How to Spot the Hidden Hints in Coding Interview Questions
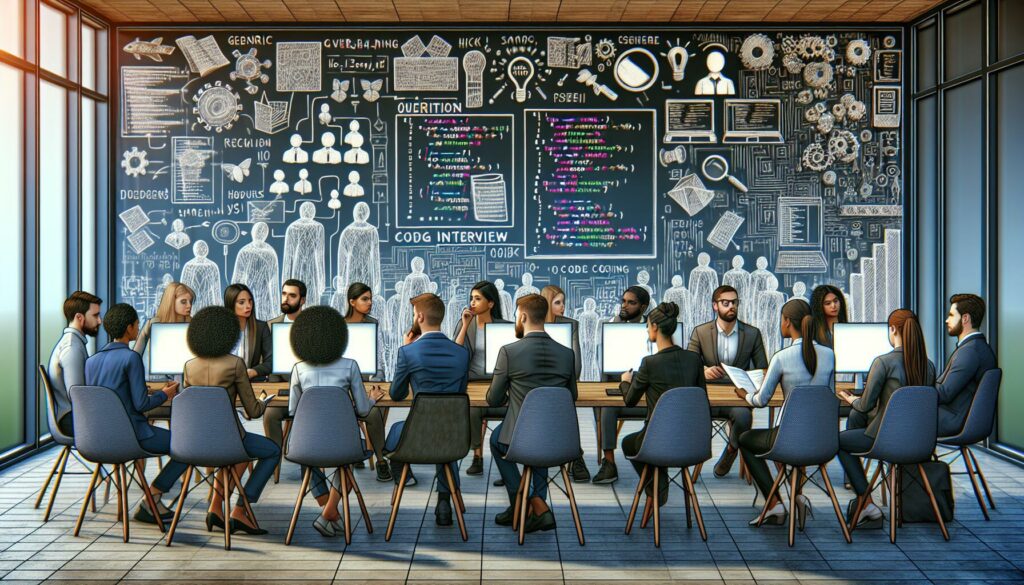
Coding interviews can be daunting, especially when you’re faced with complex algorithmic problems under time pressure. However, there’s a secret that many successful candidates have discovered: the problem descriptions often contain subtle hints that can lead you to the optimal solution. In this comprehensive guide, we’ll explore how to identify and leverage these hidden clues to ace your next coding interview.
The Importance of Reading Between the Lines
Before we dive into specific examples, it’s crucial to understand why spotting these hidden hints is so important:
- Efficiency: Recognizing hints can help you arrive at the most efficient solution more quickly.
- Interviewer Expectations: Interviewers often include these hints intentionally, expecting candidates to pick up on them.
- Problem-Solving Skills: Identifying subtle clues demonstrates your analytical thinking and attention to detail.
- Time Management: Quickly grasping the optimal approach saves valuable time during the interview.
Common Types of Hidden Hints
Let’s explore some of the most common types of hidden hints you might encounter in coding interview questions:
1. Input Characteristics
The nature of the input data often provides crucial information about the expected solution:
- Sorted vs. Unsorted
- Positive vs. Negative Numbers
- Size Constraints
- Data Types
2. Output Requirements
Pay close attention to what the question asks for in the output:
- Single Value vs. Multiple Values
- Exact Answer vs. Approximation
- Order of Results
3. Constraints and Edge Cases
Look for any mentioned constraints or special cases:
- Time Complexity Requirements
- Space Complexity Limitations
- Special Cases or Exceptions
4. Problem Wording
Sometimes, the choice of words in the problem statement can be a hint:
- Specific Terminology
- Analogies or Real-World Scenarios
- Emphasis on Certain Aspects
Real-World Examples: Decoding the Hints
Now, let’s examine some famous coding interview problems and uncover the hidden hints within them:
Example 1: Two Sum Problem
Problem Statement: Given an array of integers nums
and an integer target
, return indices of the two numbers such that they add up to target
.
Hidden Hint: The problem doesn’t specify whether the array is sorted or not. This is a crucial piece of information that leads to different optimal solutions.
Case A: Unsorted Array
When the array is unsorted, the optimal solution involves using a hash map:
def two_sum(nums, target):
num_map = {}
for i, num in enumerate(nums):
complement = target - num
if complement in num_map:
return [num_map[complement], i]
num_map[num] = i
return []
Time Complexity: O(n)
Space Complexity: O(n)
Case B: Sorted Array
If the array is sorted, we can use the two-pointer technique:
def two_sum_sorted(nums, target):
left, right = 0, len(nums) - 1
while left < right:
current_sum = nums[left] + nums[right]
if current_sum == target:
return [left, right]
elif current_sum < target:
left += 1
else:
right -= 1
return []
Time Complexity: O(n)
Space Complexity: O(1)
The key takeaway here is that the sorting status of the array dramatically affects the approach. Always clarify this with your interviewer if it’s not explicitly stated.
Example 2: Subarray Sum Equals K
Problem Statement: Given an array of integers nums
and an integer k
, return the total number of continuous subarrays whose sum equals to k
.
Hidden Hint: The problem doesn’t specify that the integers are non-negative. This subtle detail is crucial for choosing the right approach.
Case A: Array with Positive and Negative Integers
When the array can contain both positive and negative integers, we need to use a cumulative sum approach with a hash map:
def subarray_sum(nums, k):
count = 0
sum_map = {0: 1}
current_sum = 0
for num in nums:
current_sum += num
if current_sum - k in sum_map:
count += sum_map[current_sum - k]
sum_map[current_sum] = sum_map.get(current_sum, 0) + 1
return count
Time Complexity: O(n)
Space Complexity: O(n)
Case B: Array with Non-Negative Integers Only
If all integers are non-negative, we can use the sliding window technique:
def subarray_sum_non_negative(nums, k):
count = 0
left = 0
current_sum = 0
for right in range(len(nums)):
current_sum += nums[right]
while current_sum > k and left <= right:
current_sum -= nums[left]
left += 1
if current_sum == k:
count += 1
return count
Time Complexity: O(n)
Space Complexity: O(1)
The non-negative constraint allows for a more space-efficient solution, as the sum always increases or stays the same when moving the right pointer.
Example 3: Longest Palindromic Substring
Problem Statement: Given a string s
, return the longest palindromic substring in s
.
Hidden Hint: The problem asks for a substring, not a subsequence. This distinction is crucial for choosing the right approach.
Here’s an efficient solution using the expand around center technique:
def longest_palindrome(s):
if not s:
return ""
start = end = 0
def expand_around_center(left, right):
while left >= 0 and right < len(s) and s[left] == s[right]:
left -= 1
right += 1
return right - left - 1
for i in range(len(s)):
len1 = expand_around_center(i, i)
len2 = expand_around_center(i, i + 1)
max_len = max(len1, len2)
if max_len > end - start:
start = i - (max_len - 1) // 2
end = i + max_len // 2
return s[start:end+1]
Time Complexity: O(n^2)
Space Complexity: O(1)
The key insight here is that we need to consider both odd and even-length palindromes, which is why we call expand_around_center
twice for each position.
Strategies for Spotting Hidden Hints
Now that we’ve seen some examples, let’s discuss strategies for identifying these hidden hints in your coding interviews:
1. Carefully Analyze the Input
Always pay close attention to the characteristics of the input:
- Is the input sorted or unsorted?
- Are there any constraints on the values (e.g., non-negative integers only)?
- What’s the size of the input? Is it small enough for a brute force approach?
2. Consider the Output Requirements
The desired output can often guide you towards the right approach:
- Do you need to return all solutions or just one?
- Is the order of the output important?
- Are you required to modify the input in-place?
3. Look for Special Cases or Constraints
Pay attention to any mentioned edge cases or specific requirements:
- Are there any time or space complexity constraints?
- Are there any special cases that need to be handled differently?
4. Analyze the Problem Wording
Sometimes, the choice of words in the problem statement can be a hint:
- Look for specific terminology that might suggest a particular data structure or algorithm.
- Pay attention to any analogies or real-world scenarios mentioned, as they might guide you towards a solution.
5. Ask Clarifying Questions
Don’t hesitate to ask your interviewer for clarification:
- If the sorting status of an array is unclear, ask about it.
- If the range of input values isn’t specified, inquire about possible constraints.
- If you’re unsure about how to handle certain edge cases, seek clarification.
Common Hint Patterns to Watch For
As you practice more coding interview questions, you’ll start to recognize certain patterns in how hints are embedded. Here are some common ones to watch for:
1. “Efficient” or “Optimize” in the Problem Statement
When a problem explicitly asks for an efficient or optimized solution, it’s often a hint that there’s a non-obvious approach that’s better than the brute force method. This could involve using a specific data structure (like a heap or hash table) or a particular algorithm (like dynamic programming or a greedy approach).
2. Sorted Input
If an input array is specified as sorted, it’s almost always a hint. Sorted arrays often allow for more efficient solutions using techniques like binary search or the two-pointer method.
3. Constraints on Input Values
Pay attention to constraints like “non-negative integers” or “values between 1 and n”. These can often be leveraged for more efficient solutions or to use certain techniques that wouldn’t work with unrestricted values.
4. Problem Involves Searching or Matching
If a problem involves searching for elements or matching patterns, it’s often a hint to consider using hash tables or tries for efficiency.
5. Mention of “Contiguous” or “Consecutive”
When a problem mentions contiguous subarrays or consecutive elements, it’s often a hint to consider sliding window techniques or dynamic programming approaches.
6. Problems Involving Trees or Graphs
If a problem involves trees or graphs, pay attention to any mentions of “balanced”, “binary”, or specific graph types. These can be hints about which traversal or search algorithms might be most appropriate.
Practicing Hint Recognition
Improving your ability to spot hidden hints is a skill that comes with practice. Here are some ways to hone this skill:
1. Solve Many Problems
The more problems you solve, the better you’ll become at recognizing patterns and hints. Aim to solve a diverse range of problems to expose yourself to different types of hints.
2. Review Solutions
After solving a problem, always review the most efficient solutions, even if you solved it correctly. Pay attention to how these solutions leverage the problem’s characteristics.
3. Analyze Your Mistakes
When you miss a hint or choose a suboptimal approach, take time to understand why. What information did you overlook? How could you have interpreted the problem statement differently?
4. Practice Active Reading
When reading a problem statement, practice active reading. Try to identify potential hints as you go, and make note of any aspects that seem particularly important or unusual.
5. Discuss with Others
Discussing problems with other programmers can help you gain new perspectives on how to interpret problem statements and spot hints.
Conclusion
Mastering the art of spotting hidden hints in coding interview questions is a valuable skill that can significantly improve your performance. By paying close attention to the problem statement, input characteristics, and constraints, you can often uncover clues that lead to more efficient solutions.
Remember, the ability to recognize these hints comes with practice and experience. As you work through more coding problems, you’ll develop a sharper eye for these subtle clues. Don’t be discouraged if you miss some hints initially – even experienced programmers sometimes overlook them.
Keep practicing, stay curious, and always be on the lookout for those hidden gems of information in your coding interviews. With time and dedication, you’ll find yourself naturally gravitating towards optimal solutions, impressing your interviewers, and boosting your confidence in tackling complex algorithmic challenges.
Happy coding, and may your next coding interview be filled with successfully spotted hints and brilliantly optimized solutions!