How to Study Graph Algorithms for Interviews: A Comprehensive Guide
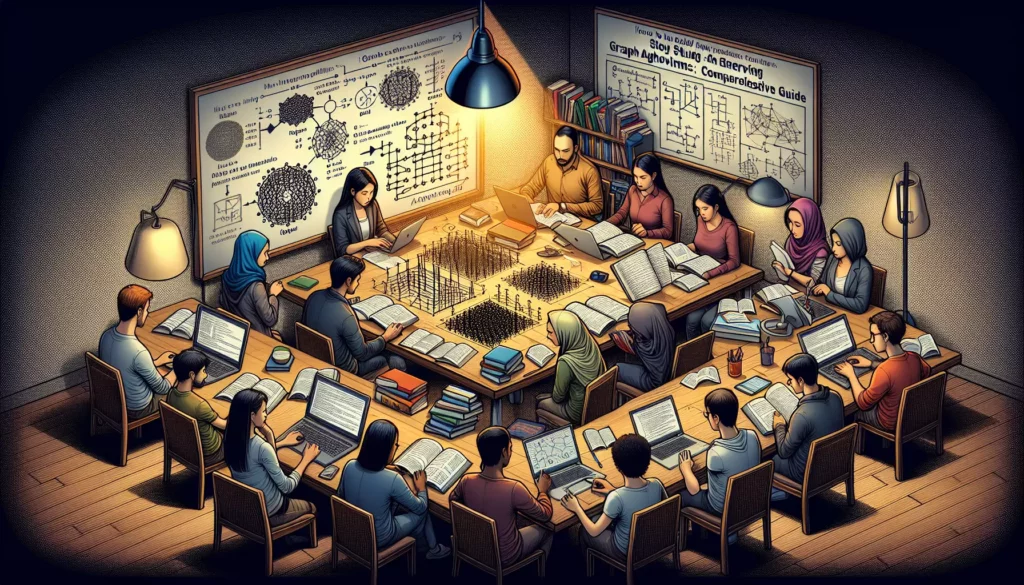
Graph algorithms are a crucial component of computer science and a common topic in technical interviews, especially for positions at major tech companies. Whether you’re preparing for a FAANG (Facebook, Amazon, Apple, Netflix, Google) interview or aiming to enhance your problem-solving skills, mastering graph algorithms is essential. In this comprehensive guide, we’ll explore effective strategies to study graph algorithms, common types of graph problems, and practical tips to ace your technical interviews.
Table of Contents
- Understanding Graphs: The Foundation
- Common Graph Algorithms You Need to Know
- Effective Study Strategies for Graph Algorithms
- Problem-Solving Techniques for Graph Questions
- Practice Resources and Platforms
- Interview Tips for Graph Algorithm Questions
- Advanced Graph Topics to Consider
- Real-World Applications of Graph Algorithms
- Common Mistakes to Avoid
- Conclusion: Mastering Graph Algorithms for Interview Success
1. Understanding Graphs: The Foundation
Before diving into complex algorithms, it’s crucial to have a solid understanding of what graphs are and how they’re represented in computer science.
What is a Graph?
A graph is a data structure consisting of a finite set of vertices (or nodes) and a set of edges connecting these vertices. Graphs are used to represent relationships between various entities and are fundamental in solving many real-world problems.
Types of Graphs
- Undirected Graphs: Edges have no direction.
- Directed Graphs (Digraphs): Edges have a direction.
- Weighted Graphs: Edges have associated weights or costs.
- Connected vs. Disconnected Graphs
- Cyclic vs. Acyclic Graphs
Graph Representations
Understanding how graphs are represented in code is crucial. The two most common representations are:
- Adjacency Matrix: A 2D array where matrix[i][j] represents an edge between vertices i and j.
- Adjacency List: An array of lists where each list contains the neighbors of a vertex.
Here’s a simple example of how you might represent a graph using an adjacency list in Python:
class Graph:
def __init__(self, num_vertices):
self.num_vertices = num_vertices
self.adj_list = [[] for _ in range(num_vertices)]
def add_edge(self, v1, v2):
self.adj_list[v1].append(v2)
self.adj_list[v2].append(v1) # For undirected graph
def print_graph(self):
for i in range(self.num_vertices):
print(f"Vertex {i}: {' -> '.join(map(str, self.adj_list[i]))}")
# Example usage
g = Graph(4)
g.add_edge(0, 1)
g.add_edge(0, 2)
g.add_edge(1, 2)
g.add_edge(2, 3)
g.print_graph()
Understanding these fundamentals will provide a strong foundation for learning more complex graph algorithms.
2. Common Graph Algorithms You Need to Know
To prepare effectively for interviews, focus on mastering these essential graph algorithms:
Breadth-First Search (BFS)
BFS explores a graph level by level, visiting all neighbors of a node before moving to the next level. It’s useful for finding the shortest path in an unweighted graph.
Depth-First Search (DFS)
DFS explores as far as possible along each branch before backtracking. It’s often used to detect cycles, topological sorting, and solving maze-like problems.
Dijkstra’s Algorithm
Used to find the shortest path between nodes in a weighted graph with non-negative edge weights.
Bellman-Ford Algorithm
Similar to Dijkstra’s but can handle negative edge weights and detect negative cycles.
Floyd-Warshall Algorithm
Finds shortest paths between all pairs of vertices in a weighted graph.
Prim’s Algorithm
Finds a minimum spanning tree for a weighted undirected graph.
Kruskal’s Algorithm
Another algorithm for finding a minimum spanning tree, often more efficient for sparse graphs.
Topological Sorting
Orders the vertices of a directed acyclic graph (DAG) such that for every directed edge (u, v), vertex u comes before v in the ordering.
Here’s a simple implementation of DFS in Python to give you an idea:
def dfs(graph, start, visited=None):
if visited is None:
visited = set()
visited.add(start)
print(start, end=' ')
for next in graph[start] - visited:
dfs(graph, next, visited)
return visited
# Example usage
graph = {'0': set(['1', '2']),
'1': set(['0', '3', '4']),
'2': set(['0']),
'3': set(['1']),
'4': set(['2', '3'])}
dfs(graph, '0')
Understanding these algorithms and their applications is crucial for technical interviews.
3. Effective Study Strategies for Graph Algorithms
Mastering graph algorithms requires a structured approach. Here are some effective strategies to enhance your learning:
Start with the Basics
Ensure you have a solid understanding of basic data structures like arrays, linked lists, stacks, and queues. These are often used in implementing graph algorithms.
Visualize the Algorithms
Use tools like draw.io or even pen and paper to visualize how algorithms work on different graph structures. This helps in understanding the logic behind each step.
Implement from Scratch
Don’t just memorize algorithms. Implement them from scratch in your preferred programming language. This deepens your understanding and prepares you for coding interviews.
Use Spaced Repetition
Review algorithms at increasing intervals. This technique, known as spaced repetition, helps in long-term retention of information.
Study the Time and Space Complexity
For each algorithm, understand its time and space complexity. This is crucial for optimizing solutions in interviews.
Learn Through Teaching
Explain the algorithms to others or write blog posts about them. Teaching reinforces your own understanding.
Use Interactive Learning Platforms
Platforms like AlgoCademy offer interactive tutorials and step-by-step guidance, which can be particularly helpful for visual learners.
Create Cheat Sheets
Summarize key points, time complexities, and use cases for each algorithm in a cheat sheet for quick revision.
4. Problem-Solving Techniques for Graph Questions
When faced with a graph problem in an interview, follow these steps:
1. Identify the Problem Type
Determine if it’s a shortest path problem, connectivity issue, cycle detection, etc. This helps in choosing the right algorithm.
2. Choose the Appropriate Data Structure
Decide whether to use an adjacency matrix or adjacency list based on the graph’s properties and the problem requirements.
3. Select the Right Algorithm
Based on the problem type, choose the most suitable algorithm (BFS, DFS, Dijkstra’s, etc.).
4. Consider Edge Cases
Think about special cases like disconnected graphs, negative weights, or cycles.
5. Optimize Your Solution
After implementing a basic solution, think about ways to optimize it for better time or space complexity.
6. Test Your Solution
Use various test cases to verify your solution, including edge cases.
Here’s an example of how you might approach a problem to find the shortest path in an unweighted graph:
from collections import deque
def shortest_path(graph, start, end):
queue = deque([[start]])
visited = set([start])
while queue:
path = queue.popleft()
node = path[-1]
if node == end:
return path
for neighbor in graph[node]:
if neighbor not in visited:
visited.add(neighbor)
new_path = list(path)
new_path.append(neighbor)
queue.append(new_path)
return None # No path found
# Example usage
graph = {
'A': ['B', 'C'],
'B': ['A', 'D', 'E'],
'C': ['A', 'F'],
'D': ['B'],
'E': ['B', 'F'],
'F': ['C', 'E']
}
print(shortest_path(graph, 'A', 'F')) # Output: ['A', 'C', 'F']
5. Practice Resources and Platforms
To master graph algorithms, consistent practice is key. Here are some excellent resources and platforms to hone your skills:
Online Coding Platforms
- LeetCode: Offers a wide range of graph problems with varying difficulty levels.
- HackerRank: Has a dedicated graph theory track with numerous problems.
- CodeForces: Great for more advanced graph problems and competitive programming.
Interactive Learning Platforms
- AlgoCademy: Provides interactive tutorials and AI-powered assistance for learning algorithms, including graph algorithms.
- Visualgo: Offers visualizations of various algorithms, including graph algorithms, which can be incredibly helpful for understanding their workings.
Books
- “Introduction to Algorithms” by Cormen, Leiserson, Rivest, and Stein
- “Algorithm Design” by Kleinberg and Tardos
- “Competitive Programming 3” by Steven and Felix Halim
Online Courses
- Coursera’s “Algorithms Specialization” by Stanford University
- edX’s “Algorithm Design and Analysis” by PennX
GitHub Repositories
Many developers maintain repositories with implementations of various algorithms. Studying these can provide insights into different coding styles and optimizations.
Practice Strategy
When using these resources, follow this strategy:
- Start with easier problems to build confidence.
- Gradually increase difficulty as you become more comfortable.
- Time yourself to simulate interview conditions.
- Review solutions after attempting problems, even if you solved them correctly.
- Revisit problems after a few weeks to reinforce your understanding.
6. Interview Tips for Graph Algorithm Questions
When facing graph algorithm questions in an interview, keep these tips in mind:
1. Clarify the Problem
Ask questions to ensure you understand the problem completely. Clarify any ambiguities about the graph structure, constraints, or expected output.
2. Think Aloud
Verbalize your thought process. This gives the interviewer insight into your problem-solving approach and allows them to provide hints if needed.
3. Start with a Brute Force Approach
If you’re stuck, start with a simple, inefficient solution. You can optimize it later, and it shows you can at least solve the problem.
4. Draw the Graph
Visualizing the graph can help you understand the problem better and might reveal patterns or solutions.
5. Discuss Trade-offs
If there are multiple ways to solve the problem, discuss the trade-offs between different approaches in terms of time and space complexity.
6. Test Your Solution
Before declaring you’re done, walk through your solution with a small test case to catch any logical errors.
7. Optimize
After implementing a working solution, think about ways to optimize it. Can you reduce the time complexity? Can you use less memory?
8. Handle Edge Cases
Consider and discuss how your solution handles edge cases like empty graphs, disconnected components, or cycles.
9. Be Familiar with Standard Library Functions
Know how to use standard library functions related to graphs and data structures in your preferred programming language.
10. Practice Coding Without an IDE
Many interviews require coding on a whiteboard or in a simple text editor. Practice writing code without the help of an IDE to simulate these conditions.
7. Advanced Graph Topics to Consider
While mastering the basics is crucial, being familiar with some advanced graph topics can set you apart in interviews:
1. Network Flow Algorithms
- Ford-Fulkerson Algorithm
- Edmonds-Karp Algorithm
- Dinic’s Algorithm
2. Strongly Connected Components
- Kosaraju’s Algorithm
- Tarjan’s Algorithm
3. Articulation Points and Bridges
Identifying critical nodes and edges in a graph whose removal increases the number of connected components.
4. Euler and Hamiltonian Paths
Understanding the conditions for these special paths and cycles in graphs.
5. Graph Coloring
Assigning colors to graph elements subject to certain constraints.
6. Maximum Bipartite Matching
Finding maximum matchings in bipartite graphs, often using the Hungarian algorithm.
7. Traveling Salesman Problem
While NP-hard, understanding approximation algorithms for this problem can be valuable.
8. A* Search Algorithm
An informed search algorithm, often used in pathfinding and graph traversal.
Here’s a simple implementation of finding articulation points in a graph:
from collections import defaultdict
class Graph:
def __init__(self, vertices):
self.V = vertices
self.graph = defaultdict(list)
self.Time = 0
def addEdge(self, u, v):
self.graph[u].append(v)
self.graph[v].append(u)
def APUtil(self, u, visited, ap, parent, low, disc):
children = 0
visited[u] = True
disc[u] = self.Time
low[u] = self.Time
self.Time += 1
for v in self.graph[u]:
if visited[v] == False:
parent[v] = u
children += 1
self.APUtil(v, visited, ap, parent, low, disc)
low[u] = min(low[u], low[v])
if parent[u] == -1 and children > 1:
ap[u] = True
if parent[u] != -1 and low[v] >= disc[u]:
ap[u] = True
elif v != parent[u]:
low[u] = min(low[u], disc[v])
def AP(self):
visited = [False] * self.V
disc = [float("Inf")] * self.V
low = [float("Inf")] * self.V
parent = [-1] * self.V
ap = [False] * self.V
for i in range(self.V):
if visited[i] == False:
self.APUtil(i, visited, ap, parent, low, disc)
return [i for i in range(self.V) if ap[i] == True]
# Example usage
g = Graph(5)
g.addEdge(1, 0)
g.addEdge(0, 2)
g.addEdge(2, 1)
g.addEdge(0, 3)
g.addEdge(3, 4)
print("Articulation points in the graph:", g.AP())
Understanding these advanced topics can give you an edge in complex interview questions and real-world problem-solving scenarios.
8. Real-World Applications of Graph Algorithms
Understanding the practical applications of graph algorithms can help you appreciate their importance and may come in handy during interviews. Here are some real-world applications:
1. Social Networks
- Friend recommendations (using clustering algorithms)
- Influence analysis (using centrality measures)
- Community detection (using graph partitioning algorithms)
2. Transportation and Logistics
- GPS and navigation systems (shortest path algorithms like Dijkstra’s)
- Traffic flow optimization (network flow algorithms)
- Supply chain management (minimum spanning tree algorithms)
3. Computer Networks
- Routing protocols (various shortest path algorithms)
- Network resilience (articulation points and bridges)
- Peer-to-peer networks (distributed hash tables using graph structures)
4. Bioinformatics
- Protein-protein interaction networks
- Gene regulatory networks
- Phylogenetic tree construction
5. Recommendation Systems
- Product recommendations in e-commerce
- Movie or music recommendations
6. Web Crawling and Indexing
- Search engine algorithms (PageRank is essentially a graph algorithm)
- Web crawling (using BFS or DFS)
7. Computer Vision
- Image segmentation (graph cuts algorithm)
- Feature matching in object recognition
8. Resource Allocation
- Task scheduling (topological sorting)
- Resource allocation in operating systems
Being able to discuss these applications can demonstrate your understanding of the broader impact of graph algorithms beyond just solving coding problems.
9. Common Mistakes to Avoid
When studying and implementing graph algorithms, be aware of these common pitfalls:
1. Neglecting Graph Representation
Choosing the wrong graph representation (adjacency matrix vs. adjacency list) can lead to suboptimal solutions.
2. Forgetting About Disconnected Graphs
Always consider that a graph might not be fully connected and adjust your algorithms accordingly.
3. Ignoring Direction in Directed Graphs
When dealing with directed graphs, ensure your algorithm respects edge directions.
4. Mishandling Cycles
In traversal algorithms, forgetting to handle cycles can lead to infinite loops.
5. Overlooking Edge Weights
In weighted graphs, using algorithms designed for unweighted graphs can lead to incorrect results.
6. Inefficient Data Structures
Using inefficient data structures (like using a list instead of a priority queue in Dijkstra’s algorithm) can significantly impact performance.
7. Not Considering Space Complexity
While optimizing for time, don’t forget about space complexity, especially for large graphs.
8. Overcomplicating Solutions
Sometimes, a simple BFS or DFS can solve a problem that might seem to require a more complex algorithm at first glance.
9. Neglecting Edge Cases
Forgetting to handle edge cases like empty graphs, single-node graphs, or fully connected graphs.
10. Misunderstanding Problem Constraints
Not clarifying problem constraints (like whether a graph is directed or undirected) can lead to incorrect implementations.
10. Conclusion: Mastering Graph Algorithms for Interview Success
Mastering graph algorithms is a journey that requires dedication, practice, and a structured approach. By understanding the fundamentals, practicing common algorithms, and exploring advanced topics, you’ll be well-prepared for technical interviews, especially at top tech companies.
Remember these key points:
- Start with a solid understanding of basic graph concepts and representations.
- Focus on mastering essential algorithms like BFS, DFS, Dijkstra’s, and minimum spanning tree algorithms.
- Practice implementing these algorithms from scratch to deepen your understanding.
- Utilize various resources including online platforms, books, and courses to diversify your learning.
- Apply problem-solving techniques and optimize your solutions for both time and space complexity.
- Be familiar with real-world applications to demonstrate the practical relevance of your knowledge.
- Stay updated with advanced topics to set yourself apart in challenging interviews.
With consistent effort and the right approach, you’ll not only excel in technical interviews but also develop a valuable skill set for solving complex problems in your future career. Remember, the goal is not just to memorize algorithms, but to understand the underlying principles and develop the ability to apply them creatively to new problems.
Happy learning, and best of luck with your interview preparation!