How to Practice Time-Bounded Problem Solving: A Comprehensive Guide
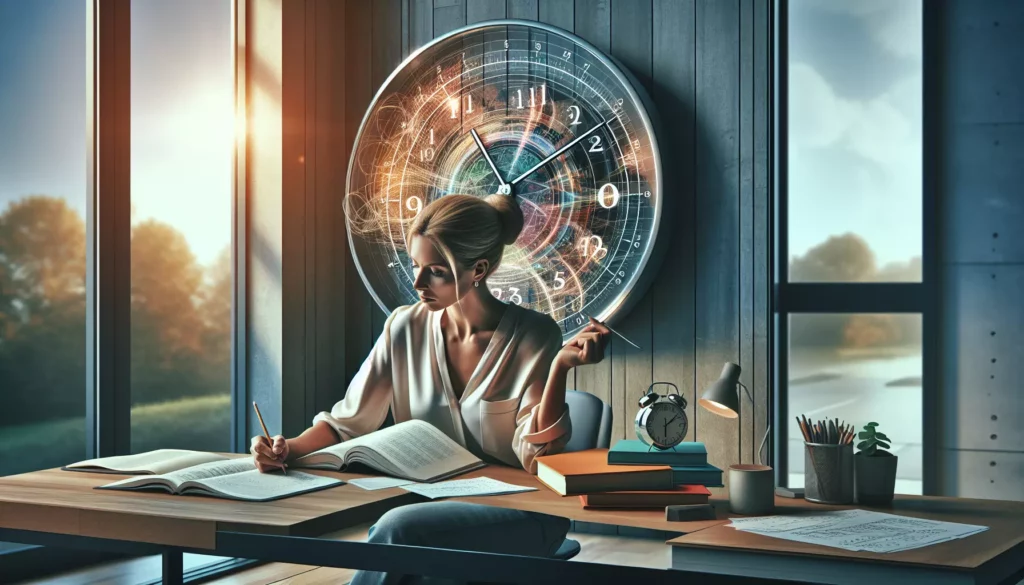
In the fast-paced world of coding interviews and technical assessments, time-bounded problem solving is a crucial skill that can make or break your chances of landing that dream job at a top tech company. Whether you’re aiming for a position at one of the FAANG (Facebook, Amazon, Apple, Netflix, Google) companies or any other competitive tech firm, mastering the art of solving complex problems under time constraints is essential. In this comprehensive guide, we’ll explore effective strategies and techniques to help you excel in time-bounded problem solving, with a focus on coding challenges and algorithmic thinking.
Understanding Time-Bounded Problem Solving
Time-bounded problem solving refers to the ability to tackle complex coding challenges within a limited timeframe. This skill is particularly important in technical interviews, where candidates are often given a set amount of time to solve a programming problem. The goal is not just to arrive at the correct solution, but to do so efficiently and within the allotted time.
Key aspects of time-bounded problem solving include:
- Quick comprehension of the problem statement
- Efficient algorithm design
- Rapid implementation of the solution
- Time management
- Handling pressure
The Importance of Time-Bounded Problem Solving in Coding Interviews
Tech companies, especially FAANG and other industry leaders, place a high value on candidates who can demonstrate strong problem-solving skills under pressure. Here’s why time-bounded problem solving is crucial in coding interviews:
- Simulates real-world scenarios: In actual work environments, developers often need to solve problems quickly and efficiently.
- Tests adaptability: It shows how well you can think on your feet and adapt to new challenges.
- Evaluates coding speed and accuracy: It allows interviewers to assess both the quality and speed of your coding.
- Demonstrates algorithmic thinking: Time constraints force you to consider optimal solutions rather than brute-force approaches.
- Assesses stress management: It reveals how well you perform under pressure, a valuable trait in high-stakes tech environments.
Strategies for Effective Time-Bounded Problem Solving
1. Understand the Problem Quickly
The first step in tackling any time-bounded problem is to quickly and accurately understand what’s being asked. Here are some tips:
- Read the problem statement carefully, but efficiently.
- Identify the key components: input, output, and constraints.
- Ask clarifying questions if anything is unclear (in an interview setting).
- Mentally summarize the problem to ensure you’ve grasped it fully.
2. Plan Before You Code
Resist the urge to start coding immediately. Spend a few minutes planning your approach:
- Sketch out a high-level solution.
- Consider different algorithms and data structures that might be applicable.
- Think about edge cases and potential pitfalls.
- Estimate the time and space complexity of your proposed solution.
3. Start with a Brute Force Solution
If you’re struggling to find an optimal solution immediately, start with a brute force approach:
- Implement the simplest solution that comes to mind.
- This ensures you have at least some working code.
- Use this as a foundation to optimize further if time allows.
4. Optimize Incrementally
Once you have a working solution, look for ways to improve it:
- Identify bottlenecks in your current implementation.
- Consider more efficient data structures or algorithms.
- Look for redundant operations that can be eliminated.
- Apply problem-specific optimizations based on the given constraints.
5. Use Pseudocode
When time is of the essence, using pseudocode can be a valuable technique:
- Quickly outline your algorithm in plain English or simplified code.
- This helps organize your thoughts before diving into the actual implementation.
- It’s easier to spot logical errors at this stage.
6. Practice Time Management
Effective time management is crucial in time-bounded problem solving:
- Allocate specific time slots for understanding, planning, coding, and testing.
- Set mental checkpoints to assess your progress.
- Be prepared to move on if you’re stuck on a particular aspect for too long.
7. Test as You Go
Don’t wait until the end to test your code:
- Write and test small sections of code as you implement them.
- This helps catch errors early and saves time in the long run.
- Consider edge cases and boundary conditions during testing.
Common Pitfalls to Avoid
When practicing time-bounded problem solving, be aware of these common mistakes:
- Rushing into coding: Skipping the planning phase can lead to inefficient solutions or misunderstanding the problem.
- Overcomplicating the solution: Sometimes, a simple approach is best, especially under time constraints.
- Ignoring time complexity: A solution that works for small inputs might fail for larger datasets.
- Neglecting edge cases: Failing to consider boundary conditions can result in incomplete solutions.
- Poor time management: Spending too much time on one aspect of the problem can leave you scrambling at the end.
- Forgetting to communicate: In an interview setting, explaining your thought process is as important as the solution itself.
Tools and Resources for Practice
To improve your time-bounded problem solving skills, consider using these resources:
- Online coding platforms: Websites like LeetCode, HackerRank, and CodeSignal offer timed coding challenges.
- Mock interview websites: Platforms like Pramp or interviewing.io provide realistic interview experiences with time constraints.
- Coding competitions: Participate in online coding contests to practice solving problems under time pressure.
- AlgoCademy: Utilize AlgoCademy’s interactive tutorials and AI-powered assistance to enhance your problem-solving skills.
- Data structures and algorithms books: Classic texts like “Introduction to Algorithms” by Cormen et al. can deepen your understanding of efficient problem-solving techniques.
Implementing a Practice Routine
To effectively improve your time-bounded problem solving skills, establish a consistent practice routine:
- Set aside dedicated practice time: Aim for at least 1-2 hours of focused practice daily.
- Start with easier problems: Build confidence by solving simpler problems within time constraints before moving to more complex ones.
- Gradually increase difficulty: As you improve, challenge yourself with harder problems and shorter time limits.
- Review and reflect: After each practice session, analyze your performance and identify areas for improvement.
- Learn from solutions: Study efficient solutions to problems you struggled with, understanding the underlying concepts and techniques.
- Practice different problem types: Cover a wide range of algorithmic concepts and data structures in your practice.
- Simulate interview conditions: Periodically practice under conditions that mimic real coding interviews, including explaining your thought process out loud.
Advanced Techniques for Time-Bounded Problem Solving
As you become more comfortable with basic time-bounded problem solving, consider incorporating these advanced techniques:
1. Pattern Recognition
Develop the ability to quickly recognize common problem patterns. This can significantly speed up your problem-solving process. Some common patterns include:
- Two-pointer technique
- Sliding window
- Dynamic programming
- Depth-first search (DFS) and Breadth-first search (BFS)
- Binary search variations
2. Preemptive Optimization
As you read the problem, start thinking about potential optimizations:
- Consider the input size and constraints to gauge the required time complexity.
- Think about appropriate data structures that could optimize your solution.
- Look for opportunities to use mathematical properties or shortcuts.
3. Modular Coding
Write modular, reusable code to save time and improve readability:
- Create helper functions for repetitive tasks.
- Use clear, descriptive variable names to enhance code understanding.
- Structure your code in a way that makes it easy to modify or extend.
4. Space-Time Tradeoffs
Be prepared to make intelligent tradeoffs between time and space complexity:
- Understand when it’s beneficial to use extra space to reduce time complexity.
- Consider in-place algorithms when space is a constraint.
- Be familiar with techniques like memoization and tabulation for optimizing recursive solutions.
Handling Stress and Pressure
Time-bounded problem solving can be stressful, especially in high-stakes situations like coding interviews. Here are some strategies to manage stress and perform well under pressure:
- Practice mindfulness: Incorporate mindfulness techniques or meditation into your routine to improve focus and reduce anxiety.
- Simulate pressure: Regularly practice under conditions that mimic the stress of real interviews.
- Develop a pre-solving ritual: Create a short routine (e.g., taking a deep breath, mentally reviewing your problem-solving steps) to center yourself before tackling each problem.
- Embrace the challenge: Try to view time constraints as an exciting challenge rather than a source of stress.
- Stay physically healthy: Regular exercise, adequate sleep, and a balanced diet can significantly impact your mental performance and stress resistance.
Leveraging AI and Modern Tools
While developing your problem-solving skills is crucial, it’s also important to be aware of modern tools and AI-powered resources that can aid in your learning and practice:
- AI-powered code assistants: Tools like GitHub Copilot or AlgoCademy’s AI assistant can help you learn efficient coding patterns and suggest optimizations.
- Interactive coding environments: Use platforms that provide instant feedback and visualization of your code execution.
- Code analysis tools: Utilize static code analysis tools to identify potential improvements in your solutions.
- Version control systems: Practice using Git to manage different versions of your solutions, allowing you to experiment with various approaches.
Real-World Application
While time-bounded problem solving is crucial for coding interviews, the skills you develop have broader applications in your programming career:
- Debugging under pressure: Quickly identifying and fixing critical bugs in production environments.
- Rapid prototyping: Developing proof-of-concept solutions in hackathons or time-sensitive projects.
- Optimizing legacy code: Improving the efficiency of existing codebases within time constraints.
- Meeting tight deadlines: Delivering feature implementations or bug fixes on schedule.
- Technical leadership: Guiding team members in solving complex problems efficiently.
Conclusion
Mastering time-bounded problem solving is a journey that requires consistent practice, a strategic approach, and continuous learning. By incorporating the techniques and strategies outlined in this guide, you can significantly enhance your ability to tackle coding challenges efficiently and effectively. Remember that improvement comes with time and experience, so be patient with yourself and celebrate your progress along the way.
As you continue to hone your skills, you’ll find that the ability to solve complex problems under time constraints not only helps in coding interviews but also makes you a more effective and valuable programmer in real-world scenarios. Embrace the challenge, stay curious, and keep pushing your boundaries. With dedication and the right approach, you’ll be well-equipped to excel in time-bounded problem solving and take your coding career to new heights.