How to Stay Organized in a Fast-Paced Development Team
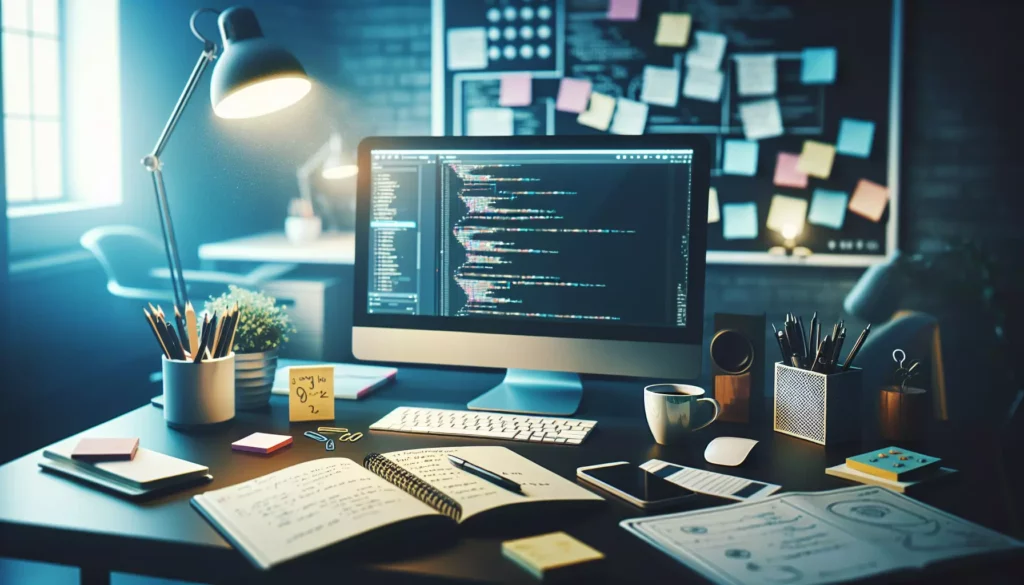
In the ever-evolving world of software development, staying organized is crucial for success, especially when working in a fast-paced team environment. As developers, we often juggle multiple tasks, collaborate with various team members, and face tight deadlines. This can quickly lead to chaos if not managed properly. In this comprehensive guide, we’ll explore effective strategies and tools to help you stay organized and productive in a high-velocity development team.
1. Embrace Agile Methodologies
Agile methodologies are designed to help teams work more efficiently and adapt to changes quickly. By implementing Agile practices, you can improve organization and productivity within your development team.
1.1. Scrum Framework
Scrum is one of the most popular Agile frameworks used in software development. It breaks down work into manageable chunks called “sprints,” typically lasting 1-4 weeks. Key elements of Scrum include:
- Sprint Planning: Prioritize and assign tasks for the upcoming sprint
- Daily Stand-ups: Brief team meetings to discuss progress and roadblocks
- Sprint Review: Demonstrate completed work to stakeholders
- Sprint Retrospective: Reflect on the sprint and identify areas for improvement
1.2. Kanban Boards
Kanban boards provide a visual representation of your team’s workflow. They help you track the progress of tasks and identify bottlenecks. A basic Kanban board consists of columns representing different stages of work, such as:
- To Do
- In Progress
- Code Review
- Testing
- Done
You can use physical boards or digital tools like Trello, Jira, or GitHub Projects to implement Kanban in your team.
2. Utilize Project Management Tools
Project management tools are essential for keeping your team organized and on track. They help you plan, execute, and monitor projects effectively.
2.1. Popular Project Management Tools
- Jira: Ideal for Agile teams, offering features like sprint planning, backlog management, and customizable workflows
- Asana: Great for task management, team collaboration, and project tracking
- Trello: Simple and intuitive Kanban-style boards for visual task management
- Microsoft Project: Comprehensive project management software for larger teams and complex projects
2.2. Key Features to Look For
When choosing a project management tool, consider the following features:
- Task assignment and tracking
- Time tracking and estimation
- Collaboration and communication features
- Reporting and analytics
- Integration with other tools (e.g., version control systems, communication platforms)
3. Implement Effective Version Control Practices
Version control is crucial for managing code changes and collaborating with team members. Git is the most widely used version control system, and implementing good Git practices can significantly improve your team’s organization.
3.1. Branching Strategies
Adopt a clear branching strategy to keep your codebase organized. Some popular strategies include:
- Git Flow: Uses separate branches for features, releases, and hotfixes
- GitHub Flow: Simplifies the process with a main branch and feature branches
- GitLab Flow: Combines feature branches with environment branches
3.2. Commit Messages and Pull Requests
Encourage team members to write clear, descriptive commit messages and create detailed pull requests. This helps in code review processes and makes it easier to track changes over time.
3.3. Code Review Process
Implement a structured code review process to ensure code quality and knowledge sharing within the team. Tools like GitHub, GitLab, or Bitbucket can help streamline this process.
4. Use Time Management Techniques
Effective time management is crucial in a fast-paced development environment. Here are some techniques to help you manage your time more efficiently:
4.1. Pomodoro Technique
The Pomodoro Technique involves working in focused 25-minute intervals (called “Pomodoros”), followed by short breaks. This can help improve concentration and prevent burnout.
4.2. Time Blocking
Allocate specific time blocks for different tasks or types of work. For example, you might dedicate the morning to coding, the early afternoon to meetings, and the late afternoon to code reviews.
4.3. Eisenhower Matrix
Prioritize tasks using the Eisenhower Matrix, which categorizes tasks based on their urgency and importance:
- Urgent and Important: Do immediately
- Important but Not Urgent: Schedule for later
- Urgent but Not Important: Delegate if possible
- Neither Urgent nor Important: Eliminate
5. Streamline Communication
Effective communication is essential for keeping your team organized and aligned. Here are some strategies to improve communication within your development team:
5.1. Choose the Right Communication Tools
Select appropriate tools for different types of communication:
- Slack or Microsoft Teams for instant messaging and quick discussions
- Zoom or Google Meet for video conferences
- Email for formal communications or discussions that require a paper trail
- Project management tools for task-related communication
5.2. Establish Communication Guidelines
Create clear guidelines for team communication, including:
- Response time expectations for different communication channels
- When to use each communication tool
- How to structure messages for clarity (e.g., using subject lines, tagging relevant people)
- Guidelines for asynchronous communication, especially for remote or distributed teams
5.3. Regular Team Meetings
Schedule regular team meetings to ensure everyone is aligned and up-to-date:
- Daily stand-ups for quick progress updates
- Weekly team meetings for more in-depth discussions
- Monthly or quarterly retrospectives to reflect on team performance and identify areas for improvement
6. Maintain Clean and Organized Codebases
A well-organized codebase is crucial for team productivity and code maintainability. Here are some strategies to keep your codebase clean and organized:
6.1. Follow Coding Standards
Establish and enforce coding standards within your team. This includes:
- Naming conventions for variables, functions, and classes
- Code formatting rules (indentation, line length, etc.)
- Best practices for your specific programming language(s)
Use linters and code formatters to automate the enforcement of these standards. For example, in a JavaScript project, you might use ESLint for linting and Prettier for code formatting:
.eslintrc.json:
{
"extends": ["eslint:recommended", "prettier"],
"plugins": ["prettier"],
"rules": {
"prettier/prettier": "error"
}
}
.prettierrc:
{
"singleQuote": true,
"trailingComma": "es5",
"printWidth": 100
}
6.2. Implement Modular Architecture
Design your codebase with modularity in mind. This makes it easier to understand, maintain, and scale your project. Some principles to follow include:
- Single Responsibility Principle: Each module or class should have a single, well-defined purpose
- Separation of Concerns: Divide your codebase into distinct sections, each addressing a separate concern
- Don’t Repeat Yourself (DRY): Avoid code duplication by extracting common functionality into reusable components
6.3. Documentation
Maintain up-to-date documentation for your codebase. This includes:
- README files explaining project setup and basic usage
- Inline code comments for complex logic
- API documentation for public interfaces
- Architecture diagrams for overall system design
Consider using documentation generators like JSDoc for JavaScript or Sphinx for Python to create comprehensive API documentation:
// Example JSDoc comment
/**
* Calculates the sum of two numbers.
* @param {number} a - The first number.
* @param {number} b - The second number.
* @returns {number} The sum of a and b.
*/
function sum(a, b) {
return a + b;
}
7. Continuous Integration and Continuous Deployment (CI/CD)
Implementing CI/CD practices can help keep your development process organized and efficient. CI/CD automates the building, testing, and deployment of your application, reducing manual errors and speeding up the development cycle.
7.1. Continuous Integration
CI involves automatically building and testing your code whenever changes are pushed to the repository. This helps catch integration issues early and ensures that the main branch is always in a working state. Popular CI tools include:
- Jenkins
- Travis CI
- CircleCI
- GitHub Actions
Here’s an example of a simple GitHub Actions workflow for a Node.js project:
.github/workflows/ci.yml:
name: CI
on: [push, pull_request]
jobs:
build:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Use Node.js
uses: actions/setup-node@v2
with:
node-version: '14.x'
- run: npm ci
- run: npm run build --if-present
- run: npm test
7.2. Continuous Deployment
CD automates the deployment process, allowing you to release new features and bug fixes more frequently and with less risk. This can involve:
- Automatic deployment to staging environments for testing
- One-click or fully automated deployment to production
- Rollback mechanisms in case of issues
Tools like Heroku, AWS CodeDeploy, or Kubernetes can help implement CD in your development workflow.
8. Regular Refactoring and Technical Debt Management
In fast-paced development environments, it’s easy to accumulate technical debt. Regular refactoring and proactive management of technical debt are crucial for maintaining a clean and organized codebase.
8.1. Schedule Refactoring Sessions
Allocate time for regular refactoring sessions. This could be:
- A dedicated “refactoring sprint” every few months
- A percentage of each sprint dedicated to refactoring tasks
- Regular “cleanup days” where the team focuses on improving code quality
8.2. Boy Scout Rule
Encourage team members to follow the “Boy Scout Rule”: Always leave the code better than you found it. This means making small improvements whenever you work on a piece of code, even if it’s not the main focus of your task.
8.3. Track Technical Debt
Use tools to track and visualize technical debt in your codebase. Some options include:
- SonarQube for code quality and security analysis
- CodeClimate for automated code review and quality metrics
- Creating and maintaining a technical debt backlog in your project management tool
9. Encourage Knowledge Sharing and Skill Development
Promoting a culture of knowledge sharing and continuous learning can help keep your team organized and up-to-date with best practices and new technologies.
9.1. Pair Programming and Code Reviews
Encourage pair programming sessions and thorough code reviews. These practices help spread knowledge throughout the team and catch potential issues early.
9.2. Internal Tech Talks and Workshops
Organize regular tech talks or workshops where team members can share their expertise or learnings from recent projects. This could include:
- Presentations on new technologies or techniques
- Deep dives into complex parts of your codebase
- Lessons learned from challenging projects or bugs
9.3. Learning Resources and Training
Provide access to learning resources and encourage continuous skill development. This could include:
- Subscriptions to online learning platforms like Pluralsight or Udemy
- Budget for attending conferences or workshops
- Internal mentorship programs
10. Regular Team Retrospectives
Conducting regular team retrospectives is crucial for continuous improvement and maintaining organization in a fast-paced environment.
10.1. Sprint Retrospectives
If you’re using Scrum, hold a retrospective at the end of each sprint. This meeting should focus on:
- What went well during the sprint
- What could be improved
- Action items for the next sprint
10.2. Longer-term Retrospectives
In addition to sprint retrospectives, consider holding longer-term retrospectives (e.g., quarterly) to look at broader trends and challenges. These sessions can help identify systemic issues and opportunities for improvement in your team’s processes and organization.
10.3. Action-oriented Outcomes
Ensure that retrospectives lead to concrete actions. For each identified area of improvement, assign an owner and a timeline for implementation. Follow up on these action items in subsequent meetings to ensure progress is being made.
Conclusion
Staying organized in a fast-paced development team is an ongoing process that requires commitment from every team member. By implementing the strategies discussed in this guide – from embracing Agile methodologies and utilizing project management tools to maintaining clean codebases and encouraging knowledge sharing – you can create a more efficient, productive, and organized development environment.
Remember, the key to success is not just implementing these practices, but also regularly reviewing and adjusting them to fit your team’s evolving needs. With consistent effort and a willingness to adapt, your team can thrive even in the most fast-paced development environments.