How to Transition from Tutorial Learning to Building Projects
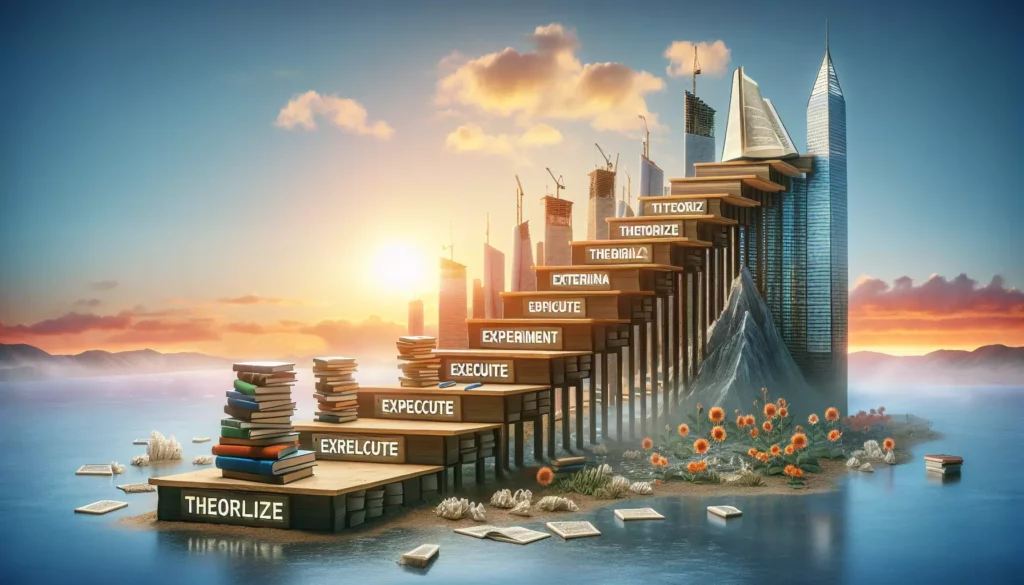
Learning to code can be an exciting journey, filled with new discoveries and challenges. Many aspiring developers start their coding adventure by following tutorials, watching video courses, or reading programming books. While these resources are invaluable for building a foundation, there comes a point when you need to transition from passive learning to active project building. This shift is crucial for cementing your skills, gaining practical experience, and preparing yourself for real-world development scenarios.
In this comprehensive guide, we’ll explore the importance of moving beyond tutorials and dive into strategies that will help you make the leap from tutorial-based learning to creating your own projects. We’ll cover everything from overcoming common challenges to selecting the right projects and building a portfolio that showcases your skills to potential employers.
Why Transitioning to Project-Based Learning is Important
Before we delve into the how-to’s, let’s understand why this transition is so crucial for your development as a programmer:
- Practical Application: Building projects allows you to apply theoretical knowledge in real-world scenarios.
- Problem-Solving Skills: You’ll encounter and overcome challenges that tutorials can’t prepare you for.
- Portfolio Building: Projects provide tangible evidence of your skills to potential employers.
- Deeper Understanding: Creating projects from scratch reinforces your understanding of programming concepts.
- Creativity and Innovation: You’ll learn to think creatively and come up with unique solutions.
- Confidence Boost: Successfully completing projects builds confidence in your abilities.
Signs You’re Ready to Move Beyond Tutorials
How do you know when it’s time to take the plunge into project-based learning? Here are some indicators:
- You can understand and write basic code without constant reference to tutorials.
- You’re familiar with fundamental programming concepts in your chosen language.
- You find yourself thinking, “I could build that” when using apps or websites.
- Tutorial exercises feel repetitive or too easy.
- You’re curious about how to apply your skills to solve real-world problems.
If you resonate with these points, it’s likely time to start your transition to project-based learning.
Overcoming the Tutorial Comfort Zone
Moving away from tutorials can be daunting. Here are some strategies to help you overcome the initial hurdles:
1. Start Small
Don’t jump straight into building a full-scale application. Begin with small, manageable projects that slightly exceed your current skill level. This approach, known as the “Zone of Proximal Development,” helps you grow without feeling overwhelmed.
2. Set Realistic Goals
Break down your project into smaller, achievable milestones. This makes the overall task less intimidating and provides a sense of progress as you complete each step.
3. Embrace the Learning Process
Accept that you’ll make mistakes and encounter problems. Each challenge is an opportunity to learn and improve your skills. Remember, even experienced developers face issues and need to search for solutions regularly.
4. Join Coding Communities
Engage with other developers through forums, social media, or local meetups. These communities can provide support, inspiration, and help when you’re stuck.
5. Use Tutorials as Reference, Not a Crutch
Instead of following tutorials step-by-step, use them as reference materials when you encounter specific problems in your projects.
Choosing Your First Projects
Selecting the right projects is crucial for a smooth transition. Here are some ideas to get you started:
1. Personal Website
Create a simple personal website or portfolio. This project allows you to practice HTML, CSS, and potentially JavaScript while creating something useful for your career.
2. To-Do List Application
Build a basic to-do list app. This project covers fundamental concepts like data storage, user input, and dynamic content updating.
3. Weather App
Develop a weather application that fetches data from an API. This project introduces you to working with external data sources and asynchronous programming.
4. Simple Game
Create a basic game like Tic-Tac-Toe or a quiz game. This will help you practice logic implementation and user interaction.
5. Calculator
Build a calculator application. This project involves handling user input, performing calculations, and displaying results dynamically.
Remember, the goal is to choose projects that interest you and align with your learning objectives. As you progress, gradually increase the complexity of your projects.
Essential Skills for Project-Based Learning
To successfully transition to building projects, you’ll need to develop or strengthen several key skills:
1. Problem Decomposition
Learn to break down large problems into smaller, manageable tasks. This skill is crucial for tackling complex projects without feeling overwhelmed.
2. Research and Self-Learning
Develop the ability to find solutions to problems on your own. This includes effectively using search engines, reading documentation, and understanding code examples.
3. Debugging
Improve your debugging skills to efficiently identify and fix issues in your code. Familiarize yourself with debugging tools available in your development environment.
4. Version Control
Learn to use version control systems like Git. This skill is essential for managing your project’s codebase and collaborating with others.
5. Code Organization and Best Practices
Study and implement coding best practices and design patterns. This will help you write cleaner, more maintainable code.
Building Your First Project: A Step-by-Step Guide
Let’s walk through the process of building a simple project to illustrate the transition from tutorials to project-based learning. We’ll create a basic weather application using HTML, CSS, and JavaScript.
Step 1: Plan Your Project
Before writing any code, outline your project’s features and structure:
- User can enter a city name
- Application fetches weather data from an API
- Display current temperature, weather condition, and icon
- Show additional information like humidity and wind speed
Step 2: Set Up Your Development Environment
Create a new directory for your project and set up the basic file structure:
<!-- index.html -->
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Weather App</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<div id="app">
<h1>Weather App</h1>
<input type="text" id="cityInput" placeholder="Enter city name">
<button id="searchBtn">Search</button>
<div id="weatherInfo"></div>
</div>
<script src="app.js"></script>
</body>
</html>
/* styles.css */
body {
font-family: Arial, sans-serif;
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
margin: 0;
background-color: #f0f0f0;
}
#app {
background-color: white;
padding: 20px;
border-radius: 8px;
box-shadow: 0 0 10px rgba(0, 0, 0, 0.1);
}
input, button {
margin: 10px 0;
padding: 5px;
}
#weatherInfo {
margin-top: 20px;
}
// app.js
const apiKey = 'YOUR_API_KEY'; // Replace with your actual API key
const cityInput = document.getElementById('cityInput');
const searchBtn = document.getElementById('searchBtn');
const weatherInfo = document.getElementById('weatherInfo');
searchBtn.addEventListener('click', () => {
const city = cityInput.value;
if (city) {
fetchWeather(city);
}
});
async function fetchWeather(city) {
try {
const response = await fetch(`https://api.openweathermap.org/data/2.5/weather?q=${city}&appid=${apiKey}&units=metric`);
const data = await response.json();
displayWeather(data);
} catch (error) {
console.error('Error fetching weather data:', error);
weatherInfo.innerHTML = '<p>Failed to fetch weather data. Please try again.</p>';
}
}
function displayWeather(data) {
const { name, main, weather, wind } = data;
weatherInfo.innerHTML = `
<h2>${name}</h2>
<p>Temperature: ${main.temp}°C</p>
<p>Weather: ${weather[0].description}</p>
<p>Humidity: ${main.humidity}%</p>
<p>Wind Speed: ${wind.speed} m/s</p>
`;
}
Step 3: Implement Core Functionality
In this step, you’ll write the JavaScript code to fetch weather data from an API and display it to the user. You’ll need to sign up for a free API key from a weather service like OpenWeatherMap.
Step 4: Test and Debug
Run your application and test it with different city names. Use browser developer tools to debug any issues you encounter.
Step 5: Refine and Enhance
Once the basic functionality is working, consider adding enhancements such as:
- Error handling for invalid city names
- Adding weather icons
- Implementing a loading indicator
- Saving the last searched city in local storage
Step 6: Reflect on the Process
After completing the project, take some time to reflect on what you’ve learned. What challenges did you face? How did you overcome them? What would you do differently next time?
Common Challenges and How to Overcome Them
As you transition to project-based learning, you’re likely to encounter several challenges. Here’s how to address them:
1. Imposter Syndrome
Challenge: Feeling like you’re not skilled enough to build projects.
Solution: Remember that everyone starts somewhere. Focus on your progress rather than comparing yourself to others. Celebrate small victories and learn from mistakes.
2. Analysis Paralysis
Challenge: Getting stuck in the planning phase, unable to start coding.
Solution: Set a time limit for planning. Start with a minimal viable product (MVP) and iterate from there. Remember, it’s okay to adjust your plan as you go.
3. Scope Creep
Challenge: Project growing beyond your initial plans, becoming overwhelming.
Solution: Clearly define your project’s core features before starting. Use a project management tool to track tasks and prioritize features.
4. Technical Roadblocks
Challenge: Encountering problems you don’t know how to solve.
Solution: Break the problem down into smaller parts. Research each part individually. Don’t hesitate to ask for help in coding communities or forums.
5. Motivation Fluctuations
Challenge: Losing motivation when progress feels slow.
Solution: Set small, achievable goals. Regularly review your progress. Connect with other learners for mutual support and accountability.
Building a Project Portfolio
As you complete projects, it’s important to showcase your work. A well-curated portfolio can be a powerful tool for job hunting or freelance opportunities. Here’s how to build an effective project portfolio:
1. Choose Quality Over Quantity
Focus on including your best work rather than every project you’ve ever completed. Select projects that demonstrate a range of skills and problem-solving abilities.
2. Provide Context
For each project, include:
- A brief description of the project’s purpose
- The technologies and tools used
- Your role in the project
- Challenges faced and how you overcame them
- Links to live demos or GitHub repositories
3. Showcase Your Process
Include information about your development process, such as wireframes, architecture diagrams, or blog posts about your project journey. This gives insight into your thought process and problem-solving skills.
4. Keep It Updated
Regularly update your portfolio with new projects and improvements to existing ones. This shows continuous learning and growth.
5. Make It Accessible
Ensure your portfolio is easy to navigate and accessible across different devices. Consider hosting it on platforms like GitHub Pages or Netlify for easy sharing.
Leveraging Your Projects for Career Growth
Your projects can be powerful tools for advancing your programming career. Here’s how to make the most of them:
1. Use Projects in Job Applications
Highlight relevant projects in your resume and cover letters. Be prepared to discuss your projects in detail during interviews.
2. Contribute to Open Source
Contribute to open-source projects related to your interests. This can help you gain experience working on larger codebases and collaborating with other developers.
3. Write About Your Projects
Start a blog or write articles about your project experiences. This can showcase your communication skills and deepen your understanding of the topics.
4. Network with Other Developers
Share your projects in developer communities and social media. Engage in discussions and be open to feedback and collaboration opportunities.
5. Consider Freelancing
Use your projects as a springboard into freelancing. Platforms like Upwork or Freelancer can be good starting points for finding clients.
Continuing Your Learning Journey
Transitioning to project-based learning is not the end of your educational journey, but rather the beginning of a new phase. Here are some ways to continue growing as a developer:
1. Take on Increasingly Complex Projects
Gradually increase the complexity and scope of your projects. This could involve working with new technologies, tackling more challenging problems, or building larger-scale applications.
2. Learn from Code Reviews
Seek code reviews from more experienced developers. Their feedback can be invaluable for improving your coding skills and learning best practices.
3. Explore New Technologies
Stay current with emerging technologies and frameworks. Experiment with them in side projects to broaden your skill set.
4. Attend Workshops and Conferences
Participate in coding workshops, hackathons, and tech conferences. These events can expose you to new ideas and help you network with other professionals.
5. Consider Advanced Education
Look into specialized courses, bootcamps, or even advanced degrees if you want to deepen your expertise in specific areas of computer science or software engineering.
Conclusion
Transitioning from tutorial-based learning to building your own projects is a significant step in your journey as a programmer. It can be challenging, but it’s also incredibly rewarding. By taking this step, you’re not just learning to code; you’re learning to create, problem-solve, and think like a developer.
Remember, every experienced developer was once in your shoes. They faced similar challenges and doubts. What sets successful developers apart is their willingness to push through difficulties, learn from mistakes, and continuously improve their skills.
As you embark on this new phase of your learning journey, stay curious, be persistent, and don’t be afraid to take risks. Embrace the process of building, breaking, and fixing. Each project you complete, regardless of its size or complexity, is a step forward in your development as a programmer.
The transition from tutorials to projects is not just about coding; it’s about developing a mindset of continuous learning and problem-solving. It’s about building the confidence to tackle real-world challenges and the resilience to overcome obstacles. These skills will serve you well throughout your career in technology.
So, take that first step. Choose a project, start coding, and begin your journey from a tutorial follower to a project creator. The world of possibilities that opens up when you start building your own projects is vast and exciting. Embrace it, enjoy it, and happy coding!