How to Use Database Normalization to Understand Your Relationships
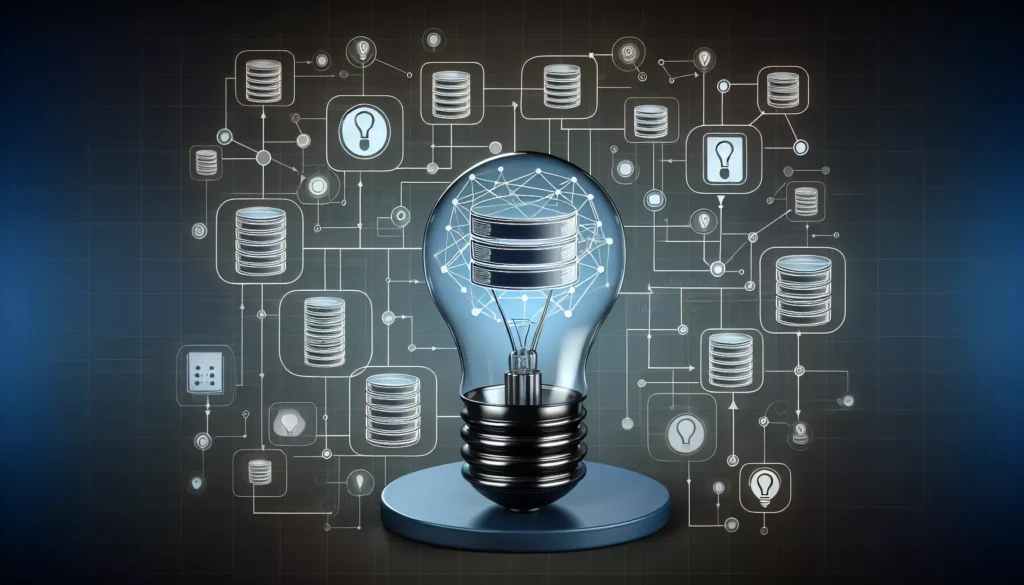
In the world of database design, understanding and implementing proper relationships between data entities is crucial for creating efficient, maintainable, and scalable systems. One of the most powerful tools in a database designer’s arsenal is the process of normalization. This technique not only helps in organizing data effectively but also provides deep insights into the relationships between different pieces of information. In this comprehensive guide, we’ll explore how database normalization can be used to understand and optimize your data relationships, with a focus on its application in coding education and programming skills development.
What is Database Normalization?
Database normalization is a systematic approach to organizing data in a relational database. It involves breaking down larger tables into smaller, more focused tables and establishing relationships between them. The primary goals of normalization are to:
- Eliminate redundant data
- Ensure data dependencies make sense
- Facilitate data maintenance and reduce update anomalies
- Improve data integrity
Normalization is typically carried out through a series of steps, each known as a “normal form.” The most commonly used normal forms are First Normal Form (1NF), Second Normal Form (2NF), and Third Normal Form (3NF), although higher normal forms exist for more specialized cases.
The Importance of Normalization in Coding Education
In the context of coding education and programming skills development, understanding database normalization is crucial for several reasons:
- Foundational Knowledge: Normalization concepts form the basis of relational database design, which is a fundamental skill for many software development roles.
- Problem-Solving Skills: The process of normalization enhances analytical and problem-solving abilities, which are essential for algorithmic thinking and coding challenges.
- Data Modeling: It teaches students how to model real-world relationships in a digital format, a skill applicable across various programming domains.
- Optimization: Understanding normalization helps in creating efficient database structures, which is crucial for developing high-performance applications.
- Interview Preparation: Database design and normalization are common topics in technical interviews, especially for roles involving backend development or data engineering.
The Process of Normalization: Understanding Relationships Step by Step
Let’s walk through the normalization process using an example relevant to a coding education platform. We’ll start with a denormalized table and progress through the normal forms, explaining how each step helps in understanding and refining data relationships.
Initial Denormalized Table: CourseEnrollments
Imagine we have a table that stores information about course enrollments on a coding education platform:
CourseEnrollments
--------------------
StudentID
StudentName
StudentEmail
CourseID
CourseName
InstructorID
InstructorName
EnrollmentDate
CompletionStatus
This table contains redundant data and has several potential issues. Let’s normalize it to understand the relationships better.
First Normal Form (1NF)
The first step in normalization is to ensure that the table adheres to First Normal Form (1NF). To achieve 1NF:
- Eliminate repeating groups
- Create a separate table for each set of related data
- Identify each set of related data with a primary key
Our initial table is already in 1NF as it doesn’t contain any repeating groups. Each column contains atomic values, and we can identify unique rows using a combination of StudentID and CourseID.
Second Normal Form (2NF)
To achieve Second Normal Form, we need to remove partial dependencies. This means that all non-key attributes should depend on the entire primary key, not just a part of it. In our case, we have a composite key (StudentID, CourseID), and some attributes depend only on StudentID or CourseID.
Let’s break this down into separate tables:
Students
--------
StudentID (Primary Key)
StudentName
StudentEmail
Courses
-------
CourseID (Primary Key)
CourseName
InstructorID
Instructors
-----------
InstructorID (Primary Key)
InstructorName
Enrollments
-----------
StudentID (Foreign Key)
CourseID (Foreign Key)
EnrollmentDate
CompletionStatus
Now we have four tables, each focusing on a specific entity. This structure helps us understand the relationships better:
- Students have their own information (name, email) independent of courses
- Courses are associated with instructors
- Instructors are separate entities with their own information
- Enrollments represent the many-to-many relationship between students and courses
Third Normal Form (3NF)
To achieve Third Normal Form, we need to remove transitive dependencies. This means that non-key attributes should not depend on other non-key attributes. Our current structure is already in 3NF, as we don’t have any transitive dependencies.
Understanding Relationships Through Normalization
Now that we’ve normalized our database, let’s examine how this process has helped us understand the relationships between different entities in our coding education platform:
1. Student-Course Relationship
The normalization process revealed that students and courses have a many-to-many relationship. A student can enroll in multiple courses, and a course can have multiple students. This relationship is represented by the Enrollments table, which serves as a junction table.
This insight is crucial for designing features like:
- Displaying a student’s course list
- Showing enrolled students for each course
- Implementing course recommendations based on a student’s enrollment history
2. Course-Instructor Relationship
By separating courses and instructors into different tables, we’ve identified that there’s a one-to-many relationship between instructors and courses. An instructor can teach multiple courses, but (in this model) a course is taught by one instructor.
This relationship helps in implementing features such as:
- Displaying an instructor’s course portfolio
- Filtering courses by instructor
- Managing instructor workload and course assignments
3. Student Information Management
By isolating student information in its own table, we’ve made it clear that student details are independent of their course enrollments. This separation allows for easier management of student profiles and ensures that updating a student’s information (like email) doesn’t require changes to enrollment records.
4. Enrollment Tracking
The Enrollments table now clearly represents the act of a student enrolling in a course. It includes time-sensitive information like the enrollment date and completion status. This structure facilitates:
- Tracking student progress across multiple courses
- Generating reports on course popularity and completion rates
- Implementing features like course prerequisites based on completion status
Applying Normalization Concepts in Coding Challenges
Understanding database normalization not only helps in designing efficient databases but also enhances problem-solving skills for coding challenges. Let’s explore how normalization concepts can be applied in algorithmic thinking and problem-solving:
1. Data Structure Design
The principles of normalization can be applied when designing data structures for complex problems. For example, when solving a problem that involves managing relationships between different entities (like users, posts, and comments in a social media application), thinking in terms of normalized structures can lead to more efficient and maintainable solutions.
2. Reducing Redundancy in Algorithms
The concept of eliminating redundancy in normalization can be applied to algorithm design. When solving coding challenges, look for opportunities to remove duplicate logic or data, similar to how normalization removes redundant data in databases.
3. Identifying Dependencies
The process of identifying functional dependencies in normalization enhances the ability to recognize relationships and dependencies in various coding problems. This skill is particularly useful in optimizing algorithms and designing efficient data processing pipelines.
Example: Optimizing a Coding Challenge Solution
Consider a coding challenge where you need to implement a system for managing coding bootcamp enrollments. Here’s how normalization concepts can be applied:
class Student:
def __init__(self, id, name, email):
self.id = id
self.name = name
self.email = email
class Course:
def __init__(self, id, name, instructor_id):
self.id = id
self.name = name
self.instructor_id = instructor_id
class Enrollment:
def __init__(self, student_id, course_id, date, status):
self.student_id = student_id
self.course_id = course_id
self.date = date
self.status = status
class BootcampSystem:
def __init__(self):
self.students = {}
self.courses = {}
self.enrollments = []
def add_student(self, id, name, email):
self.students[id] = Student(id, name, email)
def add_course(self, id, name, instructor_id):
self.courses[id] = Course(id, name, instructor_id)
def enroll_student(self, student_id, course_id, date):
if student_id in self.students and course_id in self.courses:
enrollment = Enrollment(student_id, course_id, date, "In Progress")
self.enrollments.append(enrollment)
return True
return False
def get_student_courses(self, student_id):
return [e.course_id for e in self.enrollments if e.student_id == student_id]
def get_course_students(self, course_id):
return [e.student_id for e in self.enrollments if e.course_id == course_id]
In this example, we’ve applied normalization concepts by:
- Separating entities into distinct classes (Student, Course, Enrollment)
- Avoiding redundant data storage
- Establishing clear relationships between entities
- Implementing methods that utilize these relationships efficiently
Normalization and Database Performance
While normalization is crucial for maintaining data integrity and understanding relationships, it’s important to consider its impact on database performance. In some cases, denormalization might be necessary for optimizing query performance. Let’s explore this balance:
Advantages of Normalized Databases
- Reduced Data Redundancy: Minimizes storage requirements and reduces the risk of data inconsistencies.
- Easier Data Maintenance: Updates need to be made in only one place, reducing the chance of update anomalies.
- Better Data Integrity: Enforces data consistency and reduces the risk of data corruption.
- Flexibility for Future Changes: Makes it easier to extend the database schema as requirements evolve.
Potential Performance Considerations
- Join Operations: Highly normalized databases often require multiple joins to retrieve related data, which can impact query performance.
- Complex Queries: Some queries might become more complex and potentially slower in a fully normalized database.
- Read-Heavy vs. Write-Heavy Workloads: Normalization typically benefits write-heavy workloads but might slow down read-heavy operations.
Balancing Normalization and Performance
To strike a balance between normalization and performance:
- Analyze Query Patterns: Understand the most common and performance-critical queries in your application.
- Consider Selective Denormalization: In some cases, it might be beneficial to denormalize specific parts of the database to optimize for frequently accessed data.
- Use Indexing Strategies: Properly indexed tables can significantly improve query performance in normalized databases.
- Implement Caching: For read-heavy operations, caching can mitigate the performance impact of complex joins.
- Utilize Database Views: Create views that combine data from multiple tables for frequently used query patterns.
Example: Optimizing for Performance
Let’s consider an example where we might want to optimize our coding education platform for quick access to student enrollment information:
CREATE VIEW student_enrollment_summary AS
SELECT
s.StudentID,
s.StudentName,
c.CourseID,
c.CourseName,
e.EnrollmentDate,
e.CompletionStatus
FROM
Students s
JOIN
Enrollments e ON s.StudentID = e.StudentID
JOIN
Courses c ON e.CourseID = c.CourseID;
This view combines information from multiple tables, providing quick access to commonly needed enrollment data without sacrificing the benefits of our normalized structure.
Normalization in Modern Database Systems
As database technologies evolve, the application of normalization principles has adapted to new paradigms. Let’s explore how normalization concepts apply in modern database systems:
1. NoSQL Databases
NoSQL databases, such as MongoDB or Cassandra, often use a denormalized data model to optimize for read performance and scalability. However, normalization concepts are still relevant:
- Document Databases: While they allow for nested structures, applying normalization concepts can help in designing efficient document schemas.
- Key-Value Stores: Normalization principles can guide the design of composite keys and value structures.
2. Graph Databases
Graph databases, like Neo4j, are inherently good at representing relationships. Normalization concepts can be applied to:
- Design efficient node properties
- Determine what should be a node vs. a relationship property
- Optimize graph structures for specific query patterns
3. NewSQL Databases
NewSQL databases aim to provide the scalability of NoSQL systems with the ACID guarantees of traditional relational databases. In these systems:
- Normalization remains important for data integrity
- The impact on performance is often mitigated by advanced distributed architectures
Practical Tips for Applying Normalization in Coding Projects
When working on coding projects, especially those involving data management, applying normalization principles can greatly enhance the quality and maintainability of your code. Here are some practical tips:
1. Start with a Clear Entity-Relationship Diagram (ERD)
Before diving into code, create an ERD to visualize the relationships between different entities in your system. This visual representation can help you identify potential normalization issues early in the design process.
2. Implement Data Access Objects (DAOs) or Repositories
Use the DAO pattern or repository pattern to encapsulate data access logic. This abstraction makes it easier to maintain a normalized database structure while providing a clean API for your application logic.
3. Utilize Object-Relational Mapping (ORM) Tools
ORM tools like SQLAlchemy (Python), Hibernate (Java), or Entity Framework (C#) can help maintain a normalized database structure while allowing you to work with object-oriented code.
4. Regular Database Reviews
As your project evolves, regularly review your database structure. Look for signs of denormalization creeping in, such as redundant data or complex update processes.
5. Performance Profiling
Use database profiling tools to identify performance bottlenecks. This can help you make informed decisions about when to denormalize for performance reasons.
6. Educate Your Team
Ensure that all team members understand the importance of normalization. This shared knowledge helps maintain data integrity throughout the development process.
Conclusion
Database normalization is a powerful technique that goes beyond just organizing data efficiently. It provides deep insights into the relationships between different entities in your system, which is crucial for designing robust and scalable applications. In the context of coding education and skill development, understanding normalization not only prepares you for database-related tasks but also enhances your overall problem-solving and data modeling abilities.
By applying normalization principles, you can:
- Create more maintainable and flexible database structures
- Improve data integrity and reduce redundancy
- Develop a deeper understanding of data relationships
- Enhance your ability to design efficient algorithms and data structures
- Better prepare for technical interviews and real-world software development challenges
Remember, while normalization is a valuable tool, it’s important to balance it with performance considerations and the specific needs of your application. As you continue to develop your coding skills, practice applying these normalization concepts to various projects and coding challenges. This will not only improve your database design skills but also contribute to your overall growth as a programmer.