How to Prepare for a Data Structures and Algorithms Interview: A Comprehensive Guide
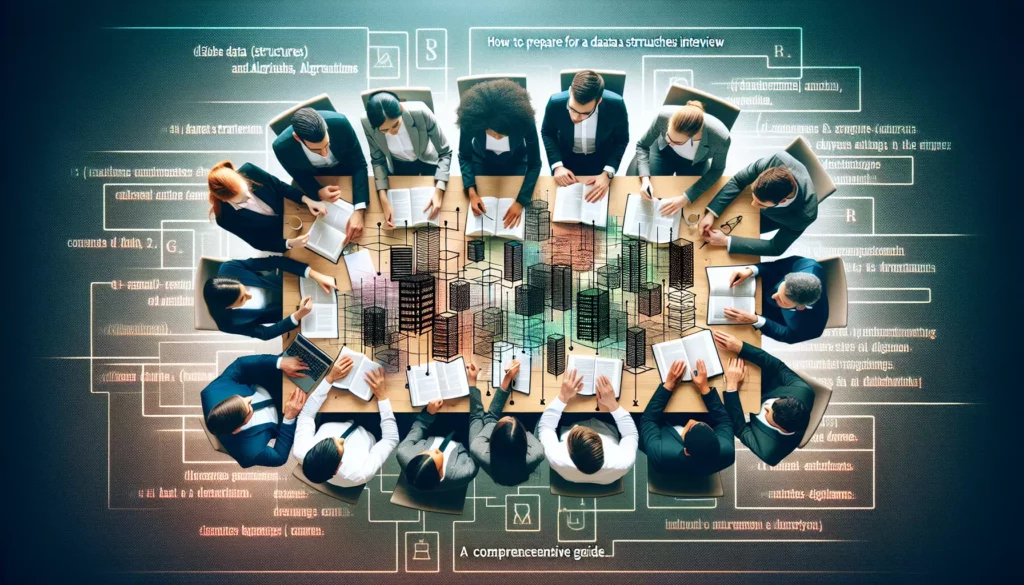
Preparing for a data structures and algorithms (DSA) interview can be a daunting task, especially if you’re aiming for positions at top tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google). These interviews are known for their rigorous assessment of candidates’ problem-solving skills, algorithmic thinking, and coding proficiency. In this comprehensive guide, we’ll walk you through the essential steps to effectively prepare for a DSA interview, helping you boost your confidence and increase your chances of success.
1. Understanding the Importance of DSA Interviews
Before diving into the preparation process, it’s crucial to understand why tech companies place such a high emphasis on DSA interviews:
- Problem-solving skills: DSA questions assess your ability to analyze complex problems and devise efficient solutions.
- Algorithmic thinking: These interviews evaluate your capacity to think algorithmically and optimize solutions.
- Code quality: Your coding style, cleanliness, and ability to write bug-free code are also under scrutiny.
- Performance under pressure: DSA interviews simulate real-world scenarios where you need to think and code quickly.
2. Establishing a Strong Foundation
To excel in DSA interviews, you need a solid foundation in core computer science concepts. Focus on the following areas:
2.1. Data Structures
Master the following fundamental data structures:
- Arrays and Strings
- Linked Lists
- Stacks and Queues
- Trees (Binary Trees, Binary Search Trees, AVL Trees)
- Heaps
- Hash Tables
- Graphs
- Tries
For each data structure, understand its properties, implementation details, time and space complexities, and common operations.
2.2. Algorithms
Familiarize yourself with essential algorithmic techniques:
- Sorting algorithms (QuickSort, MergeSort, HeapSort)
- Searching algorithms (Binary Search, Depth-First Search, Breadth-First Search)
- Dynamic Programming
- Greedy Algorithms
- Divide and Conquer
- Recursion and Backtracking
- Two Pointers
- Sliding Window
2.3. Time and Space Complexity Analysis
Develop a strong understanding of Big O notation and how to analyze the time and space complexity of algorithms. This skill is crucial for optimizing your solutions and discussing trade-offs during interviews.
3. Creating a Study Plan
With a clear understanding of the fundamentals, it’s time to create a structured study plan:
3.1. Set Realistic Goals
Determine how much time you can dedicate to preparation and set achievable goals. For example, aim to solve a certain number of problems per week or master a specific topic within a given timeframe.
3.2. Choose Learning Resources
Select high-quality learning materials to guide your preparation:
- Books: “Cracking the Coding Interview” by Gayle Laakmann McDowell, “Introduction to Algorithms” by Cormen et al.
- Online Platforms: LeetCode, HackerRank, AlgoExpert, InterviewBit
- Video Courses: Coursera, edX, or Udemy courses on DSA
- Interactive Coding Platforms: AlgoCademy, which offers AI-powered assistance and step-by-step guidance
3.3. Develop a Routine
Establish a consistent study routine that fits your schedule. Dedicate specific times for learning new concepts, solving problems, and reviewing your progress.
4. Problem-Solving Strategies
Effective problem-solving is at the heart of DSA interviews. Here are some strategies to improve your skills:
4.1. Use the UMPIRE Method
When approaching a problem, follow these steps:
- Understand: Carefully read and comprehend the problem statement.
- Match: Identify similar problems you’ve encountered before.
- Plan: Devise a strategy to solve the problem.
- Implement: Write clean, efficient code to implement your solution.
- Review: Double-check your code for correctness and optimization opportunities.
- Evaluate: Analyze the time and space complexity of your solution.
4.2. Practice Regularly
Consistent practice is key to improving your problem-solving skills. Aim to solve at least one or two problems daily, gradually increasing the difficulty level as you progress.
4.3. Time Yourself
Practice solving problems within time constraints to simulate interview conditions. Start with 45-60 minutes per problem and work on improving your speed over time.
4.4. Implement Solutions from Scratch
After solving a problem, try implementing the solution again from scratch without referring to your previous code. This helps reinforce your understanding and improves your coding speed.
5. Mastering Common Problem Types
Familiarize yourself with frequently asked problem types in DSA interviews:
5.1. Array and String Manipulation
Practice problems involving:
- Two-pointer techniques
- Sliding window
- Prefix sums
- String matching and parsing
5.2. Linked List Operations
Focus on:
- Reversing a linked list
- Detecting cycles
- Merging sorted lists
- Finding the middle element
5.3. Tree and Graph Traversals
Master various traversal techniques:
- Inorder, Preorder, and Postorder traversals
- Level-order traversal
- Depth-First Search (DFS)
- Breadth-First Search (BFS)
5.4. Dynamic Programming
Practice classic DP problems like:
- Fibonacci sequence
- Longest Common Subsequence
- Knapsack problem
- Coin change problem
5.5. Sorting and Searching
Implement and understand various sorting and searching algorithms:
- QuickSort, MergeSort, HeapSort
- Binary Search and its variations
- Counting Sort and Radix Sort for specific scenarios
6. Optimizing Your Solutions
Interviewers often ask candidates to optimize their initial solutions. Here are some techniques to improve your code:
6.1. Space-Time Trade-offs
Learn to identify opportunities to trade space for time or vice versa. For example, using a hash table to reduce time complexity at the cost of additional space.
6.2. Preprocessing
Consider preprocessing the input data to speed up subsequent operations. This is particularly useful in problems involving multiple queries on the same dataset.
6.3. Caching and Memoization
Use caching techniques to store and reuse previously computed results, especially in recursive or dynamic programming problems.
6.4. Bit Manipulation
Learn common bit manipulation techniques to optimize certain operations, particularly for problems involving integers or binary representations.
7. Mock Interviews and Code Reviews
Simulating real interview conditions is crucial for preparation:
7.1. Conduct Mock Interviews
Practice with friends, colleagues, or use platforms like Pramp or InterviewBit that offer peer-to-peer mock interviews. This helps you get comfortable with explaining your thought process while coding.
7.2. Code Reviews
Regularly review your code and seek feedback from more experienced developers. This helps improve your code quality and exposes you to different problem-solving approaches.
7.3. Virtual Whiteboarding
Practice coding on a virtual whiteboard or a simple text editor to simulate the constraints of a real interview environment where you might not have access to an IDE.
8. Behavioral Preparation
While technical skills are crucial, don’t neglect the behavioral aspect of interviews:
8.1. Communicate Clearly
Practice explaining your thought process out loud as you solve problems. This skill is highly valued by interviewers.
8.2. Ask Clarifying Questions
Don’t hesitate to ask for clarification on problem requirements or constraints. This shows your attention to detail and commitment to understanding the problem fully.
8.3. Handle Pressure
Develop strategies to manage stress during interviews, such as deep breathing exercises or positive self-talk.
9. Final Preparation Tips
As your interview date approaches, keep these final tips in mind:
9.1. Review Your Past Solutions
Go through the problems you’ve solved and refresh your memory on the approaches you used.
9.2. Focus on Your Weak Areas
Identify topics or problem types you struggle with and dedicate extra time to improving in these areas.
9.3. Stay Updated
Keep abreast of the latest developments in data structures and algorithms, especially if you’re interviewing for a specific company known for certain technologies.
9.4. Rest and Relax
Ensure you get enough rest in the days leading up to your interview. A well-rested mind performs better under pressure.
Conclusion
Preparing for a data structures and algorithms interview requires dedication, consistent practice, and a structured approach. By following this comprehensive guide and leveraging resources like AlgoCademy’s interactive coding tutorials and AI-powered assistance, you can significantly improve your chances of success in these challenging interviews.
Remember that the journey of mastering DSA is ongoing, and each problem you solve contributes to your growth as a developer. Stay persistent, embrace the learning process, and approach each interview as an opportunity to showcase your skills and learn something new.
With thorough preparation and the right mindset, you’ll be well-equipped to tackle even the most demanding DSA interviews at top tech companies. Good luck!