How to Start Thinking Like a Computer: Learning to Develop Step-by-Step Algorithms
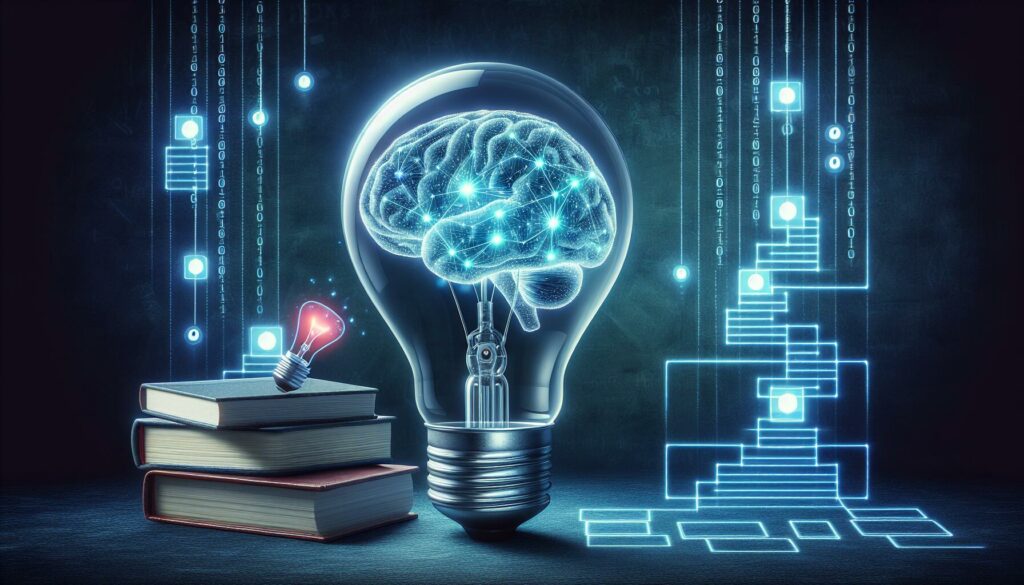
In today’s digital age, understanding how computers think and process information is becoming increasingly important. Whether you’re aspiring to become a programmer, looking to enhance your problem-solving skills, or simply curious about the inner workings of technology, learning to develop step-by-step algorithms is a valuable skill. This blog post will guide you through the process of thinking like a computer and developing algorithmic thinking skills that can be applied to various aspects of life and work.
What is Algorithmic Thinking?
Before we dive into the how-to, let’s first understand what algorithmic thinking means. Algorithmic thinking is a way of approaching problems and tasks by breaking them down into a series of clear, logical steps that can be followed to achieve a desired outcome. It’s the foundation of computer programming and is essential for creating efficient and effective solutions to complex problems.
In essence, algorithmic thinking involves:
- Breaking down problems into smaller, manageable parts
- Identifying patterns and repetitive tasks
- Creating step-by-step instructions to solve problems
- Considering different scenarios and edge cases
- Optimizing solutions for efficiency
Why is Algorithmic Thinking Important?
Developing algorithmic thinking skills offers numerous benefits, both in the world of programming and in everyday life:
- Improved Problem-Solving: It helps you approach problems systematically and find efficient solutions.
- Enhanced Logical Reasoning: You’ll become better at analyzing situations and making rational decisions.
- Increased Efficiency: By breaking down tasks into steps, you can identify areas for optimization and streamline processes.
- Better Communication: Algorithmic thinking improves your ability to explain complex ideas clearly and concisely.
- Career Opportunities: It’s a crucial skill for programming and many tech-related fields.
Steps to Develop Algorithmic Thinking
1. Start with Simple Problems
Begin by practicing with simple, everyday tasks. For example, let’s create an algorithm for making a peanut butter and jelly sandwich:
1. Gather ingredients: bread, peanut butter, jelly, and a knife
2. Place two slices of bread on a plate
3. Open the peanut butter jar
4. Use the knife to spread peanut butter on one slice of bread
5. Clean the knife
6. Open the jelly jar
7. Use the knife to spread jelly on the other slice of bread
8. Put the two slices together, with the peanut butter and jelly sides facing each other
9. Cut the sandwich diagonally (optional)
10. Serve and enjoy!
This simple example demonstrates how to break down a task into clear, sequential steps. As you practice, you’ll become more adept at identifying the necessary steps and their optimal order.
2. Learn to Identify Patterns
Computers excel at recognizing and executing patterns. Train yourself to spot repetitive elements in problems or tasks. For instance, consider the process of doing laundry:
1. Sort clothes by color
2. For each color group:
a. Load clothes into the washing machine
b. Add detergent
c. Set appropriate wash cycle
d. Start the machine
e. Wait for the cycle to complete
f. Transfer clothes to the dryer
g. Set appropriate drying cycle
h. Start the dryer
i. Wait for the cycle to complete
j. Remove clothes and fold
3. Put away folded clothes
Notice how steps 2a through 2j are repeated for each color group. Identifying such patterns is crucial in algorithmic thinking and programming, as it often leads to more efficient solutions through loops and functions.
3. Practice Pseudocode
Pseudocode is a way of describing algorithms using a mixture of natural language and basic programming constructs. It’s an excellent tool for planning and communicating your thought process without getting bogged down in specific programming language syntax. Here’s an example of pseudocode for a simple guessing game:
SET secretNumber to a random number between 1 and 100
SET attempts to 0
WHILE true
INCREMENT attempts
PROMPT user for a guess
IF guess equals secretNumber
PRINT "Congratulations! You guessed the number in " + attempts + " attempts."
BREAK
ELSE IF guess < secretNumber
PRINT "Too low. Try again."
ELSE
PRINT "Too high. Try again."
END IF
END WHILE
Practice writing pseudocode for various problems to improve your ability to structure algorithms logically.
4. Learn Basic Programming Concepts
While not strictly necessary for algorithmic thinking, understanding basic programming concepts can significantly enhance your ability to think like a computer. Familiarize yourself with concepts such as:
- Variables and data types
- Conditional statements (if-else)
- Loops (for, while)
- Functions
- Arrays and lists
These concepts form the building blocks of most algorithms and can help you express your ideas more precisely.
5. Practice Problem Decomposition
Problem decomposition is the art of breaking down complex problems into smaller, more manageable subproblems. This skill is crucial for tackling larger, more challenging tasks. Let’s practice with a more complex example: designing a basic ATM system.
First, let’s identify the main components of an ATM system:
- User Interface
- Account Verification
- Transaction Processing
- Cash Dispensing
- Receipt Printing
Now, let’s break down one of these components further. For example, the Transaction Processing component might include:
1. Check Balance
a. Retrieve account balance from database
b. Display balance to user
2. Withdraw Cash
a. Prompt user for withdrawal amount
b. Check if amount is available in account
c. If available:
i. Update account balance in database
ii. Signal cash dispenser to release funds
iii. Print receipt
d. If not available:
i. Display insufficient funds message
3. Deposit Cash
a. Prompt user to insert cash
b. Count inserted cash
c. Update account balance in database
d. Print receipt
4. Transfer Funds
a. Prompt user for recipient account number
b. Prompt user for transfer amount
c. Check if amount is available in account
d. If available:
i. Deduct amount from user's account
ii. Add amount to recipient's account
iii. Print receipt
e. If not available:
i. Display insufficient funds message
By breaking down the problem into smaller parts, you can focus on solving each subproblem individually, making the overall task more manageable.
6. Consider Edge Cases and Error Handling
An important aspect of algorithmic thinking is considering various scenarios, including edge cases and potential errors. This helps create more robust and reliable solutions. For example, in our ATM system, we should consider scenarios such as:
- What if the user enters an invalid PIN multiple times?
- How should the system handle network failures?
- What if the cash dispenser runs out of money?
- How to handle decimal amounts in transactions?
Thinking through these scenarios and incorporating appropriate error handling and edge case management is a crucial skill in algorithmic thinking and programming.
7. Learn to Optimize
As you become more comfortable with creating algorithms, start focusing on optimization. This involves finding ways to make your algorithms more efficient in terms of time (speed) and space (memory usage). Some common optimization techniques include:
- Reducing redundant operations
- Using appropriate data structures
- Implementing caching mechanisms
- Applying divide-and-conquer strategies
For example, let’s optimize a simple algorithm for finding the sum of numbers from 1 to n:
Naive approach:
function sumToN(n) {
let sum = 0;
for (let i = 1; i <= n; i++) {
sum += i;
}
return sum;
}
Optimized approach using the mathematical formula (n * (n + 1)) / 2:
function sumToN(n) {
return (n * (n + 1)) / 2;
}
The optimized version is much faster, especially for large values of n, as it performs a constant number of operations regardless of the input size.
8. Practice, Practice, Practice
Like any skill, algorithmic thinking improves with practice. Here are some ways to hone your skills:
- Solve Coding Challenges: Websites like LeetCode, HackerRank, and CodeWars offer a wide range of programming problems to solve.
- Participate in Coding Competitions: Platforms like Codeforces and TopCoder host regular coding contests that can help you improve your problem-solving skills under time pressure.
- Work on Personal Projects: Apply your algorithmic thinking to real-world problems by building your own applications or tools.
- Analyze Existing Algorithms: Study well-known algorithms and data structures to understand different problem-solving approaches.
- Collaborate with Others: Join coding communities or study groups to learn from and share ideas with fellow enthusiasts.
Tools and Resources for Developing Algorithmic Thinking
To aid your journey in developing algorithmic thinking skills, consider using the following tools and resources:
- Flowchart Tools: Platforms like Lucidchart or Draw.io can help you visualize algorithms and processes.
- Algorithm Visualization Websites: Websites like VisuAlgo offer interactive visualizations of various algorithms and data structures.
- Online Courses: Platforms like Coursera, edX, and Udacity offer courses on algorithms and computational thinking.
- Books: “Introduction to Algorithms” by Cormen et al. and “Grokking Algorithms” by Aditya Bhargava are excellent resources for learning about algorithms.
- Coding Platforms: Use platforms like repl.it or CodePen to quickly write and test code snippets.
Applying Algorithmic Thinking Beyond Programming
While algorithmic thinking is crucial for programming, its applications extend far beyond the realm of computer science. Here are some ways you can apply algorithmic thinking in everyday life:
- Project Management: Break down large projects into smaller, manageable tasks and create step-by-step plans for execution.
- Decision Making: Develop systematic approaches to evaluate options and make informed decisions.
- Time Management: Create efficient routines and processes to optimize your daily schedule.
- Problem Solving: Apply structured thinking to personal or professional challenges to find effective solutions.
- Learning New Skills: Break down complex skills into smaller, learnable components and create a structured learning plan.
Conclusion
Learning to think like a computer and develop step-by-step algorithms is a valuable skill that can enhance your problem-solving abilities, improve your logical reasoning, and open up new opportunities in the world of technology. By starting with simple problems, practicing pseudocode, learning basic programming concepts, and continually challenging yourself with more complex problems, you can develop strong algorithmic thinking skills.
Remember that becoming proficient in algorithmic thinking takes time and practice. Don’t get discouraged if you find some concepts challenging at first. Keep practicing, stay curious, and don’t hesitate to seek help from online resources or coding communities when you need it.
As you progress in your journey of algorithmic thinking, you’ll find that it not only helps you in programming but also enhances your ability to tackle complex problems in various aspects of life. So, start thinking like a computer today, and unlock a world of logical problem-solving and efficient decision-making!