How to Set Up Git Hooks to Automate Tasks: A Comprehensive Guide
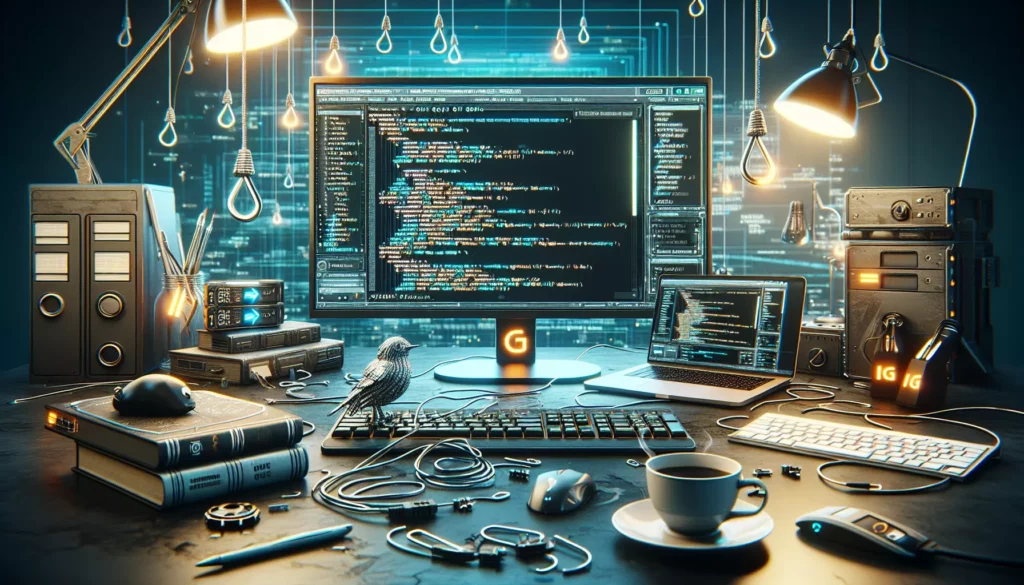
In the world of software development, efficiency and consistency are key. As projects grow in complexity, managing code quality, running tests, and maintaining a smooth workflow becomes increasingly challenging. This is where Git hooks come to the rescue. In this comprehensive guide, we’ll explore how to set up Git hooks to automate various tasks, streamline your development process, and ensure code quality across your team.
Table of Contents
- What Are Git Hooks?
- Types of Git Hooks
- Setting Up Git Hooks
- Common Use Cases for Git Hooks
- Best Practices for Using Git Hooks
- Advanced Techniques with Git Hooks
- Troubleshooting Git Hooks
- Conclusion
1. What Are Git Hooks?
Git hooks are scripts that Git executes before or after events such as: commit, push, and receive. They let you customize Git’s internal behavior and trigger customizable actions at key points in the development lifecycle. Essentially, Git hooks allow you to automate tasks, enforce policies, and streamline your workflow.
These hooks can be written in any scripting language that your system can execute, such as Bash, Python, Ruby, or Perl. This flexibility allows developers to create powerful and tailored automation scripts that integrate seamlessly with their Git workflow.
2. Types of Git Hooks
Git hooks can be categorized into two main types: client-side and server-side hooks. Let’s explore each type and some common examples:
Client-Side Hooks
- pre-commit: Runs before a commit is created. Useful for checking the snapshot that’s about to be committed.
- prepare-commit-msg: Runs before the commit message editor is fired up but after the default message is created.
- commit-msg: Used to validate project or commit message conventions.
- post-commit: Runs after the entire commit process is completed.
- pre-push: Runs during git push, after the remote refs have been updated but before any objects have been transferred.
Server-Side Hooks
- pre-receive: Runs when the server receives a push before any refs are updated.
- update: Similar to pre-receive, but runs once for each branch being pushed to.
- post-receive: Runs after the entire process is completed and can be used for notifications or triggering a deployment process.
3. Setting Up Git Hooks
Now that we understand what Git hooks are and their types, let’s dive into setting them up:
Step 1: Locate the Hooks Directory
Git hooks are stored in the .git/hooks
directory of your Git repository. Navigate to this directory:
cd /path/to/your/repo/.git/hooks
Step 2: Create a New Hook
To create a new hook, simply create a new file in the hooks directory with the name of the hook you want to implement. For example, to create a pre-commit hook:
touch pre-commit
Step 3: Make the Hook Executable
Ensure that your new hook file is executable:
chmod +x pre-commit
Step 4: Edit the Hook
Open the hook file in your preferred text editor and add your script. Here’s a simple example of a pre-commit hook that checks for debugging statements:
#!/bin/sh
if git diff --cached | grep -q 'debugger'
then
echo "Error: Debugging statement detected in commit."
exit 1
fi
This script checks if any lines containing ‘debugger’ are being committed and prevents the commit if found.
Step 5: Test Your Hook
Try to make a commit that would trigger your hook. If set up correctly, the hook should execute and either allow or prevent the action based on your script’s logic.
4. Common Use Cases for Git Hooks
Git hooks can be used for a wide variety of tasks. Here are some common use cases:
1. Code Linting
Use a pre-commit hook to run a linter on your code before allowing a commit. This ensures that all committed code adheres to your project’s style guidelines.
#!/bin/sh
files=$(git diff --cached --name-only --diff-filter=ACM "*.js" "*.jsx")
if [ -n "$files" ]; then
eslint $files
if [ $? -ne 0 ]; then
echo "ESLint failed, commit aborted"
exit 1
fi
fi
2. Running Tests
Use a pre-push hook to run your test suite before pushing to a remote repository:
#!/bin/sh
npm test
if [ $? -ne 0 ]; then
echo "Tests failed, push aborted"
exit 1
fi
3. Enforcing Commit Message Conventions
Use a commit-msg hook to enforce a specific commit message format:
#!/bin/sh
commit_msg=$(cat "$1")
pattern="^(feat|fix|docs|style|refactor|test|chore)(\(.+\))?: .{1,50}$"
if ! echo "$commit_msg" | grep -iqE "$pattern"; then
echo "Commit message format is incorrect. It should be:"
echo "<type>(<scope>): <subject>"
echo "Example: feat(login): add remember me checkbox"
exit 1
fi
4. Preventing Commits to Specific Branches
Use a pre-commit hook to prevent direct commits to protected branches:
#!/bin/sh
branch="$(git rev-parse --abbrev-ref HEAD)"
if [ "$branch" = "main" ]; then
echo "You can't commit directly to main branch"
exit 1
fi
5. Best Practices for Using Git Hooks
While Git hooks are powerful, it’s important to use them wisely. Here are some best practices to keep in mind:
1. Keep Hooks Simple and Fast
Hooks should execute quickly to avoid disrupting the developer’s workflow. If a hook needs to perform a time-consuming task, consider running it asynchronously or moving it to a pre-push or post-commit hook instead of a pre-commit hook.
2. Make Hooks Configurable
Allow developers to bypass hooks when necessary, especially for hooks that might prevent commits or pushes. You can do this by checking for an environment variable:
#!/bin/sh
if [ "$SKIP_TESTS" = "true" ]; then
echo "Skipping tests..."
exit 0
fi
npm test
3. Version Control Your Hooks
Store your hooks in version control to ensure all team members are using the same hooks. You can do this by storing them in a separate directory and symlinking them to the .git/hooks directory.
4. Document Your Hooks
Provide clear documentation for your hooks, including what they do, why they’re necessary, and how to bypass them if needed.
5. Use a Hook Management Tool
Consider using a tool like Husky or pre-commit to manage your Git hooks. These tools can make it easier to share and maintain hooks across your team.
6. Advanced Techniques with Git Hooks
Once you’re comfortable with basic Git hooks, you can explore more advanced techniques:
1. Language-Specific Hooks
While shell scripts are common for Git hooks, you can use any scripting language. Here’s an example of a Python pre-commit hook that checks for PEP 8 compliance:
#!/usr/bin/env python
import subprocess
import sys
def main():
files = subprocess.check_output(['git', 'diff', '--cached', '--name-only', '--diff-filter=ACM', '*.py']).decode('utf-8').split('\n')
files = [f for f in files if f]
if not files:
return 0
result = subprocess.run(['flake8'] + files, capture_output=True, text=True)
if result.returncode != 0:
print(result.stdout)
print(result.stderr)
print("PEP 8 style violations detected. Commit aborted.")
return 1
return 0
if __name__ == "__main__":
sys.exit(main())
2. Multi-Repository Hooks
For projects spanning multiple repositories, you can create hooks that operate across repositories. This could involve checking for consistency in versioning, documentation, or dependencies.
3. Integration with CI/CD
Use post-receive hooks on your Git server to trigger CI/CD pipelines. This can automate the process of building, testing, and deploying your application whenever changes are pushed.
4. Custom Git Commands
You can use Git hooks to create custom Git commands. For example, you could create a git publish command that runs tests, updates the version number, and pushes to a specific branch.
7. Troubleshooting Git Hooks
If you’re having issues with your Git hooks, here are some troubleshooting steps:
1. Check Hook Permissions
Ensure your hook files are executable. You can check this with:
ls -l .git/hooks
If a hook isn’t executable, make it so with:
chmod +x .git/hooks/hook-name
2. Verify Hook Path
Make sure your hooks are in the correct location (.git/hooks in your repository).
3. Debug Your Scripts
Add echo statements to your hook scripts to help identify where issues might be occurring. For example:
#!/bin/sh
echo "Starting pre-commit hook"
# Your hook logic here
echo "Pre-commit hook completed successfully"
4. Check for Syntax Errors
Run your hook scripts manually to check for any syntax errors or runtime issues.
5. Verify Git Configuration
Check your Git configuration to ensure hooks are enabled:
git config --get core.hooksPath
If this returns a value, it means Git is looking for hooks in a custom location. Remove this configuration if you want to use the default .git/hooks directory:
git config --unset core.hooksPath
8. Conclusion
Git hooks are a powerful tool for automating tasks and enforcing standards in your development workflow. By setting up appropriate hooks, you can catch errors early, maintain code quality, and streamline your development process. Remember to keep your hooks simple, fast, and well-documented to ensure they enhance rather than hinder your team’s productivity.
As you become more comfortable with Git hooks, you’ll find countless ways to customize and optimize your development workflow. Whether you’re enforcing coding standards, running tests, or triggering deployments, Git hooks provide a flexible and powerful way to automate your development tasks.
Remember, the key to successful use of Git hooks is finding the right balance between automation and flexibility. Use them to enforce important standards and catch common mistakes, but be careful not to create overly restrictive processes that might frustrate your team or slow down development.
Happy coding, and may your Git hooks serve you well in your journey towards more efficient and higher-quality software development!