How to Prepare for Algorithm-Based Interviews: A Comprehensive Guide
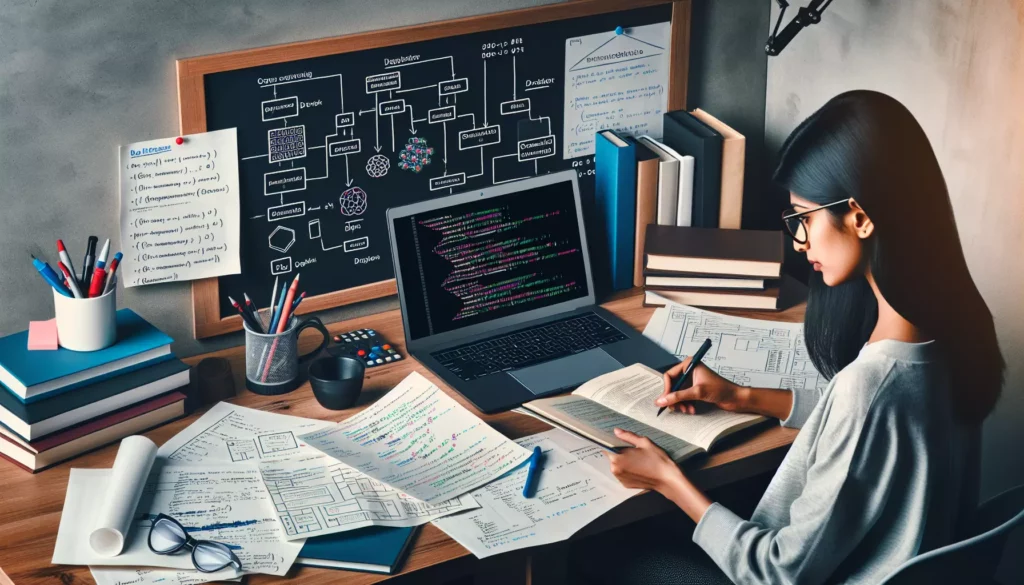
In today’s competitive tech industry, algorithm-based interviews have become a standard part of the hiring process for software engineering positions, especially at major tech companies often referred to as FAANG (Facebook, Amazon, Apple, Netflix, Google) or MANGA (Meta, Amazon, Netflix, Google, Apple). These interviews are designed to assess a candidate’s problem-solving skills, algorithmic thinking, and ability to write efficient code under pressure. If you’re aiming to land a job at one of these tech giants or any company that uses similar interview processes, it’s crucial to be well-prepared. In this comprehensive guide, we’ll walk you through the steps to effectively prepare for algorithm-based interviews.
1. Understanding the Nature of Algorithm-Based Interviews
Before diving into preparation strategies, it’s important to understand what algorithm-based interviews entail. These interviews typically involve:
- Solving coding problems in real-time
- Explaining your thought process as you work
- Analyzing time and space complexity of your solutions
- Optimizing your initial solutions
- Answering follow-up questions about your approach
The problems can range from basic data structure manipulations to complex algorithmic challenges. The interviewer is not just looking for a correct solution, but also assessing your problem-solving approach, coding style, and ability to communicate technical concepts clearly.
2. Building a Strong Foundation in Data Structures and Algorithms
A solid understanding of fundamental data structures and algorithms is crucial for success in these interviews. Focus on mastering the following:
Data Structures:
- Arrays and Strings
- Linked Lists
- Stacks and Queues
- Trees (Binary Trees, Binary Search Trees, Tries)
- Graphs
- Hash Tables
- Heaps
Algorithms:
- Sorting (Quick Sort, Merge Sort, Heap Sort)
- Searching (Binary Search, Depth-First Search, Breadth-First Search)
- Dynamic Programming
- Greedy Algorithms
- Recursion and Backtracking
- Graph Algorithms (Shortest Path, Minimum Spanning Tree)
For each of these topics, make sure you understand:
- The basic concept and implementation
- Time and space complexity
- Common use cases and problem-solving applications
- Advantages and disadvantages compared to other structures or algorithms
3. Practice, Practice, Practice
The key to excelling in algorithm-based interviews is consistent practice. Here are some effective ways to practice:
3.1 Solve Problems on Coding Platforms
Utilize online coding platforms that offer a wide range of algorithmic problems. Some popular options include:
- LeetCode
- HackerRank
- CodeSignal
- AlgoExpert
- Project Euler
Start with easier problems and gradually move to more complex ones. Try to solve at least one problem every day.
3.2 Implement Data Structures from Scratch
To deepen your understanding, try implementing common data structures from scratch in your preferred programming language. This exercise will help you grasp the inner workings of these structures and make you more comfortable using them in problem-solving.
3.3 Participate in Coding Contests
Platforms like Codeforces, TopCoder, and AtCoder host regular coding contests. Participating in these can help you improve your problem-solving speed and expose you to a variety of problem types.
3.4 Mock Interviews
Practice with a friend or use platforms like Pramp or InterviewBit that offer peer-to-peer mock interviews. This will help you get comfortable with explaining your thought process out loud and coding under time pressure.
4. Develop a Problem-Solving Approach
Having a structured approach to problem-solving is crucial. Here’s a recommended step-by-step process:
- Understand the problem: Read the problem statement carefully. Ask clarifying questions if needed.
- Identify the inputs and outputs: Clearly define what the function should take as input and what it should return.
- Consider edge cases: Think about potential edge cases or special scenarios that your solution needs to handle.
- Brainstorm solutions: Think of multiple approaches to solve the problem. Consider the trade-offs between different solutions.
- Choose an approach: Select the most appropriate solution based on the problem constraints and requirements.
- Plan your code: Outline the steps of your chosen approach before starting to code.
- Implement the solution: Write clean, readable code to implement your solution.
- Test your code: Run through example inputs and edge cases to verify your solution.
- Optimize: If time allows, think about ways to optimize your solution for better time or space complexity.
Practice applying this approach to every problem you solve. It will become second nature over time and help you tackle unfamiliar problems during interviews.
5. Master Time and Space Complexity Analysis
Understanding the efficiency of your algorithms is crucial. Interviewers often ask about the time and space complexity of your solutions. Make sure you can:
- Analyze the time and space complexity of your code using Big O notation
- Identify bottlenecks in your algorithms
- Explain trade-offs between time and space complexity
- Optimize your solutions for better efficiency
Practice analyzing the complexity of different algorithms and data structure operations. Be prepared to discuss how your solution’s complexity changes with different inputs or constraints.
6. Learn to Communicate Effectively
Communication is a crucial aspect of algorithm-based interviews. You need to articulate your thoughts clearly and explain your problem-solving process. Here are some tips:
- Think out loud: Verbalize your thought process as you work through the problem.
- Explain your approach: Before coding, explain your planned approach to the interviewer.
- Ask questions: If something is unclear, don’t hesitate to ask for clarification.
- Discuss trade-offs: When considering different approaches, explain the pros and cons of each.
- Use proper terminology: Use correct technical terms when discussing data structures and algorithms.
Practice explaining your solutions to a friend or record yourself solving problems out loud. This will help you become more comfortable with verbalizing your thoughts during the actual interview.
7. Familiarize Yourself with Common Interview Patterns
While each interview is unique, certain patterns and types of problems appear frequently. Familiarizing yourself with these can give you an edge. Some common patterns include:
- Two Pointers
- Sliding Window
- Fast and Slow Pointers
- Merge Intervals
- Cyclic Sort
- In-place Reversal of a Linked List
- Tree Breadth-First Search
- Tree Depth-First Search
- Two Heaps
- Subsets
- Modified Binary Search
- Top K Elements
- K-way Merge
- Topological Sort
Study these patterns and practice problems that utilize them. Understanding these patterns can help you quickly identify the type of problem you’re dealing with and apply the appropriate technique.
8. Choose a Programming Language and Master It
While most companies allow you to code in the language of your choice, it’s crucial to be proficient in at least one language. Choose a language you’re comfortable with and master its syntax, standard libraries, and built-in functions. Some popular choices for coding interviews include:
- Python
- Java
- C++
- JavaScript
Whichever language you choose, make sure you can:
- Write clean, readable code
- Implement common data structures efficiently
- Use built-in functions and libraries effectively
- Handle input/output operations
- Debug your code quickly
Practice coding without relying on an IDE’s auto-complete features, as you may not have access to these during the interview.
9. Study System Design (for Senior Roles)
If you’re interviewing for a senior software engineering role, you may also encounter system design questions. While not strictly algorithm-based, these questions often involve algorithmic thinking on a larger scale. To prepare for system design interviews:
- Study distributed systems concepts
- Learn about scalability, load balancing, and caching
- Understand database design and SQL vs NoSQL trade-offs
- Familiarize yourself with common architectures (microservices, event-driven, etc.)
- Practice designing popular systems (e.g., a URL shortener, a social media feed, a ride-sharing app)
Resources like “Designing Data-Intensive Applications” by Martin Kleppmann and “System Design Interview” by Alex Xu can be valuable for this aspect of preparation.
10. Develop Good Coding Habits
During the interview, it’s not just about getting the right answer—how you arrive at the solution matters too. Develop these good coding habits:
- Write clean, well-indented code
- Use meaningful variable and function names
- Break down complex problems into smaller, manageable functions
- Add comments to explain non-obvious parts of your code
- Handle edge cases and potential errors gracefully
- Write modular, reusable code when possible
Practice these habits in your daily coding and problem-solving sessions so they become second nature during interviews.
11. Stay Calm and Manage Your Time
Interview pressure can be overwhelming, but staying calm is crucial for clear thinking. Here are some tips to manage stress and time during the interview:
- Take a deep breath before starting the problem
- If you’re stuck, communicate with the interviewer—they may provide hints
- Don’t spend too much time on a single approach if it’s not working
- If you can’t solve the entire problem, solve as much as you can
- Practice time management during your preparation—give yourself time limits for solving problems
Remember, the interviewer is not just looking for the correct answer, but also assessing how you handle challenges and pressure.
12. Leverage Online Resources and Courses
There are numerous online resources available to help you prepare for algorithm-based interviews. Some recommended resources include:
- “Cracking the Coding Interview” by Gayle Laakmann McDowell
- “Elements of Programming Interviews” by Adnan Aziz, Tsung-Hsien Lee, and Amit Prakash
- AlgoExpert’s video explanations and coding problems
- MIT OpenCourseWare’s Algorithms course
- Coursera’s Algorithms Specialization by Stanford University
- YouTube channels like Back To Back SWE and Abdul Bari for algorithm explanations
Utilize these resources to supplement your learning and gain different perspectives on problem-solving approaches.
13. Review Your Past Work and Projects
While algorithm-based questions form a significant part of the interview, be prepared to discuss your past work experiences and projects. Review your resume and be ready to:
- Explain the technologies and algorithms you’ve used in your projects
- Discuss challenges you faced and how you overcame them
- Highlight your contributions to team projects
- Explain how you’ve applied algorithmic thinking to real-world problems
This preparation will help you draw connections between your practical experience and the theoretical knowledge tested in algorithm questions.
14. Prepare Questions for the Interviewer
At the end of most interviews, you’ll have the opportunity to ask questions. Prepare thoughtful questions about the company, team, and role. This shows your genuine interest and engagement. Some example questions could be:
- “What kind of projects would I be working on in this role?”
- “How does the team approach problem-solving and algorithm optimization in your day-to-day work?”
- “Can you tell me about the engineering culture and how it promotes learning and growth?”
These questions can also help you gauge if the company and role align with your career goals and interests.
Conclusion
Preparing for algorithm-based interviews requires dedication, consistent practice, and a structured approach. By following the steps outlined in this guide, you’ll be well-equipped to tackle even the most challenging interview questions. Remember, the goal is not just to memorize solutions, but to develop strong problem-solving skills and the ability to apply algorithmic thinking to new challenges.
As you prepare, keep in mind that interview processes can vary between companies. Some may include additional stages like behavioral interviews or take-home coding assignments. Always research the specific interview process of the company you’re applying to and tailor your preparation accordingly.
Lastly, don’t get discouraged if you don’t solve every problem perfectly during your interviews. The process is designed to be challenging, and interviewers are often more interested in your problem-solving approach and how you handle difficult questions than in perfect solutions. Stay positive, keep practicing, and approach each interview as a learning opportunity. With persistence and the right preparation, you’ll be well on your way to succeeding in algorithm-based interviews and landing your dream job in the tech industry.