How to Structure Your Code for Readability During Interviews
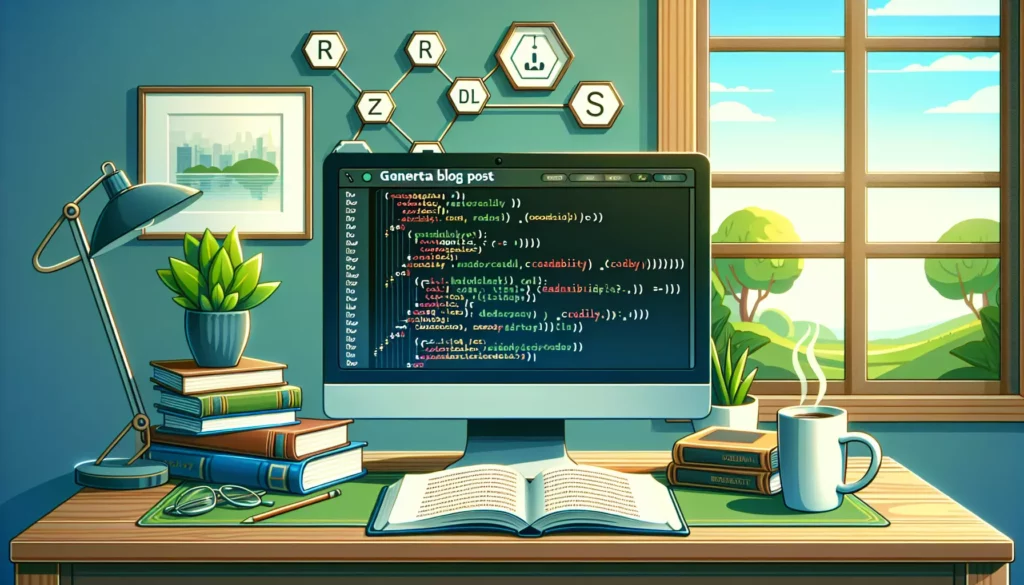
When it comes to technical interviews, particularly for positions at major tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google), your coding skills are put to the test. However, it’s not just about solving the problem; how you structure and present your code matters immensely. In this comprehensive guide, we’ll explore the art of structuring your code for maximum readability during interviews, a skill that can set you apart from other candidates and demonstrate your proficiency as a software engineer.
Why Code Structure and Readability Matter in Interviews
Before diving into the specifics of how to structure your code, it’s crucial to understand why this aspect is so important in an interview setting:
- First Impressions Count: Well-structured code creates a positive first impression, showing that you’re organized and professional.
- Demonstrates Clear Thinking: Clean code structure reflects clear, logical thinking – a highly valued trait in software development.
- Easier to Explain: When your code is well-structured, it’s easier to walk your interviewer through your thought process and solution.
- Simulates Real-world Scenarios: In actual work environments, you’ll often need to write code that others can easily read and maintain.
- Facilitates Debugging: If you need to debug or optimize your code during the interview, a clear structure makes this process much smoother.
Key Principles of Code Structuring for Readability
Now that we understand the importance of code structure, let’s explore the key principles that can help you achieve optimal readability:
1. Use Consistent Indentation
Proper indentation is the foundation of readable code. It visually represents the logical structure of your program, making it easier to understand the flow and hierarchy of your code.
Example of poor indentation:
def calculate_sum(a,b):
result = a + b
return result
for i in range(5):
print(calculate_sum(i, i+1))
Example of good indentation:
def calculate_sum(a, b):
result = a + b
return result
for i in range(5):
print(calculate_sum(i, i+1))
2. Use Meaningful Variable and Function Names
Choose descriptive names for your variables and functions. This practice makes your code self-documenting and easier to understand at a glance.
Poor naming:
def f(x, y):
return x * y
result = f(5, 3)
print(result)
Good naming:
def calculate_area(length, width):
return length * width
rectangle_area = calculate_area(5, 3)
print(rectangle_area)
3. Keep Functions Short and Focused
Each function should have a single, well-defined purpose. This principle, known as the Single Responsibility Principle, enhances readability and maintainability.
Example of a function doing too much:
def process_data(data):
# Sort the data
sorted_data = sorted(data)
# Calculate average
total = sum(sorted_data)
average = total / len(sorted_data)
# Find maximum
maximum = max(sorted_data)
# Print results
print(f"Sorted data: {sorted_data}")
print(f"Average: {average}")
print(f"Maximum: {maximum}")
return sorted_data, average, maximum
Better approach with separate functions:
def sort_data(data):
return sorted(data)
def calculate_average(data):
return sum(data) / len(data)
def find_maximum(data):
return max(data)
def print_results(sorted_data, average, maximum):
print(f"Sorted data: {sorted_data}")
print(f"Average: {average}")
print(f"Maximum: {maximum}")
def process_data(data):
sorted_data = sort_data(data)
average = calculate_average(sorted_data)
maximum = find_maximum(sorted_data)
print_results(sorted_data, average, maximum)
return sorted_data, average, maximum
4. Use Comments Judiciously
While comments can be helpful, aim for self-explanatory code. Use comments to explain why something is done, not what is being done.
Overuse of comments:
# Function to add two numbers
def add(a, b):
# Add a and b
result = a + b
# Return the result
return result
# Call the function and print the result
print(add(5, 3)) # This will print 8
Better use of comments:
def add(a, b):
return a + b
# Example usage of the add function
print(add(5, 3))
5. Consistent Formatting
Adhere to a consistent coding style throughout your solution. This includes consistent spacing, line breaks, and placement of brackets.
Inconsistent formatting:
def function1( param1, param2 ):
# Some code here
return result
def function2(param1,param2):
# Some code here
return result
Consistent formatting:
def function1(param1, param2):
# Some code here
return result
def function2(param1, param2):
# Some code here
return result
Advanced Structuring Techniques
As you progress in your coding journey and tackle more complex problems during interviews, consider these advanced structuring techniques:
1. Modularization
Break your solution into logical modules or components. This is particularly useful for complex problems.
class Solution:
def solve_complex_problem(self, input_data):
preprocessed_data = self._preprocess(input_data)
result = self._core_algorithm(preprocessed_data)
return self._postprocess(result)
def _preprocess(self, data):
# Preprocessing logic
pass
def _core_algorithm(self, data):
# Main algorithm logic
pass
def _postprocess(self, data):
# Postprocessing logic
pass
2. Use of Design Patterns
Implement appropriate design patterns when applicable. This demonstrates your understanding of software design principles.
class Singleton:
_instance = None
def __new__(cls):
if cls._instance is None:
cls._instance = super(Singleton, cls).__new__(cls)
return cls._instance
def some_business_logic(self):
# Singleton-specific logic
pass
3. Error Handling
Implement proper error handling to make your code more robust and show that you consider edge cases.
def divide(a, b):
try:
result = a / b
except ZeroDivisionError:
print("Error: Division by zero is not allowed.")
return None
except TypeError:
print("Error: Both arguments must be numbers.")
return None
else:
return result
4. Type Hinting (for Python)
Use type hints to make your code more self-documenting and demonstrate your attention to detail.
from typing import List, Tuple
def process_data(numbers: List[int]) -> Tuple[float, int]:
average = sum(numbers) / len(numbers)
maximum = max(numbers)
return average, maximum
Practical Tips for Structuring Code During Interviews
Now that we’ve covered the principles and techniques, let’s look at some practical tips to apply these concepts during an actual interview:
1. Start with a High-Level Plan
Before diving into coding, outline your approach. This can be done as comments or pseudocode.
# 1. Initialize data structures
# 2. Implement main algorithm logic
# 3. Handle edge cases
# 4. Optimize if time permits
2. Implement Core Logic First
Focus on getting the main algorithm working before optimizing or handling edge cases. This ensures you have a working solution to discuss.
3. Refactor as You Go
Don’t hesitate to refactor your code if you see an opportunity to improve its structure. Explain your thought process to the interviewer as you do this.
4. Use Helper Functions
Extract repeated or complex logic into helper functions. This improves readability and shows your ability to write modular code.
def is_valid(board, row, col, num):
# Check if the number can be placed in the given cell
pass
def solve_sudoku(board):
for row in range(9):
for col in range(9):
if board[row][col] == 0:
for num in range(1, 10):
if is_valid(board, row, col, num):
board[row][col] = num
if solve_sudoku(board):
return True
board[row][col] = 0
return False
return True
5. Be Consistent with Naming Conventions
Stick to a single naming convention throughout your code. For example, if you’re using snake_case for function names, use it consistently.
6. Leave Room for Improvements
If time allows, mention potential optimizations or improvements you would make to your code. This shows your ability to critically evaluate your own work.
Common Pitfalls to Avoid
While striving for well-structured code, be aware of these common pitfalls:
1. Over-Engineering
Don’t make your solution unnecessarily complex. Aim for simplicity and clarity.
2. Ignoring Time Constraints
Remember that you have limited time. Focus on a working solution first, then improve structure if time permits.
3. Neglecting to Explain
Don’t code in silence. Explain your thought process and structuring decisions as you go.
4. Inconsistent Formatting
Switching between different formatting styles can make your code look messy and hard to follow.
Adapting to Different Programming Languages
While the principles of code structuring are largely language-agnostic, some aspects may vary depending on the programming language you’re using in your interview. Here’s how you might adapt these principles for different popular languages:
Python
- Use 4 spaces for indentation (not tabs)
- Follow PEP 8 style guide
- Utilize list comprehensions and generator expressions for concise code
def get_even_numbers(numbers):
return [num for num in numbers if num % 2 == 0]
even_nums = get_even_numbers([1, 2, 3, 4, 5, 6])
print(even_nums) # Output: [2, 4, 6]
Java
- Use camelCase for method names and variables
- Place opening braces on the same line as the declaration
- Utilize interfaces and abstract classes for better structure
public class Solution {
public List<Integer> getEvenNumbers(List<Integer> numbers) {
return numbers.stream()
.filter(num -> num % 2 == 0)
.collect(Collectors.toList());
}
public static void main(String[] args) {
Solution solution = new Solution();
List<Integer> evenNums = solution.getEvenNumbers(Arrays.asList(1, 2, 3, 4, 5, 6));
System.out.println(evenNums); // Output: [2, 4, 6]
}
}
JavaScript
- Use camelCase for function and variable names
- Consider using ES6+ features like arrow functions and destructuring
- Utilize functional programming concepts when appropriate
const getEvenNumbers = (numbers) => numbers.filter(num => num % 2 === 0);
const evenNums = getEvenNumbers([1, 2, 3, 4, 5, 6]);
console.log(evenNums); // Output: [2, 4, 6]
C++
- Use snake_case for function and variable names
- Place opening braces on a new line
- Utilize header files for declarations and source files for implementations
#include <iostream>
#include <vector>
#include <algorithm>
std::vector<int> get_even_numbers(const std::vector<int>& numbers)
{
std::vector<int> even_nums;
std::copy_if(numbers.begin(), numbers.end(), std::back_inserter(even_nums),
[](int num) { return num % 2 == 0; });
return even_nums;
}
int main()
{
std::vector<int> nums = {1, 2, 3, 4, 5, 6};
auto even_nums = get_even_numbers(nums);
for (int num : even_nums)
{
std::cout << num << " ";
}
// Output: 2 4 6
return 0;
}
Conclusion: The Art of Structured Coding in Interviews
Mastering the art of structuring your code for readability is a crucial skill for succeeding in technical interviews, especially when aiming for positions at top tech companies. By following the principles and techniques outlined in this guide, you can demonstrate not just your problem-solving abilities, but also your capacity to write clean, maintainable, and professional-grade code.
Remember, the goal is not just to solve the problem, but to do so in a way that showcases your skills as a software engineer. Well-structured code tells a story – it reveals your thought process, your attention to detail, and your consideration for those who might read or work with your code in the future.
As you prepare for your interviews, practice these structuring techniques alongside your algorithm and data structure studies. Work on real-world problems, participate in coding challenges, and seek feedback on your code structure. With time and practice, writing well-structured code will become second nature, giving you a significant advantage in your technical interviews and your future career as a software developer.
Remember, every line of code you write is an opportunity to demonstrate your expertise. Make each one count!