How to Train Your Brain for Algorithmic Thinking: A Comprehensive Guide
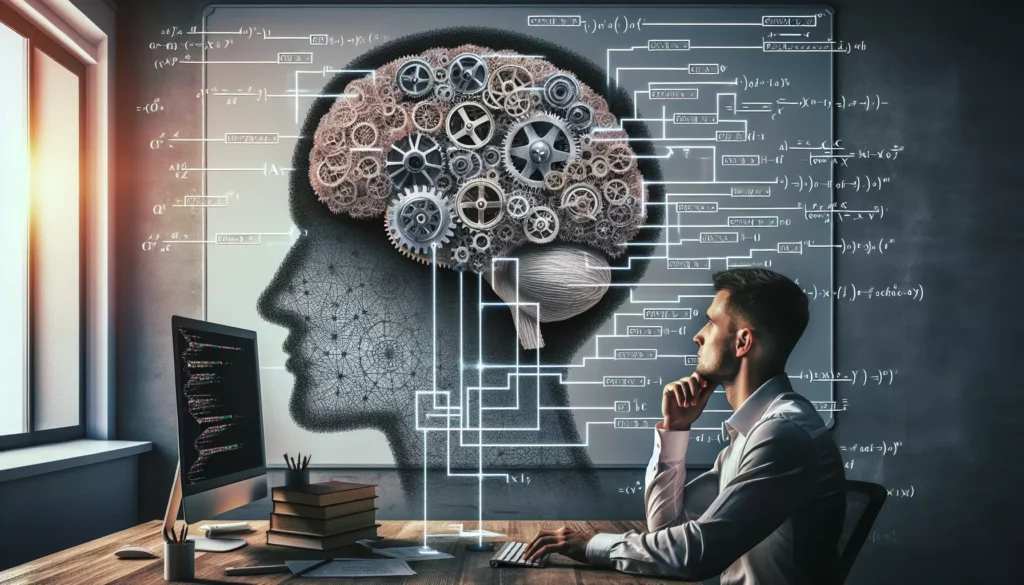
In today’s digital age, the ability to think algorithmically is becoming increasingly valuable across various fields, especially in computer science and software engineering. Algorithmic thinking is not just a skill for programmers; it’s a powerful cognitive tool that can enhance problem-solving abilities in many aspects of life. This comprehensive guide will explore what algorithmic thinking is, why it’s important, and most crucially, how you can train your brain to master this essential skill.
What is Algorithmic Thinking?
Algorithmic thinking is a problem-solving approach that involves breaking down complex problems into smaller, more manageable steps. It’s the foundation of computer programming and a crucial skill for anyone looking to excel in the tech industry. At its core, algorithmic thinking is about:
- Identifying and defining problems clearly
- Breaking down problems into smaller, solvable components
- Developing step-by-step solutions
- Analyzing the efficiency and effectiveness of solutions
- Iterating and improving upon initial solutions
This way of thinking is not limited to coding; it’s a versatile skill that can be applied to various real-world scenarios, from organizing a project to planning a trip.
Why is Algorithmic Thinking Important?
The importance of algorithmic thinking extends far beyond the realm of computer science. Here are some key reasons why developing this skill is crucial:
- Enhanced Problem-Solving Skills: Algorithmic thinking sharpens your ability to approach complex problems systematically.
- Improved Logical Reasoning: It strengthens your capacity to think logically and make sound decisions based on available information.
- Increased Efficiency: By breaking down problems and creating step-by-step solutions, you can tackle tasks more efficiently.
- Better Career Prospects: In the tech industry, algorithmic thinking is a highly sought-after skill, especially for roles involving software development and data analysis.
- Adaptability: The principles of algorithmic thinking can be applied to various fields, making you more adaptable in different professional contexts.
How to Train Your Brain for Algorithmic Thinking
Now that we understand the importance of algorithmic thinking, let’s dive into practical strategies to develop and strengthen this skill.
1. Start with the Basics of Programming
If you’re new to programming, start by learning the fundamentals of a programming language. Python is often recommended for beginners due to its readable syntax and versatility. Here’s a simple example of how you might start thinking algorithmically with Python:
def find_max(numbers):
if not numbers:
return None
max_num = numbers[0]
for num in numbers:
if num > max_num:
max_num = num
return max_num
# Example usage
numbers = [3, 7, 2, 9, 1, 5]
print(find_max(numbers)) # Output: 9
This simple function demonstrates algorithmic thinking by breaking down the problem of finding the maximum number into steps: initializing a variable, iterating through the list, and comparing each number.
2. Practice Problem Decomposition
One of the key aspects of algorithmic thinking is the ability to break down complex problems into smaller, more manageable parts. Start with simple problems and practice decomposing them into steps. For example, if you’re tasked with creating a program to calculate the average of a list of numbers, you might break it down like this:
- Sum all the numbers in the list
- Count how many numbers are in the list
- Divide the sum by the count
Implementing this in Python might look like:
def calculate_average(numbers):
if not numbers:
return 0
total = sum(numbers)
count = len(numbers)
return total / count
# Example usage
numbers = [1, 2, 3, 4, 5]
print(calculate_average(numbers)) # Output: 3.0
3. Learn and Apply Common Algorithms
Familiarize yourself with common algorithms and data structures. Start with basic sorting algorithms like bubble sort, selection sort, and insertion sort. Then move on to more advanced algorithms like merge sort and quicksort. Understanding these algorithms will help you recognize patterns and apply them to new problems.
Here’s an example of a simple bubble sort implementation in Python:
def bubble_sort(arr):
n = len(arr)
for i in range(n):
for j in range(0, n-i-1):
if arr[j] > arr[j+1]:
arr[j], arr[j+1] = arr[j+1], arr[j]
return arr
# Example usage
numbers = [64, 34, 25, 12, 22, 11, 90]
sorted_numbers = bubble_sort(numbers)
print(sorted_numbers) # Output: [11, 12, 22, 25, 34, 64, 90]
4. Solve Coding Challenges Regularly
Platforms like LeetCode, HackerRank, and CodeSignal offer a wide range of coding challenges that can help you improve your algorithmic thinking skills. Start with easy problems and gradually move to more complex ones. Remember, the goal is not just to solve the problem but to understand the underlying concepts and patterns.
5. Analyze and Optimize Your Solutions
Once you’ve solved a problem, don’t stop there. Analyze your solution for efficiency and consider alternative approaches. This practice will help you develop a more nuanced understanding of algorithmic thinking. Ask yourself:
- Is there a more efficient way to solve this problem?
- Can I reduce the time or space complexity of my solution?
- Are there edge cases I haven’t considered?
6. Study Time and Space Complexity
Understanding the concepts of time and space complexity is crucial for developing efficient algorithms. Learn about Big O notation and how to analyze the efficiency of your code. This knowledge will help you make informed decisions when designing algorithms.
For example, consider the time complexity of the bubble sort algorithm we implemented earlier:
# Bubble Sort Time Complexity Analysis
def bubble_sort(arr):
n = len(arr)
for i in range(n): # O(n)
for j in range(0, n-i-1): # O(n)
if arr[j] > arr[j+1]:
arr[j], arr[j+1] = arr[j+1], arr[j]
return arr
# The time complexity of bubble sort is O(n^2) due to the nested loops
7. Learn to Think Recursively
Recursive thinking is a powerful tool in algorithmic problem-solving. Many complex problems can be solved elegantly using recursion. Practice writing recursive functions and understanding how they work. Here’s a simple example of a recursive function to calculate factorial:
def factorial(n):
if n == 0 or n == 1:
return 1
else:
return n * factorial(n-1)
# Example usage
print(factorial(5)) # Output: 120
8. Visualize Algorithms
Visual representations can greatly enhance your understanding of how algorithms work. Use flowcharts, diagrams, or even animation tools to visualize the step-by-step process of an algorithm. This practice can help you better grasp complex concepts and identify potential improvements in your algorithms.
9. Collaborate and Discuss with Others
Join coding communities, participate in hackathons, or find a study group. Discussing problems and solutions with others can expose you to different perspectives and approaches. Platforms like GitHub, Stack Overflow, and Reddit have active communities where you can engage in discussions about algorithms and programming.
10. Apply Algorithmic Thinking to Real-World Problems
Don’t limit your algorithmic thinking to coding problems. Try to apply this approach to everyday situations. For example, you could use algorithmic thinking to optimize your daily routine or plan a complex project at work. This practice will help reinforce the skill and demonstrate its versatility.
Tools and Resources for Developing Algorithmic Thinking
To support your journey in developing algorithmic thinking skills, consider using the following tools and resources:
- Online Coding Platforms: LeetCode, HackerRank, CodeSignal, and Project Euler offer a wide range of coding challenges to practice your skills.
- Books: “Introduction to Algorithms” by Cormen, Leiserson, Rivest, and Stein is a comprehensive resource for learning about algorithms.
- Online Courses: Platforms like Coursera, edX, and Udacity offer courses on algorithms and data structures from top universities.
- Visualization Tools: Websites like VisuAlgo and Algorithm Visualizer can help you understand how different algorithms work through interactive visualizations.
- IDE and Code Editors: Use tools like PyCharm, Visual Studio Code, or Jupyter Notebooks to write and test your code efficiently.
Common Pitfalls to Avoid
As you work on developing your algorithmic thinking skills, be aware of these common pitfalls:
- Overlooking Edge Cases: Always consider extreme or unusual inputs when designing your algorithms.
- Premature Optimization: Focus on creating a working solution first, then optimize if necessary.
- Neglecting Code Readability: Write clean, well-documented code that others (and your future self) can understand.
- Ignoring Time and Space Complexity: Always consider the efficiency of your solutions, especially for larger inputs.
- Not Learning from Mistakes: Analyze your errors and use them as learning opportunities to improve your skills.
Conclusion
Training your brain for algorithmic thinking is a journey that requires patience, practice, and persistence. By following the strategies outlined in this guide and consistently challenging yourself with new problems, you can develop this valuable skill over time. Remember that algorithmic thinking is not just about coding; it’s a powerful approach to problem-solving that can benefit you in many areas of life.
As you continue to develop your algorithmic thinking skills, you’ll find that complex problems become more manageable, and you’ll be better equipped to tackle challenges in both your professional and personal life. Embrace the process, stay curious, and keep pushing yourself to learn and grow. With dedication and the right approach, you can master the art of algorithmic thinking and open up a world of opportunities in the ever-evolving landscape of technology and beyond.