How to Think About Project Architecture: Building a Strong Foundation for Long-Term Success
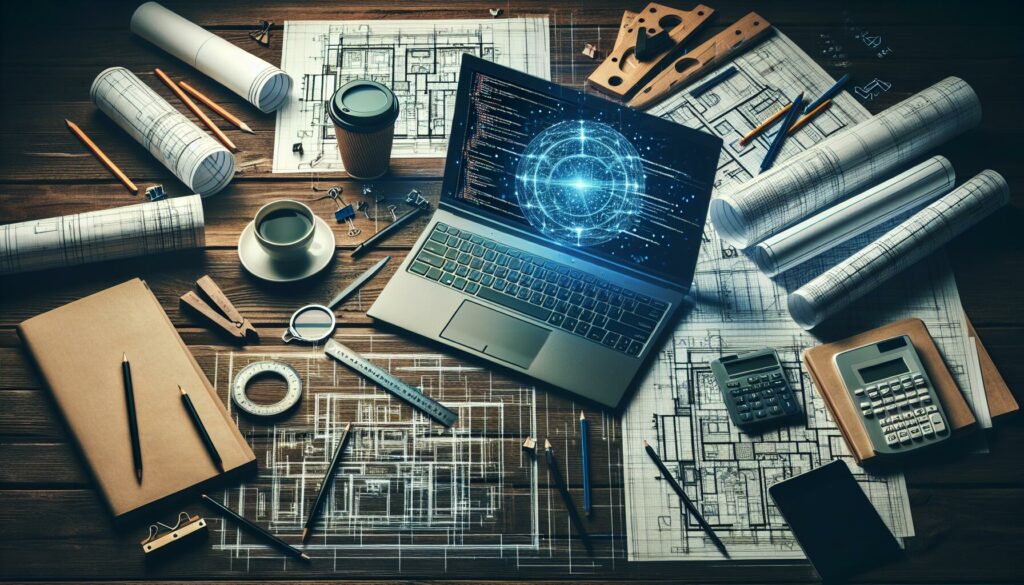
In the world of software development, project architecture is the backbone that supports the entire structure of your application. It’s the blueprint that guides developers through the intricate process of building robust, scalable, and maintainable software systems. Whether you’re a beginner just starting your coding journey or an experienced developer looking to refine your skills, understanding project architecture is crucial for long-term success.
In this comprehensive guide, we’ll dive deep into the world of software architecture, exploring everything from choosing the right frameworks and libraries to setting up proper data flow. We’ll also examine case studies of simple projects and their architectural decisions to give you practical insights into real-world applications.
Table of Contents
- Understanding Project Architecture
- Key Components of Project Architecture
- Choosing the Right Frameworks and Libraries
- Setting Up Proper Data Flow
- Case Studies: Simple Projects and Their Architectural Decisions
- Best Practices for Project Architecture
- Common Pitfalls to Avoid
- Future-Proofing Your Architecture
- Conclusion
1. Understanding Project Architecture
Project architecture is the high-level structure of a software system. It defines the major components of the system, their relationships, and how they interact with each other. A well-designed architecture provides a solid foundation for your project, making it easier to develop, maintain, and scale over time.
When thinking about project architecture, consider the following aspects:
- Modularity: Breaking down the system into smaller, manageable components.
- Scalability: Designing the system to handle growth in users, data, or functionality.
- Maintainability: Ensuring the code is easy to understand, modify, and debug.
- Performance: Optimizing the system for speed and efficiency.
- Security: Implementing measures to protect against vulnerabilities and threats.
- Testability: Designing components that can be easily tested in isolation and as part of the whole system.
2. Key Components of Project Architecture
Every project architecture consists of several key components that work together to create a functional system. Understanding these components is crucial for designing effective architectures:
2.1. Frontend
The frontend is the user-facing part of your application. It’s responsible for presenting data to users and handling their interactions. Key considerations for frontend architecture include:
- Choosing a framework (e.g., React, Vue, Angular)
- Implementing responsive design
- Managing state (e.g., Redux, Vuex)
- Handling routing
- Implementing user authentication and authorization
2.2. Backend
The backend is the server-side of your application, responsible for processing requests, managing data, and implementing business logic. Key considerations for backend architecture include:
- Choosing a programming language and framework (e.g., Node.js with Express, Python with Django, Ruby on Rails)
- Designing APIs (RESTful or GraphQL)
- Implementing authentication and authorization
- Handling data validation and sanitization
- Managing database connections and queries
2.3. Database
The database stores and manages the application’s data. Key considerations for database architecture include:
- Choosing the right database type (relational vs. non-relational)
- Designing the schema and relationships
- Implementing indexing for performance
- Planning for data backup and recovery
- Considering data migration strategies
2.4. APIs
APIs (Application Programming Interfaces) define how different components of your system communicate with each other. Key considerations for API architecture include:
- Choosing between REST and GraphQL
- Designing clear and consistent endpoints
- Implementing versioning
- Handling rate limiting and caching
- Documenting the API for developers
2.5. Infrastructure
Infrastructure refers to the underlying systems and services that support your application. Key considerations for infrastructure architecture include:
- Choosing between on-premises, cloud, or hybrid solutions
- Implementing containerization (e.g., Docker)
- Setting up continuous integration and deployment (CI/CD) pipelines
- Planning for scalability and load balancing
- Implementing monitoring and logging systems
3. Choosing the Right Frameworks and Libraries
Selecting the appropriate frameworks and libraries is a crucial decision in project architecture. The right choices can significantly impact your development speed, maintainability, and scalability. Here are some factors to consider when making these decisions:
3.1. Project Requirements
Start by clearly defining your project requirements. Consider factors such as:
- The type of application you’re building (e.g., web, mobile, desktop)
- Expected user base and scalability needs
- Performance requirements
- Integration with existing systems
- Time-to-market constraints
3.2. Community and Ecosystem
Look for frameworks and libraries with active communities and rich ecosystems. This ensures:
- Regular updates and bug fixes
- Availability of third-party plugins and extensions
- Abundant learning resources and documentation
- Easy recruitment of developers familiar with the technology
3.3. Learning Curve
Consider the learning curve associated with each framework or library. While it’s often beneficial to choose popular and powerful tools, ensure that your team has the capacity to learn and implement them effectively within your project timeline.
3.4. Performance and Scalability
Evaluate the performance characteristics of potential frameworks and libraries. Look for benchmarks and case studies that demonstrate their ability to handle your expected load and scale as your application grows.
3.5. Long-term Viability
Consider the long-term viability of the technologies you choose. Look for frameworks and libraries that have a track record of stability and are likely to be maintained and supported in the future.
3.6. Examples of Popular Frameworks and Libraries
Here are some examples of popular frameworks and libraries for different aspects of project architecture:
Frontend Frameworks:
- React
- Vue.js
- Angular
- Svelte
Backend Frameworks:
- Express.js (Node.js)
- Django (Python)
- Ruby on Rails (Ruby)
- Spring Boot (Java)
Database Libraries:
- Sequelize (ORM for Node.js)
- SQLAlchemy (ORM for Python)
- Mongoose (ODM for MongoDB and Node.js)
- Hibernate (ORM for Java)
Testing Frameworks:
- Jest (JavaScript)
- PyTest (Python)
- JUnit (Java)
- RSpec (Ruby)
4. Setting Up Proper Data Flow
Designing an efficient and scalable data flow is crucial for the performance and maintainability of your application. Here are some key considerations when setting up data flow in your project architecture:
4.1. Data Access Patterns
Analyze how data will be accessed and used within your application. Consider factors such as:
- Read/write ratios
- Data volume and growth rate
- Real-time requirements
- Data consistency needs
4.2. Data Storage
Choose appropriate data storage solutions based on your data access patterns. This may include:
- Relational databases for structured data with complex relationships
- NoSQL databases for unstructured or semi-structured data
- In-memory databases for high-performance caching
- File storage for large binary objects
4.3. Data Caching
Implement caching strategies to improve performance and reduce database load. Consider:
- In-memory caching (e.g., Redis, Memcached)
- Content Delivery Networks (CDNs) for static assets
- Application-level caching
- Database query result caching
4.4. Data Validation and Sanitization
Implement robust data validation and sanitization processes to ensure data integrity and security. This includes:
- Input validation on both client and server sides
- Data type checking and conversion
- Sanitization of user-generated content
- Handling of edge cases and error conditions
4.5. Asynchronous Processing
Use asynchronous processing for time-consuming tasks to improve responsiveness and scalability. Consider:
- Message queues (e.g., RabbitMQ, Apache Kafka)
- Background job processors (e.g., Sidekiq, Celery)
- Event-driven architectures
4.6. Data Consistency
Ensure data consistency across different components of your system. Consider:
- Transactional operations for critical data changes
- Eventual consistency for distributed systems
- Conflict resolution strategies for concurrent updates
4.7. Example: Setting Up Data Flow in a Web Application
Let’s consider a simple example of setting up data flow in a web application:
+-------------+ +-------------+ +-------------+
| | | | | |
| Client |<--->| API |<--->| Database |
| (React) | | (Express.js)| | (MongoDB) |
| | | | | |
+-------------+ +-------------+ +-------------+
^
|
+-------------+
| |
| Cache |
| (Redis) |
| |
+-------------+
In this architecture:
- The React client sends requests to the Express.js API.
- The API first checks the Redis cache for the requested data.
- If the data is not in the cache, the API queries the MongoDB database.
- The API then caches the retrieved data in Redis for future requests.
- The API sends the data back to the client.
This setup provides a balance between performance (through caching) and data consistency (by using a database as the source of truth).
5. Case Studies: Simple Projects and Their Architectural Decisions
To better understand how architectural decisions are made in real-world scenarios, let’s examine a few case studies of simple projects and the reasoning behind their architectural choices.
5.1. Case Study: Todo List Application
Project Requirements:
- Allow users to create, read, update, and delete tasks
- Provide user authentication
- Store tasks persistently
- Offer a responsive web interface
Architectural Decisions:
- Frontend: React
- Reasoning: React’s component-based architecture makes it easy to create reusable UI elements, which is beneficial for a task-oriented application like a todo list.
- Backend: Node.js with Express
- Reasoning: JavaScript on both frontend and backend allows for code reuse and a unified development experience.
- Database: MongoDB
- Reasoning: The flexible schema of MongoDB is well-suited for storing task data, which may have varying attributes.
- Authentication: JSON Web Tokens (JWT)
- Reasoning: JWTs provide a stateless authentication mechanism, reducing server load and improving scalability.
- API: RESTful API
- Reasoning: REST is simple to implement and well-understood, making it a good choice for a straightforward CRUD application.
Data Flow:
+-------------+ +-------------+ +-------------+
| | | | | |
| React |<--->| Express.js |<--->| MongoDB |
| Frontend | | API | | Database |
| | | | | |
+-------------+ +-------------+ +-------------+
^
|
+-------------+
| |
| JWT |
| Auth Service|
| |
+-------------+
5.2. Case Study: Simple E-commerce Platform
Project Requirements:
- Display product catalog
- Allow users to add items to cart
- Process orders
- Manage inventory
- Provide user authentication and profiles
Architectural Decisions:
- Frontend: Vue.js
- Reasoning: Vue.js offers a gentle learning curve and excellent performance, making it suitable for a small to medium-sized e-commerce platform.
- Backend: Python with Django
- Reasoning: Django’s “batteries-included” approach provides many built-in features useful for e-commerce, such as user authentication and admin interfaces.
- Database: PostgreSQL
- Reasoning: A relational database is well-suited for handling complex relationships between products, orders, and users in an e-commerce system.
- Caching: Redis
- Reasoning: Redis can cache frequently accessed data like product information and user sessions, improving response times.
- API: GraphQL
- Reasoning: GraphQL allows for flexible querying, which is beneficial when dealing with varied product data and complex relationships.
- Payment Processing: Stripe API
- Reasoning: Stripe offers a robust and secure payment processing solution with good documentation and ease of integration.
Data Flow:
+-------------+ +-------------+ +-------------+
| | | | | |
| Vue.js |<--->| Django |<--->| PostgreSQL |
| Frontend | | GraphQL API| | Database |
| | | | | |
+-------------+ +-------------+ +-------------+
^ ^
| |
+-------------+ +-------------+
| | | |
| Redis | | Stripe |
| Cache | | API |
| | | |
+-------------+ +-------------+
5.3. Case Study: Real-time Chat Application
Project Requirements:
- Support real-time messaging
- Allow creation of chat rooms
- Provide user authentication
- Store chat history
- Support file sharing
Architectural Decisions:
- Frontend: React with Redux
- Reasoning: React’s virtual DOM is efficient for updating UI in real-time applications, while Redux helps manage complex application state.
- Backend: Node.js with Socket.io
- Reasoning: Node.js’s event-driven architecture is well-suited for real-time applications, and Socket.io simplifies WebSocket implementation.
- Database: MongoDB
- Reasoning: MongoDB’s flexible schema is suitable for storing varied message types and chat room configurations.
- Message Queue: RabbitMQ
- Reasoning: RabbitMQ can handle high volumes of messages and ensure reliable delivery, which is crucial for a chat application.
- File Storage: Amazon S3
- Reasoning: S3 provides scalable and reliable object storage for shared files.
- Authentication: OAuth 2.0
- Reasoning: OAuth 2.0 allows for secure authentication and the option to integrate with third-party identity providers.
Data Flow:
+-------------+ +-------------+ +-------------+
| | | | | |
| React |<--->| Node.js |<--->| MongoDB |
| Frontend | | Socket.io | | Database |
| | | | | |
+-------------+ +-------------+ +-------------+
^ ^
| |
+-------------+ +-------------+
| | | |
| RabbitMQ | | Amazon S3 |
| Queue | | Storage |
| | | |
+-------------+ +-------------+
^
|
+-------------+
| |
| OAuth 2.0 |
| Auth |
| |
+-------------+
These case studies demonstrate how architectural decisions are influenced by project requirements, scalability needs, and the nature of the application. Each architecture is tailored to address the specific challenges of the project while leveraging appropriate technologies and patterns.
6. Best Practices for Project Architecture
When designing and implementing your project architecture, following best practices can help ensure a robust, maintainable, and scalable system. Here are some key best practices to consider:
6.1. Separation of Concerns
Divide your application into distinct modules or components, each responsible for a specific functionality. This makes the codebase easier to understand, maintain, and test.
6.2. Don’t Repeat Yourself (DRY)
Avoid duplicating code across your project. Instead, create reusable components, functions, or modules that can be shared across different parts of your application.
6.3. SOLID Principles
Follow the SOLID principles of object-oriented design:
- Single Responsibility Principle
- Open/Closed Principle
- Liskov Substitution Principle
- Interface Segregation Principle
- Dependency Inversion Principle
6.4. Use Design Patterns
Leverage established design patterns to solve common architectural challenges. Some useful patterns include:
- Factory Pattern
- Singleton Pattern
- Observer Pattern
- Strategy Pattern
- Decorator Pattern
6.5. Implement Proper Error Handling
Design a robust error handling strategy that includes logging, user-friendly error messages, and appropriate error responses for different types of errors.
6.6. Write Clean, Readable Code
Follow coding standards and best practices for the languages and frameworks you’re using. Use meaningful variable and function names, and include comments where necessary to explain complex logic.
6.7. Implement Continuous Integration and Continuous Deployment (CI/CD)
Set up automated build, test, and deployment pipelines to ensure code quality and streamline the development process.
6.8. Use Version Control
Utilize a version control system like Git to track changes, collaborate with team members, and manage different versions of your codebase.
6.9. Document Your Architecture
Create and maintain documentation for your project architecture, including diagrams, API specifications, and setup instructions. This helps onboard new team members and serves as a reference for future development.
6.10. Plan for Scalability
Design your architecture with scalability in mind, even if your current needs are modest. This might include using microservices, implementing load balancing, or choosing cloud-based solutions that can easily scale.
7. Common Pitfalls to Avoid
While designing and implementing your project architecture, be aware of these common pitfalls:
7.1. Overengineering
Avoid adding unnecessary complexity to your architecture. Start with a simple design and add complexity only when needed.
7.2. Neglecting Security
Don’t treat security as an afterthought. Incorporate security considerations into your architecture from the beginning, including data encryption, secure authentication, and protection against common vulnerabilities.
7.3. Ignoring Performance
Consider performance implications in your architectural decisions. Poor performance can lead to a poor user experience and scalability issues.
7.4. Tight Coupling
Avoid creating tightly coupled components that are difficult to modify or replace. Use dependency injection and interfaces to create loosely coupled systems.
7.5. Neglecting Testing
Don’t skip on testing. Implement unit tests, integration tests, and end-to-end tests to ensure the reliability and correctness of your system.
7.6. Ignoring Monitoring and Logging
Implement robust monitoring and logging from the start. This will help you identify and resolve issues quickly in production.
7.7. Not Planning for Data Growth
Consider how your data will grow over time and design your database schema and storage solutions accordingly.
7.8. Choosing Trendy Technologies Without Proper Evaluation
Don’t choose technologies just because they’re popular. Evaluate each technology based on its merits and how well it fits your project requirements.
8. Future-Proofing Your Architecture
To ensure your project architecture remains viable and efficient in the long term, consider these strategies for future-proofing:
8.1. Use Microservices Architecture
Consider breaking your application into microservices, which can be independently developed, deployed, and scaled. This approach provides flexibility for future changes and additions.
8.2. Implement API Versioning
Use API versioning to introduce changes without breaking existing client integrations. This allows for gradual updates and maintains backward compatibility.
8.3. Adopt Cloud-Native Technologies
Leverage cloud-native technologies and services that can easily scale and adapt to changing requirements. This might include containerization (e.g., Docker) and orchestration tools (e.g., Kubernetes).
8.4. Use Feature Flags
Implement feature flags to easily enable or disable features without requiring code changes. This allows for easier A/B testing and gradual rollouts of new functionality.
8.5. Plan for Data Migration
Design your data storage solutions with future migrations in mind. This might involve using database-agnostic ORMs or implementing a data access layer that can be easily swapped out.
8.6. Implement Automated Testing
Develop a comprehensive suite of automated tests, including unit tests, integration tests, and end-to-end tests. This will make it easier to refactor and update your codebase with confidence.
8.7. Use Dependency Injection
Implement dependency injection to reduce coupling between components. This makes it easier to swap out implementations or add new features in the future.
8.8. Stay Informed About Industry Trends
Keep up-to-date with emerging technologies and industry best practices. This will help you make informed decisions about when and how to update your architecture.
9. Conclusion
Thinking about project architecture is a crucial skill for any developer, from beginners to seasoned professionals. A well-designed architecture provides a solid foundation for your project, enabling easier development, maintenance, and scalability.
Remember these key takeaways:
- Understand the components of project architecture and how they interact.
- Choose frameworks and libraries that align with your project requirements and team skills.
- Design efficient data flow that considers performance, scalability, and data integrity.
- Learn from real-world case studies to inform your architectural decisions.
- Follow best practices and avoid common pitfalls in architectural design.
- Plan for the future by implementing strategies that allow for flexibility and growth.
By applying these principles and continuously refining your approach, you’ll be well-equipped to build robust, scalable, and maintainable software systems. Remember, good architecture is not about predicting the future, but about creating a flexible foundation that can adapt to changing requirements and technologies.
As you continue your journey in software development, keep exploring new architectural patterns, stay curious about emerging technologies, and always be willing to learn and adapt. With a solid understanding of project architecture, you’ll be well-prepared to tackle complex projects and contribute meaningfully to the world of software development.