How to Use Comments to Document and Clarify Your Code
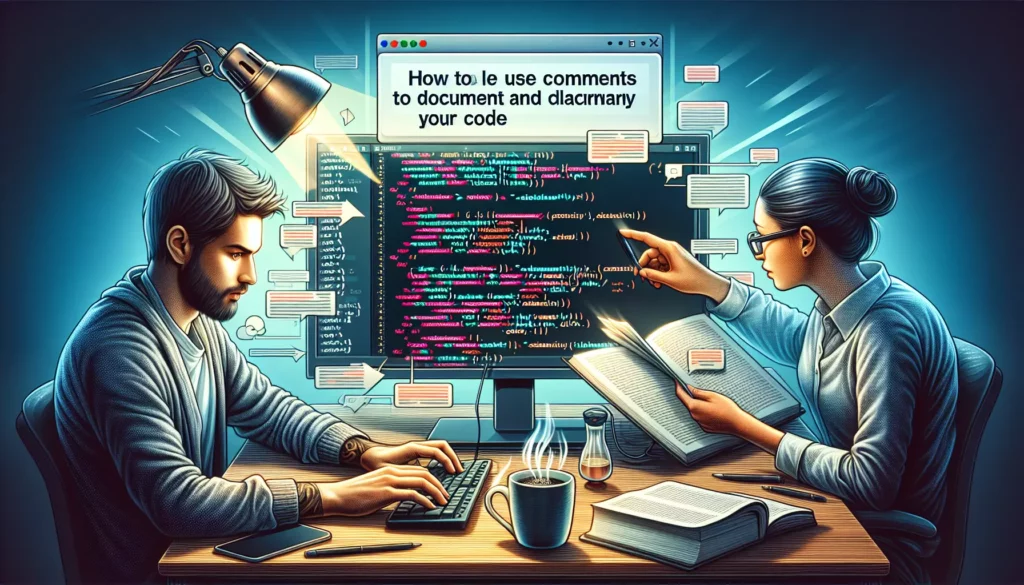
In the world of programming, writing clean, readable, and maintainable code is a crucial skill. One of the most effective ways to achieve this is through the proper use of comments. Comments are non-executable lines of text within your code that provide explanations, documentation, and clarity for both you and other developers who may work on the project in the future. In this comprehensive guide, we’ll explore the art of using comments effectively to document and clarify your code, helping you become a more proficient programmer.
1. The Importance of Comments in Code
Before diving into the specifics of how to use comments, let’s first understand why they are so important:
- Code Clarity: Comments help explain complex logic, algorithms, or unconventional approaches, making the code easier to understand.
- Documentation: They serve as inline documentation, providing context and explaining the purpose of specific code blocks or functions.
- Collaboration: In team environments, comments facilitate better collaboration by helping other developers quickly grasp the intent and functionality of the code.
- Maintenance: When revisiting code after a long time, comments can help you (or others) understand the reasoning behind certain decisions, making maintenance and updates easier.
- Debugging: Well-placed comments can aid in the debugging process by providing insights into the expected behavior of the code.
2. Types of Comments
Most programming languages support two main types of comments:
2.1. Single-line Comments
Single-line comments are used for brief explanations or notes that fit on one line. In many programming languages, they start with a specific symbol or set of characters. For example:
// This is a single-line comment in JavaScript, Java, C++, etc.
# This is a single-line comment in Python, Ruby, etc.
2.2. Multi-line Comments
Multi-line comments are used for longer explanations that span multiple lines. The syntax varies between languages, but commonly looks like this:
/*
This is a multi-line comment in JavaScript, Java, C++, etc.
It can span multiple lines and is useful for longer explanations.
*/
"""
This is a multi-line comment in Python.
It uses triple quotes and can also span multiple lines.
"""
3. Best Practices for Using Comments
Now that we understand the types of comments, let’s explore some best practices for using them effectively:
3.1. Be Clear and Concise
Write comments that are easy to understand and to the point. Avoid unnecessary verbosity, but provide enough information to convey the purpose clearly.
// Good: Calculate the average of the array elements
let average = calculateAverage(numbers);
// Bad: This line of code is used to calculate the average of all the elements in the array by calling the calculateAverage function and passing the numbers array as an argument, then storing the result in the average variable
let average = calculateAverage(numbers);
3.2. Comment on the Why, Not the What
Focus on explaining why certain decisions were made or why a particular approach was chosen, rather than simply restating what the code does.
// Good: Use quicksort for large arrays to improve performance
if (array.length > 1000) {
quickSort(array);
} else {
bubbleSort(array);
}
// Bad: If array length is greater than 1000, use quicksort, else use bubble sort
if (array.length > 1000) {
quickSort(array);
} else {
bubbleSort(array);
}
3.3. Use Comments to Explain Complex Logic
When dealing with intricate algorithms or complex business logic, use comments to break down the steps and explain the reasoning.
function calculateCompoundInterest(principal, rate, time, n) {
// Formula: A = P(1 + r/n)^(nt)
// Where:
// A = final amount
// P = principal balance
// r = annual interest rate (in decimal form)
// n = number of times interest is compounded per year
// t = number of years
const amount = principal * Math.pow(1 + (rate / n), n * time);
return amount - principal; // Return only the interest earned
}
3.4. Use TODO Comments
TODO comments are helpful for marking areas of code that need further attention or improvement. They serve as reminders for future tasks.
function processData(data) {
// TODO: Implement data validation before processing
// TODO: Add error handling for edge cases
// Process the data
// ...
}
3.5. Avoid Redundant Comments
Don’t add comments that simply restate what the code obviously does. This adds unnecessary clutter and can make the code harder to read.
// Bad: Increment the counter
counter++;
// Good: No comment needed for simple, self-explanatory operations
3.6. Keep Comments Updated
As code evolves, make sure to update the corresponding comments. Outdated comments can be misleading and cause confusion.
// Bad: Calculate tax for orders over $100
// (Note: The threshold has changed, but the comment wasn't updated)
if (orderTotal > 50) {
calculateTax(orderTotal);
}
// Good: Calculate tax for orders over $50
if (orderTotal > 50) {
calculateTax(orderTotal);
}
4. Documentation Comments
Documentation comments are a special type of comment used to generate automated documentation for functions, classes, and modules. Many programming languages have specific formats for documentation comments that can be processed by documentation generation tools.
4.1. JavaDoc Style (Java, JavaScript)
/**
* Calculates the sum of two numbers.
*
* @param {number} a - The first number.
* @param {number} b - The second number.
* @returns {number} The sum of a and b.
*/
function sum(a, b) {
return a + b;
}
4.2. Python Docstrings
def calculate_area(length, width):
"""
Calculate the area of a rectangle.
Args:
length (float): The length of the rectangle.
width (float): The width of the rectangle.
Returns:
float: The area of the rectangle.
"""
return length * width
5. Using Comments for Code Organization
Comments can also be used to improve the overall structure and organization of your code:
5.1. Section Headers
Use comments to create clear sections within your code, making it easier to navigate and understand the overall structure.
// ===== User Authentication =====
// ... authentication-related functions ...
// ===== Data Processing =====
// ... data processing functions ...
// ===== Utility Functions =====
// ... utility functions ...
5.2. Function Description Blocks
For complex functions, consider adding a description block at the beginning to explain its purpose, parameters, and return values.
/*
* Function: processUserData
* -------------------------
* This function takes raw user data, validates it,
* and transforms it into the format required by the database.
*
* Parameters:
* - userData: An object containing user information
*
* Returns:
* A processed user object ready for database insertion
*/
function processUserData(userData) {
// ... function implementation ...
}
6. Comments in Different Programming Paradigms
The way you use comments may vary depending on the programming paradigm you’re working with:
6.1. Object-Oriented Programming (OOP)
In OOP, use comments to describe classes, methods, and their relationships. Focus on explaining the responsibilities of each class and how they interact.
class BankAccount:
"""
Represents a bank account with basic operations.
Attributes:
account_number (str): The unique account identifier.
balance (float): The current balance of the account.
Methods:
deposit(amount): Adds the specified amount to the balance.
withdraw(amount): Subtracts the specified amount from the balance.
"""
def __init__(self, account_number, initial_balance=0):
self.account_number = account_number
self.balance = initial_balance
def deposit(self, amount):
"""
Deposits money into the account.
Args:
amount (float): The amount to deposit.
Raises:
ValueError: If the amount is negative.
"""
if amount < 0:
raise ValueError("Deposit amount must be positive")
self.balance += amount
# ... other methods ...
6.2. Functional Programming
In functional programming, focus on describing the purpose and behavior of functions, especially for higher-order functions and complex transformations.
// Higher-order function to create a multiplier
const createMultiplier = (factor) => {
// Returns a new function that multiplies its argument by the given factor
return (x) => x * factor;
};
// Create a function that doubles its input
const double = createMultiplier(2);
// Create a function that triples its input
const triple = createMultiplier(3);
// Usage example
console.log(double(5)); // Output: 10
console.log(triple(5)); // Output: 15
7. Comments in Different Programming Languages
While the principles of good commenting remain consistent across languages, the syntax and conventions may vary. Here are some examples:
7.1. Python
# Single-line comment
"""
Multi-line comment or docstring
"""
def example_function():
"""
This is a function docstring.
It describes the purpose and usage of the function.
"""
pass
7.2. JavaScript
// Single-line comment
/*
Multi-line comment
*/
/**
* JSDoc comment for function documentation
* @param {string} name - The name parameter
* @returns {string} A greeting message
*/
function greet(name) {
return `Hello, ${name}!`;
}
7.3. Java
// Single-line comment
/*
Multi-line comment
*/
/**
* Javadoc comment for class or method documentation
* @param args Command line arguments
*/
public static void main(String[] args) {
// Method implementation
}
7.4. C++
// Single-line comment
/*
Multi-line comment
*/
/**
* Doxygen-style comment for function documentation
* @param x The input value
* @return The square of the input
*/
int square(int x) {
return x * x;
}
8. Tools and IDE Features for Comment Management
Many Integrated Development Environments (IDEs) and code editors offer features to help manage and generate comments:
- Auto-generation of function/method comments: IDEs like Visual Studio Code, PyCharm, and IntelliJ IDEA can automatically generate comment templates for functions based on their signatures.
- Comment folding: The ability to collapse and expand comment blocks, making it easier to focus on the code when needed.
- TODO tracking: Many IDEs can track and list all TODO comments in your project, helping you manage tasks and improvements.
- Documentation generation: Tools like JSDoc for JavaScript, Sphinx for Python, and Javadoc for Java can generate comprehensive documentation from properly formatted comments.
9. Common Pitfalls to Avoid
While comments are valuable, there are some common mistakes to avoid:
- Over-commenting: Don’t comment on every line of code. Focus on explaining complex parts or non-obvious decisions.
- Commenting out code: Instead of commenting out large blocks of code, use version control systems to manage different versions of your codebase.
- Misleading comments: Ensure your comments accurately reflect the code’s behavior. Incorrect comments are worse than no comments at all.
- Ignoring naming conventions: Good variable and function names can reduce the need for comments. Use descriptive names to make your code self-documenting where possible.
10. The Role of Comments in Code Reviews
Comments play a crucial role in the code review process:
- Explaining design decisions: Use comments to explain why certain approaches were chosen, making it easier for reviewers to understand your reasoning.
- Highlighting areas of concern: If you’re unsure about a particular implementation, use comments to draw attention to these areas and ask for specific feedback.
- Documenting temporary solutions: If you’ve implemented a temporary fix or workaround, use comments to explain why and what the long-term solution should be.
11. Comments and Code Maintainability
Well-written comments contribute significantly to code maintainability:
- Onboarding new developers: Comments help new team members understand the codebase more quickly.
- Facilitating refactoring: Clear comments make it easier to understand the intent behind the code, which is crucial when refactoring or optimizing.
- Preserving knowledge: Comments can capture important context and decisions that might otherwise be lost when team members change or time passes.
12. Balancing Comments and Self-Documenting Code
While comments are important, it’s equally crucial to strive for self-documenting code:
- Use descriptive variable and function names: Clear names can often convey intent without the need for additional comments.
- Break complex operations into smaller, well-named functions: This can make the code more readable and reduce the need for explanatory comments.
- Use comments to explain “why” rather than “what”: The code itself should show what it’s doing; comments should explain why it’s doing it that way.
Conclusion
Mastering the art of using comments effectively is a valuable skill for any programmer. Comments, when used judiciously, can significantly enhance code readability, maintainability, and collaboration. By following the best practices outlined in this guide, you can improve your coding skills and contribute to creating more robust and understandable software.
Remember, the goal is not to comment every line of code, but to provide clarity where it’s most needed. Strive for a balance between self-documenting code and insightful comments. As you gain experience, you’ll develop a sense for when and how to use comments most effectively in your projects.
Keep practicing, stay consistent with your commenting style, and always consider the future readers of your code – including your future self. With time, you’ll find that well-commented code not only helps others but also makes your own development process smoother and more efficient.