How to Read and Understand Legacy Code: A Comprehensive Guide
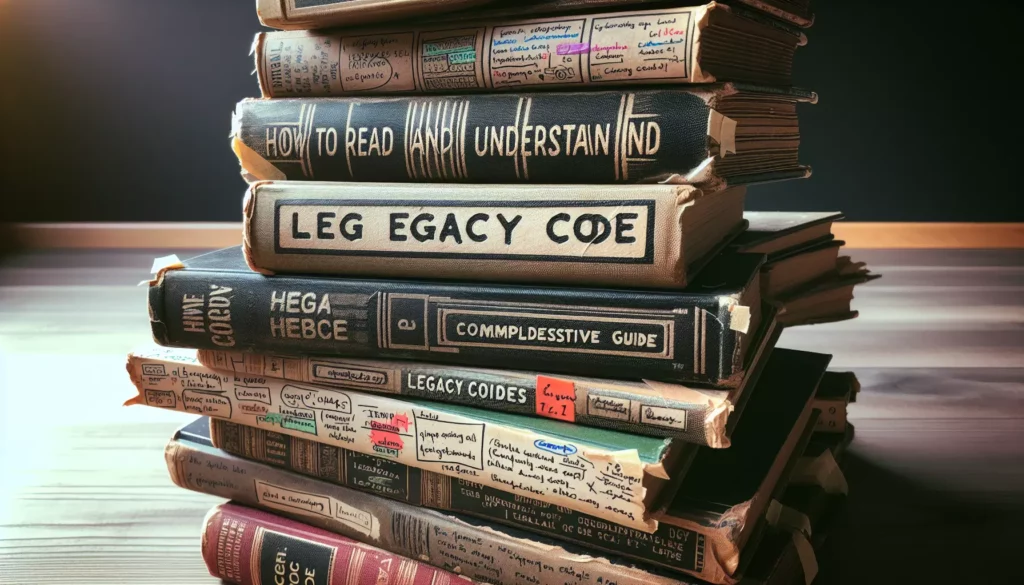
In the world of software development, encountering legacy code is almost inevitable. Whether you’re a seasoned developer or just starting your journey, the ability to read and understand legacy code is a crucial skill. This comprehensive guide will walk you through the process of tackling legacy code, providing you with strategies and techniques to navigate through complex, outdated codebases effectively.
What is Legacy Code?
Before diving into the strategies for reading and understanding legacy code, let’s first define what we mean by “legacy code.” Legacy code typically refers to source code that:
- Is no longer actively maintained
- Uses outdated programming languages or practices
- Lacks proper documentation
- Has been inherited from previous developers or teams
- Is difficult to modify or extend
Legacy code often presents challenges due to its complexity, lack of documentation, and use of outdated technologies. However, it’s essential to approach legacy code with respect, as it often represents years of business logic and problem-solving that have kept an application running.
The Importance of Understanding Legacy Code
Understanding legacy code is crucial for several reasons:
- Maintenance and Updates: Legacy systems often require ongoing maintenance and updates to remain functional and secure.
- Integration: New features or systems may need to be integrated with existing legacy code.
- Refactoring: Improving the code structure without changing its external behavior is often necessary for long-term sustainability.
- Migration: When moving to new technologies or platforms, understanding the existing codebase is crucial for a successful transition.
- Bug Fixing: Identifying and fixing bugs in legacy code requires a deep understanding of how the system works.
Strategies for Reading and Understanding Legacy Code
1. Start with the Big Picture
Before diving into the code details, try to understand the overall structure and purpose of the application:
- Review any available documentation, even if it’s outdated
- Examine the file structure and organization of the codebase
- Identify the main components or modules of the application
- Understand the application’s architecture (e.g., MVC, microservices)
2. Use Version Control History
Version control systems like Git can provide valuable insights:
- Review commit history to understand how the code has evolved
- Look for comments in commit messages that might explain certain decisions
- Identify the most active parts of the codebase
3. Leverage Code Analysis Tools
Static code analysis tools can help you understand the codebase more quickly:
- Use tools like SonarQube or CodeClimate for code quality analysis
- Generate class diagrams or dependency graphs to visualize relationships between components
- Utilize IDE features like “Find Usages” and “Go to Definition” to navigate the code
4. Start with Entry Points
Identify the main entry points of the application:
- For web applications, look for the main controller or router
- In desktop applications, find the main method or application startup code
- For libraries, examine the public API or exposed interfaces
5. Follow the Data Flow
Tracing the flow of data through the application can help you understand its logic:
- Start with user inputs or API endpoints
- Follow how data is processed, transformed, and stored
- Examine database interactions and data persistence logic
6. Use Debugging Techniques
Debugging can be an effective way to understand code behavior:
- Set breakpoints at key points in the code
- Step through the code execution to understand the flow
- Examine variable values and function calls during runtime
7. Write Tests
Writing tests for legacy code can help you understand its behavior and document your findings:
- Start with simple unit tests for individual functions or methods
- Gradually build up to integration tests for larger components
- Use tests to verify your understanding of the code’s behavior
8. Document Your Findings
As you explore the legacy code, document your discoveries:
- Create diagrams to visualize code structure and relationships
- Write comments explaining complex logic or non-obvious decisions
- Update or create README files with high-level overviews
9. Refactor Gradually
As you gain understanding, consider making small improvements:
- Rename variables or functions to be more descriptive
- Extract complex logic into separate functions for better readability
- Apply consistent formatting and coding standards
Common Challenges and How to Overcome Them
Lack of Documentation
Challenge: Legacy code often lacks proper documentation, making it difficult to understand the intended behavior and design decisions.
Solution:
- Create your own documentation as you explore the code
- Use code comments to explain complex sections
- Develop a knowledge base or wiki for the team
Outdated Technologies
Challenge: Legacy code may use outdated libraries, frameworks, or programming languages that are no longer widely used or supported.
Solution:
- Research the older technologies to understand their capabilities and limitations
- Look for modern equivalents or alternatives to help bridge the knowledge gap
- Consider gradual migration strategies for updating the technology stack
Complex and Tangled Code
Challenge: Legacy code often becomes complex and intertwined over time, making it difficult to isolate and understand specific components.
Solution:
- Use code analysis tools to identify dependencies and coupling
- Apply the “Strangler Fig” pattern to gradually replace complex parts
- Refactor small sections at a time to improve readability
Lack of Tests
Challenge: Legacy code often lacks comprehensive test coverage, making it risky to modify and difficult to verify behavior.
Solution:
- Implement characterization tests to document existing behavior
- Gradually increase test coverage as you work on different parts of the code
- Use code coverage tools to identify untested areas
Tools and Resources for Working with Legacy Code
Static Analysis Tools
- SonarQube
- CodeClimate
- ESLint (for JavaScript)
- RuboCop (for Ruby)
Debugging Tools
- Chrome DevTools (for web applications)
- Visual Studio Debugger
- GDB (GNU Debugger)
- PyCharm Debugger (for Python)
Version Control Systems
- Git
- Subversion (SVN)
- Mercurial
Documentation Tools
- Doxygen
- Javadoc
- Sphinx (for Python)
Refactoring Tools
- ReSharper (for .NET)
- IntelliJ IDEA (for Java)
- PyCharm (for Python)
Best Practices for Maintaining Legacy Code
1. Follow the Boy Scout Rule
Always leave the code better than you found it. Make small improvements whenever you work on a piece of legacy code.
2. Use the Strangler Fig Pattern
Gradually replace parts of the legacy system with new implementations, allowing for incremental improvements without a complete rewrite.
3. Maintain a Comprehensive Test Suite
Continuously improve and maintain test coverage to catch regressions and document expected behavior.
4. Keep Dependencies Updated
Regularly update libraries and dependencies to ensure security and compatibility with modern systems.
5. Document Architectural Decisions
Use Architecture Decision Records (ADRs) to document important design decisions and their rationale.
6. Implement Continuous Integration
Set up automated builds and tests to catch issues early and ensure code quality.
7. Plan for Knowledge Transfer
Document key insights and conduct knowledge-sharing sessions to spread understanding across the team.
Case Study: Refactoring a Legacy Payment Processing System
Let’s walk through a hypothetical case study to illustrate the process of understanding and refactoring legacy code.
The Scenario
You’ve joined a company that has a 10-year-old payment processing system written in PHP. The system handles credit card transactions for an e-commerce platform. The code is monolithic, lacks proper documentation, and has minimal test coverage.
Step 1: Understanding the Current System
First, you start by examining the overall structure of the codebase:
payment_system/
├── index.php
├── config.php
├── database.php
├── functions.php
├── process_payment.php
├── validate_card.php
└── generate_report.php
You notice that all the logic is contained in a few large PHP files with no clear separation of concerns.
Step 2: Identifying Core Functionality
After some investigation, you determine that the main functions of the system are:
- Validating credit card information
- Processing payments
- Generating transaction reports
Step 3: Creating a Test Harness
To ensure that you don’t break existing functionality, you start by writing characterization tests. Here’s an example of a simple test for the card validation function:
<?php
use PHPUnit\Framework\TestCase;
class CardValidationTest extends TestCase
{
public function testValidCreditCard()
{
$result = validate_credit_card("1234567890123456", "12", "2025", "123");
$this->assertTrue($result);
}
public function testInvalidCreditCard()
{
$result = validate_credit_card("1234567890123456", "13", "2025", "123");
$this->assertFalse($result);
}
}
Step 4: Refactoring the Code
With tests in place, you start refactoring the code. You decide to apply the principles of object-oriented programming and separate concerns. Here’s an example of how you might refactor the card validation logic:
<?php
class CreditCard
{
private $number;
private $expiryMonth;
private $expiryYear;
private $cvv;
public function __construct($number, $expiryMonth, $expiryYear, $cvv)
{
$this->number = $number;
$this->expiryMonth = $expiryMonth;
$this->expiryYear = $expiryYear;
$this->cvv = $cvv;
}
public function isValid()
{
return $this->validateNumber()
&& $this->validateExpiry()
&& $this->validateCVV();
}
private function validateNumber()
{
// Implementation of credit card number validation
}
private function validateExpiry()
{
// Implementation of expiry date validation
}
private function validateCVV()
{
// Implementation of CVV validation
}
}
Step 5: Implementing New Features
As you refactor, you also implement new features requested by the business, such as support for additional payment methods. You create a new abstract class for payment methods:
<?php
abstract class PaymentMethod
{
abstract public function processPayment($amount);
}
class CreditCardPayment extends PaymentMethod
{
private $creditCard;
public function __construct(CreditCard $creditCard)
{
$this->creditCard = $creditCard;
}
public function processPayment($amount)
{
// Implementation of credit card payment processing
}
}
class PayPalPayment extends PaymentMethod
{
private $email;
public function __construct($email)
{
$this->email = $email;
}
public function processPayment($amount)
{
// Implementation of PayPal payment processing
}
}
Step 6: Continuous Improvement
You continue to refactor and improve the codebase incrementally, always ensuring that existing functionality is maintained through your test suite. You also document your changes and the new architecture to help future developers understand the system.
Conclusion
Reading and understanding legacy code is a valuable skill that takes time and practice to master. By following the strategies outlined in this guide, you can approach legacy codebases with confidence and effectiveness. Remember that working with legacy code is often a gradual process of improvement rather than a complete overhaul.
Key takeaways include:
- Start with the big picture before diving into details
- Use tools and techniques to aid in code comprehension
- Document your findings and create tests to verify behavior
- Refactor gradually while maintaining existing functionality
- Be patient and respectful of the existing codebase
By applying these principles, you’ll be better equipped to handle legacy code challenges and contribute to the long-term success of your software projects. Remember, today’s new code is tomorrow’s legacy code, so always strive to write clean, well-documented, and maintainable code in your current projects.