How to Use Command-Line Interfaces in Programming: A Comprehensive Guide
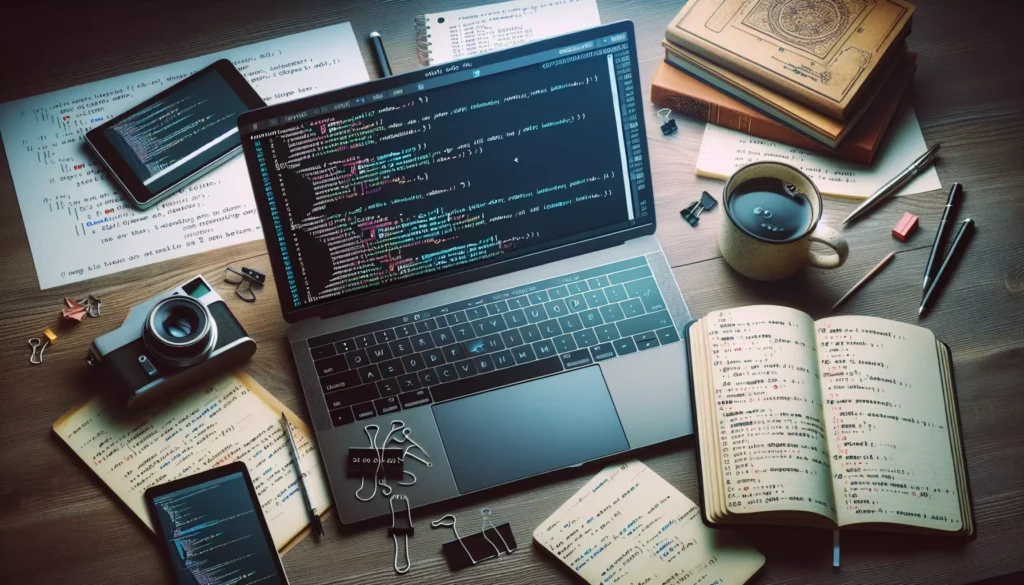
In the world of programming, mastering the command-line interface (CLI) is an essential skill that can significantly boost your productivity and efficiency. Whether you’re a beginner just starting your coding journey or an experienced developer looking to sharpen your skills, understanding how to navigate and utilize the command line is crucial. In this comprehensive guide, we’ll explore the ins and outs of command-line interfaces, their importance in programming, and how to effectively use them in your day-to-day coding tasks.
Table of Contents
- What is a Command-Line Interface?
- The Importance of CLI in Programming
- Basic CLI Commands Every Programmer Should Know
- Advanced CLI Techniques
- Popular CLI Tools for Programmers
- Customizing Your CLI Environment
- Best Practices for Using CLI in Programming
- Troubleshooting Common CLI Issues
- CLI vs. GUI: When to Use Each
- The Future of Command-Line Interfaces
What is a Command-Line Interface?
A command-line interface, often abbreviated as CLI, is a text-based interface used to interact with a computer’s operating system or software applications. Instead of using a graphical user interface (GUI) with buttons and menus, users input text commands to perform various tasks, manipulate files, and control the system.
CLIs are typically accessed through terminal emulators or command prompt applications, depending on the operating system. Some common examples include:
- Terminal (macOS and Linux)
- Command Prompt (Windows)
- PowerShell (Windows)
- Bash (Unix-based systems)
The Importance of CLI in Programming
Command-line interfaces play a crucial role in programming for several reasons:
- Efficiency: CLI commands are typically faster to execute than navigating through a GUI, especially for repetitive tasks.
- Automation: CLIs allow for easy scripting and automation of tasks, saving time and reducing human error.
- Remote Access: CLIs enable remote access and management of servers and systems without the need for a graphical interface.
- Resource Management: CLIs generally use fewer system resources compared to GUIs, making them ideal for resource-constrained environments.
- Precise Control: CLIs offer more granular control over system operations and configurations.
- Development Tools: Many development tools and frameworks are primarily or exclusively accessed through the command line.
Basic CLI Commands Every Programmer Should Know
Regardless of your programming language or development environment, there are several basic CLI commands that every programmer should be familiar with:
1. Navigation Commands
cd
– Change directoryls
(Unix) ordir
(Windows) – List directory contentspwd
– Print working directory
2. File Management Commands
cp
– Copy files or directoriesmv
– Move or rename files or directoriesrm
– Remove files or directoriesmkdir
– Create a new directorytouch
(Unix) orecho.
(Windows) – Create an empty file
3. Text Manipulation Commands
cat
(Unix) ortype
(Windows) – Display file contentsgrep
(Unix) orfindstr
(Windows) – Search for patterns in filesecho
– Print text to the console
4. System Commands
sudo
(Unix) – Execute a command with superuser privilegeschmod
(Unix) – Change file permissionsps
(Unix) ortasklist
(Windows) – List running processes
Example: Navigating and Creating a New Project Directory
Let’s walk through a simple example of using CLI commands to create a new project directory:
# Navigate to the desktop
cd ~/Desktop
# Create a new directory for your project
mkdir my_new_project
# Move into the new directory
cd my_new_project
# Create some empty files
touch index.html style.css script.js
# List the contents of the directory
ls
# Output:
# index.html style.css script.js
Advanced CLI Techniques
Once you’ve mastered the basics, there are several advanced techniques that can further enhance your CLI proficiency:
1. Piping and Redirection
Piping (|
) allows you to chain commands together, while redirection (>
, >>
, <
) enables you to control input and output streams.
Example:
# Count the number of files in a directory
ls | wc -l
# Append the output of a command to a file
echo "New line of text" >> myfile.txt
2. Command Substitution
Command substitution allows you to use the output of one command as an argument for another command.
Example:
# Delete all files created more than 7 days ago
find . -type f -mtime +7 -exec rm {} \;
3. Regular Expressions
Many CLI tools support regular expressions for powerful pattern matching and text manipulation.
Example:
# Find all Python files containing the word "import"
grep -r "import" *.py
4. Shell Scripting
Shell scripting allows you to create reusable scripts to automate complex tasks.
Example (Bash script):
#!/bin/bash
# Script to create a new Python project structure
project_name=$1
mkdir $project_name
cd $project_name
mkdir src tests docs
touch src/__init__.py src/main.py tests/__init__.py docs/README.md
echo "# $project_name" > README.md
echo "Project structure created successfully!"
Popular CLI Tools for Programmers
There are numerous CLI tools that can significantly enhance your productivity as a programmer. Here are some popular ones:
1. Version Control Systems
- Git: The most widely used distributed version control system.
Example Git commands:
# Initialize a new Git repository
git init
# Add files to staging
git add .
# Commit changes
git commit -m "Initial commit"
# Push changes to a remote repository
git push origin main
2. Package Managers
- npm: Package manager for JavaScript and Node.js
- pip: Package installer for Python
- Composer: Dependency manager for PHP
Example npm commands:
# Initialize a new Node.js project
npm init -y
# Install a package
npm install express
# Run a script defined in package.json
npm run start
3. Build Tools
- Make: A build automation tool
- Gradle: Build automation tool for Java projects
Example Makefile:
CC = gcc
CFLAGS = -Wall -g
all: myprogram
myprogram: main.o helper.o
$(CC) $(CFLAGS) -o myprogram main.o helper.o
main.o: main.c
$(CC) $(CFLAGS) -c main.c
helper.o: helper.c
$(CC) $(CFLAGS) -c helper.c
clean:
rm -f *.o myprogram
4. Text Editors and IDEs
- Vim: A highly configurable text editor
- Emacs: An extensible, customizable text editor
Example Vim commands:
# Open a file in Vim
vim myfile.txt
# Enter insert mode
i
# Save changes and exit
:wq
# Search for a pattern
/pattern
Customizing Your CLI Environment
Customizing your CLI environment can greatly improve your workflow and productivity. Here are some ways to personalize your command-line experience:
1. Shell Configuration Files
Customize your shell by modifying configuration files such as .bashrc
, .zshrc
, or .profile
. These files allow you to set environment variables, define aliases, and configure your prompt.
Example .bashrc
customizations:
# Set custom prompt
PS1="\u@\h:\w\$ "
# Define aliases
alias ll="ls -la"
alias gst="git status"
# Add custom directory to PATH
export PATH=$PATH:/path/to/custom/directory
2. Color Schemes
Many terminal emulators support custom color schemes to improve readability and aesthetics. You can often find pre-made color schemes or create your own.
3. Command-line Completion
Enable and customize command-line completion to speed up your typing and reduce errors. Many shells offer built-in completion features that can be further enhanced with additional tools.
4. Custom Scripts and Functions
Create your own scripts and functions to automate repetitive tasks or add new functionality to your CLI environment.
Example custom function (in .bashrc
or .zshrc
):
# Function to create a new directory and navigate into it
function mkcd() {
mkdir -p "$1" && cd "$1"
}
Best Practices for Using CLI in Programming
To make the most of command-line interfaces in your programming workflow, consider the following best practices:
- Learn keyboard shortcuts: Familiarize yourself with common keyboard shortcuts to navigate and edit commands more efficiently.
- Use tab completion: Leverage tab completion to save time and avoid typos when entering commands or file names.
- Understand command options: Read man pages or use the
--help
flag to understand the various options available for each command. - Create aliases for common tasks: Define aliases for frequently used commands or command combinations to save time.
- Use version control: Integrate version control systems like Git into your CLI workflow to manage your code effectively.
- Practice safe command execution: Be cautious when running commands with elevated privileges or that modify system files.
- Document your CLI workflows: Keep notes or create documentation for complex CLI procedures to help yourself and others in the future.
- Explore new tools: Continuously explore and learn about new CLI tools that can enhance your productivity.
Troubleshooting Common CLI Issues
Even experienced programmers encounter issues when working with command-line interfaces. Here are some common problems and their solutions:
1. Command Not Found
If you receive a “command not found” error, check the following:
- Verify that the command is installed on your system.
- Ensure that the command’s location is in your system’s PATH.
- Check for typos in the command name.
2. Permission Denied
When encountering “permission denied” errors:
- Check if you have the necessary permissions to execute the command or access the file/directory.
- Use
sudo
(on Unix-based systems) to run the command with elevated privileges, if appropriate. - Modify file permissions using
chmod
if necessary.
3. Unexpected Command Behavior
If a command doesn’t behave as expected:
- Double-check the command syntax and options.
- Consult the command’s manual page or online documentation.
- Try running the command with verbose or debug options for more information.
4. Shell Script Errors
When troubleshooting shell scripts:
- Use
set -x
at the beginning of your script to enable debug mode. - Check for syntax errors and proper quoting.
- Verify that the script has the correct permissions to execute.
CLI vs. GUI: When to Use Each
While command-line interfaces offer many advantages, there are situations where graphical user interfaces (GUIs) may be more appropriate. Understanding when to use each can help you work more efficiently:
Use CLI when:
- Performing repetitive tasks that can be easily automated
- Working with remote servers or systems without graphical interfaces
- Executing complex operations that require precise control
- Dealing with large amounts of data or files
- Scripting and automation are required
- System resources are limited
Use GUI when:
- Performing visual tasks, such as image or video editing
- Working with complex graphical applications (e.g., 3D modeling software)
- Exploring unfamiliar systems or tools where visual cues are helpful
- Presenting information to non-technical users
- Multitasking with multiple windows or applications
In many cases, a combination of both CLI and GUI tools can provide the best workflow, leveraging the strengths of each interface type.
The Future of Command-Line Interfaces
As technology continues to evolve, command-line interfaces are also adapting to meet the changing needs of programmers and system administrators. Some trends and developments in the future of CLIs include:
1. AI-Powered CLIs
Artificial intelligence and machine learning are being integrated into CLI tools to provide smarter suggestions, autocompletion, and even natural language processing capabilities.
2. Cross-Platform Compatibility
With the increasing popularity of cross-platform development, CLI tools are becoming more consistent across different operating systems, making it easier for developers to work in diverse environments.
3. Enhanced Visualization
Modern CLIs are incorporating more visual elements, such as progress bars, charts, and even basic graphical outputs, to provide a more informative and user-friendly experience without sacrificing the efficiency of text-based interfaces.
4. Integration with Cloud Services
As cloud computing continues to grow, CLI tools are evolving to better integrate with cloud services, allowing developers to manage and interact with cloud resources directly from the command line.
5. Improved Security Features
With increasing concerns about cybersecurity, CLI tools are incorporating more advanced security features, such as built-in encryption, secure credential management, and enhanced auditing capabilities.
Conclusion
Mastering command-line interfaces is an invaluable skill for any programmer. By understanding the basics, exploring advanced techniques, and leveraging powerful CLI tools, you can significantly enhance your productivity and efficiency in software development. As you continue to grow as a programmer, remember to stay curious, explore new CLI tools and techniques, and adapt your workflow to make the most of these powerful text-based interfaces.
Whether you’re just starting your coding journey or preparing for technical interviews at major tech companies, developing your CLI skills will serve you well throughout your career. Embrace the command line, and unlock a new level of control and efficiency in your programming endeavors.