How to Protect Your Code from Security Vulnerabilities: A Comprehensive Guide
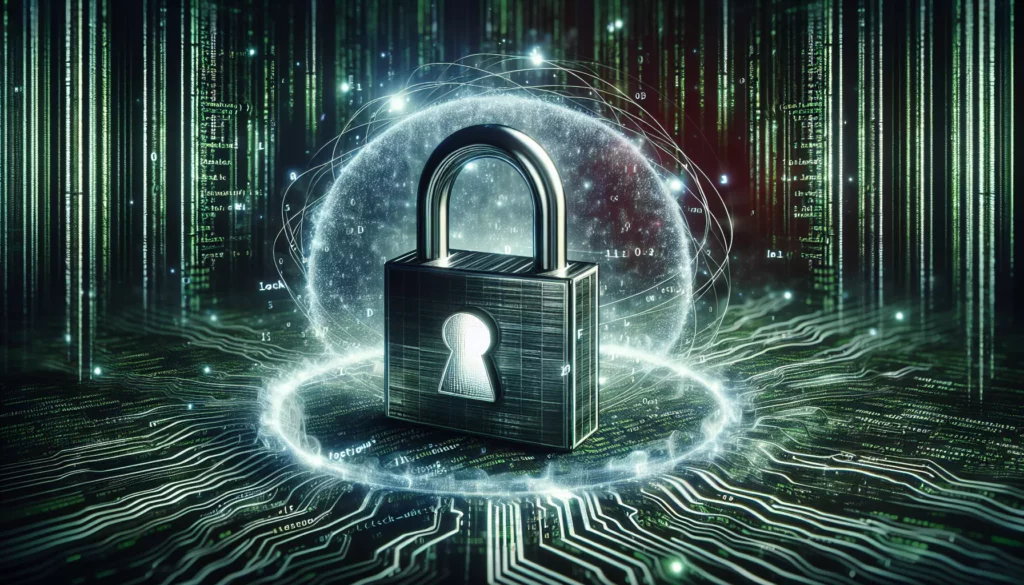
In today’s interconnected digital landscape, security vulnerabilities in software can have far-reaching consequences. As developers, it’s our responsibility to ensure that the code we write is not only functional but also secure. This comprehensive guide will walk you through various strategies and best practices to protect your code from security vulnerabilities, helping you build more robust and resilient applications.
Table of Contents
- Understanding Security Vulnerabilities
- Secure Coding Practices
- Input Validation and Sanitization
- Authentication and Authorization
- Data Encryption
- Managing Third-Party Dependencies
- Secure Error Handling
- Security Testing and Code Review
- Keeping Your Software Updated
- Implementing Security Headers
- Logging and Monitoring
- Security Training and Awareness
- Conclusion
1. Understanding Security Vulnerabilities
Before diving into protection strategies, it’s crucial to understand what security vulnerabilities are and how they can affect your code. A security vulnerability is a weakness in your software that can be exploited by malicious actors to compromise the confidentiality, integrity, or availability of your system.
Common types of security vulnerabilities include:
- Injection flaws (e.g., SQL injection, XSS)
- Broken authentication and session management
- Sensitive data exposure
- XML External Entities (XXE)
- Broken access control
- Security misconfiguration
- Cross-Site Scripting (XSS)
- Insecure deserialization
- Using components with known vulnerabilities
- Insufficient logging and monitoring
Understanding these vulnerabilities is the first step in protecting your code against them.
2. Secure Coding Practices
Adopting secure coding practices is fundamental to creating robust and secure software. Here are some key practices to follow:
2.1 Principle of Least Privilege
Always grant the minimum level of access necessary for a component or user to perform its function. This limits the potential damage if a component is compromised.
2.2 Defense in Depth
Implement multiple layers of security controls. This approach ensures that if one layer fails, others are in place to protect your system.
2.3 Secure by Default
Design your system to be secure out of the box. Default configurations should be the most secure options available.
2.4 Fail Securely
When your system encounters an error, it should fail in a way that doesn’t create security vulnerabilities.
2.5 Input Validation
Never trust user input. Always validate and sanitize all input before processing it.
2.6 Output Encoding
Encode all output to prevent injection attacks, especially when displaying user-supplied data.
3. Input Validation and Sanitization
Input validation is one of the most critical aspects of secure coding. It involves checking all input data to ensure it meets the expected format and falls within acceptable boundaries.
3.1 Server-Side Validation
Always perform input validation on the server side, even if client-side validation is in place. Client-side validation can be bypassed and should not be relied upon for security.
3.2 Whitelist vs. Blacklist
Prefer whitelist validation over blacklist. Whitelist validation specifies exactly what is allowed, while blacklist tries to specify what is not allowed, which can be error-prone.
3.3 Sanitization
In addition to validation, sanitize input by removing or escaping potentially dangerous characters. This is particularly important when dealing with data that will be output to web pages or used in database queries.
3.4 Example of Input Validation in Python
import re
def validate_email(email):
pattern = r'^[\w\.-]+@[\w\.-]+\.\w+$'
return re.match(pattern, email) is not None
# Usage
email = input("Enter your email: ")
if validate_email(email):
print("Valid email")
else:
print("Invalid email")
4. Authentication and Authorization
Proper authentication and authorization mechanisms are crucial for protecting your application from unauthorized access.
4.1 Strong Password Policies
Implement and enforce strong password policies. Require a minimum length, complexity, and consider using multi-factor authentication (MFA) for added security.
4.2 Secure Session Management
Use secure, randomly generated session tokens. Implement proper session timeout and invalidation mechanisms.
4.3 OAuth and OpenID Connect
Consider using standardized protocols like OAuth 2.0 and OpenID Connect for authentication and authorization, especially when dealing with third-party integrations.
4.4 Role-Based Access Control (RBAC)
Implement RBAC to ensure users only have access to the resources and actions necessary for their role.
4.5 Example of Basic Authentication in Python (Flask)
from flask import Flask, request, jsonify
from werkzeug.security import generate_password_hash, check_password_hash
app = Flask(__name__)
# In a real application, you'd store this in a database
users = {
"user@example.com": generate_password_hash("password123")
}
@app.route('/login', methods=['POST'])
def login():
data = request.json
email = data.get('email')
password = data.get('password')
if email not in users or not check_password_hash(users[email], password):
return jsonify({"message": "Invalid credentials"}), 401
return jsonify({"message": "Login successful"}), 200
if __name__ == '__main__':
app.run(debug=True)
5. Data Encryption
Encryption is a critical component of data protection, both in transit and at rest.
5.1 Transport Layer Security (TLS)
Always use HTTPS to encrypt data in transit. This protects against man-in-the-middle attacks and eavesdropping.
5.2 Encryption at Rest
Encrypt sensitive data before storing it in databases or file systems. Use strong, industry-standard encryption algorithms.
5.3 Key Management
Implement proper key management practices. Never hardcode encryption keys in your source code.
5.4 Example of Data Encryption in Python
from cryptography.fernet import Fernet
# Generate a key
key = Fernet.generate_key()
# Create a Fernet instance
f = Fernet(key)
# Encrypt data
message = b"Secret message"
encrypted = f.encrypt(message)
# Decrypt data
decrypted = f.decrypt(encrypted)
print(f"Original: {message}")
print(f"Encrypted: {encrypted}")
print(f"Decrypted: {decrypted}")
6. Managing Third-Party Dependencies
Third-party dependencies can introduce vulnerabilities into your application. It’s crucial to manage them properly.
6.1 Keep Dependencies Updated
Regularly update your dependencies to ensure you have the latest security patches.
6.2 Vulnerability Scanning
Use tools like npm audit, OWASP Dependency-Check, or Snyk to scan your dependencies for known vulnerabilities.
6.3 Minimize Dependencies
Only include dependencies that are absolutely necessary for your project. The fewer dependencies you have, the smaller your attack surface.
6.4 Example of Dependency Scanning in Node.js
// Run this command in your terminal
npm audit
// To fix vulnerabilities automatically (when possible)
npm audit fix
7. Secure Error Handling
Proper error handling is crucial for both security and user experience.
7.1 Don’t Expose Sensitive Information
Ensure that error messages don’t reveal sensitive information about your system or application structure.
7.2 Log Errors Securely
Log errors for debugging purposes, but ensure that logs are stored securely and don’t contain sensitive information.
7.3 Custom Error Pages
Implement custom error pages to prevent default server error messages from being displayed to users.
7.4 Example of Secure Error Handling in Python (Flask)
from flask import Flask, jsonify
app = Flask(__name__)
@app.errorhandler(Exception)
def handle_exception(e):
# Log the error here (e.g., using a logging framework)
# Return a generic error message
return jsonify({"error": "An unexpected error occurred"}), 500
@app.route('/example')
def example():
# Simulate an error
raise Exception("This is a test error")
if __name__ == '__main__':
app.run(debug=False) # Set debug to False in production
8. Security Testing and Code Review
Regular security testing and code reviews are essential for identifying and addressing vulnerabilities.
8.1 Static Application Security Testing (SAST)
Use SAST tools to analyze your source code for potential security vulnerabilities without executing the program.
8.2 Dynamic Application Security Testing (DAST)
Employ DAST tools to test your running application for vulnerabilities by simulating attacks.
8.3 Penetration Testing
Conduct regular penetration testing to identify vulnerabilities that automated tools might miss.
8.4 Code Reviews
Implement a thorough code review process with a focus on security. Use pair programming or pull request reviews to catch security issues early.
8.5 Example of Using a SAST Tool (Bandit for Python)
# Install Bandit
pip install bandit
# Run Bandit on your project
bandit -r /path/to/your/python/project
9. Keeping Your Software Updated
Keeping your software and all its components up-to-date is crucial for security.
9.1 Regular Updates
Regularly update your application’s codebase, dependencies, and the underlying operating system and software stack.
9.2 Security Patches
Promptly apply security patches as soon as they are available.
9.3 Automated Update Checks
Implement automated systems to check for and notify about available updates.
10. Implementing Security Headers
HTTP security headers can significantly improve your application’s security posture.
10.1 Content Security Policy (CSP)
Implement a strong Content Security Policy to prevent XSS attacks and other code injection vulnerabilities.
10.2 X-Frame-Options
Use the X-Frame-Options header to prevent clickjacking attacks.
10.3 Strict-Transport-Security
Implement HSTS to ensure that your application is always accessed over HTTPS.
10.4 Example of Implementing Security Headers in Express.js
const express = require('express');
const helmet = require('helmet');
const app = express();
// Use Helmet!
app.use(helmet());
app.get('/', (req, res) => {
res.send('Hello, secure world!');
});
app.listen(3000);
11. Logging and Monitoring
Proper logging and monitoring are essential for detecting and responding to security incidents.
11.1 Implement Comprehensive Logging
Log all security-relevant events, including authentication attempts, access control decisions, and data modifications.
11.2 Secure Log Storage
Store logs securely and ensure they cannot be tampered with.
11.3 Real-time Monitoring
Implement real-time monitoring and alerting for suspicious activities.
11.4 Example of Logging in Python
import logging
# Configure logging
logging.basicConfig(filename='app.log', level=logging.INFO,
format='%(asctime)s - %(levelname)s - %(message)s')
def login(username, password):
# Perform login logic here
success = True # This should be the result of your actual login logic
if success:
logging.info(f"Successful login attempt for user: {username}")
else:
logging.warning(f"Failed login attempt for user: {username}")
return success
# Usage
login("example_user", "password123")
12. Security Training and Awareness
Cultivating a security-aware development culture is crucial for maintaining code security.
12.1 Regular Training
Provide regular security training for all developers and team members involved in the software development lifecycle.
12.2 Stay Informed
Keep up-to-date with the latest security threats and best practices. Subscribe to security mailing lists and follow reputable security blogs.
12.3 Encourage a Security-First Mindset
Foster a culture where security is considered at every stage of development, not just as an afterthought.
13. Conclusion
Protecting your code from security vulnerabilities is an ongoing process that requires vigilance, knowledge, and consistent effort. By implementing the strategies outlined in this guide, you can significantly reduce the risk of security breaches and build more robust, secure applications.
Remember that security is not a one-time task but a continuous process. Stay informed about new threats and best practices, regularly review and update your security measures, and always prioritize security in your development process.
By making security an integral part of your development workflow, you not only protect your code but also build trust with your users and stakeholders. In today’s digital landscape, where security breaches can have far-reaching consequences, the importance of secure coding practices cannot be overstated.
As you continue your journey in software development, whether you’re a beginner learning the ropes or an experienced developer preparing for technical interviews at major tech companies, always keep security at the forefront of your mind. It’s not just about writing functional code; it’s about writing secure, robust code that can withstand the ever-evolving landscape of cyber threats.
Stay vigilant, keep learning, and happy secure coding!