How to Solve Real-World Problems Using Dynamic Programming
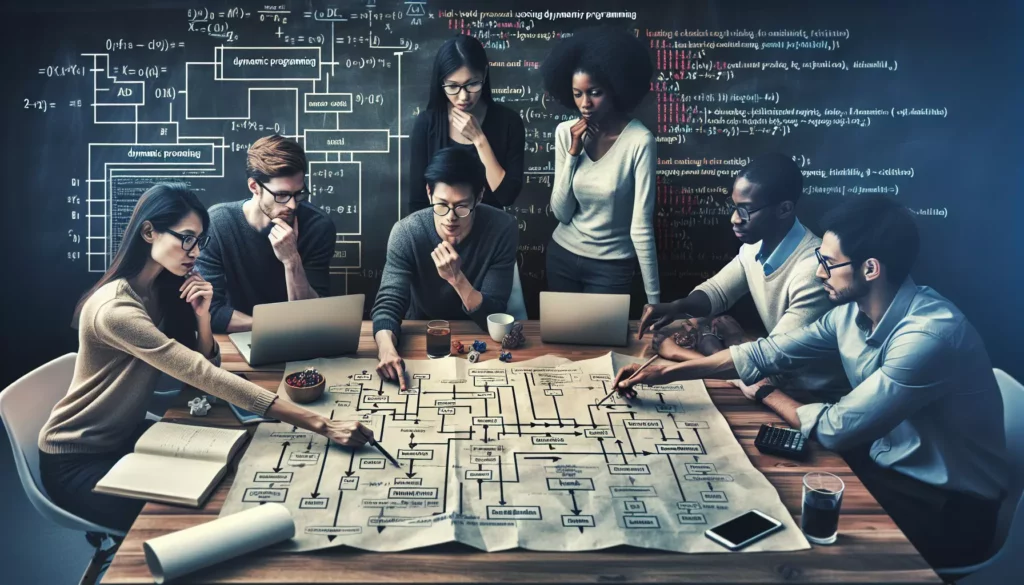
Dynamic programming (DP) is a powerful problem-solving technique that can be applied to a wide range of real-world problems. It’s an essential tool in the arsenal of any programmer or computer scientist, particularly when preparing for technical interviews at major tech companies. In this comprehensive guide, we’ll explore how to use dynamic programming to tackle complex problems efficiently, with a focus on practical applications and step-by-step problem-solving strategies.
Understanding Dynamic Programming
Before diving into real-world applications, let’s briefly review what dynamic programming is and why it’s so valuable.
What is Dynamic Programming?
Dynamic programming is an algorithmic paradigm that solves complex problems by breaking them down into simpler subproblems. It is applicable when the subproblems overlap and have optimal substructure. The key idea is to store the results of subproblems to avoid redundant computations, thus improving efficiency.
Key Characteristics of Dynamic Programming Problems
- Overlapping Subproblems: The problem can be broken down into subproblems which are reused several times.
- Optimal Substructure: The optimal solution to the problem can be constructed from optimal solutions of its subproblems.
Approaches to Dynamic Programming
- Top-down (Memoization): Start with the main problem and recursively break it down, storing results for subproblems.
- Bottom-up (Tabulation): Start by solving the smallest subproblems and work your way up to the main problem.
Real-World Applications of Dynamic Programming
Now, let’s explore some real-world problems that can be efficiently solved using dynamic programming techniques.
1. Optimizing Resource Allocation
Problem: A company needs to allocate resources (e.g., time, money, personnel) across different projects to maximize overall productivity.
DP Approach:
- Define the state: dp[i][j] represents the maximum productivity achieved using the first i resources and j projects.
- Establish the recurrence relation: dp[i][j] = max(dp[i-1][j], dp[i-1][j-1] + productivity[i][j])
- Initialize base cases: dp[0][j] = 0 and dp[i][0] = 0
- Iterate through all states and fill the DP table
- The final answer will be in dp[n][m], where n is the number of resources and m is the number of projects
Code Example:
def optimize_resource_allocation(productivity, n, m):
dp = [[0] * (m + 1) for _ in range(n + 1)]
for i in range(1, n + 1):
for j in range(1, m + 1):
dp[i][j] = max(dp[i-1][j], dp[i-1][j-1] + productivity[i-1][j-1])
return dp[n][m]
# Example usage
productivity = [
[3, 2, 4],
[4, 1, 5],
[5, 3, 2]
]
n, m = 3, 3
max_productivity = optimize_resource_allocation(productivity, n, m)
print(f"Maximum productivity: {max_productivity}")
2. Shortest Path in a Network
Problem: Finding the shortest path between two nodes in a network (e.g., road networks, computer networks).
DP Approach: We can use the Floyd-Warshall algorithm, which is a dynamic programming solution for finding shortest paths in a weighted graph with positive or negative edge weights.
Code Example:
def floyd_warshall(graph):
n = len(graph)
dist = [row[:] for row in graph] # Create a copy of the graph
for k in range(n):
for i in range(n):
for j in range(n):
dist[i][j] = min(dist[i][j], dist[i][k] + dist[k][j])
return dist
# Example usage
INF = float('inf')
graph = [
[0, 5, INF, 10],
[INF, 0, 3, INF],
[INF, INF, 0, 1],
[INF, INF, INF, 0]
]
shortest_paths = floyd_warshall(graph)
print("Shortest distances between all pairs of vertices:")
for row in shortest_paths:
print(row)
3. Text Justification
Problem: Given a sequence of words and a line width, format the text such that each line has exactly the given number of spaces, distributing spaces evenly between words.
DP Approach:
- Define the state: dp[i] represents the minimum cost of justifying words from index i to the end.
- Establish the recurrence relation: dp[i] = min(cost(i,j) + dp[j+1]) for all valid j
- Initialize base case: dp[n] = 0, where n is the number of words
- Iterate through all states from right to left
- Reconstruct the solution using the computed dp values
Code Example:
def text_justification(words, line_width):
n = len(words)
dp = [float('inf')] * (n + 1)
dp[n] = 0
def cost(i, j):
line_length = sum(len(word) for word in words[i:j+1]) + (j - i)
if line_length > line_width:
return float('inf')
return (line_width - line_length) ** 2
for i in range(n - 1, -1, -1):
for j in range(i, n):
dp[i] = min(dp[i], cost(i, j) + dp[j+1])
# Reconstruct the solution
result = []
i = 0
while i < n:
for j in range(i, n):
if dp[i] == cost(i, j) + dp[j+1]:
result.append(' '.join(words[i:j+1]))
i = j + 1
break
return result
# Example usage
words = ["This", "is", "an", "example", "of", "text", "justification."]
line_width = 16
justified_text = text_justification(words, line_width)
for line in justified_text:
print(line)
4. Portfolio Optimization
Problem: Determine the optimal allocation of funds across different investment options to maximize returns while minimizing risk.
DP Approach:
- Define the state: dp[i][j] represents the maximum return achievable with i investment options and j units of investment.
- Establish the recurrence relation: dp[i][j] = max(dp[i-1][j], dp[i-1][j-k] + returns[i][k]) for all valid k
- Initialize base cases: dp[0][j] = 0 and dp[i][0] = 0
- Iterate through all states and fill the DP table
- The final answer will be in dp[n][m], where n is the number of investment options and m is the total investment amount
Code Example:
def portfolio_optimization(returns, risk_levels, max_risk, total_investment):
n = len(returns)
dp = [[0] * (total_investment + 1) for _ in range(n + 1)]
for i in range(1, n + 1):
for j in range(1, total_investment + 1):
for k in range(j + 1):
if risk_levels[i-1] * k <= max_risk:
dp[i][j] = max(dp[i][j], dp[i-1][j-k] + returns[i-1] * k)
return dp[n][total_investment]
# Example usage
returns = [0.05, 0.07, 0.10, 0.12] # Expected returns
risk_levels = [1, 2, 3, 4] # Risk levels (1-10 scale)
max_risk = 5
total_investment = 10000
max_return = portfolio_optimization(returns, risk_levels, max_risk, total_investment)
print(f"Maximum return: ${max_return:.2f}")
5. Supply Chain Optimization
Problem: Optimize the flow of goods through a supply chain network to minimize costs while meeting demand.
DP Approach:
- Define the state: dp[i][j] represents the minimum cost to satisfy demand up to node i with j units of inventory.
- Establish the recurrence relation: dp[i][j] = min(dp[i-1][k] + transport_cost(k, j) + storage_cost(j)) for all valid k
- Initialize base cases: dp[0][j] = initial_cost(j)
- Iterate through all nodes and inventory levels
- The final answer will be the minimum value in the last row of the DP table
Code Example:
def supply_chain_optimization(demands, transport_costs, storage_costs, max_inventory):
n = len(demands)
dp = [[float('inf')] * (max_inventory + 1) for _ in range(n)]
# Initialize base case
for j in range(max_inventory + 1):
dp[0][j] = transport_costs[0][j]
for i in range(1, n):
for j in range(demands[i], max_inventory + 1):
for k in range(demands[i-1], j + 1):
cost = dp[i-1][k] + transport_costs[i][j-k] + storage_costs[i][j-demands[i]]
dp[i][j] = min(dp[i][j], cost)
return min(dp[n-1])
# Example usage
demands = [10, 20, 15, 25]
transport_costs = [
[0, 10, 20, 30, 40],
[0, 8, 16, 24, 32],
[0, 12, 24, 36, 48],
[0, 9, 18, 27, 36]
]
storage_costs = [
[0, 2, 4, 6, 8],
[0, 3, 6, 9, 12],
[0, 1, 2, 3, 4],
[0, 4, 8, 12, 16]
]
max_inventory = 40
min_cost = supply_chain_optimization(demands, transport_costs, storage_costs, max_inventory)
print(f"Minimum supply chain cost: ${min_cost}")
Strategies for Solving DP Problems
When approaching a problem that might be solvable using dynamic programming, consider the following strategies:
- Identify the subproblems: Break down the main problem into smaller, overlapping subproblems.
- Define the state: Determine what information needs to be stored to represent a subproblem.
- Establish the recurrence relation: Figure out how the solution to a subproblem relates to solutions of smaller subproblems.
- Identify base cases: Determine the simplest subproblems that can be solved directly.
- Decide on the DP approach: Choose between top-down (memoization) or bottom-up (tabulation) based on the problem’s characteristics and constraints.
- Implement the solution: Write the code, ensuring proper handling of the DP table or memoization cache.
- Optimize if necessary: Look for opportunities to reduce time or space complexity, such as using rolling arrays or eliminating unnecessary state variables.
Common Pitfalls and How to Avoid Them
When working with dynamic programming, be aware of these common pitfalls:
- Incorrect state definition: Ensure your state captures all necessary information to solve subproblems.
- Overlooking edge cases: Always consider and handle edge cases in your implementation.
- Inefficient state space: Try to minimize the dimensions of your DP table to reduce time and space complexity.
- Redundant computations: Make sure you’re not recomputing subproblems unnecessarily, especially in recursive implementations.
- Off-by-one errors: Be careful with array indexing, especially when dealing with 0-based and 1-based indexing.
- Incorrect initialization: Properly initialize your DP table or memoization cache, including base cases.
Conclusion
Dynamic programming is a powerful technique for solving complex problems efficiently by breaking them down into smaller, manageable subproblems. By applying DP to real-world scenarios such as resource allocation, network optimization, text formatting, portfolio management, and supply chain optimization, we can develop efficient solutions to challenging problems.
As you continue to practice and apply dynamic programming, you’ll develop a better intuition for recognizing DP-solvable problems and implementing elegant solutions. This skill is particularly valuable when preparing for technical interviews at major tech companies, where algorithmic problem-solving abilities are highly prized.
Remember that mastering dynamic programming takes time and practice. Start with simpler problems and gradually work your way up to more complex scenarios. With persistence and dedication, you’ll be able to tackle a wide range of real-world problems using this powerful algorithmic technique.