How to Solve Problems with Bitwise Manipulation
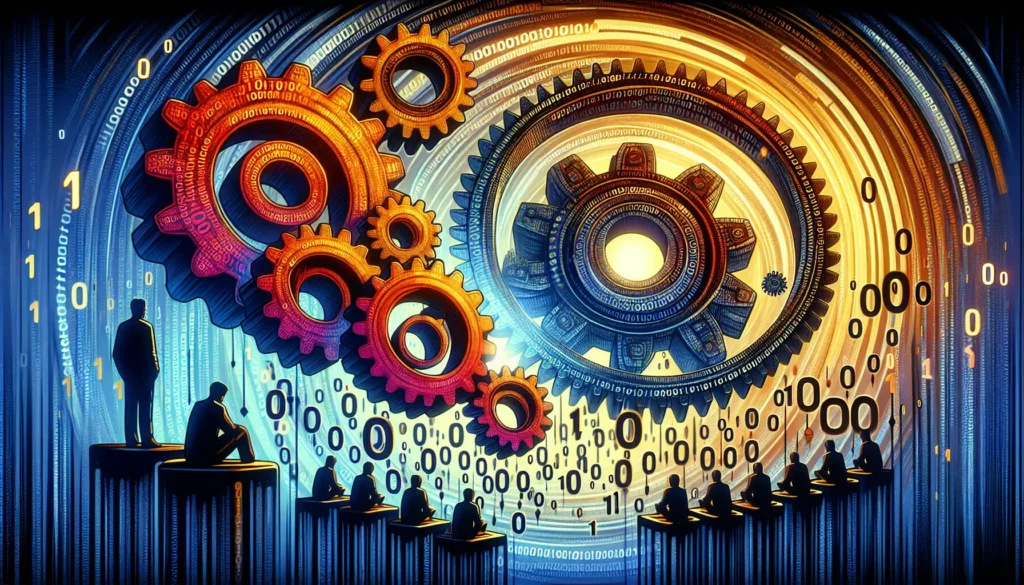
In the world of computer programming, bitwise manipulation is a powerful technique that can significantly optimize your code and solve complex problems efficiently. This article will dive deep into the art of bitwise manipulation, exploring its fundamentals, common operations, and practical applications in problem-solving. Whether you’re a beginner looking to expand your coding skills or an experienced developer preparing for technical interviews at top tech companies, mastering bitwise manipulation can give you a competitive edge.
Understanding Bitwise Operations
Before we delve into problem-solving techniques, it’s crucial to understand the basic bitwise operations. These operations work directly on the binary representation of numbers, allowing for efficient manipulation of individual bits.
1. Bitwise AND (&)
The bitwise AND operation compares corresponding bits of two numbers and returns 1 if both bits are 1, otherwise 0.
5 & 3 = 1
(101 & 011 = 001 in binary)
2. Bitwise OR (|)
The bitwise OR operation compares corresponding bits of two numbers and returns 1 if at least one of the bits is 1, otherwise 0.
5 | 3 = 7
(101 | 011 = 111 in binary)
3. Bitwise XOR (^)
The bitwise XOR (exclusive OR) operation compares corresponding bits of two numbers and returns 1 if the bits are different, otherwise 0.
5 ^ 3 = 6
(101 ^ 011 = 110 in binary)
4. Bitwise NOT (~)
The bitwise NOT operation inverts all the bits of a number, changing 0 to 1 and 1 to 0.
~5 = -6
(~00000101 = 11111010 in binary, which is -6 in two's complement)
5. Left Shift (<<)
The left shift operation moves all bits to the left by a specified number of positions, filling the rightmost bits with 0.
5 << 1 = 10
(101 << 1 = 1010 in binary)
6. Right Shift (>>)
The right shift operation moves all bits to the right by a specified number of positions. For unsigned integers, it fills the leftmost bits with 0. For signed integers, it performs sign extension.
5 >> 1 = 2
(101 >> 1 = 010 in binary)
Common Bitwise Manipulation Techniques
Now that we’ve covered the basic operations, let’s explore some common techniques used in bitwise manipulation for problem-solving.
1. Checking if a number is even or odd
We can use the bitwise AND operation to check if a number is even or odd. If the least significant bit is 0, the number is even; if it’s 1, the number is odd.
bool isEven(int n) {
return !(n & 1);
}
bool isOdd(int n) {
return n & 1;
}
2. Multiplying or dividing by powers of 2
Left shift can be used to multiply a number by powers of 2, while right shift can be used to divide by powers of 2.
int multiplyByPowerOf2(int n, int power) {
return n << power;
}
int divideByPowerOf2(int n, int power) {
return n >> power;
}
3. Setting, clearing, and toggling bits
These operations are fundamental in bitwise manipulation and are often used in more complex algorithms.
int setBit(int n, int position) {
return n | (1 << position);
}
int clearBit(int n, int position) {
return n & ~(1 << position);
}
int toggleBit(int n, int position) {
return n ^ (1 << position);
}
4. Checking if a bit is set
We can check if a specific bit is set (1) or not (0) using the bitwise AND operation.
bool isBitSet(int n, int position) {
return (n & (1 << position)) != 0;
}
5. Finding the rightmost set bit
This technique is useful in various algorithms, including those dealing with binary trees.
int rightmostSetBit(int n) {
return n & -n;
}
Solving Problems with Bitwise Manipulation
Now that we’ve covered the basics and some common techniques, let’s explore how to apply bitwise manipulation to solve real-world problems. We’ll start with simpler problems and gradually move to more complex ones.
Problem 1: Find the single number
Given an array where every element appears twice except for one, find that single element.
int findSingleNumber(vector<int>& nums) {
int result = 0;
for (int num : nums) {
result ^= num;
}
return result;
}
This solution uses the XOR operation’s property that XOR of a number with itself is 0, and XOR of a number with 0 is the number itself. By XORing all numbers in the array, the duplicates cancel out, leaving only the single number.
Problem 2: Count the number of set bits
Count the number of 1s in the binary representation of a given integer.
int countSetBits(int n) {
int count = 0;
while (n) {
count += n & 1;
n >>= 1;
}
return count;
}
This method, known as Brian Kernighan’s algorithm, is more efficient than checking each bit:
int countSetBits(int n) {
int count = 0;
while (n) {
n &= (n - 1);
count++;
}
return count;
}
Problem 3: Swap two numbers without using a temporary variable
This classic interview question can be solved elegantly using XOR.
void swapNumbers(int& a, int& b) {
a ^= b;
b ^= a;
a ^= b;
}
Problem 4: Find the missing number
Given an array containing n distinct numbers taken from 0 to n, find the missing number.
int findMissingNumber(vector<int>& nums) {
int n = nums.size();
int result = n;
for (int i = 0; i < n; i++) {
result ^= i ^ nums[i];
}
return result;
}
This solution uses the property of XOR to cancel out all numbers that appear in both the array and the range 0 to n, leaving only the missing number.
Problem 5: Reverse bits of an integer
Reverse the bits of a given 32-bit unsigned integer.
uint32_t reverseBits(uint32_t n) {
uint32_t result = 0;
for (int i = 0; i < 32; i++) {
result = (result << 1) | (n & 1);
n >>= 1;
}
return result;
}
Problem 6: Generate all possible subsets of a set
Given a set of distinct integers, generate all possible subsets (the power set).
vector<vector<int>> subsets(vector<int>& nums) {
int n = nums.size();
int subsetCount = 1 << n;
vector<vector<int>> result;
for (int i = 0; i < subsetCount; i++) {
vector<int> subset;
for (int j = 0; j < n; j++) {
if (i & (1 << j)) {
subset.push_back(nums[j]);
}
}
result.push_back(subset);
}
return result;
}
This solution uses the fact that for a set of n elements, there are 2^n possible subsets. We represent each subset as a binary number where 1 indicates the presence of an element and 0 indicates its absence.
Advanced Bitwise Manipulation Techniques
As you become more comfortable with basic bitwise operations, you can explore more advanced techniques that are often used in competitive programming and technical interviews.
1. Isolating the rightmost 0 bit
This technique is useful in various bit manipulation problems.
int isolateRightmostZeroBit(int n) {
return ~n & (n + 1);
}
2. Turning off the rightmost 1 bit
This operation is the basis of Brian Kernighan’s algorithm for counting set bits.
int turnOffRightmost1Bit(int n) {
return n & (n - 1);
}
3. Extracting the lowest set bit
This technique is often used in problems involving binary trees and other binary structures.
int extractLowestSetBit(int n) {
return n & (-n);
}
4. Checking if a number is a power of 2
This is a common interview question that can be solved efficiently using bitwise operations.
bool isPowerOfTwo(int n) {
return n > 0 && !(n & (n - 1));
}
Real-world Applications of Bitwise Manipulation
Bitwise manipulation isn’t just for coding interviews; it has numerous practical applications in real-world software development:
1. Flags and Options
Bitwise operations are often used to efficiently store and manipulate flags or options. For example, file permissions in Unix-like systems use bitwise flags.
const int READ = 4; // 100 in binary
const int WRITE = 2; // 010 in binary
const int EXECUTE = 1; // 001 in binary
int permissions = READ | WRITE; // 110 in binary
if (permissions & EXECUTE) {
cout << "File is executable" << endl;
}
2. Color Manipulation
In computer graphics, colors are often represented as 32-bit integers. Bitwise operations can be used to efficiently extract or modify color components.
int color = 0xFF336699; // ARGB format
int alpha = (color >> 24) & 0xFF;
int red = (color >> 16) & 0xFF;
int green = (color >> 8) & 0xFF;
int blue = color & 0xFF;
3. Network Protocols
Bitwise operations are extensively used in network programming for tasks like IP address manipulation and packet header parsing.
uint32_t ipToInt(const string& ip) {
uint32_t result = 0;
istringstream iss(ip);
string octet;
int shift = 24;
while (getline(iss, octet, '.')) {
result |= (stoi(octet) << shift);
shift -= 8;
}
return result;
}
4. Cryptography
Many cryptographic algorithms rely heavily on bitwise operations for efficiency and security.
5. Data Compression
Bitwise operations are fundamental in many data compression algorithms, allowing for efficient storage and transmission of data.
Tips for Mastering Bitwise Manipulation
- Practice Regularly: Like any skill, proficiency in bitwise manipulation comes with practice. Solve problems on platforms like LeetCode, HackerRank, or CodeForces that specifically focus on bit manipulation.
- Visualize Binary Representations: When working with bitwise operations, it’s helpful to visualize the binary representation of numbers. Use tools or write simple functions to convert between decimal and binary.
- Understand the Underlying Principles: Don’t just memorize tricks; understand why they work. This will help you apply these techniques to novel problems.
- Learn Common Bit Manipulation Idioms: Familiarize yourself with common bit manipulation patterns and idioms used in programming.
- Study Real-world Applications: Look at how bitwise operations are used in real software projects, especially in areas like systems programming, game development, and embedded systems.
- Be Mindful of Performance: While bitwise operations are generally fast, be aware of potential performance implications, especially when working with large datasets or in performance-critical code.
Conclusion
Mastering bitwise manipulation is a valuable skill for any programmer, especially those aiming for positions at top tech companies. It not only helps in solving specific types of problems efficiently but also deepens your understanding of how computers work at a fundamental level.
As you continue to practice and apply these techniques, you’ll find that many problems that seem complex at first glance can be elegantly solved using bitwise operations. Remember, the key to mastery is consistent practice and a deep understanding of the underlying principles.
Whether you’re preparing for technical interviews, working on performance-critical systems, or simply looking to expand your programming toolkit, the skills you’ve learned in this article will serve you well. Keep exploring, keep practicing, and don’t be afraid to tackle complex problems using these powerful bitwise manipulation techniques.