How to Prepare for Coding Interviews with Limited Time: A Comprehensive Guide
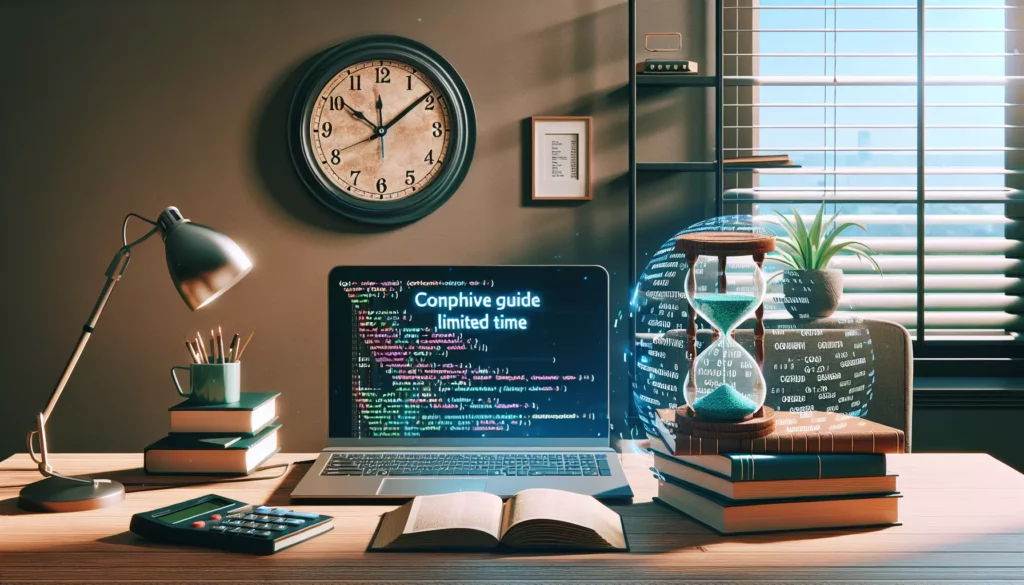
Preparing for coding interviews can be a daunting task, especially when you’re short on time. Whether you’re a busy professional looking to switch careers or a student juggling multiple responsibilities, mastering the art of efficient interview preparation is crucial. In this comprehensive guide, we’ll explore effective strategies and techniques to help you make the most of your limited time and increase your chances of success in coding interviews.
Understanding the Challenge
Coding interviews, particularly those conducted by major tech companies (often referred to as FAANG – Facebook, Amazon, Apple, Netflix, and Google), are known for their rigorous and challenging nature. These interviews typically assess your problem-solving skills, algorithmic thinking, and coding proficiency. With limited time to prepare, it’s essential to focus on the most critical aspects and adopt a strategic approach.
1. Prioritize Your Study Areas
When time is of the essence, it’s crucial to prioritize your study areas. Focus on the most commonly asked topics in coding interviews:
- Data Structures: Arrays, Linked Lists, Stacks, Queues, Trees, Graphs, Hash Tables
- Algorithms: Sorting, Searching, Recursion, Dynamic Programming
- Big O Notation and Time/Space Complexity Analysis
- Object-Oriented Programming Concepts
- System Design Basics (for more senior positions)
By concentrating on these core areas, you’ll cover a significant portion of what’s likely to be asked in your interviews.
2. Utilize Efficient Learning Resources
With limited time, it’s crucial to use resources that provide maximum value. Consider the following:
- Online Platforms: Websites like AlgoCademy offer interactive coding tutorials and AI-powered assistance, which can significantly speed up your learning process.
- Coding Books: “Cracking the Coding Interview” by Gayle Laakmann McDowell is a widely recommended resource that covers essential topics concisely.
- Video Tutorials: Platforms like YouTube offer free, high-quality content on various coding topics. Look for channels that focus on interview preparation.
- Coding Challenge Websites: LeetCode, HackerRank, and CodeSignal offer a wide range of coding problems similar to those asked in interviews.
3. Develop a Structured Study Plan
Creating a structured study plan is crucial when time is limited. Here’s a sample 4-week plan:
Week 1: Fundamentals and Easy Problems
- Review basic data structures and algorithms
- Solve 2-3 easy problems daily on LeetCode or similar platforms
- Focus on array and string manipulation problems
Week 2: Intermediate Concepts and Medium Problems
- Study trees, graphs, and dynamic programming
- Solve 2 medium problems daily
- Review and optimize your solutions
Week 3: Advanced Topics and Hard Problems
- Dive deeper into system design and OOP concepts
- Attempt 1-2 hard problems daily
- Practice explaining your thought process out loud
Week 4: Mock Interviews and Final Review
- Conduct mock interviews with friends or online platforms
- Review your weak areas
- Practice time management during problem-solving
4. Master the Art of Problem-Solving
Developing a systematic approach to problem-solving is crucial for coding interviews. Follow these steps:
- Understand the Problem: Carefully read the problem statement and ask clarifying questions if needed.
- Analyze the Input and Output: Identify the given inputs and expected outputs.
- Consider Edge Cases: Think about potential edge cases and how to handle them.
- Develop an Algorithm: Sketch out a high-level approach to solving the problem.
- Code the Solution: Implement your algorithm in the chosen programming language.
- Test and Debug: Run your code with various test cases and fix any issues.
- Optimize: Consider ways to improve the time and space complexity of your solution.
Practice this approach consistently to make it second nature during interviews.
5. Optimize Your Coding Environment
Efficiency is key when time is limited. Set up your coding environment for maximum productivity:
- Choose a Familiar IDE: Use an Integrated Development Environment (IDE) you’re comfortable with.
- Set Up Shortcuts: Learn and use keyboard shortcuts to speed up your coding.
- Use Code Snippets: Create and use code snippets for common operations to save time.
- Practice on a Whiteboard: Some interviews may require coding on a whiteboard, so practice this skill as well.
6. Focus on Time Management
During coding interviews, time management is crucial. Practice these techniques:
- Use the Pomodoro Technique: Work in focused 25-minute intervals with short breaks in between.
- Set Time Limits: When practicing problems, set a time limit to simulate interview conditions.
- Learn to Recognize When to Move On: If you’re stuck on a problem, know when to ask for a hint or move to the next question.
7. Leverage the Power of Spaced Repetition
Spaced repetition is a learning technique that involves reviewing material at increasing intervals. This method can help you retain information more effectively:
- Review concepts and problems you’ve studied after 1 day, then 3 days, then a week, and so on.
- Use flashcards or spaced repetition software to implement this technique efficiently.
- Focus more time on areas where you struggle and less on those you’ve mastered.
8. Practice Explaining Your Thought Process
In coding interviews, it’s not just about finding the right solution; it’s also about communicating your thought process clearly. Practice these skills:
- Think Aloud: As you solve problems, verbalize your thoughts and reasoning.
- Explain Your Approach: Before coding, explain your planned approach to the interviewer.
- Justify Your Decisions: Be prepared to explain why you chose a particular data structure or algorithm.
9. Familiarize Yourself with Common Coding Patterns
Many coding interview questions follow certain patterns. Recognizing these patterns can save you time during interviews. Some common patterns include:
- Two Pointers
- Sliding Window
- Fast and Slow Pointers
- Merge Intervals
- Cyclic Sort
- In-place Reversal of a Linked List
- Tree Breadth-First Search
- Tree Depth-First Search
- Two Heaps
- Subsets
- Modified Binary Search
- Top K Elements
- K-way Merge
- Topological Sort
Familiarize yourself with these patterns and practice problems that utilize them.
10. Implement a Mock Interview Strategy
Mock interviews are an excellent way to simulate real interview conditions and identify areas for improvement. Here’s how to make the most of them:
- Find a Study Buddy: Partner with a friend or classmate to conduct mock interviews for each other.
- Use Online Platforms: Websites like Pramp offer free peer-to-peer mock interviews.
- Record Your Sessions: If possible, record your mock interviews to review your performance later.
- Seek Feedback: Ask your mock interviewer for honest feedback on your problem-solving approach, communication skills, and areas for improvement.
11. Master the Art of Coding on a Whiteboard
Many tech companies conduct whiteboard coding interviews. To prepare for this unique challenge:
- Practice writing code on a physical whiteboard or using a digital whiteboard tool.
- Focus on writing clean, legible code.
- Learn to manage space effectively on the whiteboard.
- Practice erasing and rewriting parts of your code without losing track of your solution.
12. Develop Your System Design Skills
For more senior positions, system design questions are common. While this topic is vast, you can focus on key areas:
- Understand basic distributed systems concepts
- Learn about scalability, load balancing, and caching
- Familiarize yourself with database design and SQL vs. NoSQL databases
- Study real-world system architectures of popular services (e.g., Twitter, Netflix)
13. Brush Up on Computer Science Fundamentals
While problem-solving is crucial, don’t neglect computer science fundamentals. Quickly review:
- Operating Systems concepts
- Networking basics
- Database Management Systems
- Object-Oriented Programming principles
14. Practice Coding in Multiple Languages
While it’s important to have a primary language you’re comfortable with, being familiar with multiple languages can be beneficial:
- Choose a main language for your interviews (e.g., Python, Java, C++)
- Familiarize yourself with the syntax and basic operations of 1-2 additional languages
- Understand the strengths and weaknesses of different languages for various types of problems
15. Develop a Strategy for Handling Stress
Coding interviews can be stressful, especially when you’re under time pressure. Develop strategies to manage stress:
- Practice deep breathing exercises
- Use positive self-talk and affirmations
- Visualize success in your interviews
- Get enough sleep and exercise regularly to maintain overall well-being
16. Utilize Code Visualization Tools
Code visualization tools can help you understand complex algorithms and data structures more quickly:
- Use websites like VisuAlgo to visualize algorithms in action
- Practice tracing code execution mentally
- Draw diagrams to represent data structures and algorithm flow
17. Learn to Optimize Your Solutions
Interviewers often ask candidates to optimize their initial solutions. Practice these optimization techniques:
- Identify and eliminate unnecessary operations
- Use appropriate data structures to improve time complexity
- Apply caching or memoization to avoid redundant computations
- Consider space-time tradeoffs
18. Prepare for Behavioral Questions
While technical skills are crucial, don’t neglect preparing for behavioral questions. Many interviews include a mix of technical and behavioral components:
- Prepare stories about your past projects and experiences
- Practice answering common behavioral questions (e.g., “Tell me about a time you faced a challenge in a project”)
- Use the STAR method (Situation, Task, Action, Result) to structure your responses
19. Stay Updated with Industry Trends
Demonstrating awareness of current industry trends can impress interviewers:
- Follow tech news websites and blogs
- Familiarize yourself with emerging technologies relevant to the companies you’re interviewing with
- Participate in coding forums and communities to stay connected with industry developments
20. Prepare Questions for Your Interviewer
At the end of most interviews, you’ll have the opportunity to ask questions. Prepare thoughtful questions that demonstrate your interest and engagement:
- Ask about the company’s technology stack and development processes
- Inquire about opportunities for growth and learning within the role
- Ask about the team structure and collaboration practices
Conclusion
Preparing for coding interviews with limited time is challenging, but with the right strategies and focused effort, it’s entirely possible to succeed. Remember to prioritize your study areas, use efficient learning resources, and practice consistently. Focus on developing a solid problem-solving approach and communicating your thoughts clearly.
Platforms like AlgoCademy can be invaluable in your preparation, offering interactive tutorials, AI-powered assistance, and a wealth of resources tailored for coding interview preparation. Utilize these tools to maximize your learning efficiency and boost your confidence.
Lastly, remember that interview preparation is not just about memorizing solutions but about developing a problem-solving mindset. Even with limited time, aim to understand the underlying principles and patterns in coding problems. This understanding will serve you well not just in interviews, but throughout your programming career.
Good luck with your preparation, and remember that each practice session brings you one step closer to acing your coding interviews!