How to Prepare for Problem-Solving Under Pressure: A Comprehensive Guide
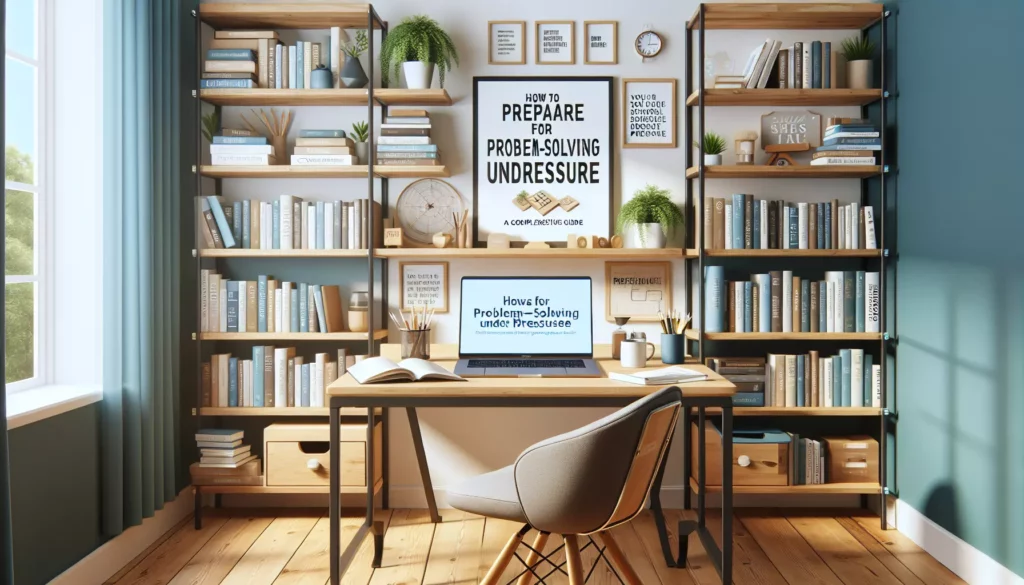
In the world of programming and technical interviews, the ability to solve problems under pressure is a crucial skill. Whether you’re aiming for a position at a FAANG company (Facebook, Amazon, Apple, Netflix, Google) or any other tech giant, being able to think clearly and code effectively when the stakes are high can make all the difference. This comprehensive guide will walk you through strategies and techniques to enhance your problem-solving abilities, particularly when you’re under the gun.
1. Understanding the Importance of Problem-Solving Under Pressure
Before diving into preparation techniques, it’s essential to understand why problem-solving under pressure is so valued in the tech industry:
- Real-world scenarios: In actual work environments, you’ll often face time-sensitive issues that require quick thinking and efficient solutions.
- Assessing adaptability: Employers use pressure situations to gauge how well you can adapt to unexpected challenges.
- Testing fundamental skills: Under pressure, your core programming and algorithmic knowledge are put to the test.
- Evaluating communication: Your ability to articulate your thought process while solving problems is crucial.
2. Building a Strong Foundation
The key to performing well under pressure is having a solid foundation of knowledge and skills. Here’s how to build that foundation:
2.1. Master the Basics
Ensure you have a strong grasp of fundamental programming concepts:
- Data structures (arrays, linked lists, trees, graphs, etc.)
- Algorithms (sorting, searching, dynamic programming, etc.)
- Time and space complexity analysis
- Object-oriented programming principles
2.2. Practice Regularly
Consistent practice is crucial. Set aside time each day to solve coding problems:
- Use platforms like LeetCode, HackerRank, or CodeSignal
- Participate in coding competitions
- Contribute to open-source projects
2.3. Learn Multiple Programming Languages
While it’s important to have a primary language you’re comfortable with, being familiar with multiple languages can be beneficial:
- Python for its simplicity and powerful libraries
- Java for its widespread use in enterprise applications
- JavaScript for web development
- C++ for its performance in competitive programming
3. Developing Problem-Solving Strategies
Having a structured approach to problem-solving can help you stay calm and focused under pressure:
3.1. Understand the Problem
- Read the problem statement carefully
- Identify the input and expected output
- Note any constraints or special conditions
3.2. Plan Your Approach
- Break down the problem into smaller, manageable steps
- Consider multiple solutions and their trade-offs
- Choose the most appropriate data structures and algorithms
3.3. Implement the Solution
- Write clean, readable code
- Use meaningful variable names and add comments where necessary
- Implement error handling and edge cases
3.4. Test and Optimize
- Test your solution with various inputs, including edge cases
- Analyze the time and space complexity
- Optimize your code if necessary
4. Simulating Pressure Situations
To prepare for high-pressure scenarios, it’s important to simulate them in your practice:
4.1. Timed Practice
Set a timer when solving problems to mimic the time constraints of real interviews:
- Start with longer time limits and gradually reduce them
- Practice solving problems within typical interview durations (30-60 minutes)
4.2. Mock Interviews
Participate in mock interviews to get a feel for the real thing:
- Use platforms like Pramp or InterviewBit for peer mock interviews
- Ask friends or mentors to conduct mock interviews for you
- Record yourself solving problems to review your performance later
4.3. Whiteboard Practice
Many technical interviews involve coding on a whiteboard:
- Practice writing code by hand or on a whiteboard
- Focus on clear, legible handwriting
- Learn to manage space effectively on a limited surface
5. Mastering Common Problem Types
Familiarize yourself with frequently asked problem types in technical interviews:
5.1. Array and String Manipulation
These problems often involve techniques like two-pointer, sliding window, or hash tables.
Example problem: Two Sum
def two_sum(nums, target):
num_dict = {}
for i, num in enumerate(nums):
complement = target - num
if complement in num_dict:
return [num_dict[complement], i]
num_dict[num] = i
return []
# Test the function
print(two_sum([2, 7, 11, 15], 9)) # Output: [0, 1]
5.2. Linked Lists
Common operations include reversing, finding cycles, and merging sorted lists.
Example problem: Reverse a Linked List
class ListNode:
def __init__(self, val=0, next=None):
self.val = val
self.next = next
def reverse_list(head):
prev = None
current = head
while current:
next_node = current.next
current.next = prev
prev = current
current = next_node
return prev
# Test the function
# Create a linked list: 1 -> 2 -> 3 -> 4 -> 5
head = ListNode(1)
head.next = ListNode(2)
head.next.next = ListNode(3)
head.next.next.next = ListNode(4)
head.next.next.next.next = ListNode(5)
# Reverse the list
reversed_head = reverse_list(head)
# Print the reversed list
current = reversed_head
while current:
print(current.val, end=" ")
current = current.next
# Output: 5 4 3 2 1
5.3. Trees and Graphs
Focus on traversal algorithms, balancing, and path-finding problems.
Example problem: Binary Tree Level Order Traversal
from collections import deque
class TreeNode:
def __init__(self, val=0, left=None, right=None):
self.val = val
self.left = left
self.right = right
def level_order_traversal(root):
if not root:
return []
result = []
queue = deque([root])
while queue:
level_size = len(queue)
current_level = []
for _ in range(level_size):
node = queue.popleft()
current_level.append(node.val)
if node.left:
queue.append(node.left)
if node.right:
queue.append(node.right)
result.append(current_level)
return result
# Test the function
# Create a binary tree:
# 3
# / \
# 9 20
# / \
# 15 7
root = TreeNode(3)
root.left = TreeNode(9)
root.right = TreeNode(20)
root.right.left = TreeNode(15)
root.right.right = TreeNode(7)
print(level_order_traversal(root))
# Output: [[3], [9, 20], [15, 7]]
5.4. Dynamic Programming
Practice identifying overlapping subproblems and optimal substructure.
Example problem: Fibonacci Sequence
def fibonacci(n):
if n <= 1:
return n
dp = [0] * (n + 1)
dp[1] = 1
for i in range(2, n + 1):
dp[i] = dp[i-1] + dp[i-2]
return dp[n]
# Test the function
print(fibonacci(10)) # Output: 55
6. Improving Your Soft Skills
Technical skills alone aren’t enough. Soft skills play a crucial role in problem-solving under pressure:
6.1. Communication
- Practice explaining your thought process out loud
- Learn to ask clarifying questions
- Develop the ability to discuss trade-offs in your solutions
6.2. Time Management
- Learn to estimate how long different parts of a problem will take
- Prioritize the most critical aspects of the solution
- Know when to move on if you’re stuck
6.3. Stress Management
- Practice deep breathing exercises
- Learn to recognize and manage anxiety symptoms
- Develop a positive mindset towards challenges
7. Leveraging Tools and Resources
Make use of available resources to enhance your problem-solving skills:
7.1. Online Platforms
- AlgoCademy: For interactive coding tutorials and AI-powered assistance
- LeetCode: For a vast collection of coding problems and company-specific question banks
- HackerRank: For practicing coding challenges and participating in contests
7.2. Books
- “Cracking the Coding Interview” by Gayle Laakmann McDowell
- “Introduction to Algorithms” by Thomas H. Cormen, et al.
- “The Algorithm Design Manual” by Steven S. Skiena
7.3. Online Courses
- Coursera’s “Algorithms Specialization” by Stanford University
- edX’s “Introduction to Computer Science” by Harvard University
- Udacity’s “Data Structures and Algorithms Nanodegree”
8. Developing a Growth Mindset
Approaching problem-solving with a growth mindset can significantly impact your performance under pressure:
- Embrace challenges as opportunities to learn
- View mistakes as valuable learning experiences
- Believe in your ability to improve through effort and practice
- Seek feedback and learn from criticism
9. The Day Before the Interview
Proper preparation the day before can help you feel more confident and relaxed:
- Review your strongest areas to boost confidence
- Get a good night’s sleep
- Prepare your interview outfit and any necessary materials
- Plan your route to the interview location or set up your space for a virtual interview
10. During the Interview
When you’re in the actual interview, remember these tips:
- Take a deep breath and calm yourself before starting
- Read the problem carefully and ask questions if anything is unclear
- Think out loud to involve the interviewer in your thought process
- If you get stuck, don’t panic – take a step back and consider alternative approaches
- If you finish early, take time to optimize your solution or discuss potential improvements
Conclusion
Preparing for problem-solving under pressure is a journey that requires dedication, consistent practice, and a strategic approach. By building a strong foundation, developing effective problem-solving strategies, simulating pressure situations, and honing both your technical and soft skills, you’ll be well-equipped to tackle even the most challenging coding interviews.
Remember, the goal isn’t just to pass the interview but to become a better problem solver overall. This skill will serve you well throughout your career in tech, helping you tackle real-world challenges and contribute meaningfully to your future projects and teams.
Keep practicing, stay curious, and approach each problem as an opportunity to learn and grow. With time and effort, you’ll find that what once seemed daunting becomes an exciting challenge you’re ready to take on. Good luck on your coding journey!