How to Set Up a Git Repository from Scratch: A Comprehensive Guide
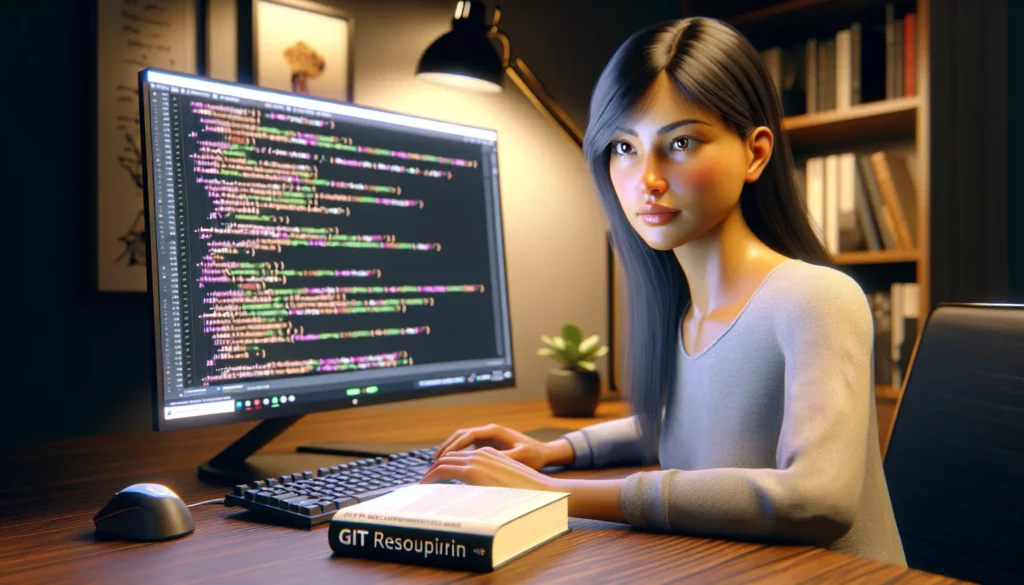
In the world of software development, version control is an essential skill that every programmer should master. Git, the most widely used version control system, has become an industry standard. Whether you’re a beginner just starting your coding journey or an experienced developer looking to refresh your knowledge, understanding how to set up a Git repository from scratch is a crucial skill. In this comprehensive guide, we’ll walk you through the process step-by-step, ensuring you have a solid foundation for managing your projects effectively.
Table of Contents
- What is Git?
- Installing Git
- Configuring Git
- Creating a Local Git Repository
- Basic Git Commands
- Setting Up a Remote Repository
- Connecting Local and Remote Repositories
- Best Practices for Git Repositories
- Troubleshooting Common Issues
- Advanced Git Topics
- Conclusion
1. What is Git?
Before we dive into the setup process, let’s briefly discuss what Git is and why it’s so important in modern software development.
Git is a distributed version control system that allows developers to track changes in their code over time. It enables collaboration among team members, provides a history of all modifications, and allows for easy branching and merging of different versions of a project. Some key benefits of using Git include:
- Version tracking: Keep a complete history of your project
- Collaboration: Work seamlessly with other developers
- Branching and merging: Experiment with new features without affecting the main codebase
- Backup: Store your code securely on remote servers
- Open-source contribution: Easily contribute to open-source projects
2. Installing Git
Before you can set up a Git repository, you need to install Git on your system. The installation process varies depending on your operating system.
For Windows:
- Visit the official Git website: https://git-scm.com/download/win
- Download the installer for your system architecture (32-bit or 64-bit)
- Run the installer and follow the prompts, using the default settings unless you have specific preferences
For macOS:
- If you have Homebrew installed, open Terminal and run:
brew install git
- Alternatively, you can download the installer from https://git-scm.com/download/mac
For Linux:
Most Linux distributions come with Git pre-installed. If not, you can install it using your package manager. For example, on Ubuntu or Debian:
sudo apt-get update
sudo apt-get install git
After installation, verify that Git is correctly installed by opening a terminal or command prompt and running:
git --version
This should display the installed Git version.
3. Configuring Git
Before you start using Git, it’s important to configure your identity. This information will be associated with your commits.
- Set your name:
git config --global user.name "Your Name"
- Set your email address:
git config --global user.email "your.email@example.com"
You can verify your configuration by running:
git config --list
4. Creating a Local Git Repository
Now that Git is installed and configured, let’s create a local repository from scratch.
- Create a new directory for your project:
mkdir my_project cd my_project
- Initialize the Git repository:
git init
This command creates a new subdirectory named .git that contains all of your necessary repository files.
- Create some files in your project directory:
touch README.md echo "# My Project" > README.md
- Add the files to the staging area:
git add .
The period (.) means add all files in the current directory.
- Commit the changes:
git commit -m "Initial commit"
Congratulations! You’ve just created your first Git repository and made your first commit.
5. Basic Git Commands
Let’s review some basic Git commands that you’ll use frequently:
git status
: Check the status of your working directorygit add <file>
: Add a file to the staging areagit commit -m "Message"
: Commit changes with a descriptive messagegit log
: View commit historygit branch
: List, create, or delete branchesgit checkout <branch>
: Switch to a different branchgit merge <branch>
: Merge changes from one branch into the current branch
6. Setting Up a Remote Repository
While local repositories are useful, collaborating with others often requires a remote repository. GitHub, GitLab, and Bitbucket are popular platforms for hosting remote Git repositories. Let’s use GitHub as an example:
- Create a GitHub account if you don’t have one: https://github.com/join
- Click the “+” icon in the top-right corner and select “New repository”
- Give your repository a name, choose public or private, and click “Create repository”
- GitHub will provide instructions for pushing an existing repository. We’ll use these in the next section.
7. Connecting Local and Remote Repositories
Now that you have both a local and remote repository, let’s connect them:
- Add the remote repository URL to your local Git config:
git remote add origin https://github.com/yourusername/your-repo-name.git
- Verify the new remote:
git remote -v
- Push your local repository to the remote:
git push -u origin main
Note: If you’re using an older version of Git, you might need to use “master” instead of “main”.
Your local repository is now connected to the remote repository on GitHub, and your code is backed up online.
8. Best Practices for Git Repositories
To make the most of Git, consider these best practices:
- Commit often: Make small, frequent commits rather than large, infrequent ones
- Write clear commit messages: Use descriptive, concise messages that explain what changed and why
- Use branches: Create separate branches for new features or bug fixes
- Pull before you push: Always pull the latest changes from the remote repository before pushing your own
- Use .gitignore: Create a .gitignore file to exclude unnecessary files from version control
- Review your changes: Use
git diff
to review changes before committing - Keep your repository clean: Regularly delete merged branches and use
git clean
to remove untracked files
9. Troubleshooting Common Issues
Even experienced developers encounter Git issues from time to time. Here are some common problems and their solutions:
Merge Conflicts
When Git can’t automatically merge changes, you’ll encounter a merge conflict. To resolve it:
- Open the conflicting file(s) and look for the conflict markers (<<<<<<<, =======, >>>>>>>)
- Manually edit the file to resolve the conflict
- Remove the conflict markers
- Add the resolved file(s) and commit the changes
Accidentally Committing to the Wrong Branch
If you’ve committed changes to the wrong branch, you can move them to the correct branch:
- Create a new branch at the current commit:
git branch newbranch
- Reset the current branch to the previous commit:
git reset HEAD~ --hard
- Checkout the new branch:
git checkout newbranch
Undoing the Last Commit
If you need to undo the last commit but keep the changes:
git reset --soft HEAD~1
Removing a File from Git but Keeping it Locally
git rm --cached filename
10. Advanced Git Topics
As you become more comfortable with Git, you may want to explore these advanced topics:
Rebasing
Rebasing is an alternative to merging that can create a cleaner project history. To rebase your feature branch onto the main branch:
git checkout feature-branch
git rebase main
Interactive Rebasing
Interactive rebasing allows you to modify commits in various ways:
git rebase -i HEAD~3
This opens an interactive prompt where you can reorder, edit, squash, or drop commits.
Git Hooks
Git hooks are scripts that Git executes before or after events such as commit, push, and receive. They’re useful for enforcing coding standards or running tests automatically.
Submodules
Submodules allow you to keep a Git repository as a subdirectory of another Git repository. This is useful for including external dependencies in your project.
Git Large File Storage (LFS)
Git LFS is an extension that replaces large files with text pointers inside Git, while storing the file contents on a remote server. This can significantly improve performance for repositories with large files.
11. Conclusion
Setting up a Git repository from scratch is a fundamental skill for any developer. By following this guide, you’ve learned how to install Git, create local and remote repositories, connect them, and use basic Git commands. You’ve also gained insights into best practices, troubleshooting common issues, and advanced Git topics.
Remember, mastering Git takes practice. Don’t be afraid to experiment with different commands and workflows in a test repository. As you continue to use Git in your projects, you’ll become more comfortable with its features and discover how it can enhance your development process.
Git’s power lies not just in its ability to track changes, but in how it facilitates collaboration and maintains a clear history of your project’s evolution. Whether you’re working on personal projects or contributing to large open-source initiatives, the skills you’ve learned here will serve you well throughout your programming career.
Keep exploring, keep learning, and happy coding!