How to Prepare for Coding Interviews at Top Tech Companies: A Comprehensive Guide
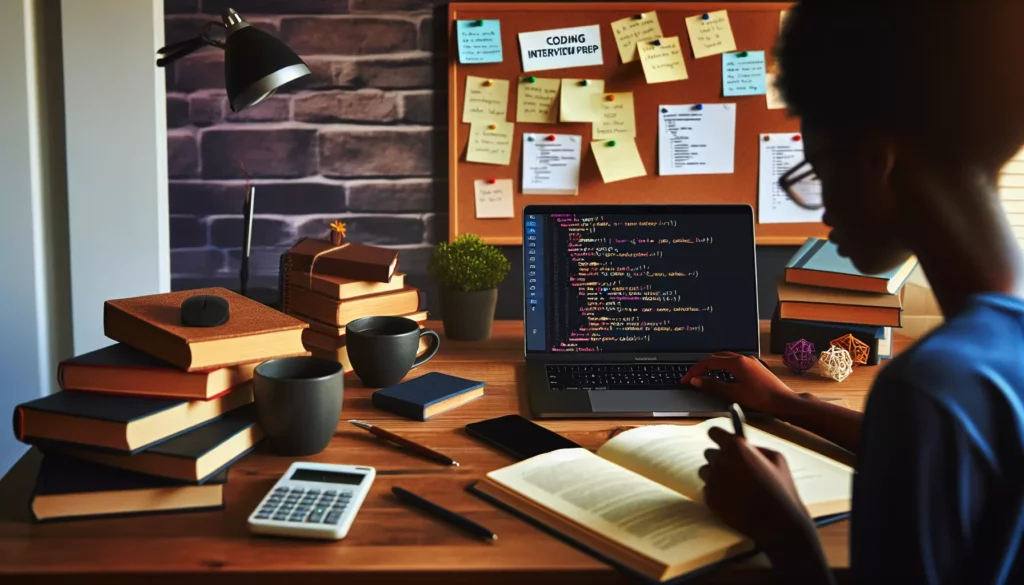
Landing a job at a top tech company like Google, Amazon, Facebook, Apple, or Microsoft (often collectively referred to as FAANG) is a dream for many software developers. However, the road to securing a position at these tech giants is paved with challenging coding interviews that test not only your programming skills but also your problem-solving abilities and algorithmic thinking. In this comprehensive guide, we’ll walk you through the essential steps to prepare for coding interviews at top tech companies, ensuring you’re ready to tackle any challenge that comes your way.
1. Understanding the Interview Process
Before diving into preparation strategies, it’s crucial to understand what the coding interview process typically entails at top tech companies:
- Phone Screening: An initial conversation with a recruiter to assess your background and interest.
- Technical Phone Interview: A remote coding interview where you’ll solve problems while explaining your thought process.
- On-site Interviews: Multiple rounds of face-to-face interviews, including coding challenges, system design discussions, and behavioral questions.
- Final Decision: Based on feedback from all interviewers, the company makes a hiring decision.
2. Mastering Data Structures and Algorithms
A solid foundation in data structures and algorithms is non-negotiable for coding interviews at top tech companies. Focus on the following areas:
2.1 Essential Data Structures
- Arrays and Strings
- Linked Lists
- Stacks and Queues
- Trees and Graphs
- Hash Tables
- Heaps
2.2 Key Algorithms
- Sorting (Quick Sort, Merge Sort, Heap Sort)
- Searching (Binary Search, Depth-First Search, Breadth-First Search)
- Dynamic Programming
- Greedy Algorithms
- Recursion and Backtracking
2.3 Practice Implementation
Don’t just memorize concepts; implement these data structures and algorithms from scratch. This hands-on practice will deepen your understanding and improve your coding skills.
// Example: Implementing a Binary Search Tree in Python
class Node:
def __init__(self, value):
self.value = value
self.left = None
self.right = None
class BinarySearchTree:
def __init__(self):
self.root = None
def insert(self, value):
if not self.root:
self.root = Node(value)
else:
self._insert_recursive(self.root, value)
def _insert_recursive(self, node, value):
if value < node.value:
if node.left is None:
node.left = Node(value)
else:
self._insert_recursive(node.left, value)
else:
if node.right is None:
node.right = Node(value)
else:
self._insert_recursive(node.right, value)
# Other methods like search, delete, etc. can be implemented here
3. Solving Coding Problems
Practice makes perfect, especially when it comes to coding interviews. Here’s how to approach problem-solving effectively:
3.1 Use Online Platforms
Leverage coding platforms that offer a wide range of problems similar to those asked in tech interviews:
- LeetCode
- HackerRank
- CodeSignal
- AlgoExpert
3.2 Follow a Structured Approach
When solving problems, follow these steps:
- Understand the problem thoroughly
- Clarify any ambiguities
- Identify the inputs and expected outputs
- Consider edge cases
- Develop a high-level approach
- Code the solution
- Test your code with various inputs
- Optimize if necessary
3.3 Time Your Practice Sessions
Most coding interviews have time constraints. Practice solving problems within a set timeframe to improve your speed and efficiency.
4. Mastering System Design
For senior roles or positions at top tech companies, system design questions are often a crucial part of the interview process. Here’s how to prepare:
4.1 Understand Key Concepts
- Scalability
- Load Balancing
- Caching
- Database Sharding
- Microservices Architecture
- API Design
4.2 Study Real-World Systems
Analyze how popular services like Twitter, Netflix, or Uber are designed. Understanding their architecture will give you insights into building large-scale systems.
4.3 Practice Design Exercises
Work on designing systems like:
- A URL shortener
- A social media feed
- A ride-sharing application
- A distributed file storage system
5. Improving Your Coding Skills
Beyond solving problems, focus on writing clean, efficient, and readable code:
5.1 Learn Best Practices
- Write modular and reusable code
- Follow naming conventions
- Comment your code effectively
- Understand and apply design patterns
5.2 Master Your Chosen Programming Language
While most top tech companies allow you to code in your preferred language, it’s crucial to have in-depth knowledge of at least one programming language. Know its syntax, standard libraries, and unique features inside out.
// Example: Using Python's list comprehension for concise code
# Instead of:
squares = []
for i in range(10):
squares.append(i**2)
# Use:
squares = [i**2 for i in range(10)]
5.3 Practice Code Reviews
Participate in open-source projects or review code with peers. This will improve your ability to read and understand others’ code, a skill often tested in interviews.
6. Behavioral Interview Preparation
Top tech companies don’t just assess your technical skills; they also evaluate your soft skills and cultural fit:
6.1 Prepare Your Stories
Have specific examples ready for common behavioral questions like:
- “Tell me about a time you faced a challenging problem at work.”
- “How do you handle conflicts in a team?”
- “Describe a project you’re particularly proud of.”
6.2 Use the STAR Method
Structure your responses using the STAR (Situation, Task, Action, Result) method to provide concise and relevant answers.
6.3 Research the Company
Understand the company’s culture, values, and recent projects. This knowledge will help you align your responses with what the company is looking for in candidates.
7. Mock Interviews and Feedback
Simulating the interview experience is crucial for success:
7.1 Conduct Mock Interviews
Practice with friends, mentors, or use platforms like Pramp or InterviewBit that offer peer-to-peer mock interviews.
7.2 Record Yourself
Record your mock interviews to review your performance, body language, and communication skills.
7.3 Seek Constructive Feedback
Ask your mock interviewers for honest feedback on your technical skills, problem-solving approach, and communication style.
8. Staying Updated with Industry Trends
Top tech companies value candidates who are passionate about technology and stay current with industry trends:
8.1 Follow Tech Blogs and News
Stay informed about the latest developments in technology, particularly in areas relevant to the companies you’re targeting.
8.2 Attend Tech Meetups and Conferences
Participate in local tech meetups or online conferences to network and learn about cutting-edge technologies.
8.3 Contribute to Open Source
Contributing to open-source projects demonstrates your passion for coding and ability to work on real-world applications.
9. Managing Interview Anxiety
Even with thorough preparation, interviews can be nerve-wracking. Here are some strategies to manage anxiety:
9.1 Practice Mindfulness
Incorporate mindfulness techniques like deep breathing or meditation into your daily routine to stay calm under pressure.
9.2 Visualize Success
Spend time visualizing yourself performing well in the interview. This positive mental rehearsal can boost your confidence.
9.3 Maintain a Healthy Lifestyle
Regular exercise, a balanced diet, and adequate sleep can significantly impact your mental state and interview performance.
10. The Day of the Interview
As the big day approaches, keep these final tips in mind:
10.1 Prepare Your Environment
For remote interviews, ensure you have a quiet space with a stable internet connection. Test your audio and video beforehand.
10.2 Arrive Early
Whether it’s a virtual or in-person interview, be ready at least 10-15 minutes before the scheduled time.
10.3 Bring Necessary Materials
Have a notebook, pen, and a copy of your resume handy. For virtual interviews, have a glass of water nearby.
10.4 Stay Calm and Communicate Clearly
Take deep breaths, speak clearly, and don’t hesitate to ask for clarification if you need it.
Conclusion
Preparing for coding interviews at top tech companies is a challenging but rewarding process. It requires dedication, consistent practice, and a strategic approach to learning and problem-solving. By focusing on mastering data structures and algorithms, honing your coding skills, practicing system design, and preparing for behavioral questions, you’ll be well-equipped to face even the most demanding interviews.
Remember, the journey to landing a job at a top tech company is as much about personal growth as it is about securing a position. Each problem you solve, each concept you master, and each mock interview you complete brings you one step closer to your goal. Stay persistent, embrace the learning process, and approach each interview as an opportunity to showcase your skills and passion for technology.
With thorough preparation and the right mindset, you’ll be well on your way to impressing interviewers and landing your dream job at a top tech company. Good luck!