How to Use Divide and Conquer to Solve Algorithms Efficiently
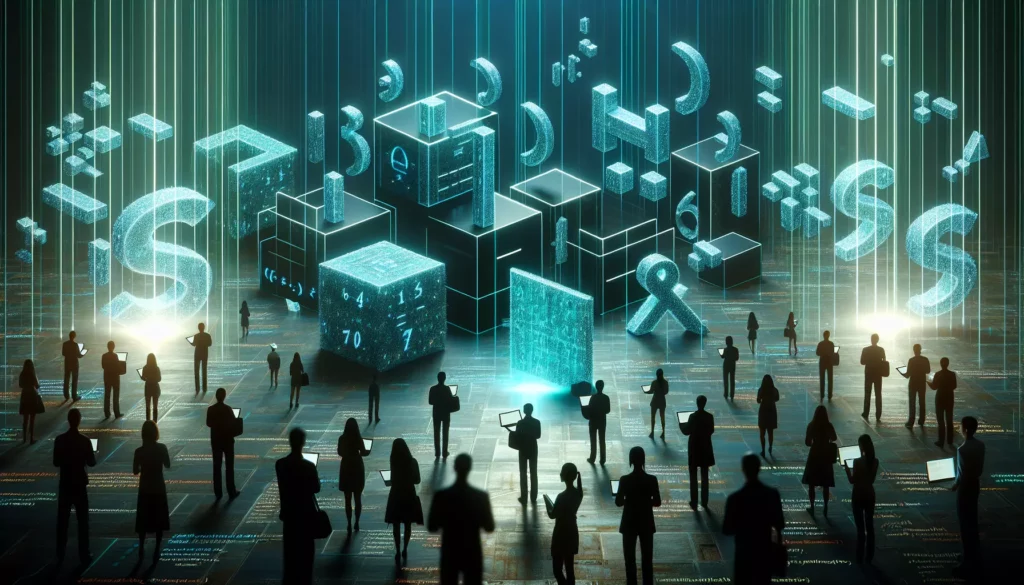
In the world of algorithm design and problem-solving, the divide and conquer approach stands out as a powerful technique that can significantly improve the efficiency of your solutions. This strategy is not only crucial for coding interviews at top tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google) but also forms the backbone of many real-world applications. In this comprehensive guide, we’ll explore how to effectively use divide and conquer to solve algorithms efficiently, providing you with the tools you need to tackle complex problems with confidence.
Understanding Divide and Conquer
Before we dive into the specifics of implementing divide and conquer algorithms, it’s essential to understand the core concept. The divide and conquer paradigm follows a three-step process:
- Divide: Break the problem into smaller, manageable subproblems.
- Conquer: Solve these subproblems recursively.
- Combine: Merge the solutions of the subproblems to create a solution to the original problem.
This approach is particularly effective for problems that can be broken down into similar, smaller instances of the same problem. By recursively applying this technique, we can often achieve more efficient solutions compared to straightforward iterative approaches.
Key Advantages of Divide and Conquer
Understanding the benefits of divide and conquer will help you recognize when to apply this technique:
- Efficiency: Many divide and conquer algorithms have logarithmic time complexity, making them highly efficient for large datasets.
- Parallelization: The independent nature of subproblems in divide and conquer algorithms often allows for easy parallelization, further improving performance on multi-core systems.
- Simplicity: Breaking complex problems into smaller, more manageable pieces can lead to cleaner, more understandable code.
- Reusability: The solutions to subproblems can often be reused, promoting code efficiency and reducing redundancy.
Classic Examples of Divide and Conquer Algorithms
To better understand how divide and conquer works in practice, let’s examine some classic algorithms that utilize this approach:
1. Merge Sort
Merge Sort is a prime example of a divide and conquer algorithm. It sorts an array by recursively dividing it into two halves, sorting each half, and then merging the sorted halves.
def merge_sort(arr):
if len(arr) <= 1:
return arr
mid = len(arr) // 2
left = merge_sort(arr[:mid])
right = merge_sort(arr[mid:])
return merge(left, right)
def merge(left, right):
result = []
i, j = 0, 0
while i < len(left) and j < len(right):
if left[i] < right[j]:
result.append(left[i])
i += 1
else:
result.append(right[j])
j += 1
result.extend(left[i:])
result.extend(right[j:])
return result
Time Complexity: O(n log n)
2. Quick Sort
Quick Sort is another sorting algorithm that uses divide and conquer. It selects a ‘pivot’ element and partitions the array around it, recursively sorting the subarrays on either side of the pivot.
def quick_sort(arr):
if len(arr) <= 1:
return arr
pivot = arr[len(arr) // 2]
left = [x for x in arr if x < pivot]
middle = [x for x in arr if x == pivot]
right = [x for x in arr if x > pivot]
return quick_sort(left) + middle + quick_sort(right)
Time Complexity: O(n log n) on average, O(n^2) in the worst case
3. Binary Search
Binary Search is a classic divide and conquer algorithm used to efficiently search a sorted array by repeatedly dividing the search interval in half.
def binary_search(arr, target):
left, right = 0, len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1 # Target not found
Time Complexity: O(log n)
Implementing Divide and Conquer: A Step-by-Step Guide
Now that we’ve seen some examples, let’s break down the process of implementing a divide and conquer algorithm:
- Identify the base case: Determine the simplest version of the problem that can be solved directly.
- Define the divide step: Decide how to break the problem into smaller subproblems.
- Implement the conquer step: Solve the subproblems recursively.
- Design the combine step: Determine how to merge the solutions of the subproblems.
- Optimize and refine: Look for opportunities to improve efficiency, such as memoization or tail recursion.
Advanced Divide and Conquer Techniques
As you become more comfortable with basic divide and conquer algorithms, you can explore more advanced techniques:
1. Strassen’s Matrix Multiplication
This algorithm improves upon the naive O(n^3) matrix multiplication algorithm by using clever matrix partitioning and recursive calls.
2. Karatsuba Algorithm for Fast Multiplication
Karatsuba’s algorithm provides a faster way to multiply large numbers by reducing the problem to multiplying smaller numbers.
3. Closest Pair of Points
This geometric problem uses divide and conquer to efficiently find the closest pair of points in a set of points in a plane.
Common Pitfalls and How to Avoid Them
While divide and conquer is a powerful technique, there are some common mistakes to watch out for:
- Incorrect base case: Ensure your base case correctly handles the simplest version of the problem.
- Inefficient division: The way you divide the problem can significantly impact efficiency. Aim for balanced divisions when possible.
- Overlooking the combine step: Sometimes, the combine step can be as complex as the divide step. Don’t underestimate its importance.
- Redundant computations: Without proper optimization, you might end up solving the same subproblems multiple times. Consider memoization or dynamic programming to avoid this.
Divide and Conquer in Technical Interviews
For those preparing for technical interviews at FAANG companies or other top tech firms, mastering divide and conquer is crucial. Here are some tips for applying this technique in interview settings:
- Recognize the pattern: Train yourself to identify problems that can benefit from a divide and conquer approach.
- Communicate your thought process: Explain your reasoning as you break down the problem and design your solution.
- Analyze time and space complexity: Be prepared to discuss the efficiency of your solution in terms of big O notation.
- Consider edge cases: Think about how your algorithm handles extreme or unusual inputs.
- Practice, practice, practice: Solve a variety of divide and conquer problems to build your intuition and speed.
Real-World Applications of Divide and Conquer
Divide and conquer isn’t just for coding interviews; it has numerous practical applications in software development:
- Database systems: Many database operations, such as indexing and query optimization, use divide and conquer principles.
- Image processing: Algorithms for image compression, such as the Fast Fourier Transform (FFT), often employ divide and conquer techniques.
- Parallel computing: The independent nature of subproblems in divide and conquer algorithms makes them ideal for parallel processing.
- Machine learning: Some clustering algorithms and decision tree construction methods use divide and conquer approaches.
Enhancing Your Divide and Conquer Skills
To truly master divide and conquer, consider the following strategies:
- Study mathematical foundations: Understanding concepts like recurrence relations and the master theorem can help you analyze and design divide and conquer algorithms more effectively.
- Implement variations: Try implementing different versions of classic algorithms, such as randomized quicksort or external merge sort.
- Solve related problems: After mastering a divide and conquer algorithm, look for similar problems that can be solved using the same approach.
- Analyze trade-offs: Compare divide and conquer solutions with other algorithmic approaches to understand their strengths and weaknesses in different scenarios.
- Explore hybrid approaches: Learn how to combine divide and conquer with other techniques like dynamic programming or greedy algorithms for more complex problems.
Conclusion
Mastering the divide and conquer technique is an essential skill for any programmer looking to solve complex problems efficiently. By breaking down large problems into smaller, more manageable pieces, you can create elegant and efficient solutions that scale well with input size. Whether you’re preparing for a technical interview at a top tech company or working on real-world software projects, the ability to apply divide and conquer principles will serve you well throughout your career.
Remember, like any skill, proficiency in divide and conquer comes with practice. Challenge yourself with diverse problems, analyze your solutions, and continuously refine your approach. With dedication and persistence, you’ll find that even the most daunting algorithmic challenges become conquerable when approached with a divide and conquer mindset.
As you continue your journey in algorithm design and problem-solving, keep exploring new applications of divide and conquer and stay curious about emerging techniques in the field. The world of algorithms is vast and ever-evolving, and mastering fundamental approaches like divide and conquer will provide you with a solid foundation for tackling whatever challenges come your way.