How to Use Coding Challenges to Improve Your Skills
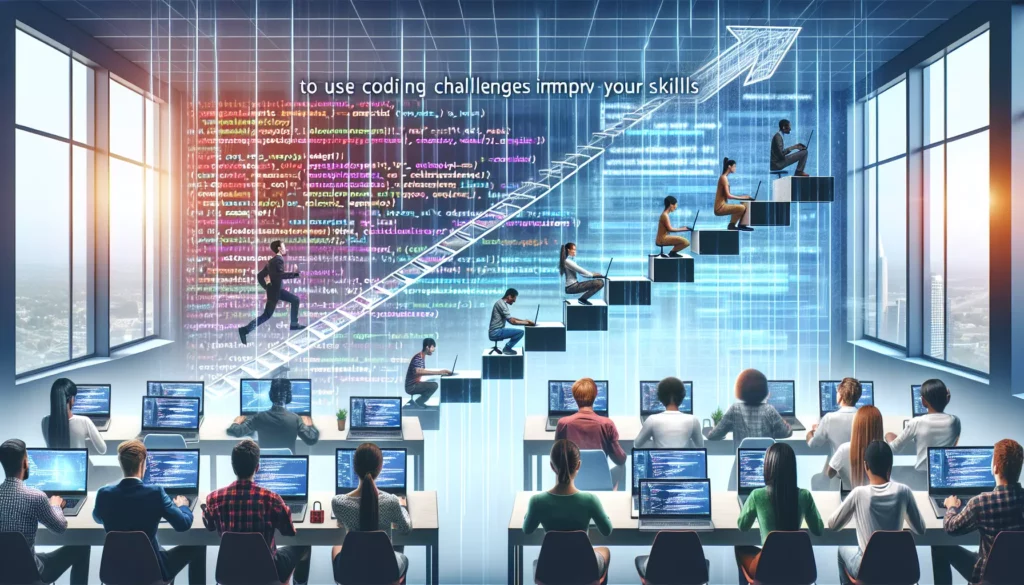
In the ever-evolving world of technology, staying sharp and continually improving your coding skills is crucial for success. Whether you’re a beginner looking to establish a solid foundation or an experienced developer aiming to crack those coveted FAANG (Facebook, Amazon, Apple, Netflix, Google) interviews, coding challenges can be an invaluable tool in your learning arsenal. This comprehensive guide will explore how to effectively use coding challenges to enhance your programming prowess, problem-solving abilities, and overall technical acumen.
Understanding the Value of Coding Challenges
Before diving into the strategies for using coding challenges, it’s essential to understand why they’re so beneficial:
- Practical Application: Challenges allow you to apply theoretical knowledge in real-world scenarios.
- Problem-Solving Skills: They enhance your ability to break down complex problems into manageable parts.
- Algorithm Proficiency: Regular practice improves your understanding and implementation of various algorithms.
- Time Management: Many challenges are timed, helping you work efficiently under pressure.
- Interview Preparation: Challenges often mimic the types of problems asked in technical interviews.
- Language Mastery: You can reinforce your knowledge of programming languages and their nuances.
Getting Started with Coding Challenges
If you’re new to coding challenges or looking to create a structured approach, here are some steps to get you started:
1. Choose the Right Platform
There are numerous platforms available for coding challenges. Some popular options include:
- AlgoCademy: Offers interactive tutorials and AI-powered assistance.
- LeetCode: Known for its extensive problem set and interview preparation focus.
- HackerRank: Provides a wide range of challenges across multiple domains.
- CodeWars: Features a unique ranking system and community-contributed challenges.
- Project Euler: Focuses on mathematical-oriented programming problems.
Choose a platform that aligns with your goals and learning style. AlgoCademy, for instance, is an excellent choice for those looking for guided learning with step-by-step tutorials and AI assistance.
2. Assess Your Current Skill Level
Most platforms offer challenges of varying difficulty levels. Start by taking an honest assessment of your skills:
- Beginner: Focus on basic syntax, simple algorithms, and fundamental data structures.
- Intermediate: Tackle more complex algorithms, efficiency optimization, and advanced data structures.
- Advanced: Dive into system design, complex problem-solving, and highly optimized solutions.
3. Create a Study Plan
Consistency is key when it comes to improving your coding skills. Develop a study plan that fits your schedule:
- Set aside dedicated time each day or week for coding challenges.
- Start with easier problems and gradually increase difficulty.
- Focus on specific topics or algorithms for a set period before moving on.
- Use spaced repetition to revisit and reinforce previously learned concepts.
Strategies for Maximizing Learning from Coding Challenges
Now that you’ve got the basics down, let’s explore some strategies to get the most out of your coding challenge practice:
1. Understand the Problem Thoroughly
Before writing a single line of code, make sure you fully understand the problem statement:
- Read the problem multiple times if necessary.
- Identify the input format and expected output.
- Look for any constraints or edge cases mentioned.
- If provided, analyze the sample inputs and outputs.
2. Plan Your Approach
Resist the urge to start coding immediately. Instead, take time to plan your approach:
- Break down the problem into smaller, manageable steps.
- Consider multiple solutions and their trade-offs.
- Sketch out your algorithm on paper or a whiteboard.
- Think about potential edge cases and how to handle them.
3. Implement Your Solution
Once you have a solid plan, start implementing your solution:
- Write clean, readable code with proper indentation and naming conventions.
- Add comments to explain your thought process and any complex logic.
- Test your code with the provided sample inputs and your own test cases.
- Debug and refine your solution as needed.
4. Optimize and Refactor
After getting a working solution, consider ways to improve it:
- Analyze the time and space complexity of your solution.
- Look for opportunities to optimize algorithms or data structures.
- Refactor your code to make it more efficient or readable.
- Consider alternative approaches that might be more elegant or performant.
5. Learn from Others
One of the most valuable aspects of coding challenges is the opportunity to learn from others:
- After submitting your solution, review other users’ submissions.
- Analyze solutions that are more efficient or elegant than yours.
- Participate in discussion forums to share insights and ask questions.
- Contribute by explaining your approach to help others learn.
6. Review and Reflect
To solidify your learning, take time to review and reflect on each challenge:
- Summarize the key concepts or algorithms used in the problem.
- Note any new techniques or tricks you learned.
- Identify areas where you struggled and plan to focus on them in future practice.
- Keep a log of completed challenges and your progress over time.
Advanced Techniques for Coding Challenge Mastery
As you become more comfortable with coding challenges, consider these advanced techniques to further enhance your skills:
1. Time-Boxed Problem Solving
Simulate interview conditions by setting a time limit for each challenge:
- Start with 45-60 minutes per problem and adjust based on difficulty.
- Practice explaining your thought process out loud as you solve the problem.
- Learn to manage your time effectively, balancing planning and implementation.
2. Implement Multiple Solutions
Don’t stop at your first working solution:
- Try to come up with at least two different approaches to each problem.
- Implement solutions using different algorithms or data structures.
- Compare the trade-offs between different solutions in terms of time complexity, space complexity, and readability.
3. Deep Dive into Algorithms and Data Structures
Use coding challenges as a springboard for deeper learning:
- When you encounter a new algorithm or data structure, spend time studying its principles and applications.
- Implement common algorithms and data structures from scratch to understand their inner workings.
- Explore variations and optimizations of classic algorithms.
4. Focus on Specific Topics
Dedicate periods to mastering specific areas:
- Spend a week focusing on graph algorithms, then move to dynamic programming, and so on.
- Create custom problem sets on platforms like AlgoCademy to target your weak areas.
- Revisit topics periodically to reinforce your knowledge and track improvement.
5. Participate in Coding Competitions
Take your skills to the next level by participating in coding competitions:
- Join platforms like Codeforces or TopCoder for regular contests.
- Participate in hackathons to apply your skills in a team setting.
- Analyze your performance in competitions to identify areas for improvement.
Leveraging AI-Powered Assistance
Platforms like AlgoCademy offer AI-powered assistance, which can be a game-changer in your learning journey:
1. Personalized Hints and Guidance
AI can provide tailored hints when you’re stuck:
- Get suggestions on which data structures or algorithms might be useful.
- Receive step-by-step guidance without seeing the full solution.
- Learn to ask the right questions to prompt helpful AI responses.
2. Code Analysis and Feedback
Utilize AI to analyze your code:
- Get instant feedback on code efficiency and style.
- Receive suggestions for optimization and best practices.
- Learn to write more readable and maintainable code.
3. Customized Learning Paths
AI can help create personalized learning experiences:
- Receive recommendations for challenges that target your weak areas.
- Get suggestions for related problems to reinforce concepts.
- Track your progress and adjust your learning path accordingly.
Common Pitfalls to Avoid
As you embark on your journey of using coding challenges to improve your skills, be aware of these common pitfalls:
1. Memorizing Solutions
While it’s important to learn from others’ solutions, avoid the temptation to simply memorize them:
- Focus on understanding the underlying principles and thought processes.
- Try to solve problems on your own before looking at solutions.
- If you do study a solution, try to implement it from memory later.
2. Neglecting Fundamentals
Don’t get so caught up in solving complex problems that you neglect the basics:
- Regularly review and practice fundamental algorithms and data structures.
- Ensure you have a solid understanding of time and space complexity analysis.
- Don’t shy away from “easy” problems – they can often teach valuable lessons.
3. Focusing Too Narrowly
While specialization is important, don’t neglect breadth in your learning:
- Explore a variety of problem types and domains.
- Practice with different programming languages to broaden your perspective.
- Stay updated with industry trends and new technologies.
4. Burnout from Overcommitment
Consistency is key, but be careful not to overcommit:
- Set realistic goals that fit your schedule and lifestyle.
- Take breaks and allow time for the concepts to sink in.
- Balance coding challenges with other aspects of your learning and life.
Applying Your Skills Beyond Challenges
Remember that coding challenges are a means to an end, not the end itself. Apply your improved skills in real-world scenarios:
1. Personal Projects
Use your enhanced problem-solving skills to build personal projects:
- Apply algorithms and data structures you’ve learned to solve real problems.
- Build applications that showcase your ability to write efficient and scalable code.
- Open-source your projects to get feedback from the community.
2. Contribute to Open Source
Put your skills to the test by contributing to open-source projects:
- Look for projects that align with your interests and skill level.
- Start with small contributions like bug fixes or documentation improvements.
- Gradually take on more complex issues as your confidence grows.
3. Mentor Others
Teaching is one of the best ways to solidify your own knowledge:
- Offer to mentor junior developers or students.
- Explain concepts and solutions on forums or your personal blog.
- Create tutorials or video content to share your knowledge.
Conclusion
Coding challenges are a powerful tool for improving your programming skills, preparing for technical interviews, and becoming a more well-rounded developer. By following the strategies outlined in this guide and leveraging platforms like AlgoCademy with their AI-powered assistance, you can create a structured and effective learning path.
Remember that improvement is a journey, not a destination. Embrace the process of continuous learning, be patient with yourself, and celebrate your progress along the way. Whether you’re aiming to land a job at a top tech company or simply become the best developer you can be, consistent practice with coding challenges will help you achieve your goals.
Start today, stay consistent, and watch as your problem-solving abilities and coding skills soar to new heights. Happy coding!