How to Set Up a Development Environment for Programming: A Comprehensive Guide
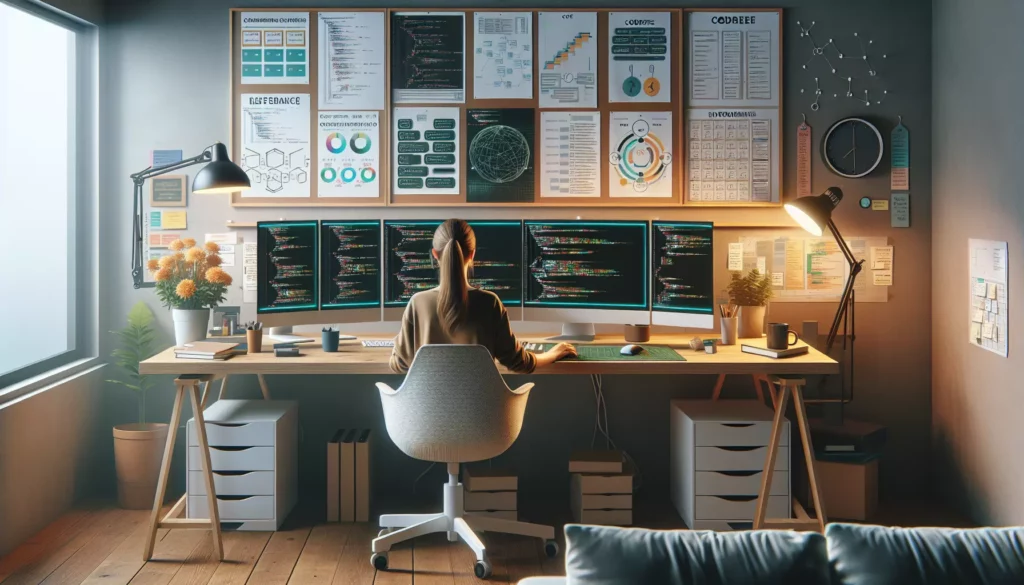
Setting up a proper development environment is a crucial step for any programmer, whether you’re a beginner just starting your coding journey or an experienced developer looking to optimize your workflow. A well-configured development environment can significantly boost your productivity, streamline your coding process, and help you write better, more efficient code. In this comprehensive guide, we’ll walk you through the essential steps to create a robust development environment for programming, tailored to various skill levels and programming languages.
Table of Contents
- Understanding Development Environments
- Choosing the Right Operating System
- Essential Tools for Your Development Environment
- Selecting the Right IDE or Text Editor
- Setting Up Version Control
- Package Managers and Dependency Management
- Working with Virtual Environments
- Mastering the Command Line
- Debugging Tools and Techniques
- Productivity Tools and Extensions
- Cloud-based Development Environments
- Language-Specific Considerations
- Best Practices for Maintaining Your Development Environment
- Troubleshooting Common Issues
- Conclusion
1. Understanding Development Environments
A development environment is a set of tools, software, and configurations that developers use to write, test, and debug code. It typically includes:
- An operating system
- A text editor or Integrated Development Environment (IDE)
- Compilers or interpreters for your programming language(s)
- Version control systems
- Debugging tools
- Package managers
- Command-line interfaces
The goal is to create a comfortable and efficient workspace that allows you to focus on writing code and solving problems without getting bogged down by technical issues or inefficient workflows.
2. Choosing the Right Operating System
Your choice of operating system can significantly impact your development experience. Here are some popular options:
Windows
Pros:
- Widely used and familiar to many users
- Excellent support for .NET development
- Windows Subsystem for Linux (WSL) allows for Linux development on Windows
Cons:
- Some development tools may have limited support compared to Unix-based systems
- Command-line interface (PowerShell) is different from Unix-like systems
macOS
Pros:
- Unix-based, providing a powerful command-line interface
- Popular among developers, especially for web and mobile development
- Excellent support for most programming languages and tools
Cons:
- Limited hardware options and potentially higher cost
- Some enterprise software may have limited support
Linux
Pros:
- Open-source and highly customizable
- Excellent for server-side development and system administration
- Wide range of development tools available through package managers
Cons:
Ultimately, the choice of operating system depends on your specific needs, the type of development you’ll be doing, and your personal preferences. Many developers use multiple operating systems or virtual machines to work across different environments.
3. Essential Tools for Your Development Environment
Regardless of your chosen operating system, there are several essential tools that every developer should have in their environment:
Version Control System
Git is the most widely used version control system. It allows you to track changes in your code, collaborate with others, and manage different versions of your projects. Install Git and create a GitHub or GitLab account to get started.
Package Manager
Package managers help you install, update, and manage software dependencies. Some popular package managers include:
- npm or Yarn for JavaScript
- pip for Python
- Maven or Gradle for Java
- Homebrew for macOS
- apt or yum for Linux distributions
Command-line Interface
Familiarize yourself with the command-line interface (CLI) for your operating system:
- Terminal for macOS and Linux
- Command Prompt or PowerShell for Windows
Compiler or Interpreter
Install the necessary compiler or interpreter for your programming language(s) of choice. For example:
- GCC or Clang for C/C++
- Python interpreter for Python
- Node.js for JavaScript
- JDK for Java
4. Selecting the Right IDE or Text Editor
Choosing the right Integrated Development Environment (IDE) or text editor is crucial for your productivity. Here are some popular options:
IDEs
- Visual Studio: Powerful IDE for Windows development, especially .NET
- IntelliJ IDEA: Excellent for Java development, with versions for other languages
- PyCharm: Specialized IDE for Python development
- Eclipse: Versatile IDE supporting multiple languages
Text Editors
- Visual Studio Code: Lightweight, extensible editor with excellent language support
- Sublime Text: Fast, customizable editor popular among web developers
- Atom: Open-source editor with a large community and plugin ecosystem
- Vim or Emacs: Powerful, keyboard-driven editors with a steeper learning curve
When choosing an IDE or text editor, consider factors such as:
- Language support and available plugins
- Performance and resource usage
- Customization options
- Debugging capabilities
- Integration with other tools (e.g., version control, linters)
5. Setting Up Version Control
Version control is essential for managing your code and collaborating with others. Here’s how to set up Git:
Installing Git
Download and install Git from the official website: https://git-scm.com/downloads
Configuring Git
Set up your Git configuration with your name and email:
git config --global user.name "Your Name"
git config --global user.email "your.email@example.com"
Creating a Repository
To create a new Git repository in your project folder:
cd /path/to/your/project
git init
Basic Git Commands
- Add files to staging:
git add <filename>
orgit add .
for all files - Commit changes:
git commit -m "Your commit message"
- Push changes to a remote repository:
git push origin <branch-name>
- Pull changes from a remote repository:
git pull origin <branch-name>
6. Package Managers and Dependency Management
Package managers help you install, update, and manage external libraries and tools. Here’s how to set up some popular package managers:
npm (Node Package Manager) for JavaScript
Install Node.js, which includes npm: https://nodejs.org/
Initialize a new project:
npm init
Install a package:
npm install <package-name>
pip for Python
pip comes pre-installed with Python 3.4+. To upgrade pip:
python -m pip install --upgrade pip
Install a package:
pip install <package-name>
Maven for Java
Download and install Maven: https://maven.apache.org/download.cgi
Create a new Maven project:
mvn archetype:generate -DgroupId=com.example -DartifactId=my-app -DarchetypeArtifactId=maven-archetype-quickstart -DinteractiveMode=false
7. Working with Virtual Environments
Virtual environments allow you to create isolated spaces for your projects, preventing conflicts between dependencies. Here’s how to set up virtual environments for Python and Node.js:
Python Virtual Environments
Create a new virtual environment:
python -m venv myenv
Activate the virtual environment:
- On Windows:
myenv\Scripts\activate
- On macOS and Linux:
source myenv/bin/activate
Node.js Virtual Environments
Use nvm (Node Version Manager) to manage multiple Node.js versions:
Install nvm: https://github.com/nvm-sh/nvm#installing-and-updating
Install and use a specific Node.js version:
nvm install 14.17.0
nvm use 14.17.0
8. Mastering the Command Line
Becoming proficient with the command line can significantly boost your productivity. Here are some essential commands to get you started:
Navigation and File Management
cd
: Change directoryls
(Unix) ordir
(Windows): List directory contentsmkdir
: Create a new directorytouch
(Unix) orecho.
(Windows): Create a new filecp
(Unix) orcopy
(Windows): Copy files or directoriesmv
(Unix) ormove
(Windows): Move or rename files or directoriesrm
(Unix) ordel
(Windows): Remove files or directories
Text Manipulation
cat
(Unix) ortype
(Windows): Display file contentsgrep
(Unix) orfindstr
(Windows): Search for patterns in filessed
(Unix): Stream editor for filtering and transforming textawk
(Unix): Text processing tool for data extraction and reporting
Process Management
ps
(Unix) ortasklist
(Windows): List running processeskill
(Unix) ortaskkill
(Windows): Terminate processes
9. Debugging Tools and Techniques
Effective debugging is crucial for identifying and fixing issues in your code. Here are some essential debugging tools and techniques:
Integrated Debuggers
Most IDEs come with built-in debuggers that allow you to:
- Set breakpoints
- Step through code line by line
- Inspect variables and their values
- Evaluate expressions
Logging
Implement logging in your code to track the flow of execution and variable values. Use logging libraries like:
- Python:
logging
module - JavaScript:
console.log()
or libraries like Winston - Java: Log4j or SLF4J
Browser Developer Tools
For web development, browser developer tools offer powerful debugging capabilities:
- Console for logging and error messages
- Network tab for monitoring HTTP requests
- Elements tab for inspecting and modifying the DOM
- Sources tab for JavaScript debugging
Command-line Debuggers
- GDB for C/C++
- pdb for Python
- Node.js built-in debugger
10. Productivity Tools and Extensions
Enhance your development environment with productivity tools and extensions:
Code Formatting and Linting
- Prettier: Opinionated code formatter for JavaScript, CSS, and more
- ESLint: Linting tool for JavaScript
- Black: Code formatter for Python
- Checkstyle: Code style checker for Java
Code Snippets and Templates
Use code snippets to quickly insert commonly used code patterns. Most IDEs and text editors support custom snippets.
Task Runners and Build Tools
- Grunt or Gulp for JavaScript
- Make for C/C++
- Gradle or Maven for Java
Continuous Integration/Continuous Deployment (CI/CD) Tools
- Jenkins
- Travis CI
- GitLab CI
- GitHub Actions
11. Cloud-based Development Environments
Cloud-based development environments offer several advantages, including easy setup, collaboration, and access from any device. Some popular options include:
GitHub Codespaces
GitHub Codespaces provides a complete, configurable development environment in the cloud, integrated with GitHub repositories.
Gitpod
Gitpod offers browser-based development environments that can be launched from any GitHub, GitLab, or Bitbucket project.
Repl.it
Repl.it is an online IDE that supports multiple programming languages and provides a collaborative coding environment.
AWS Cloud9
AWS Cloud9 is a cloud-based IDE that allows you to write, run, and debug code from a web browser.
12. Language-Specific Considerations
Different programming languages may require specific tools or configurations. Here are some considerations for popular languages:
Python
- Use virtual environments (venv or conda) to manage dependencies
- Install IPython for an enhanced interactive shell
- Use tools like mypy for static type checking
JavaScript
- Use nvm to manage multiple Node.js versions
- Configure Babel for transpiling modern JavaScript
- Set up Webpack or Parcel for module bundling
Java
- Install the Java Development Kit (JDK)
- Use build tools like Maven or Gradle
- Configure your IDE for automatic imports and code formatting
C/C++
- Install a compiler (GCC or Clang)
- Use CMake for cross-platform build configuration
- Set up a debugger like GDB or LLDB
13. Best Practices for Maintaining Your Development Environment
To keep your development environment running smoothly, follow these best practices:
Regular Updates
- Keep your operating system and software up to date
- Regularly update your IDE, text editor, and extensions
- Update language runtimes and package managers
Backup and Version Control
- Use version control for all your projects
- Regularly push your changes to remote repositories
- Back up your configuration files and important data
Documentation
- Document your development environment setup
- Keep a README file in each project with setup instructions
- Use comments in configuration files to explain non-obvious settings
Automation
- Create scripts to automate repetitive tasks
- Use dotfiles to manage and version your configuration files
- Implement CI/CD pipelines for your projects
14. Troubleshooting Common Issues
Even with a well-configured development environment, you may encounter issues. Here are some common problems and their solutions:
Path-related Issues
If you’re having trouble running commands or executables, check your system’s PATH environment variable. Ensure that the necessary directories are included in the PATH.
Dependency Conflicts
Use virtual environments and package managers to isolate project dependencies. If conflicts persist, try the following:
- Clear package caches and reinstall dependencies
- Use tools like
pip-compile
ornpm shrinkwrap
to lock dependency versions - Review your project’s dependency tree for conflicts
Performance Issues
If your development environment is running slowly:
- Close unnecessary applications and browser tabs
- Disable unused extensions or plugins in your IDE
- Upgrade your hardware (e.g., add more RAM or switch to an SSD)
Version Incompatibilities
When facing version incompatibilities between tools or libraries:
- Check the documentation for compatibility requirements
- Use version managers (e.g., nvm for Node.js, pyenv for Python) to switch between versions
- Consider using containerization (e.g., Docker) to isolate environments
15. Conclusion
Setting up a robust development environment is a crucial step in becoming a productive and efficient programmer. By carefully selecting and configuring your tools, maintaining good practices, and continuously improving your setup, you can create an environment that enhances your coding experience and helps you tackle complex programming challenges with ease.
Remember that your development environment should evolve with your needs and the technologies you work with. Regularly reassess your setup, explore new tools, and don’t be afraid to make changes that improve your workflow. With a well-tuned development environment, you’ll be better equipped to write high-quality code, collaborate effectively with others, and tackle even the most challenging programming tasks.
As you continue your journey in programming and software development, platforms like AlgoCademy can provide valuable resources and guidance to help you improve your coding skills, master algorithms, and prepare for technical interviews. By combining a solid development environment with continuous learning and practice, you’ll be well on your way to becoming a skilled and successful programmer.