How to Think Algorithmically During a Coding Interview: From Brute Force to Optimization
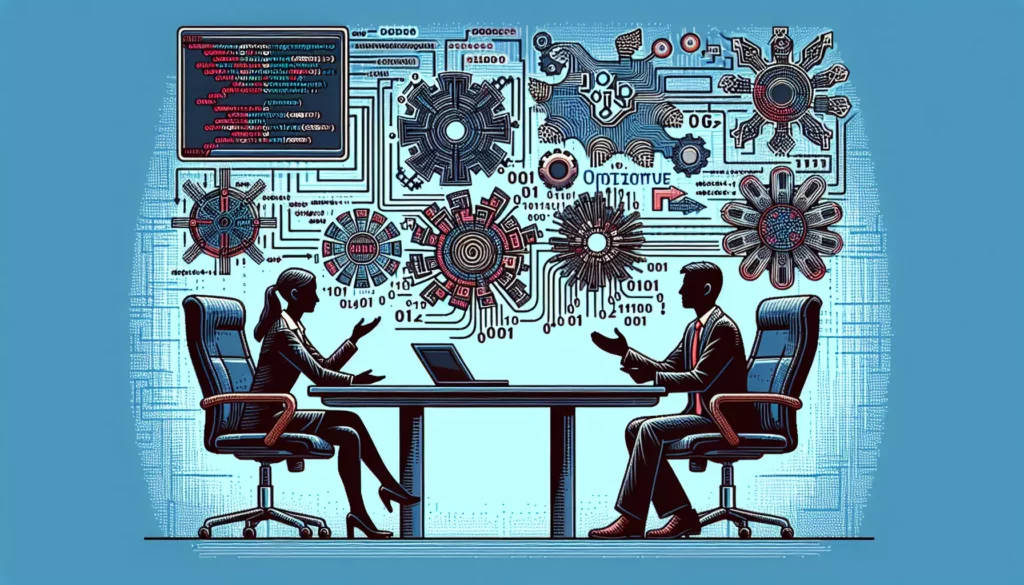
Coding interviews can be nerve-wracking experiences, especially when you’re faced with complex algorithmic problems under time pressure. The ability to think algorithmically and efficiently optimize your solutions is a crucial skill that can set you apart from other candidates. In this comprehensive guide, we’ll explore the process of approaching coding interview problems, starting from brute force solutions and progressing towards more optimized algorithms.
Understanding the Importance of Algorithmic Thinking
Before we dive into the specifics of problem-solving during coding interviews, it’s essential to understand why algorithmic thinking is so valuable. Algorithmic thinking involves breaking down complex problems into smaller, manageable steps and developing efficient solutions. This skill is not only crucial for coding interviews but also for real-world software development.
Here are some reasons why algorithmic thinking is important:
- Efficiency: Optimized algorithms can significantly improve the performance of software applications.
- Scalability: Well-designed algorithms can handle large datasets and growing user bases.
- Problem-solving: Algorithmic thinking enhances your ability to tackle complex issues in various domains.
- Code quality: Efficient algorithms often lead to cleaner, more maintainable code.
The Step-by-Step Guide to Thinking Algorithmically Under Interview Pressure
Now, let’s break down the process of approaching a coding interview problem algorithmically. This step-by-step guide will help you navigate from the initial problem statement to an optimized solution.
1. Understand the Problem
The first and most crucial step is to fully understand the problem at hand. Many candidates rush into coding without grasping the nuances of the question, leading to incorrect or suboptimal solutions.
- Read the problem statement carefully, multiple times if necessary.
- Identify the input and expected output.
- Ask clarifying questions to the interviewer about any ambiguities or edge cases.
- Confirm your understanding by restating the problem in your own words.
2. Analyze the Constraints
Before jumping into a solution, consider the constraints of the problem. This step is crucial in determining whether a brute force approach is acceptable or if a more optimized solution is necessary from the start.
- Look for any time or space complexity requirements mentioned in the problem statement.
- Consider the size of the input data. Large inputs often require more efficient solutions.
- Think about any specific constraints on memory usage or runtime.
3. Brainstorm and Discuss Potential Approaches
Before writing any code, take some time to think about different ways to solve the problem. This step is where you can showcase your problem-solving skills to the interviewer.
- Start with the simplest approach that comes to mind, even if it’s not optimal.
- Consider various data structures and algorithms that might be applicable.
- Discuss trade-offs between different approaches with the interviewer.
- Don’t be afraid to think out loud – interviewers often value your thought process as much as the final solution.
4. Start with a Brute Force Solution
In many cases, starting with a brute force solution is an acceptable and even recommended approach. It demonstrates that you can quickly come up with a working solution and provides a baseline for optimization.
- Implement the simplest solution that solves the problem correctly.
- Focus on correctness rather than efficiency at this stage.
- Use this as an opportunity to showcase your coding skills and ability to translate ideas into code.
5. Analyze the Brute Force Solution
Once you have a working brute force solution, take a step back and analyze its performance. This analysis will guide your optimization efforts.
- Determine the time and space complexity of your brute force solution.
- Identify the bottlenecks or inefficiencies in your current approach.
- Consider whether the performance meets the problem’s constraints.
6. Optimize Incrementally
If the brute force solution doesn’t meet the required performance criteria, start optimizing your algorithm incrementally. This step-by-step optimization process allows you to showcase your problem-solving skills and algorithmic knowledge.
- Look for redundant computations or repeated work that can be eliminated.
- Consider using more efficient data structures to improve time or space complexity.
- Apply common optimization techniques such as memoization, dynamic programming, or divide-and-conquer approaches.
- Explain your optimization ideas to the interviewer as you go along.
7. Implement the Optimized Solution
Once you’ve identified potential optimizations, implement the improved solution. Be sure to maintain clear and readable code throughout this process.
- Refactor your existing code or write a new implementation based on your optimized approach.
- Keep your code modular and well-organized to facilitate further improvements if needed.
- Continue to explain your implementation choices to the interviewer.
8. Test and Verify
After implementing your optimized solution, it’s crucial to test and verify its correctness.
- Use the example test cases provided in the problem statement.
- Create additional test cases, including edge cases and larger inputs.
- Walk through your code with a small input to ensure it behaves as expected.
9. Analyze the Final Solution
Conclude by analyzing the time and space complexity of your optimized solution. This step demonstrates your ability to critically evaluate your own work.
- Explain the improved time and space complexity to the interviewer.
- Discuss any trade-offs made during the optimization process.
- Consider potential further optimizations or alternative approaches, even if you don’t implement them.
Recognizing When Brute Force is Acceptable
While optimization is often necessary, there are scenarios where a brute force solution might be acceptable as a starting point or even as a final solution. Here are some situations where you might consider sticking with a brute force approach:
1. Small Input Size
If the problem constraints specify a small input size, a brute force solution might be sufficient. For example, if you’re dealing with arrays of length 10 or less, even a quadratic time complexity algorithm might run fast enough.
2. Time Constraints
In a coding interview, you typically have limited time. If implementing an optimized solution would take too long, it might be better to present a working brute force solution and discuss potential optimizations.
3. Simplicity and Readability
Sometimes, a brute force solution is significantly simpler and more readable than an optimized version. In real-world scenarios, code maintainability is often prioritized over minor performance gains.
4. Baseline for Optimization
Starting with a brute force solution provides a clear baseline for optimization. It allows you to demonstrate your problem-solving process and ability to improve algorithms incrementally.
5. Problem Complexity
For some complex problems, a brute force solution might be the only feasible approach within the time constraints of an interview. In such cases, implementing the brute force solution and discussing potential optimizations can be a good strategy.
Transitioning from Brute Force to Optimal Algorithms
The process of moving from a brute force solution to a more optimal algorithm is a critical skill in algorithmic thinking. Here’s a detailed look at how to make this transition:
1. Identify Inefficiencies
The first step in optimization is to identify the inefficiencies in your brute force solution. Common inefficiencies include:
- Redundant calculations
- Unnecessary iterations
- Inefficient data structure usage
For example, consider a function that finds the nth Fibonacci number using recursion:
def fibonacci(n):
if n <= 1:
return n
return fibonacci(n-1) + fibonacci(n-2)
This brute force solution has an exponential time complexity due to redundant calculations.
2. Apply Optimization Techniques
Once you’ve identified the inefficiencies, apply appropriate optimization techniques. Some common techniques include:
- Memoization: Storing results of expensive function calls to avoid redundant calculations.
- Dynamic Programming: Breaking down a problem into simpler subproblems and storing their results.
- Greedy Algorithms: Making locally optimal choices at each step.
- Divide and Conquer: Breaking a problem into smaller subproblems, solving them independently, and combining the results.
Let’s optimize our Fibonacci function using memoization:
def fibonacci(n, memo={}):
if n in memo:
return memo[n]
if n <= 1:
return n
memo[n] = fibonacci(n-1, memo) + fibonacci(n-2, memo)
return memo[n]
This optimized version has a time complexity of O(n), a significant improvement over the exponential complexity of the brute force approach.
3. Utilize Efficient Data Structures
Choosing the right data structure can dramatically improve the efficiency of your algorithm. Consider the following data structures and their use cases:
- Hash Tables: For fast lookups, inserts, and deletes (average O(1) time complexity).
- Binary Search Trees: For maintaining a sorted set of data with O(log n) operations.
- Heaps: For efficiently finding the minimum or maximum element in a collection.
- Tries: For efficient prefix matching in strings.
For example, if you’re dealing with a problem that requires frequent lookups, consider using a hash table instead of repeatedly searching through an array.
4. Reduce Time Complexity
Analyze the time complexity of your algorithm and look for ways to reduce it. Common strategies include:
- Eliminating nested loops
- Using binary search instead of linear search
- Preprocessing data to enable faster queries
For instance, consider a problem where you need to find if a number exists in a sorted array. A brute force approach might involve linear search:
def linear_search(arr, target):
for num in arr:
if num == target:
return True
return False
This has a time complexity of O(n). By using binary search, we can reduce it to O(log n):
def binary_search(arr, target):
left, right = 0, len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return True
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return False
5. Space-Time Trade-offs
Sometimes, you can trade space for time or vice versa. Consider using extra space to store precomputed results if it significantly reduces time complexity. However, be mindful of the problem’s constraints and discuss trade-offs with your interviewer.
6. Amortized Analysis
In some cases, certain operations might seem expensive but are actually efficient when considered over a series of operations. Understanding amortized analysis can help you justify the efficiency of your algorithm.
For example, the operation of resizing a dynamic array might seem costly, but when amortized over many insert operations, it results in constant time complexity per insertion on average.
Common Optimization Patterns
As you practice algorithmic thinking, you’ll start to recognize common patterns that lead to optimizations. Here are some patterns to look out for:
1. Two Pointers
The two-pointer technique is often used to search pairs in a sorted array or linked list. It can reduce the time complexity from O(n^2) to O(n) in many scenarios.
Example: Finding a pair of numbers in a sorted array that sum to a target value.
def find_pair(arr, target):
left, right = 0, len(arr) - 1
while left < right:
current_sum = arr[left] + arr[right]
if current_sum == target:
return arr[left], arr[right]
elif current_sum < target:
left += 1
else:
right -= 1
return None
2. Sliding Window
The sliding window technique is useful for problems involving subarrays or substrings. It can often reduce the time complexity from O(n^2) to O(n).
Example: Finding the maximum sum subarray of a fixed size k.
def max_subarray_sum(arr, k):
if len(arr) < k:
return None
window_sum = sum(arr[:k])
max_sum = window_sum
for i in range(k, len(arr)):
window_sum = window_sum - arr[i-k] + arr[i]
max_sum = max(max_sum, window_sum)
return max_sum
3. Prefix Sum
Prefix sum is a technique where you precompute cumulative sums to answer range sum queries in O(1) time.
Example: Calculating the sum of elements in a given range of an array.
def build_prefix_sum(arr):
prefix_sum = [0] * (len(arr) + 1)
for i in range(1, len(prefix_sum)):
prefix_sum[i] = prefix_sum[i-1] + arr[i-1]
return prefix_sum
def range_sum(prefix_sum, left, right):
return prefix_sum[right+1] - prefix_sum[left]
4. Binary Search on Answer
This technique involves using binary search to find the optimal value in a range, even when the problem doesn’t explicitly involve searching.
Example: Finding the smallest number of pages to allocate to each student when dividing a book among students.
5. Graph Traversal Optimizations
For graph problems, optimizations often involve choosing the right traversal algorithm (BFS vs DFS) and using efficient data structures like priority queues for Dijkstra’s algorithm.
Practice and Continuous Improvement
Developing strong algorithmic thinking skills requires consistent practice and a commitment to continuous improvement. Here are some strategies to enhance your skills:
1. Solve Diverse Problems
Expose yourself to a wide range of problem types. Platforms like LeetCode, HackerRank, and AlgoCademy offer a vast array of coding challenges that can help you practice different algorithmic concepts.
2. Analyze Multiple Solutions
For each problem you solve, don’t stop at your first working solution. Explore alternative approaches, analyze their trade-offs, and understand why certain solutions are more efficient than others.
3. Time Your Problem-Solving Sessions
Practice solving problems under time constraints to simulate interview conditions. This will help you manage your time effectively during actual interviews.
4. Review and Reflect
After solving a problem, take time to reflect on your approach. What worked well? What could be improved? Are there any patterns or techniques you can apply to similar problems in the future?
5. Study Algorithm Fundamentals
Strengthen your understanding of fundamental algorithms and data structures. Resources like “Introduction to Algorithms” by Cormen et al. and online courses can provide a solid theoretical foundation.
6. Mock Interviews
Participate in mock interviews with peers or use platforms that offer simulated interview experiences. This will help you get comfortable with explaining your thought process while coding.
Conclusion
Thinking algorithmically during a coding interview is a skill that can be developed and refined with practice. By following the step-by-step guide outlined in this article, you can approach interview problems systematically, starting from a brute force solution and progressing towards more optimized algorithms.
Remember that the journey from brute force to optimization is not just about finding the most efficient solution—it’s about demonstrating your problem-solving process, your ability to analyze and improve algorithms, and your capacity to think critically under pressure.
As you continue to practice and refine your skills, you’ll find that algorithmic thinking becomes more intuitive. You’ll start recognizing patterns, applying optimization techniques more readily, and approaching problems with greater confidence.
Keep in mind that in real-world software development, as well as in many interview scenarios, a working solution is often more valuable than a perfect but incomplete one. Start with a brute force approach when appropriate, and then showcase your ability to optimize incrementally.
By mastering the art of algorithmic thinking, you’ll not only excel in coding interviews but also become a more effective and efficient software developer in your professional career. Happy coding, and best of luck in your future interviews!