How to Prepare for a Full Stack Developer Interview: A Comprehensive Guide
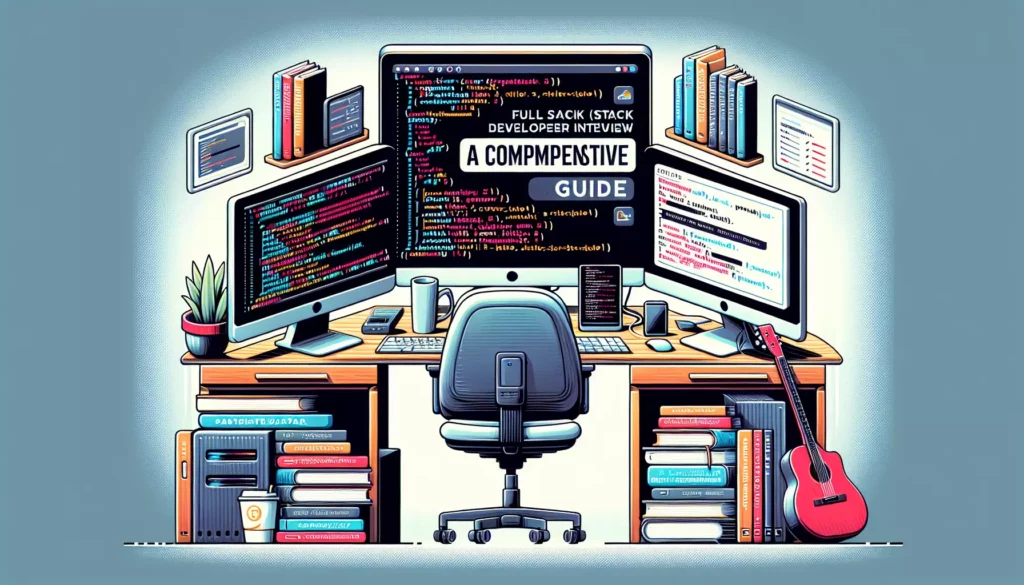
As the tech industry continues to evolve, the demand for full stack developers remains high. These versatile professionals are capable of working on both the front-end and back-end of web applications, making them valuable assets to any development team. If you’re aiming to land a full stack developer position, thorough preparation for the interview process is crucial. In this comprehensive guide, we’ll walk you through the essential steps to prepare for a full stack developer interview, covering everything from technical skills to soft skills and interview strategies.
1. Understanding the Full Stack Developer Role
Before diving into interview preparation, it’s important to have a clear understanding of what a full stack developer does. Full stack developers are expected to have a broad range of skills that span the entire web development process. This typically includes:
- Front-end technologies (HTML, CSS, JavaScript, and frameworks like React or Angular)
- Back-end programming languages (such as Python, Ruby, Java, or Node.js)
- Database management (SQL and NoSQL databases)
- Server management and deployment
- Version control systems (like Git)
- API development and integration
- Basic understanding of web security and performance optimization
Understanding the breadth of the role will help you focus your preparation efforts on the most relevant areas.
2. Brush Up on Your Technical Skills
The core of any full stack developer interview will be an assessment of your technical skills. Here are the key areas to focus on:
2.1. Front-end Development
Ensure you’re comfortable with the following:
- HTML5 semantics and best practices
- CSS3, including responsive design and CSS preprocessors (e.g., Sass or Less)
- JavaScript fundamentals and ES6+ features
- Popular front-end frameworks (React, Angular, or Vue.js)
- State management (Redux, MobX, or Vuex)
- Web accessibility principles
2.2. Back-end Development
Be prepared to discuss:
- Server-side programming languages (Python, Ruby, Java, Node.js, etc.)
- RESTful API design and implementation
- Authentication and authorization mechanisms
- Database design and management (both SQL and NoSQL)
- Server deployment and scaling strategies
2.3. DevOps and Version Control
Familiarize yourself with:
- Git workflows and best practices
- Continuous Integration/Continuous Deployment (CI/CD) pipelines
- Containerization technologies like Docker
- Cloud platforms (AWS, Google Cloud, or Azure)
2.4. Data Structures and Algorithms
Many interviews will include coding challenges or questions related to data structures and algorithms. Review key concepts such as:
- Arrays, linked lists, stacks, and queues
- Trees and graphs
- Sorting and searching algorithms
- Dynamic programming
- Time and space complexity analysis
3. Practice Coding Problems
To sharpen your problem-solving skills and prepare for technical interviews, it’s essential to practice coding problems regularly. Here are some strategies:
- Solve problems on platforms like LeetCode, HackerRank, or CodeSignal
- Participate in coding competitions or hackathons
- Contribute to open-source projects
- Implement small projects that showcase your full stack skills
When practicing, focus on:
- Writing clean, readable code
- Optimizing your solutions for time and space complexity
- Explaining your thought process as you solve problems (this will help during whiteboard interviews)
4. Build a Strong Portfolio
A well-curated portfolio can set you apart from other candidates. Consider including:
- Full stack projects that demonstrate your ability to work with various technologies
- Contributions to open-source projects
- A personal website or blog showcasing your skills and thought leadership
- Code samples that highlight your best work
Make sure your portfolio is easily accessible online and keep it up-to-date with your latest projects and skills.
5. Stay Current with Industry Trends
The tech industry evolves rapidly, and interviewers often look for candidates who stay current with new developments. To stay informed:
- Follow tech blogs and news sites
- Attend webinars and conferences
- Participate in online developer communities
- Experiment with new technologies and frameworks
Be prepared to discuss recent developments in full stack development and how they might impact your work.
6. Prepare for Common Interview Questions
While every interview is different, there are some common questions you’re likely to encounter. Practice answering questions such as:
- “Describe a challenging project you’ve worked on and how you overcame obstacles.”
- “How do you ensure the security of a web application?”
- “Explain the concept of RESTful APIs and their importance in full stack development.”
- “How would you optimize the performance of a slow-loading web page?”
- “Describe your experience with Agile development methodologies.”
Prepare concise, clear answers that highlight your experience and problem-solving skills.
7. Brush Up on System Design
For more senior positions, you may be asked system design questions. Familiarize yourself with concepts like:
- Scalability and load balancing
- Caching strategies
- Database sharding
- Microservices architecture
- Content delivery networks (CDNs)
Practice designing scalable systems and be prepared to explain your design choices.
8. Develop Your Soft Skills
Technical skills are crucial, but soft skills can often make the difference in landing a job. Focus on developing:
- Communication skills: Practice explaining complex technical concepts in simple terms
- Teamwork: Be ready to discuss your experiences working in a team environment
- Problem-solving: Showcase your ability to approach challenges methodically
- Adaptability: Demonstrate your willingness to learn new technologies and adapt to changing requirements
- Time management: Discuss how you prioritize tasks and meet deadlines
9. Research the Company and Position
Before your interview, thoroughly research the company and the specific role you’re applying for. This will help you:
- Tailor your responses to align with the company’s needs and culture
- Ask informed questions about the role and the company’s tech stack
- Demonstrate genuine interest in the position
Look for information about the company’s products, recent news, and technological challenges they might be facing.
10. Prepare for Different Interview Formats
Full stack developer interviews can take various formats. Be prepared for:
- Technical screenings: Usually involving coding challenges or technical questions
- Whiteboard interviews: Where you’ll solve problems on a whiteboard or shared screen
- Take-home projects: More extensive coding tasks to be completed in your own time
- Pair programming sessions: Coding alongside an interviewer
- Behavioral interviews: Focusing on your past experiences and how you handle various situations
Each format requires a slightly different approach, so practice accordingly.
11. Mock Interviews and Feedback
One of the best ways to prepare is through mock interviews. Consider:
- Practicing with a friend or mentor in the industry
- Using online platforms that offer mock technical interviews
- Recording yourself answering common interview questions and reviewing your performance
Seek honest feedback and work on improving any weak areas identified during these practice sessions.
12. Prepare Your Own Questions
At the end of most interviews, you’ll have the opportunity to ask questions. Prepare thoughtful questions that demonstrate your interest and knowledge, such as:
- “What are the biggest challenges facing the development team right now?”
- “How does the company approach continuous learning and professional development for its engineers?”
- “Can you tell me more about the team structure and how full stack developers collaborate with other roles?”
- “What technologies or frameworks is the company considering adopting in the near future?”
13. Technical Interview Tips
When it comes to the technical portion of your interview, keep these tips in mind:
- Think out loud: Explain your thought process as you work through problems
- Ask clarifying questions: Ensure you fully understand the problem before diving into a solution
- Consider edge cases: Show that you think about potential issues and edge cases in your solutions
- Start with a simple solution: Begin with a working solution, then optimize if time allows
- Test your code: Demonstrate your attention to detail by testing your code, even in a theoretical setting
14. Handling Coding Challenges
When faced with a coding challenge during the interview, follow these steps:
- Carefully read and understand the problem statement
- Ask any clarifying questions
- Plan your approach before starting to code
- Write clean, well-commented code
- Test your solution with various inputs, including edge cases
- Optimize your solution if time permits
- Be prepared to explain your code and discuss alternative approaches
Here’s an example of how you might approach a common coding challenge:
// Problem: Implement a function to reverse a string in-place
function reverseString(str) {
// Convert string to array for in-place manipulation
let arr = str.split('');
let left = 0;
let right = arr.length - 1;
// Swap characters from both ends moving towards the center
while (left < right) {
[arr[left], arr[right]] = [arr[right], arr[left]];
left++;
right--;
}
// Convert array back to string and return
return arr.join('');
}
// Test the function
console.log(reverseString("Hello, World!")); // Output: "!dlroW ,olleH"
Remember to explain your approach, discuss the time and space complexity, and consider any potential optimizations or alternative solutions.
15. Post-Interview Follow-up
After the interview:
- Send a thank-you email within 24 hours, reiterating your interest in the position
- Reflect on the interview experience and note any areas where you could improve
- If you don’t hear back within the expected timeframe, follow up politely
Conclusion
Preparing for a full stack developer interview requires dedication and a multifaceted approach. By focusing on strengthening your technical skills, practicing coding problems, building a strong portfolio, and developing your soft skills, you’ll be well-equipped to tackle any interview challenge. Remember that interview preparation is an ongoing process – the more you practice and learn, the more confident and competent you’ll become.
Leverage resources like AlgoCademy to enhance your algorithmic thinking and problem-solving skills. Take advantage of interactive coding tutorials, AI-powered assistance, and step-by-step guidance to refine your coding abilities and prepare for technical interviews at top tech companies.
With thorough preparation and the right mindset, you’ll be well on your way to impressing interviewers and landing your dream full stack developer position. Good luck with your interview preparation!