How to Resolve Merge Conflicts in Git: A Comprehensive Guide
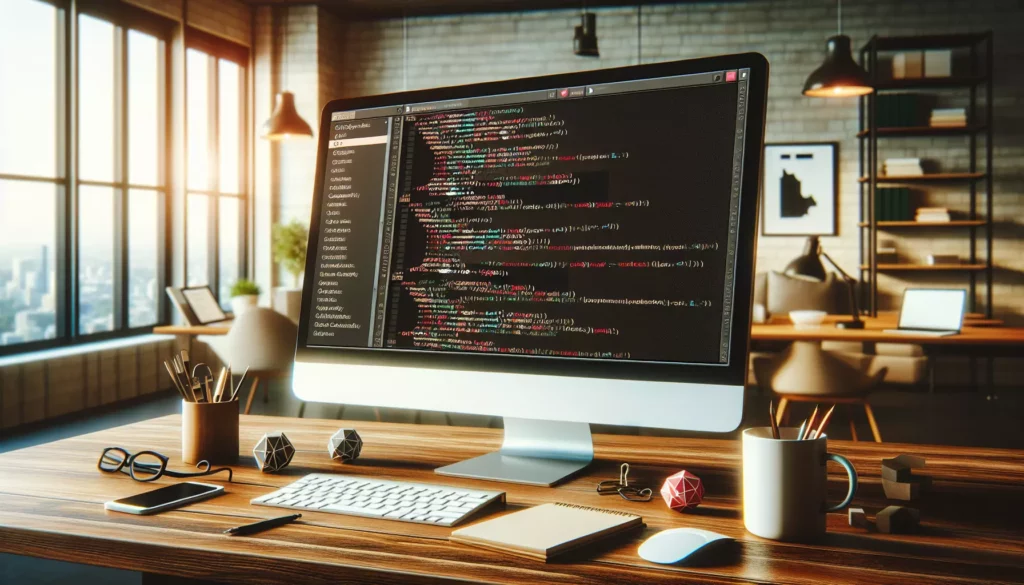
As developers, we often find ourselves collaborating on projects using Git, a powerful version control system. While Git excels at managing different versions of code and merging changes from multiple contributors, there are times when conflicts arise. These merge conflicts can be intimidating, especially for beginners, but they’re a natural part of the collaborative development process. In this comprehensive guide, we’ll walk you through the process of resolving merge conflicts in Git, providing you with the skills and confidence to handle these situations effectively.
Table of Contents
- What Are Merge Conflicts?
- When Do Merge Conflicts Occur?
- Understanding Git Merge
- Identifying Merge Conflicts
- Resolving Merge Conflicts
- Tools for Resolving Conflicts
- Best Practices for Avoiding Merge Conflicts
- Advanced Techniques for Handling Complex Conflicts
- Conclusion
1. What Are Merge Conflicts?
Merge conflicts occur when Git is unable to automatically reconcile differences in code between two commits. This typically happens when two branches have made edits to the same line in a file, or when a file has been deleted in one branch but edited in the other. Essentially, Git needs human intervention to decide which changes should be incorporated in the final merge.
2. When Do Merge Conflicts Occur?
Merge conflicts can arise in several scenarios:
- When merging branches
- During a rebase operation
- When applying a stash
- When pulling changes from a remote repository
Understanding these scenarios can help you anticipate and prepare for potential conflicts.
3. Understanding Git Merge
Before diving into conflict resolution, it’s crucial to understand how Git merge works. When you merge one branch into another, Git looks at the snapshots of both branches and tries to combine them. If changes have been made to the same part of the same file in both branches, Git can’t automatically determine which version to use, resulting in a merge conflict.
Here’s a basic example of a merge operation:
git checkout main
git merge feature-branch
If there are no conflicts, Git will automatically create a new commit that combines the changes from both branches. However, if conflicts exist, Git will pause the merge process and mark the conflicting areas in the affected files.
4. Identifying Merge Conflicts
When a merge conflict occurs, Git will modify the content of the affected files to highlight the conflicting areas. These areas are enclosed in “conflict markers”:
<<<<<<< HEAD
This is the current change
=======
This is the incoming change
>>>>>>> feature-branch
In this example:
- The content between
<<<<<<< HEAD
and=======
shows the changes in the current branch. - The content between
=======
and>>>>>>> feature-branch
shows the changes in the branch being merged.
Git will also provide a summary of the conflicting files when you run git status
:
$ git status
On branch main
You have unmerged paths.
(fix conflicts and run "git commit")
(use "git merge --abort" to abort the merge)
Unmerged paths:
(use "git add <file>..." to mark resolution)
both modified: conflicting_file.txt
no changes added to commit (use "git add" and/or "git commit -a")
5. Resolving Merge Conflicts
Resolving merge conflicts involves the following steps:
- Identify conflicting files: Use
git status
to see which files have conflicts. - Open the conflicting files: Use a text editor or IDE to open the files marked as conflicting.
- Locate the conflict markers: Find the sections enclosed by
<<<<<<<
,=======
, and>>>>>>>
. - Decide on the final content: Choose which changes to keep, or combine them as needed.
- Remove conflict markers: Delete the
<<<<<<<
,=======
, and>>>>>>>
lines. - Stage the resolved files: Use
git add <filename>
to mark the file as resolved. - Commit the changes: Once all conflicts are resolved, commit the changes to complete the merge.
Here’s an example of resolving a conflict:
// Original content in conflicting_file.txt
<<<<<<< HEAD
console.log("Hello, World!");
=======
console.log("Greetings, Universe!");
>>>>>>> feature-branch
// After resolving the conflict
console.log("Hello, Universe!");
After editing the file, stage and commit the changes:
git add conflicting_file.txt
git commit -m "Resolve merge conflict in conflicting_file.txt"
6. Tools for Resolving Conflicts
While conflicts can be resolved manually using a text editor, several tools can make the process easier:
- Git’s built-in mergetool: Run
git mergetool
to launch a visual diff tool. - IDEs with Git integration: Many IDEs like Visual Studio Code, IntelliJ IDEA, and Eclipse offer built-in conflict resolution tools.
- Dedicated merge tools: Applications like Beyond Compare, KDiff3, and P4Merge provide advanced features for comparing and merging files.
To configure a custom merge tool in Git:
git config --global merge.tool <toolname>
git config --global mergetool.<toolname>.path /path/to/tool
7. Best Practices for Avoiding Merge Conflicts
While conflicts are sometimes unavoidable, following these best practices can help minimize their occurrence:
- Communicate with your team: Coordinate who’s working on what to avoid simultaneous changes to the same code.
- Pull changes frequently: Regularly update your local branch with changes from the remote repository.
- Use feature branches: Work on new features in separate branches to isolate changes.
- Keep commits small and focused: Smaller, more frequent commits are easier to merge and understand.
- Use Git hooks: Implement pre-commit hooks to enforce code style and catch potential conflicts early.
- Utilize Git’s features: Leverage Git commands like
git rebase
andgit pull --rebase
to maintain a cleaner history.
8. Advanced Techniques for Handling Complex Conflicts
For more complex merge scenarios, consider these advanced techniques:
Aborting a Merge
If you encounter unexpected conflicts or need to start over, you can abort the merge:
git merge --abort
This command will revert your working directory to its state before the merge attempt.
Using Git Rebase
Rebasing can sometimes provide a cleaner way to integrate changes and resolve conflicts:
git checkout feature-branch
git rebase main
If conflicts occur during rebase, resolve them as you would in a merge, then use:
git rebase --continue
Cherry-Picking
For conflicts involving specific commits, cherry-picking can be useful:
git cherry-pick <commit-hash>
This allows you to apply individual commits from one branch to another, potentially avoiding some conflicts.
Using Git Stash
If you have uncommitted changes that are causing conflicts, you can temporarily stash them:
git stash
git pull
git stash pop
This sequence allows you to update your branch without losing your work-in-progress changes.
Leveraging Git Rerere
Git’s “reuse recorded resolution” (rerere) feature can be helpful for recurring conflicts:
git config --global rerere.enabled true
This setting allows Git to remember how you’ve resolved conflicts in the past and automatically apply those resolutions to similar conflicts in the future.
9. Conclusion
Merge conflicts are an inevitable part of collaborative development, but with the right knowledge and tools, they don’t have to be a source of frustration. By understanding why conflicts occur, how to identify them, and the steps to resolve them effectively, you can navigate these situations with confidence.
Remember, the key to successfully managing merge conflicts lies in clear communication with your team, regular integration of changes, and a solid understanding of Git’s capabilities. As you gain experience, you’ll develop a workflow that minimizes conflicts and allows you to resolve them quickly when they do occur.
Practice these techniques in your projects, and don’t be afraid to experiment with different approaches. With time, handling merge conflicts will become a natural part of your development process, allowing you to focus on what really matters: writing great code and building amazing software.
Happy coding, and may your merges be ever smooth!