How to Start Learning to Code from Scratch: A Comprehensive Guide
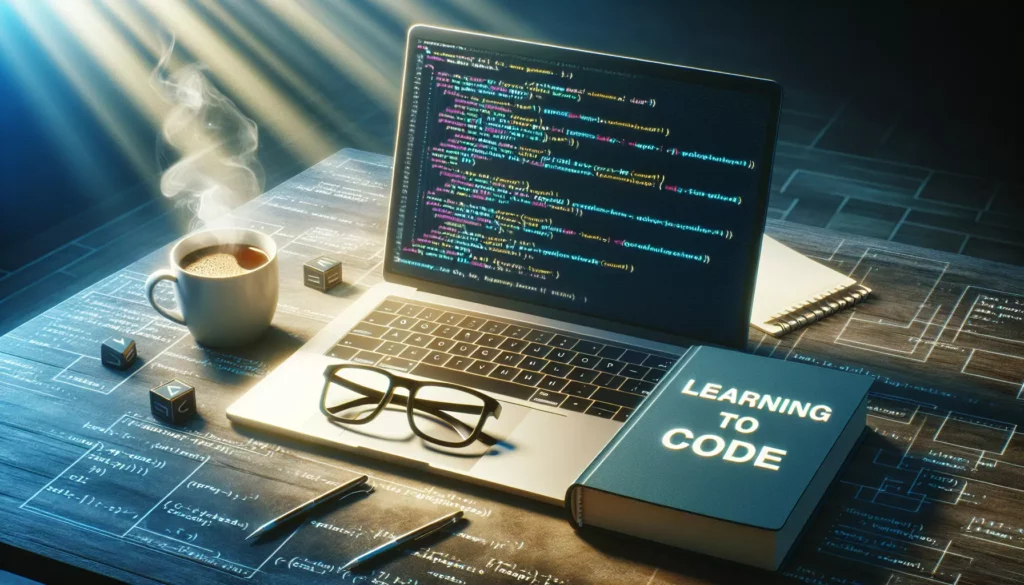
In today’s digital age, coding has become an increasingly valuable skill. Whether you’re looking to change careers, enhance your current job prospects, or simply explore a new hobby, learning to code can open up a world of opportunities. But for many, the prospect of starting from scratch can seem daunting. Where do you begin? What languages should you learn? How do you stay motivated? This comprehensive guide will walk you through the process of learning to code from the ground up, with a focus on practical steps and resources to help you succeed.
Table of Contents
- Why Learn to Code?
- Setting Your Coding Goals
- Choosing Your First Programming Language
- Essential Learning Resources
- Building a Strong Foundation
- Practice and Projects
- Joining a Coding Community
- Staying Motivated and Overcoming Challenges
- Moving to Advanced Topics
- Exploring Career Paths in Coding
- Preparing for Technical Interviews
- Continuous Learning and Growth
1. Why Learn to Code?
Before diving into the how, it’s important to understand the why. Learning to code offers numerous benefits:
- Career Opportunities: The tech industry is booming, with high demand for skilled programmers across various sectors.
- Problem-Solving Skills: Coding teaches you to think logically and approach problems systematically.
- Creativity: Programming allows you to bring your ideas to life, from websites to apps and beyond.
- Understanding Technology: Coding gives you insight into how the digital world around us works.
- Automation: You can create tools to automate repetitive tasks in your personal or professional life.
- Entrepreneurship: Coding skills can help you build your own products or start a tech-based business.
Understanding your motivation will help you stay committed to your learning journey, especially when faced with challenges.
2. Setting Your Coding Goals
Before you start learning, it’s crucial to set clear, achievable goals. This will help you focus your efforts and measure your progress. Consider the following questions:
- What do you want to achieve with coding? (e.g., career change, skill enhancement, hobby)
- What type of projects interest you? (e.g., web development, mobile apps, data analysis)
- How much time can you dedicate to learning each week?
- What’s your timeline for achieving your coding goals?
Based on your answers, create SMART (Specific, Measurable, Achievable, Relevant, Time-bound) goals. For example:
- “I will complete a basic HTML and CSS course within the next month, spending at least 5 hours per week on learning and practice.”
- “Within six months, I will build and deploy my first web application using JavaScript and a popular framework like React.”
Having clear goals will help you choose the right programming languages and resources to focus on.
3. Choosing Your First Programming Language
Selecting your first programming language can be overwhelming given the numerous options available. Here are some popular choices for beginners, along with their common applications:
- Python: Known for its simplicity and readability, Python is an excellent choice for beginners. It’s versatile and used in web development, data analysis, artificial intelligence, and more.
- JavaScript: If you’re interested in web development, JavaScript is essential. It’s used for both front-end and back-end development and is the language of the web.
- HTML/CSS: While not programming languages in the strictest sense, HTML and CSS are fundamental for web development and provide a good starting point for understanding how websites are structured and styled.
- Java: A popular choice for building large-scale applications, Java is widely used in enterprise environments and Android app development.
- C#: If you’re interested in game development or Windows application development, C# is a good option.
For absolute beginners, Python or JavaScript are often recommended due to their relatively gentle learning curves and wide applicability. Remember, the best language to start with is one that aligns with your goals and interests.
4. Essential Learning Resources
Once you’ve chosen a language, it’s time to start learning. Here are some types of resources you can use:
Online Courses and Tutorials
- Codecademy: Offers interactive courses on various programming languages.
- freeCodeCamp: Provides free, comprehensive coding courses with certifications.
- Coursera and edX: Offer university-level courses, some of which are free to audit.
- YouTube tutorials: Channels like Traversy Media, The Net Ninja, and Programming with Mosh offer free, in-depth coding tutorials.
Coding Platforms
- AlgoCademy: Offers interactive coding tutorials and resources for learners, with a focus on algorithmic thinking and problem-solving. It’s particularly useful for those preparing for technical interviews at major tech companies.
- LeetCode: Provides a platform for practicing coding problems, particularly useful for interview preparation.
- HackerRank: Offers coding challenges and competitions to improve your skills.
Books
- “Python Crash Course” by Eric Matthes
- “Eloquent JavaScript” by Marijn Haverbeke (available free online)
- “Head First Java” by Kathy Sierra and Bert Bates
Documentation
Official documentation for your chosen language is an invaluable resource. For example:
- Python: Python Documentation
- JavaScript: MDN Web Docs
Remember, everyone learns differently, so experiment with various resources to find what works best for you.
5. Building a Strong Foundation
As you begin learning, focus on building a strong foundation in programming basics. These concepts are universal across most programming languages:
Key Concepts to Master
- Variables and Data Types: Understand how to store and manipulate different types of data.
- Control Structures: Learn about if/else statements, loops, and switch statements.
- Functions: Understand how to create reusable blocks of code.
- Data Structures: Familiarize yourself with arrays, lists, dictionaries, and sets.
- Object-Oriented Programming (OOP): Learn about classes, objects, inheritance, and polymorphism.
- Basic Algorithms: Start with simple sorting and searching algorithms.
Here’s a simple Python example demonstrating some of these concepts:
# Variables and data types
name = "Alice" # string
age = 30 # integer
height = 5.6 # float
# Control structure (if statement)
if age >= 18:
print(f"{name} is an adult.")
else:
print(f"{name} is a minor.")
# Function
def greet(person):
return f"Hello, {person}!"
# Using the function
message = greet(name)
print(message)
# Data structure (list)
fruits = ["apple", "banana", "cherry"]
# Loop
for fruit in fruits:
print(f"I like {fruit}.")
# Basic class
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def introduce(self):
return f"My name is {self.name} and I'm {self.age} years old."
# Creating an object
alice = Person("Alice", 30)
print(alice.introduce())
This example covers variables, control structures, functions, data structures, loops, and a basic class. As you learn, try to understand how these concepts work together to create functional programs.
6. Practice and Projects
Theory is important, but coding is a skill best learned through practice. As you learn new concepts, immediately apply them by working on small projects or coding exercises. Here are some ideas to get you started:
Beginner Projects
- Calculator: Build a simple calculator that can perform basic arithmetic operations.
- To-Do List: Create a program that allows users to add, remove, and view tasks.
- Guess the Number Game: Develop a game where the computer generates a random number and the user tries to guess it.
- Password Generator: Build a tool that creates strong, random passwords based on user-specified criteria.
Intermediate Projects
- Weather App: Create an application that fetches and displays weather data from an API.
- Blog Website: Build a simple blog with features like creating, editing, and deleting posts.
- File Organizer: Develop a script that organizes files in a directory based on their types or other criteria.
- Tic-Tac-Toe Game: Implement the classic game with a graphical user interface.
As you work on these projects, you’ll encounter challenges that will push you to learn more and improve your problem-solving skills. Don’t be afraid to make mistakes – debugging is an essential part of the learning process.
Version Control
As you start working on projects, it’s a good time to learn about version control. Git is the most widely used version control system, and GitHub is a popular platform for hosting Git repositories. Learning Git will help you manage your code, collaborate with others, and contribute to open-source projects.
Here are some basic Git commands to get you started:
# Initialize a new Git repository
git init
# Add files to staging area
git add .
# Commit changes
git commit -m "Your commit message"
# Create a new branch
git branch new-feature
# Switch to a branch
git checkout new-feature
# Push changes to a remote repository
git push origin main
Understanding version control early in your coding journey will set you up for success in collaborative environments and help you manage your projects more effectively.
7. Joining a Coding Community
Learning to code doesn’t have to be a solitary journey. Joining a coding community can provide support, motivation, and valuable resources. Here are some ways to connect with fellow learners and experienced developers:
- Online Forums: Platforms like Stack Overflow, Reddit (r/learnprogramming, r/coding), and DEV Community are great places to ask questions and share knowledge.
- Coding Bootcamps: While often paid, bootcamps offer structured learning environments and opportunities to network with other aspiring developers.
- Meetups: Look for local coding meetups or tech events in your area. These can be great for networking and learning about industry trends.
- Open Source Projects: Contributing to open source projects is an excellent way to gain real-world experience and connect with other developers.
- Social Media: Follow developers, coding-focused accounts, and tech companies on platforms like Twitter and LinkedIn for insights and community engagement.
- Discord Servers: Many programming communities have Discord servers where you can chat with other learners and get help in real-time.
Remember to be respectful and follow community guidelines when participating in these spaces. Don’t be afraid to ask questions, but also try to help others when you can – teaching is an excellent way to reinforce your own learning.
8. Staying Motivated and Overcoming Challenges
Learning to code can be challenging, and it’s normal to face obstacles along the way. Here are some strategies to stay motivated and overcome common hurdles:
Set Realistic Expectations
Learning to code takes time and consistent effort. Don’t expect to become an expert overnight. Celebrate small victories and understand that struggling with concepts is a normal part of the learning process.
Break Down Large Goals
If you’re feeling overwhelmed, break your larger goals into smaller, manageable tasks. This makes progress more tangible and helps maintain momentum.
Establish a Routine
Set aside dedicated time for coding regularly. Consistency is key in building and retaining skills.
Use the Pomodoro Technique
Work in focused 25-minute intervals followed by short breaks. This can help maintain concentration and prevent burnout.
Find a Coding Buddy
Partner with another learner to share progress, challenges, and hold each other accountable.
Visualize Your Progress
Keep a log of what you’ve learned and the projects you’ve completed. Reflecting on your progress can be a great motivator.
Take Breaks
If you’re feeling frustrated, step away from your code for a while. Often, solutions become clearer after a short break.
Embrace the Learning Process
Remember that making mistakes and encountering bugs is part of learning. Each error is an opportunity to deepen your understanding.
When facing a particularly challenging problem, try the following approach:
- Break the problem down into smaller, manageable parts.
- Research and look for similar problems that have been solved.
- Experiment with different solutions.
- If you’re still stuck, don’t hesitate to ask for help in coding communities or forums.
9. Moving to Advanced Topics
As you become more comfortable with the basics, you’ll want to explore more advanced topics to deepen your skills. Here are some areas to consider:
Data Structures and Algorithms
A solid understanding of data structures (like trees, graphs, and hash tables) and algorithms (sorting, searching, dynamic programming) is crucial for writing efficient code and succeeding in technical interviews.
Design Patterns
Learn common solutions to recurring problems in software design. Understanding design patterns can help you write more maintainable and scalable code.
Databases
Learn about different types of databases (relational and non-relational) and how to interact with them programmatically.
APIs and Web Services
Understand how to create and consume APIs, which are crucial for building modern web and mobile applications.
Testing and Debugging
Learn about different testing methodologies (unit testing, integration testing) and debugging techniques to ensure your code is robust and error-free.
DevOps and Deployment
Familiarize yourself with concepts like continuous integration/continuous deployment (CI/CD), containerization (e.g., Docker), and cloud platforms (e.g., AWS, Azure, Google Cloud).
Specific Frameworks and Libraries
Depending on your chosen path, you might want to learn popular frameworks like React for front-end development, Django for Python web development, or TensorFlow for machine learning.
Here’s a simple example of how you might use a more advanced concept like recursion to solve a problem:
def factorial(n):
# Base case
if n == 0 or n == 1:
return 1
# Recursive case
else:
return n * factorial(n-1)
# Test the function
print(factorial(5)) # Output: 120
This recursive function calculates the factorial of a number. It demonstrates how complex problems can often be solved by breaking them down into simpler sub-problems.
10. Exploring Career Paths in Coding
As you advance in your coding journey, you might start considering potential career paths. The field of software development offers diverse opportunities, including:
- Front-End Developer: Focuses on creating the user interface and user experience of websites and web applications.
- Back-End Developer: Works on server-side logic, databases, and application integration.
- Full-Stack Developer: Combines front-end and back-end development skills.
- Mobile App Developer: Specializes in creating applications for iOS, Android, or cross-platform development.
- Data Scientist: Applies statistical and machine learning techniques to analyze and interpret complex data.
- DevOps Engineer: Focuses on the deployment, automation, and maintenance of software systems.
- Game Developer: Creates video games for various platforms.
- AI/Machine Learning Engineer: Develops systems and applications that utilize artificial intelligence and machine learning algorithms.
Research these roles to understand their specific requirements and the technologies they typically use. This can help guide your learning path and project choices.
11. Preparing for Technical Interviews
If you’re aiming for a career in software development, preparing for technical interviews is crucial. Here are some key areas to focus on:
Data Structures and Algorithms
Many technical interviews focus heavily on these topics. Practice implementing common data structures and solving algorithmic problems.
System Design
For more senior positions, you might be asked to design large-scale systems. Familiarize yourself with concepts like load balancing, caching, and database sharding.
Coding Challenges
Practice coding problems on platforms like LeetCode, HackerRank, and AlgoCademy. These can help you get comfortable with solving problems under time pressure.
Behavioral Questions
Prepare to discuss your projects, challenges you’ve overcome, and how you work in a team.
Mock Interviews
Practice with friends or use platforms that offer mock interview services to get comfortable with the interview process.
Remember, interview preparation is not just about memorizing solutions, but about understanding problem-solving approaches and being able to communicate your thought process clearly.
12. Continuous Learning and Growth
The field of technology is constantly evolving, and as a developer, continuous learning is essential. Here are some strategies for staying up-to-date and growing your skills:
Follow Tech News and Blogs
Stay informed about new technologies, languages, and industry trends. Websites like Hacker News, TechCrunch, and language-specific blogs are good sources.
Attend Conferences and Workshops
These events can provide valuable insights into new technologies and best practices, as well as networking opportunities.
Contribute to Open Source
Contributing to open source projects can help you gain experience, learn from others, and give back to the community.
Read Books and Research Papers
Dive deeper into specific topics through books and academic papers. This can give you a more thorough understanding of complex concepts.
Build Side Projects
Personal projects allow you to explore new technologies and keep your skills sharp.
Teach Others
Teaching or mentoring can deepen your own understanding and expose you to different perspectives.
Remember, learning to code is a journey, not a destination. Embrace the process of continuous improvement and stay curious about new developments in the field.
Conclusion
Starting your coding journey from scratch can seem daunting, but with the right approach and resources, it’s an achievable and rewarding endeavor. Remember to set clear goals, choose a language that aligns with your interests, utilize a variety of learning resources, practice consistently, and engage with the coding community. As you progress, don’t forget to celebrate your achievements, no matter how small they may seem.
Coding is not just about memorizing syntax or following tutorials – it’s about developing problem-solving skills, creativity, and a mindset of continuous learning. Embrace the challenges, stay curious, and don’t be afraid to make mistakes. With persistence and dedication, you’ll be amazed at how much you can achieve.
Whether your goal is to change careers, enhance your current job prospects, or simply explore a new hobby, learning to code can open up a world of possibilities. So take that first step, write your first line of code, and embark on this exciting journey of discovery and creation. Happy coding!