How to Turn Your Code Refactoring into an Olympic Sport
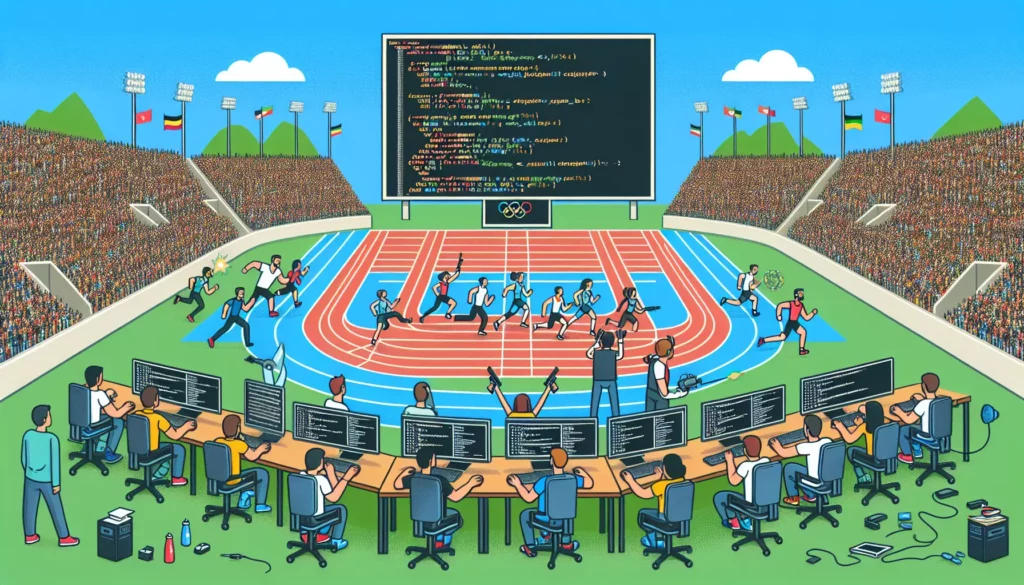
In the world of software development, code refactoring is often seen as a necessary evil – a tedious task that developers grudgingly undertake to improve the quality and maintainability of their codebase. But what if we could transform this seemingly mundane activity into something more exciting, more competitive, and dare we say, more Olympic? Welcome to the world of extreme code refactoring, where clean code is the ultimate goal and efficiency is the name of the game.
The Importance of Code Refactoring
Before we dive into the Olympic-worthy techniques of code refactoring, let’s remind ourselves why this practice is so crucial in software development:
- Improved Code Readability: Refactoring makes code easier to understand and maintain.
- Enhanced Efficiency: Well-refactored code often runs faster and uses fewer resources.
- Better Scalability: Clean code is easier to extend and modify as project requirements evolve.
- Reduced Technical Debt: Regular refactoring prevents the accumulation of “quick fixes” that can lead to long-term issues.
- Easier Debugging: Cleaner code makes it simpler to identify and fix bugs.
Now that we’ve established the importance of refactoring, let’s explore how we can elevate this practice to Olympic levels.
Training for the Code Refactoring Olympics
Just like any Olympic sport, becoming a champion code refactorer requires dedication, practice, and the right training regimen. Here’s how you can start your journey to code refactoring greatness:
1. Master the Fundamentals
Every Olympic athlete starts with the basics, and code refactoring is no different. Begin by familiarizing yourself with common refactoring techniques such as:
- Extracting Methods
- Renaming Variables
- Simplifying Conditional Expressions
- Removing Duplicate Code
- Introducing Design Patterns
Practice these techniques regularly on small code snippets to build your refactoring muscles.
2. Develop Your “Code Sense”
Olympic athletes often talk about being “in the zone” – that state of heightened awareness where everything just clicks. In code refactoring, developing your “code sense” is similar. It’s the ability to look at a piece of code and instinctively know what needs improvement. To develop this skill:
- Read lots of code, both good and bad
- Analyze open-source projects
- Participate in code reviews
- Practice identifying code smells
3. Set Personal Records (PRs)
Every athlete strives to beat their personal best. In code refactoring, you can set PRs for:
- Number of lines reduced in a single refactoring session
- Time taken to refactor a specific module
- Improvement in code complexity metrics (e.g., cyclomatic complexity)
- Number of design patterns successfully implemented
Keep track of these metrics and constantly push yourself to improve.
4. Cross-Train with Different Languages and Paradigms
Olympic athletes often cross-train to improve their overall performance. Similarly, exposing yourself to different programming languages and paradigms can make you a more versatile refactorer. Try refactoring:
- Object-oriented code in Java or C#
- Functional code in Haskell or Scala
- Dynamic code in Python or Ruby
- Low-level code in C or Rust
Each paradigm will teach you new techniques and perspectives that you can apply across your refactoring practice.
The Code Refactoring Decathlon
Now that you’ve trained like an Olympian, it’s time to put your skills to the test. Welcome to the Code Refactoring Decathlon, a series of challenges designed to push your refactoring abilities to the limit. Here are the events:
1. The Sprint: Quick Fixes
In this event, you’ll be given a small piece of code with obvious issues. Your task is to improve it as much as possible in just 5 minutes. This tests your ability to quickly identify and resolve the most pressing problems.
Example challenge:
function calculateTotal(items) {
var total = 0;
for (var i = 0; i < items.length; i++) {
var item = items[i];
total = total + item.price * item.quantity;
}
return total;
}
Can you refactor this to use modern JavaScript features and improve readability in under 5 minutes?
2. The Marathon: Large-Scale Refactoring
This event tests your endurance. You’ll be given a large, complex codebase and tasked with improving its overall structure and design over an extended period. Points are awarded for improvements in:
- Code modularity
- Adherence to SOLID principles
- Reduction in code duplication
- Improvement in test coverage
3. The Relay: Team Refactoring
Software development is often a team sport, and so is this event. Teams of 4 developers work together to refactor a shared codebase. Each team member has a specific role:
- The Architect: Focuses on high-level design improvements
- The Optimizer: Targets performance enhancements
- The Cleaner: Improves code readability and documentation
- The Tester: Ensures refactoring doesn’t break existing functionality
Success in this event requires not just technical skill, but also effective communication and collaboration.
4. The Hurdles: Refactoring with Constraints
In this challenging event, you must refactor code while adhering to specific constraints. For example:
- Improve performance without changing the public API
- Reduce code size by 30% without losing functionality
- Refactor using only pure functions
- Improve readability without changing any variable names
This event tests your creativity and ability to work within limitations.
5. The High Jump: Pattern Implementation
Here, you’ll be given a piece of code that could benefit from a specific design pattern. Your task is to refactor the code to implement the pattern correctly and elegantly. Patterns might include:
- Factory Method
- Observer
- Strategy
- Decorator
Points are awarded for correct implementation, code cleanliness, and appropriate use of the pattern.
6. The Long Jump: Code Modernization
In this event, you’ll be working with legacy code. Your task is to modernize it, bringing it up to current best practices and language features. This might involve:
- Converting callback-based code to use Promises or async/await
- Updating old JavaScript to use ES6+ features
- Migrating from older frameworks to newer ones
- Implementing type safety with TypeScript
7. The Javelin: Error Handling Improvement
This event focuses on improving error handling and overall code robustness. You’ll be given code with poor or non-existent error handling, and your task is to:
- Implement proper error checking
- Add informative error messages
- Ensure all errors are properly caught and logged
- Implement retry mechanisms where appropriate
8. The Discus: Dead Code Elimination
In this event, you’ll be working with a codebase bloated with unused functions, commented-out code, and unnecessary dependencies. Your goal is to streamline the codebase by:
- Identifying and removing unused code
- Eliminating redundant dependencies
- Consolidating similar functions
- Improving overall code organization
9. The Pole Vault: Performance Optimization
This event is all about making code run faster and more efficiently. You’ll be given a piece of poorly performing code, and your task is to optimize it. This might involve:
- Improving algorithm efficiency
- Optimizing database queries
- Implementing caching strategies
- Reducing unnecessary computations
10. The Triathlon: Full-Stack Refactoring
The final and most challenging event. You’ll be working with a full-stack application, and your task is to improve it across all layers:
- Frontend: Improve component structure and state management
- Backend: Enhance API design and data flow
- Database: Optimize schema and query performance
This event tests your ability to see the big picture and make improvements that span the entire application stack.
Training Tools for the Code Refactoring Olympian
Every Olympic athlete needs the right equipment to perform at their best. Here are some essential tools for the aspiring code refactoring champion:
1. Integrated Development Environments (IDEs)
A good IDE is like a well-equipped gym for a code refactorer. Look for IDEs with strong refactoring support, such as:
- JetBrains IDEs (IntelliJ IDEA, PyCharm, WebStorm)
- Visual Studio Code with refactoring extensions
- Eclipse with refactoring plugins
These IDEs offer features like automated refactoring, code analysis, and quick fixes that can significantly speed up your refactoring process.
2. Static Code Analysis Tools
These tools are like your personal coaches, pointing out areas of improvement in your code. Some popular options include:
- SonarQube
- ESLint for JavaScript
- RuboCop for Ruby
- Pylint for Python
Use these tools to identify code smells, potential bugs, and areas where refactoring could be beneficial.
3. Version Control Systems
Every refactoring Olympian needs a safety net. Version control systems like Git allow you to experiment with refactoring while always having the ability to revert changes if needed. Learn to use features like:
- Branching for isolated refactoring experiments
- Interactive rebasing for cleaning up commit history
- Pull requests for team review of refactoring changes
4. Profiling Tools
For events focused on performance optimization, profiling tools are essential. They help you identify bottlenecks and measure the impact of your refactoring efforts. Some options include:
- Chrome DevTools for JavaScript performance
- VisualVM for Java applications
- cProfile for Python
5. Refactoring Books and Resources
Every athlete needs to study their sport. Some essential reading for the refactoring Olympian includes:
- “Refactoring: Improving the Design of Existing Code” by Martin Fowler
- “Clean Code: A Handbook of Agile Software Craftsmanship” by Robert C. Martin
- “Working Effectively with Legacy Code” by Michael Feathers
6. Online Coding Platforms
Practice makes perfect. Use online coding platforms to sharpen your skills:
- LeetCode for algorithm challenges
- HackerRank for language-specific practice
- CodeWars for refactoring katas
The Refactoring Olympian’s Mindset
Becoming a champion code refactorer isn’t just about technical skills; it’s also about adopting the right mindset. Here are some key attitudes to cultivate:
1. Continuous Improvement
Like any Olympic athlete, a refactoring champion is never satisfied with “good enough.” Always look for ways to improve your code, your skills, and your processes.
2. Attention to Detail
In refactoring, small changes can have big impacts. Develop a keen eye for detail and strive for precision in your work.
3. Patience and Persistence
Refactoring complex systems takes time. Be patient with the process and persistent in the face of challenges.
4. Openness to Feedback
Be open to code reviews and feedback from peers. Every critique is an opportunity to improve your refactoring skills.
5. Holistic Thinking
Consider the broader impact of your refactoring efforts. How do they affect the overall system architecture, performance, and maintainability?
Conclusion: The Path to Refactoring Gold
Turning code refactoring into an Olympic sport may seem like a stretch, but approaching it with the dedication, skill, and competitive spirit of an athlete can transform this essential practice from a chore into an exciting challenge. By mastering the fundamentals, continuously pushing your limits, and using the right tools and techniques, you can elevate your refactoring skills to world-class levels.
Remember, the ultimate goal of this “sport” isn’t just personal glory – it’s about creating cleaner, more efficient, and more maintainable code that benefits your entire team and project. So put on your refactoring jersey, warm up those coding fingers, and get ready to go for the gold in the exciting world of extreme code refactoring!
As you embark on your journey to become a refactoring Olympian, keep in mind that this is a lifelong pursuit. The world of software development is constantly evolving, with new languages, frameworks, and best practices emerging all the time. Embrace this continuous learning process, and you’ll find that your refactoring skills will not only improve your code but also make you a more versatile and valuable developer overall.
So, are you ready to take your code refactoring to Olympic levels? Start training today, and who knows – you might just find yourself on the podium, accepting a gold medal for the cleanest, most elegant code refactoring the world has ever seen. Let the games begin!