How to Turn Your Code Frustration Into a Project Success Story
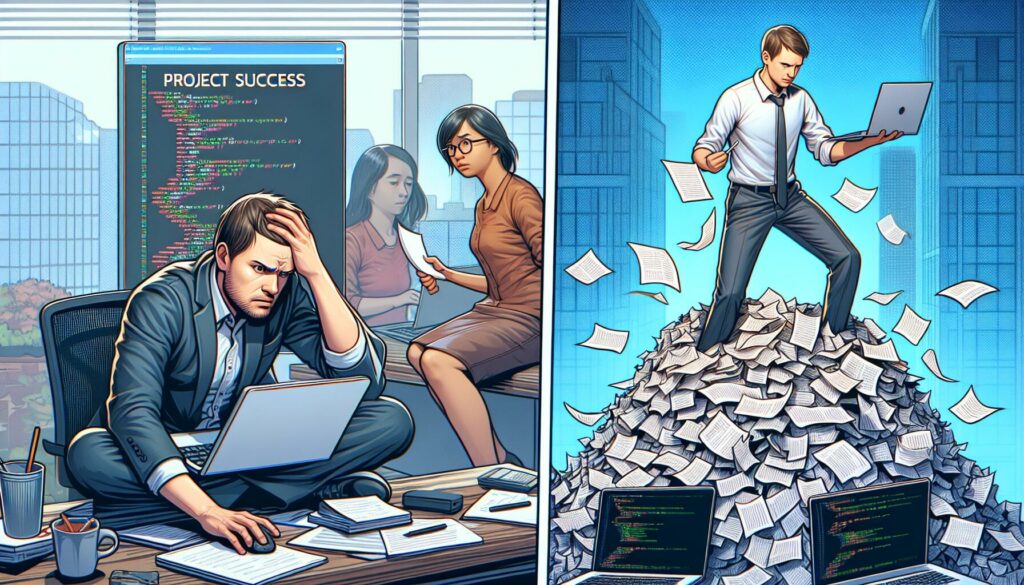
Every programmer, from novice to expert, has experienced moments of frustration when coding. Whether it’s a pesky bug that won’t go away, a concept that’s hard to grasp, or a project that seems insurmountable, coding challenges can sometimes feel overwhelming. However, these moments of frustration don’t have to define your coding journey. In fact, they can be the stepping stones to your next big success story. In this comprehensive guide, we’ll explore how to transform your coding frustrations into triumphs, drawing on strategies and insights that align with AlgoCademy’s mission of empowering coders at all levels.
1. Embrace the Learning Process
The first step in turning frustration into success is to reframe your mindset. Coding is a journey of continuous learning, and every obstacle is an opportunity for growth.
Recognize That Challenges Are Normal
Even the most experienced programmers face difficulties. What sets successful coders apart is their ability to persevere through these challenges. Remember, if you’re feeling frustrated, it means you’re pushing your boundaries and learning something new.
Set Realistic Expectations
Rome wasn’t built in a day, and neither is programming expertise. Set achievable goals for yourself and celebrate small victories along the way. This approach aligns with AlgoCademy’s step-by-step learning methodology, which breaks down complex concepts into manageable chunks.
2. Develop a Structured Problem-Solving Approach
When faced with a coding challenge, having a systematic approach can make all the difference. AlgoCademy emphasizes the importance of algorithmic thinking, which is crucial for solving complex problems efficiently.
Break Down the Problem
Large, complex problems can be intimidating. Break them down into smaller, more manageable tasks. This not only makes the problem less daunting but also allows you to tackle it piece by piece.
Use Pseudocode
Before diving into actual coding, write out your logic in plain language. This helps clarify your thinking and can reveal potential issues before you even start typing. Here’s an example of how you might use pseudocode to plan a simple sorting algorithm:
// Pseudocode for Bubble Sort
FOR each element in the list
FOR each adjacent pair of elements
IF the first element is greater than the second
SWAP the elements
END IF
END FOR
END FOR
Implement, Test, and Refine
Once you have a plan, start implementing it in code. Test your solution frequently, and be prepared to refine your approach based on the results. This iterative process is key to problem-solving in programming.
3. Leverage Resources and Tools
No programmer is an island. Successful coders know how to make the most of the resources available to them.
Use Documentation and Official Resources
Official documentation is your best friend when learning a new language or framework. It’s often the most accurate and up-to-date source of information. Make a habit of referring to it regularly.
Explore Online Communities
Platforms like Stack Overflow, GitHub, and Reddit have thriving programming communities. Don’t hesitate to ask questions or share your knowledge. Remember, even the act of formulating a clear question can often lead you to the solution.
Utilize AI-Powered Assistance
AI tools, like those offered by AlgoCademy, can provide personalized guidance and help you understand complex concepts. These tools can offer explanations, suggest optimizations, and even help debug your code.
4. Practice Debugging Techniques
Debugging is an essential skill for any programmer. The ability to efficiently identify and fix errors can turn a frustrating experience into a valuable learning opportunity.
Use Print Statements Strategically
Sometimes, the simplest methods are the most effective. Adding print statements to your code can help you understand the flow of your program and identify where things are going wrong. For example:
def calculate_sum(numbers):
print("Starting calculation")
total = 0
for num in numbers:
print(f"Adding {num} to total")
total += num
print(f"Final total: {total}")
return total
result = calculate_sum([1, 2, 3, 4, 5])
print(f"Result: {result}")
Learn to Use a Debugger
While print statements are useful, learning to use a debugger can significantly speed up your debugging process. Most modern IDEs come with built-in debuggers that allow you to step through your code line by line, inspect variables, and set breakpoints.
Read Error Messages Carefully
Error messages can be intimidating, but they often contain valuable information about what went wrong and where. Take the time to read and understand them. If you’re unsure about an error message, don’t hesitate to search for it online or ask for help in coding communities.
5. Implement Time Management Techniques
Effective time management can help prevent burnout and maintain a steady pace of progress, even when faced with challenging projects.
Use the Pomodoro Technique
The Pomodoro Technique involves working in focused 25-minute intervals, followed by short breaks. This can help maintain concentration and prevent mental fatigue during long coding sessions.
Set SMART Goals
SMART goals are Specific, Measurable, Achievable, Relevant, and Time-bound. Instead of a vague goal like “learn Python,” set a SMART goal like “Complete AlgoCademy’s Python fundamentals course and solve 20 related practice problems within the next month.”
Practice Time Boxing
Allocate specific time slots for different tasks. This can help prevent you from getting stuck on a single problem for too long. If you can’t solve a problem within the allocated time, take a break or move on to something else before coming back to it with fresh eyes.
6. Cultivate a Growth Mindset
A growth mindset is the belief that abilities can be developed through dedication and hard work. This perspective is crucial for long-term success in programming.
View Mistakes as Learning Opportunities
Every bug you encounter, every error you make, is a chance to learn something new. Instead of getting discouraged, ask yourself, “What can I learn from this?”
Embrace Challenges
Seek out coding challenges that push you out of your comfort zone. Platforms like AlgoCademy offer a variety of problems designed to stretch your skills and prepare you for real-world coding scenarios.
Celebrate Progress, Not Just Perfection
Acknowledge the progress you’ve made, no matter how small. Did you finally understand a concept that’s been troubling you? That’s worth celebrating! Recognizing your growth can help maintain motivation during challenging times.
7. Build a Support Network
Having a support network can make a significant difference in your coding journey, providing encouragement, knowledge sharing, and accountability.
Join Coding Groups or Bootcamps
Participating in coding groups or bootcamps can provide structure to your learning and connect you with peers facing similar challenges. Many of these groups offer regular meetups, coding sessions, and opportunities to work on collaborative projects.
Find a Mentor
A mentor can provide guidance, share their experiences, and offer valuable insights into the industry. Look for mentorship opportunities within your workplace, educational institution, or online communities.
Engage in Pair Programming
Pair programming involves two programmers working together at one workstation. This practice can help you learn new techniques, catch errors more quickly, and improve your communication skills. Even if you’re learning remotely, many online platforms facilitate virtual pair programming sessions.
8. Focus on Project-Based Learning
Building projects is one of the most effective ways to apply your skills, learn new technologies, and create tangible results that can boost your confidence and motivation.
Start with Small Projects
Begin with manageable projects that align with your current skill level. As you complete these, you’ll gain confidence and be better prepared to tackle larger, more complex projects.
Contribute to Open Source
Contributing to open-source projects is a great way to gain real-world experience, collaborate with other developers, and build your portfolio. Start by looking for projects labeled “beginner-friendly” or “good first issue” on platforms like GitHub.
Build Projects That Solve Real Problems
Focus on creating projects that solve problems you or others face. This not only makes your learning more engaging but also results in projects that can showcase your skills to potential employers.
9. Develop Effective Learning Habits
Establishing good learning habits can help you make consistent progress and retain information more effectively.
Practice Active Recall
Instead of passively reading or watching tutorials, actively engage with the material. Try to explain concepts in your own words, or implement what you’ve learned without referring to notes.
Use Spaced Repetition
Review concepts and skills at increasing intervals. This technique helps reinforce your learning and move information into long-term memory. Many learning platforms, including AlgoCademy, incorporate spaced repetition into their curriculum design.
Teach Others
Teaching a concept to someone else is one of the best ways to solidify your own understanding. Consider starting a blog, creating tutorial videos, or mentoring less experienced programmers.
10. Prepare for Technical Interviews
As you progress in your coding journey, you may find yourself preparing for technical interviews, especially with major tech companies. AlgoCademy’s focus on algorithmic thinking and problem-solving is particularly valuable in this context.
Practice Data Structures and Algorithms
Many technical interviews focus heavily on data structures and algorithms. Regularly practice implementing and working with common data structures like arrays, linked lists, trees, and graphs. Familiarize yourself with key algorithms for sorting, searching, and graph traversal.
Solve Coding Challenges
Platforms like AlgoCademy offer a wide range of coding challenges that mimic those you might encounter in technical interviews. Make solving these challenges a regular part of your routine.
Mock Interviews
Participate in mock interviews to practice explaining your thought process, coding under pressure, and communicating effectively. Many online platforms offer peer-to-peer mock interview services, or you can practice with friends or mentors.
Conclusion
Transforming coding frustration into project success is a journey that requires patience, persistence, and the right mindset. By embracing challenges, developing structured problem-solving approaches, leveraging resources, and cultivating good habits, you can turn obstacles into opportunities for growth and learning.
Remember, every successful programmer has faced moments of frustration. What sets them apart is their ability to persevere, learn from their mistakes, and continuously improve their skills. With the strategies outlined in this guide and the resources provided by platforms like AlgoCademy, you have all the tools you need to overcome coding challenges and achieve your programming goals.
So the next time you find yourself stuck on a difficult problem or feeling overwhelmed by a complex project, take a deep breath, remember these strategies, and approach the challenge with renewed determination. Your next coding success story is just around the corner!