How to Recover from Mistakes in a Coding Interview
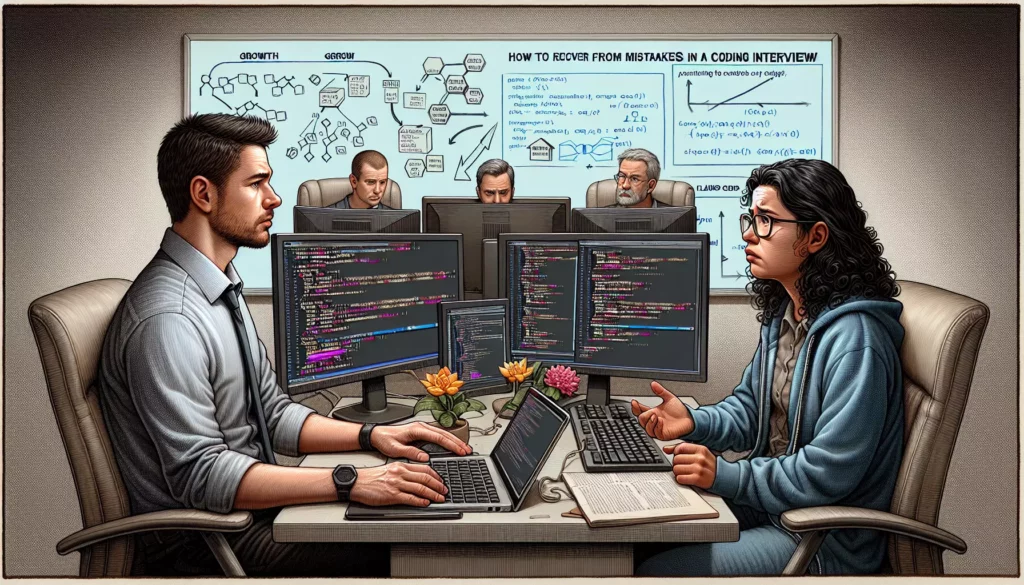
Coding interviews can be nerve-wracking experiences, even for seasoned developers. The pressure of solving complex problems in real-time while being observed by potential employers can lead to mistakes. However, it’s not the mistakes themselves that define your performance, but how you handle and recover from them. In this comprehensive guide, we’ll explore strategies to help you bounce back from errors during a coding interview, turning potential setbacks into opportunities to showcase your problem-solving skills and resilience.
Understanding the Nature of Coding Interviews
Before diving into recovery strategies, it’s crucial to understand the purpose and nature of coding interviews. These interviews are designed to assess not just your coding skills, but also your problem-solving abilities, communication, and how you handle pressure. Interviewers are often more interested in your thought process and approach than in perfect, bug-free code on the first try.
Common Types of Mistakes in Coding Interviews
Mistakes in coding interviews generally fall into several categories:
- Syntax errors
- Logic errors
- Algorithm choice errors
- Time complexity misjudgments
- Misunderstanding the problem statement
- Edge case oversights
Recognizing the type of mistake you’ve made is the first step in recovering from it effectively.
Strategies for Recovering from Mistakes
1. Stay Calm and Composed
The most crucial aspect of recovering from a mistake is maintaining your composure. Remember, it’s normal to make errors, especially under pressure. Take a deep breath and remind yourself that how you handle the situation is just as important as solving the problem itself.
2. Acknowledge the Mistake
Once you realize you’ve made a mistake, acknowledge it openly. This demonstrates honesty and self-awareness, qualities that interviewers value. You might say something like, “I see I’ve made an error here. Let me take a moment to review and correct it.”
3. Analyze the Error
Take a moment to understand what went wrong. Is it a simple syntax error? A logical flaw in your algorithm? Or did you misunderstand a part of the problem statement? Identifying the nature of the mistake will help you address it more effectively.
4. Communicate Your Thought Process
As you work on fixing the mistake, verbalize your thoughts. This gives the interviewer insight into your problem-solving approach and demonstrates your ability to think critically under pressure. For example:
“I realize now that my initial approach didn’t account for negative inputs. I’m going to modify the function to handle this edge case by adding a condition at the beginning.”
5. Implement the Fix
Once you’ve identified the issue, proceed to implement the fix. If it’s a simple syntax error, correct it quickly. For more complex issues, you might need to refactor a portion of your code or rethink your approach entirely.
6. Test Your Solution
After implementing the fix, test your solution with various inputs, including edge cases. This shows thoroughness and attention to detail. Explain your testing process to the interviewer:
“Now that I’ve made the change, let me test it with a few inputs, including the edge cases we discussed earlier.”
7. Reflect on the Lesson Learned
Take a moment to reflect on what you’ve learned from the mistake. This demonstrates growth mindset and the ability to learn from errors. You might say:
“This experience reminds me of the importance of considering all possible input scenarios before implementing a solution. In the future, I’ll make sure to ask clarifying questions about edge cases upfront.”
Specific Scenarios and How to Handle Them
Scenario 1: Syntax Error
Syntax errors are common and usually easy to fix. Here’s how to handle them:
- Acknowledge the error: “Oops, I see I’ve made a syntax error here.”
- Identify the specific issue: “I forgot to close the parenthesis in this function call.”
- Fix it quickly: “Let me add the closing parenthesis here.”
- Explain the correction: “This should resolve the syntax issue and allow the code to compile correctly.”
Scenario 2: Logic Error
Logic errors can be more challenging to spot and fix. Here’s an approach:
- Recognize the problem: “The output isn’t what I expected. There must be a logic error in my code.”
- Analyze the code: “Let me walk through the logic step by step to find where it’s going wrong.”
- Identify the issue: “I see now that my conditional statement is incorrect. It should be checking for greater than or equal to, not just greater than.”
- Explain and fix: “I’m going to change this condition from ‘>’ to ‘>=’ to include the boundary case.”
- Test: “Now let me run through some test cases to ensure this fixes the issue.”
Scenario 3: Algorithm Choice Error
Sometimes, you might realize that your chosen algorithm isn’t optimal. Here’s how to recover:
- Acknowledge the realization: “I’ve just realized that my current approach might not be the most efficient for this problem.”
- Explain your thoughts: “The current algorithm has a time complexity of O(n^2), but I think we can improve this to O(n log n) using a different approach.”
- Propose a new solution: “I’d like to switch to using a heap data structure instead, which should optimize our solution.”
- Ask for permission: “Would it be alright if I implemented this new approach? I believe it will be more efficient.”
- Implement and explain: Walk through the new implementation, explaining your reasoning as you go.
The Importance of Soft Skills in Error Recovery
Communication
Clear communication is crucial when recovering from mistakes. Articulate your thoughts, explain your reasoning, and don’t be afraid to ask for clarification if needed. This demonstrates your ability to work collaboratively and think through problems out loud.
Adaptability
Show that you can adapt to new information and changing circumstances. If your initial approach isn’t working, demonstrate flexibility by considering alternative solutions.
Positive Attitude
Maintain a positive attitude throughout the interview, even when facing challenges. This shows resilience and the ability to perform under pressure – qualities highly valued in the tech industry.
Active Listening
Pay close attention to any hints or suggestions the interviewer might provide. They often offer subtle guidance to help you get back on track.
Preparing for Potential Mistakes
While you can’t predict every mistake you might make in an interview, you can prepare to handle them effectively:
1. Practice Debugging
Regularly practice debugging code, both your own and others’. This will sharpen your ability to quickly identify and fix errors.
2. Mock Interviews
Conduct mock interviews with friends or use online platforms. Simulate the pressure of a real interview to practice staying calm and recovering from mistakes.
3. Review Common Pitfalls
Study common coding mistakes and pitfalls related to data structures, algorithms, and problem-solving approaches. Being aware of these can help you avoid them or recover more quickly if they occur.
4. Strengthen Your Fundamentals
A solid understanding of programming fundamentals will help you recover more easily from mistakes. Regularly review core concepts in your preferred programming language.
Learning from Interview Mistakes
After the interview, regardless of the outcome, take time to reflect on any mistakes you made:
1. Self-Reflection
Analyze what went wrong and why. Was it due to nervousness, lack of preparation, or a genuine gap in your knowledge?
2. Identify Areas for Improvement
Based on your reflection, identify specific areas where you can improve. This might involve deepening your understanding of certain algorithms, practicing more coding problems, or working on your communication skills.
3. Create a Study Plan
Develop a structured plan to address the areas you’ve identified for improvement. This might include dedicating time to solve similar problems, studying specific topics, or practicing your problem-solving verbalization.
4. Seek Feedback
If possible, ask the interviewer or the company for feedback. While not all companies provide detailed feedback, any insights you can gather will be valuable for your future interviews.
Tools and Resources for Interview Preparation
To minimize mistakes and improve your recovery skills, consider using these resources:
1. Online Coding Platforms
Websites like LeetCode, HackerRank, and CodeSignal offer a wide range of coding problems similar to those you might encounter in interviews. Regular practice on these platforms can help you become more comfortable with problem-solving under time constraints.
2. Interactive Coding Environments
Use interactive coding environments that allow you to run and test your code in real-time. This can help you catch and fix errors quickly, simulating the interview experience.
3. Version Control Systems
Familiarize yourself with version control systems like Git. While you won’t use these directly in most coding interviews, understanding how to track and revert changes can improve your overall code management skills.
4. Debugging Tools
Practice using debugging tools in your preferred IDE or coding environment. Being proficient with these tools can help you identify and fix errors more efficiently during an interview.
The Role of Continuous Learning
Remember that making mistakes and learning from them is a crucial part of your growth as a developer. Embrace a mindset of continuous learning:
1. Stay Updated
Keep up with the latest developments in your field. New languages, frameworks, and best practices are constantly emerging.
2. Contribute to Open Source
Contributing to open source projects can expose you to different coding styles and problem-solving approaches, broadening your skills and making you more adaptable in interviews.
3. Attend Coding Workshops and Meetups
Participating in coding workshops and meetups can provide valuable insights and allow you to learn from peers and experienced developers.
4. Read Code
Regularly read and analyze code written by others. This can help you recognize different approaches to problem-solving and improve your ability to spot and fix errors.
Conclusion
Mistakes in coding interviews are not just inevitable; they’re an opportunity to showcase your problem-solving skills, resilience, and ability to perform under pressure. By staying calm, communicating effectively, and approaching errors methodically, you can turn potential setbacks into demonstrations of your capabilities as a developer.
Remember, interviewers are often more interested in your thought process and how you handle challenges than in perfect code. Your ability to recover from mistakes can set you apart as a candidate who can thrive in real-world development scenarios where errors and unexpected issues are part of the daily routine.
As you prepare for your next coding interview, focus not just on solving problems correctly, but on developing the skills to recover gracefully when things don’t go as planned. With practice and the right mindset, you’ll be well-equipped to handle any challenge that comes your way in your coding interviews and your future career as a developer.