int tl, int tr) {
if (tl == tr) {
tree[v] = arr[tl];
} else {
int tm = (tl + tr) / 2;
build(arr, v*2, tl, tm);
build(arr, v*2+1, tm+1, tr);
tree[v] = tree[v*2] + tree[v*2+1];
}
}
void update(int v, int tl, int tr, int pos, int new_val) {
if (tl == tr) {
tree[v] = new_val;
} else {
int tm = (tl + tr) / 2;
if (pos <= tm)
update(v*2, tl, tm, pos, new_val);
else
update(v*2+1, tm+1, tr, pos, new_val);
tree[v] = tree[v*2] + tree[v*2+1];
}
}
int query(int v, int tl, int tr, int l, int r) {
if (l > r)
return 0;
if (l == tl && r == tr)
return tree[v];
int tm = (tl + tr) / 2;
return query(v*2, tl, tm, l, std::min(r, tm))
+ query(v*2+1, tm+1, tr, std::max(l, tm+1), r);
}
public:
SegmentTree(const std::vector<int>& arr) {
n = arr.size();
tree.resize(4 * n);
build(arr, 1, 0, n - 1);
}
void update(int pos, int new_val) {
update(1, 0, n - 1, pos, new_val);
}
int query(int l, int r) {
return query(1, 0, n - 1, l, r);
}
};
This implementation creates a segment tree for range sum queries. The tree is represented as a vector, where each node stores the sum of a specific range of the original array.
Using Segment Trees in Coding Challenges
Segment Trees are particularly useful in problems involving range queries and updates on arrays. Here’s an example problem:
Problem: Given an array of n integers, perform m operations. Each operation is either:
- Update: Change the value at index i to x
- Query: Find the minimum value in the range [l, r] (inclusive)
To solve this problem using a Segment Tree, we need to modify our implementation slightly to handle minimum queries instead of sum queries:
#include <iostream>
#include <vector>
#include <algorithm>
#include <limits>
class SegmentTree {
private:
std::vector<int> tree;
int n;
void build(const std::vector<int>& arr, int v, int tl, int tr) {
if (tl == tr) {
tree[v] = arr[tl];
} else {
int tm = (tl + tr) / 2;
build(arr, v*2, tl, tm);
build(arr, v*2+1, tm+1, tr);
tree[v] = std::min(tree[v*2], tree[v*2+1]);
}
}
void update(int v, int tl, int tr, int pos, int new_val) {
if (tl == tr) {
tree[v] = new_val;
} else {
int tm = (tl + tr) / 2;
if (pos <= tm)
update(v*2, tl, tm, pos, new_val);
else
update(v*2+1, tm+1, tr, pos, new_val);
tree[v] = std::min(tree[v*2], tree[v*2+1]);
}
}
int query(int v, int tl, int tr, int l, int r) {
if (l > r)
return std::numeric_limits<int>::max();
if (l == tl && r == tr)
return tree[v];
int tm = (tl + tr) / 2;
return std::min(query(v*2, tl, tm, l, std::min(r, tm)),
query(v*2+1, tm+1, tr, std::max(l, tm+1), r));
}
public:
SegmentTree(const std::vector<int>& arr) {
n = arr.size();
tree.resize(4 * n);
build(arr, 1, 0, n - 1);
}
void update(int pos, int new_val) {
update(1, 0, n - 1, pos, new_val);
}
int query(int l, int r) {
return query(1, 0, n - 1, l, r);
}
};
int main() {
int n, m;
std::cin >> n >> m;
std::vector<int> arr(n);
for (int i = 0; i < n; i++) {
std::cin >> arr[i];
}
SegmentTree st(arr);
// Process queries
for (int i = 0; i < m; i++) {
char type;
std::cin >> type;
if (type == 'U') {
int idx, val;
std::cin >> idx >> val;
st.update(idx, val);
} else if (type == 'Q') {
int l, r;
std::cin >> l >> r;
std::cout << st.query(l, r) << std::endl;
}
}
return 0;
}
This solution efficiently handles both update and minimum range query operations in O(log n) time complexity.
Comparing Fenwick Trees and Segment Trees
While both Fenwick Trees and Segment Trees are powerful data structures for handling range queries and updates, they have different strengths and use cases:
Fenwick Trees:
- Pros:
- More memory-efficient (O(n) space)
- Simpler implementation
- Slightly faster in practice for simple operations
- Cons:
- Limited to cumulative operations (like sum)
- Cannot handle more complex range queries easily
- Best for: Problems involving prefix sums, frequency counting, or simple cumulative operations
Segment Trees:
- Pros:
- Highly versatile, can handle various types of range queries
- Can be easily modified for different operations (min, max, GCD, etc.)
- Supports range updates with lazy propagation
- Cons:
- More complex implementation
- Higher memory usage (O(4n) space)
- Best for: Problems involving complex range queries, range updates, or when flexibility is needed
Advanced Techniques and Variations
Lazy Propagation in Segment Trees
Lazy propagation is an optimization technique used in Segment Trees to handle range updates efficiently. Instead of immediately propagating updates to all affected nodes, we delay the updates and only apply them when necessary. This can significantly improve performance for problems involving frequent range updates.
Here’s a basic implementation of a Segment Tree with lazy propagation for range sum queries and range updates:
#include <vector>
class SegmentTree {
private:
std::vector<long long> tree, lazy;
int n;
void push(int v, int tl, int tr) {
if (lazy[v] != 0) {
tree[v] += (tr - tl + 1) * lazy[v];
if (tl != tr) {
lazy[v*2] += lazy[v];
lazy[v*2+1] += lazy[v];
}
lazy[v] = 0;
}
}
void update(int v, int tl, int tr, int l, int r, int addend) {
push(v, tl, tr);
if (l > r)
return;
if (l == tl && tr == r) {
lazy[v] += addend;
push(v, tl, tr);
} else {
int tm = (tl + tr) / 2;
update(v*2, tl, tm, l, std::min(r, tm), addend);
update(v*2+1, tm+1, tr, std::max(l, tm+1), r, addend);
tree[v] = tree[v*2] + tree[v*2+1];
}
}
long long query(int v, int tl, int tr, int l, int r) {
push(v, tl, tr);
if (l > r)
return 0;
if (l == tl && tr == r)
return tree[v];
int tm = (tl + tr) / 2;
return query(v*2, tl, tm, l, std::min(r, tm)) +
query(v*2+1, tm+1, tr, std::max(l, tm+1), r);
}
public:
SegmentTree(int size) : n(size) {
tree.resize(4 * n);
lazy.resize(4 * n);
}
void update(int l, int r, int addend) {
update(1, 0, n - 1, l, r, addend);
}
long long query(int l, int r) {
return query(1, 0, n - 1, l, r);
}
};
This implementation allows for efficient range updates and queries, both with O(log n) time complexity.
2D Fenwick Trees
2D Fenwick Trees (or Binary Indexed Trees) are an extension of the regular Fenwick Tree to handle 2D range queries and updates. They’re particularly useful for problems involving 2D prefix sums or range sum queries on matrices.
Here’s a basic implementation of a 2D Fenwick Tree:
#include <vector>
class FenwickTree2D {
private:
std::vector<std::vector<int>> tree;
int n, m;
public:
FenwickTree2D(int rows, int cols) : n(rows), m(cols) {
tree.assign(n + 1, std::vector<int>(m + 1, 0));
}
void update(int x, int y, int delta) {
for (int i = x; i <= n; i += i & (-i))
for (int j = y; j <= m; j += j & (-j))
tree[i][j] += delta;
}
int query(int x, int y) {
int sum = 0;
for (int i = x; i > 0; i -= i & (-i))
for (int j = y; j > 0; j -= j & (-j))
sum += tree[i][j];
return sum;
}
int rangeQuery(int x1, int y1, int x2, int y2) {
return query(x2, y2) - query(x1 - 1, y2) - query(x2, y1 - 1) + query(x1 - 1, y1 - 1);
}
};
This 2D Fenwick Tree allows for point updates and range sum queries on a 2D grid, both with O(log n * log m) time complexity.
Real-world Applications
Fenwick Trees and Segment Trees find applications in various real-world scenarios beyond competitive programming:
- Database Systems: For efficiently maintaining aggregates (sum, min, max) over ranges of data.
- Financial Analysis: Calculating cumulative sums or moving averages over time series data.
- Image Processing: Computing 2D prefix sums for fast image manipulations or feature extraction.
- Geographic Information Systems (GIS): Handling range queries on spatial data.
- Network Analysis: Maintaining statistics over network traffic or log data.
- Text Editors: Implementing efficient undo/redo functionality or syntax highlighting.
Conclusion
Fenwick Trees and Segment Trees are powerful data structures that can significantly improve the efficiency of algorithms dealing with range queries and updates. While Fenwick Trees excel in simplicity and memory efficiency for cumulative operations, Segment Trees offer greater flexibility for a wide range of query types.
Mastering these data structures can give you a significant advantage in coding challenges and technical interviews, especially when dealing with problems involving range operations. As you practice and become more comfortable with these structures, you’ll find that many seemingly complex problems become much more manageable.
Remember, the key to mastering these data structures is practice. Try implementing them from scratch, solve various problems that utilize them, and experiment with different variations and optimizations. With time and experience, you’ll develop the intuition to know when and how to apply these powerful tools in your coding arsenal.
Happy coding, and may your range queries be ever efficient!