How to Start Solving Coding Challenges as a Beginner
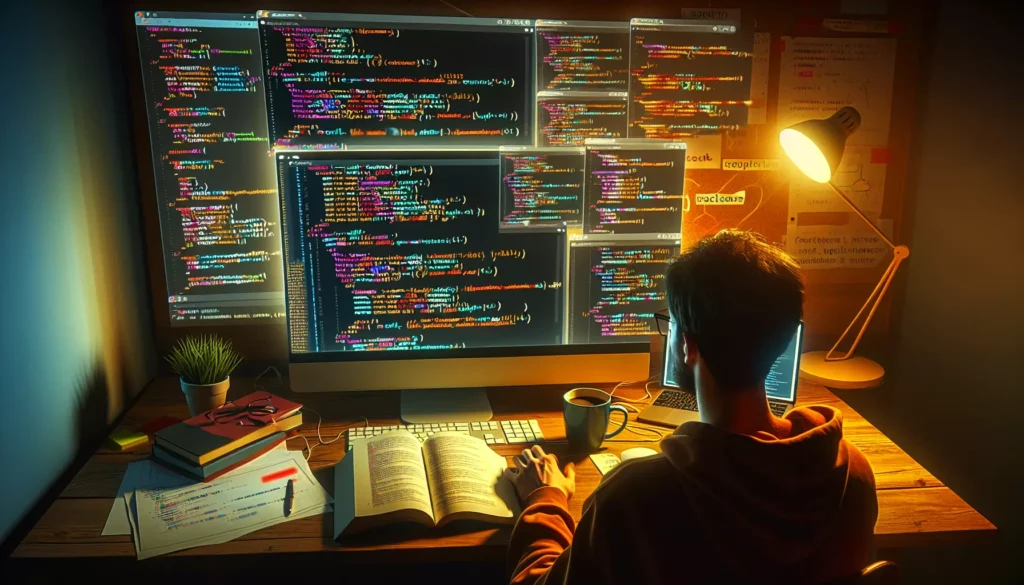
As a beginner in the world of programming, diving into coding challenges can seem like a daunting task. However, these challenges are essential for developing your problem-solving skills and preparing for technical interviews, especially if you’re aiming for positions at major tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google). In this comprehensive guide, we’ll walk you through the process of starting your journey with coding challenges, providing you with the tools and strategies you need to succeed.
1. Understanding the Importance of Coding Challenges
Before we dive into the “how,” let’s briefly discuss the “why.” Coding challenges are crucial for several reasons:
- They help you develop problem-solving skills
- They improve your understanding of data structures and algorithms
- They prepare you for technical interviews
- They enhance your coding efficiency and speed
- They expose you to different programming paradigms and techniques
By regularly practicing coding challenges, you’re not just learning to code; you’re learning to think like a programmer.
2. Setting Up Your Development Environment
Before you start solving challenges, you need a proper setup. Here’s what you’ll need:
2.1. Choose a Programming Language
As a beginner, it’s best to start with a beginner-friendly language. Python is often recommended due to its simplicity and readability. However, languages like Java, JavaScript, or C++ are also popular choices.
2.2. Install an Integrated Development Environment (IDE)
An IDE will make your coding life much easier. For Python, you might consider PyCharm or Visual Studio Code. For Java, Eclipse or IntelliJ IDEA are popular choices.
2.3. Set Up Version Control
Learn to use Git and create a GitHub account. This will help you track your progress and showcase your work to potential employers.
3. Starting with the Basics
Before jumping into complex algorithms, make sure you have a solid grasp of the basics:
3.1. Data Types and Variables
Understand different data types (integers, floats, strings, booleans) and how to declare and use variables.
3.2. Control Structures
Master if-else statements, loops (for and while), and switch cases.
3.3. Functions
Learn how to define and call functions, pass parameters, and return values.
3.4. Basic Data Structures
Familiarize yourself with arrays, lists, dictionaries (or hash maps), and sets.
4. Choosing the Right Platform for Coding Challenges
There are numerous platforms available for practicing coding challenges. Here are some popular ones:
- LeetCode: Offers a wide range of problems and is popular for interview preparation
- HackerRank: Provides challenges in various domains and skill levels
- CodeSignal: Offers both practice problems and real-world coding challenges
- Project Euler: Focuses on mathematical/computer programming problems
- AlgoCademy: Provides interactive coding tutorials and AI-powered assistance
As a beginner, you might want to start with platforms that offer a structured learning path, like AlgoCademy or HackerRank’s problem-solving track.
5. Approaching Your First Coding Challenge
When you’re ready to tackle your first challenge, follow these steps:
5.1. Read and Understand the Problem
Take your time to thoroughly read the problem statement. Make sure you understand:
- What are the inputs?
- What is the expected output?
- Are there any constraints or edge cases to consider?
5.2. Plan Your Approach
Before writing any code, think about how you would solve the problem. Consider:
- What algorithm or data structure might be useful?
- Can you break the problem down into smaller steps?
- Is there a brute force solution you can start with?
5.3. Write Pseudocode
Jot down the steps of your solution in plain English or pseudocode. This will serve as a roadmap for your actual code.
5.4. Implement Your Solution
Now, translate your pseudocode into actual code. Don’t worry about optimization at this stage; focus on getting a working solution.
5.5. Test Your Code
Run your code with the provided test cases. If there are no test cases, create your own, including edge cases.
5.6. Optimize and Refactor
Once you have a working solution, consider if there are ways to make it more efficient or readable.
6. Example: Solving a Simple Coding Challenge
Let’s walk through solving a simple coding challenge: “Write a function that returns the sum of two numbers.”
6.1. Read and Understand the Problem
Inputs: Two numbers (integers or floats)
Output: The sum of the two numbers
Constraints: None specified
6.2. Plan Your Approach
This is a straightforward arithmetic problem. We’ll create a function that takes two parameters and returns their sum.
6.3. Write Pseudocode
function sum_two_numbers(num1, num2):
return the result of num1 + num2
6.4. Implement Your Solution
Here’s how we might implement this in Python:
def sum_two_numbers(num1, num2):
return num1 + num2
# Test the function
print(sum_two_numbers(5, 3)) # Should print 8
print(sum_two_numbers(-1, 1)) # Should print 0
print(sum_two_numbers(3.14, 2.86)) # Should print 6.0
6.5. Test Your Code
Run the code with different test cases to ensure it works as expected.
6.6. Optimize and Refactor
In this case, the solution is already simple and efficient, so no further optimization is necessary.
7. Common Beginner Mistakes and How to Avoid Them
As you start solving coding challenges, be aware of these common pitfalls:
7.1. Not Reading the Problem Carefully
Always read the entire problem statement, including any constraints or special conditions.
7.2. Jumping Straight into Coding
Take the time to plan your approach before writing code. It will save you time in the long run.
7.3. Ignoring Edge Cases
Consider extreme or unusual inputs that might break your code.
7.4. Not Testing Thoroughly
Don’t rely solely on the provided test cases. Create your own to cover different scenarios.
7.5. Overcomplicating Solutions
Start with a simple, working solution before trying to optimize.
8. Developing Good Coding Habits
As you progress in your coding journey, cultivate these habits:
8.1. Write Clean, Readable Code
Use meaningful variable names, proper indentation, and comments where necessary.
8.2. Practice Regularly
Aim to solve at least one problem a day, even if it’s a simple one.
8.3. Learn from Others
After solving a problem, look at other people’s solutions to learn different approaches.
8.4. Review Core Concepts
Regularly revisit fundamental data structures and algorithms.
8.5. Time Yourself
As you improve, start timing your problem-solving to prepare for timed coding interviews.
9. Progressing to More Complex Challenges
As you become more comfortable with basic challenges, gradually increase the difficulty:
9.1. Explore Different Problem Types
Try problems involving strings, arrays, linked lists, trees, and graphs.
9.2. Learn and Implement Common Algorithms
Study sorting algorithms, searching algorithms, dynamic programming, and more.
9.3. Analyze Time and Space Complexity
Learn to evaluate the efficiency of your solutions using Big O notation.
9.4. Participate in Coding Contests
Join online coding competitions to challenge yourself and learn from others.
10. Leveraging AI-Powered Tools for Learning
As you progress, consider using AI-powered tools to enhance your learning experience. Platforms like AlgoCademy offer:
- Personalized learning paths based on your skill level
- AI-generated hints and explanations for challenging problems
- Real-time code analysis and feedback
- Virtual coding sessions that simulate interview environments
These tools can provide targeted assistance and help you identify areas for improvement more efficiently.
11. Preparing for Technical Interviews
As you become proficient in solving coding challenges, start focusing on interview preparation:
11.1. Study Common Interview Topics
Focus on data structures, algorithms, system design, and object-oriented programming concepts.
11.2. Practice Whiteboard Coding
Get comfortable explaining your thought process while coding without an IDE.
11.3. Mock Interviews
Conduct practice interviews with friends or use online platforms that offer mock interview services.
11.4. Review Company-Specific Questions
Research and practice questions commonly asked by companies you’re interested in.
12. Conclusion: Embracing the Journey
Starting to solve coding challenges as a beginner is an exciting journey of continuous learning and improvement. Remember that everyone starts somewhere, and consistent practice is key to success. Embrace the challenges, learn from your mistakes, and celebrate your progress along the way.
As you work through coding challenges, you’re not just preparing for interviews; you’re developing problem-solving skills that will serve you throughout your programming career. Whether you’re aiming for a position at a FAANG company or looking to improve your coding skills for personal projects, the process of tackling coding challenges will make you a better, more confident programmer.
Stay curious, keep challenging yourself, and don’t be afraid to seek help when you need it. With persistence and the right resources, you’ll be well on your way to mastering coding challenges and achieving your programming goals.