How to Practice Problem-Solving Consistently: A Comprehensive Guide for Aspiring Programmers
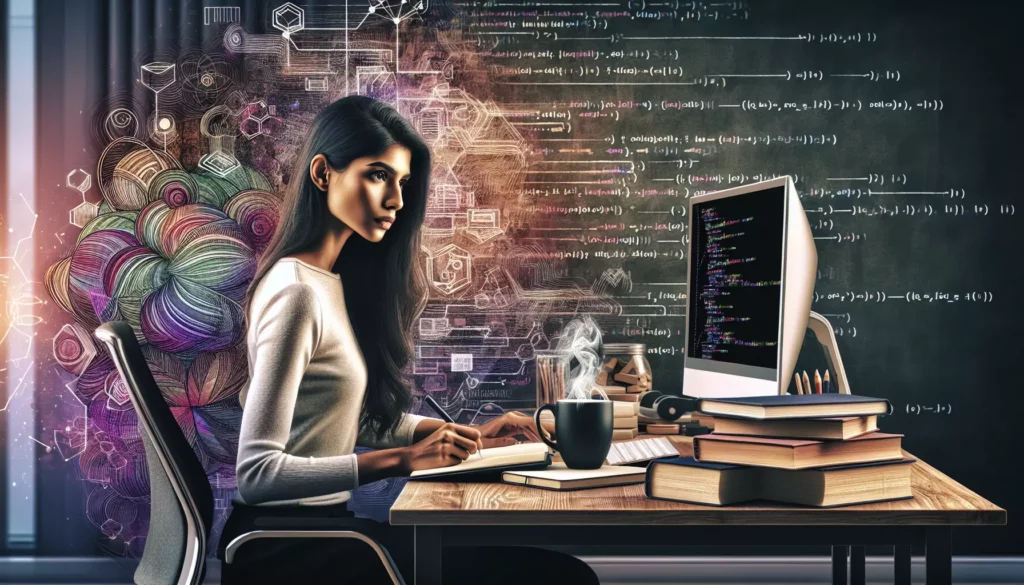
In the ever-evolving world of technology, problem-solving skills are the backbone of successful programming. Whether you’re a beginner just starting your coding journey or an experienced developer aiming to crack those coveted FAANG (Facebook, Amazon, Apple, Netflix, Google) interviews, consistent practice in problem-solving is key. This comprehensive guide will walk you through effective strategies to hone your problem-solving skills, with a focus on algorithmic thinking and practical coding techniques.
Why Consistent Problem-Solving Practice Matters
Before diving into the ‘how’, let’s understand the ‘why’. Consistent problem-solving practice is crucial because:
- It strengthens your analytical thinking abilities
- It helps you approach complex problems with confidence
- It prepares you for technical interviews, especially for top tech companies
- It improves your coding efficiency and speed
- It exposes you to various problem-solving patterns and algorithmic techniques
Now that we’ve established the importance, let’s explore how you can make problem-solving a consistent part of your coding routine.
1. Set a Regular Schedule
Consistency is key when it comes to improving your problem-solving skills. Set aside dedicated time each day or week for problem-solving practice. Even 30 minutes to an hour daily can make a significant difference over time.
Tips for Setting a Schedule:
- Choose a time when you’re most alert and focused
- Use calendar reminders or apps to stick to your schedule
- Start small and gradually increase your practice time
- Be realistic with your goals to avoid burnout
2. Use Online Platforms and Resources
Leverage online platforms that offer a wide range of coding problems and challenges. These platforms often categorize problems by difficulty level and topic, allowing you to systematically improve your skills.
Popular Platforms for Problem-Solving Practice:
- LeetCode
- HackerRank
- CodeSignal
- Project Euler
- Codeforces
- AlgoCademy
AlgoCademy, in particular, offers interactive coding tutorials and AI-powered assistance, making it an excellent resource for beginners and those preparing for technical interviews.
3. Start with the Basics and Progress Gradually
If you’re new to problem-solving, start with basic problems and gradually increase the difficulty. This approach helps build confidence and prevents frustration.
Progression Path:
- Basic array and string manipulation
- Simple math problems
- Basic data structures (linked lists, stacks, queues)
- Elementary sorting and searching algorithms
- Tree and graph basics
- Dynamic programming introduction
- Advanced algorithms and complex problem-solving
4. Focus on Understanding, Not Just Solving
While solving problems is important, understanding the underlying concepts and patterns is crucial. After solving a problem, take time to analyze different approaches and their time and space complexities.
Steps for Deep Understanding:
- Solve the problem on your own
- Look at other solutions and understand their approach
- Analyze the time and space complexity of different solutions
- Try to implement the most efficient solution
- Reflect on what you learned and how it can be applied to other problems
5. Implement a Variety of Problem-Solving Techniques
Familiarize yourself with various problem-solving techniques and algorithms. This will equip you with a toolbox of strategies to tackle different types of problems.
Essential Problem-Solving Techniques:
- Brute Force
- Divide and Conquer
- Dynamic Programming
- Greedy Algorithms
- Backtracking
- Two Pointers
- Sliding Window
- Depth-First Search (DFS) and Breadth-First Search (BFS)
6. Time Yourself
As you become more comfortable with problem-solving, start timing yourself. This mimics the pressure of a real interview situation and helps improve your efficiency.
Time Management Tips:
- Start with generous time limits and gradually reduce them
- Practice solving problems within typical interview time frames (usually 20-30 minutes per problem)
- Learn to quickly identify the problem type and choose the appropriate approach
7. Practice Explaining Your Thought Process
In technical interviews, explaining your thought process is as important as solving the problem. Practice verbalizing your approach, even when solving problems alone.
Steps to Improve Your Explanation Skills:
- Start by writing down your thought process
- Practice explaining your approach out loud
- Record yourself solving problems and review the recordings
- Participate in mock interviews or coding sessions with peers
8. Learn from Your Mistakes
Mistakes are an integral part of the learning process. When you encounter a problem you can’t solve or make a mistake, use it as a learning opportunity.
Steps to Learn from Mistakes:
- Identify where you went wrong
- Understand the correct approach
- Implement the solution and ensure you understand every step
- Revisit similar problems to reinforce your learning
9. Implement Solutions in Multiple Programming Languages
While mastering one programming language is important, being versatile can be beneficial. Try implementing solutions in different languages to broaden your skills.
Popular Languages for Coding Interviews:
- Python
- Java
- C++
- JavaScript
- Go
10. Participate in Coding Contests and Hackathons
Coding contests and hackathons provide an excellent opportunity to apply your problem-solving skills in a competitive environment. They also expose you to a wide range of problems and allow you to learn from others.
Benefits of Participating in Contests:
- Exposure to diverse problem types
- Time management under pressure
- Opportunity to learn from other participants
- Motivation to improve your skills
11. Collaborate and Discuss with Peers
Discussing problems and solutions with peers can provide new perspectives and deepen your understanding. Join coding communities or study groups to engage in collaborative learning.
Ways to Collaborate:
- Join online coding communities (e.g., Stack Overflow, Reddit’s r/learnprogramming)
- Participate in local coding meetups
- Form study groups with classmates or colleagues
- Engage in pair programming sessions
12. Understand the Underlying Data Structures
A solid understanding of data structures is crucial for effective problem-solving. Make sure you’re comfortable with the following data structures and their operations:
- Arrays and Strings
- Linked Lists
- Stacks and Queues
- Trees (Binary Trees, Binary Search Trees, Balanced Trees)
- Graphs
- Hash Tables
- Heaps
13. Focus on Time and Space Complexity
Understanding the time and space complexity of your solutions is crucial, especially for technical interviews. Practice analyzing and optimizing your code for better performance.
Steps to Improve Complexity Analysis:
- Learn Big O notation thoroughly
- Analyze the time and space complexity of every solution you implement
- Practice optimizing brute force solutions
- Study common time complexities of different algorithms and data structures
14. Implement a Spaced Repetition System
Spaced repetition is a learning technique that involves reviewing material at increasing intervals. This method can be highly effective for retaining problem-solving patterns and concepts.
Implementing Spaced Repetition:
- Solve a problem
- Review it after 1 day
- If you remember it well, review after 3 days
- If still well-remembered, review after a week, then two weeks, and so on
- If you struggle at any point, reset the interval and start over
15. Use Visualization Tools
Visual representations can greatly enhance your understanding of algorithms and data structures. Use tools and websites that offer algorithm visualizations to reinforce your learning.
Useful Visualization Resources:
- VisuAlgo
- Algorithm Visualizer
- Sorting Algorithms Visualizations
- Data Structure Visualizations
16. Practice Mock Interviews
As you progress in your problem-solving journey, start practicing mock interviews. This will help you get comfortable with the interview process and improve your ability to solve problems under pressure.
Mock Interview Resources:
- Pramp
- InterviewBit
- Gainlo
- AlgoCademy’s interview preparation tools
17. Read and Understand Other People’s Code
Reading and understanding code written by others can expose you to different problem-solving approaches and coding styles. This skill is also valuable in real-world software development.
Ways to Practice Code Reading:
- Review solutions on coding platforms after solving problems
- Contribute to open-source projects
- Read and understand popular algorithms implementations
- Review code in technical blogs and articles
18. Implement Classic Algorithms from Scratch
Understanding and implementing classic algorithms is crucial for developing strong problem-solving skills. Try implementing these algorithms from scratch:
- Sorting algorithms (Quicksort, Mergesort, Heapsort)
- Search algorithms (Binary Search, Depth-First Search, Breadth-First Search)
- Graph algorithms (Dijkstra’s, Bellman-Ford, Floyd-Warshall)
- Dynamic Programming algorithms (Knapsack, Longest Common Subsequence)
19. Keep a Problem-Solving Journal
Maintaining a journal of the problems you’ve solved, along with your approaches and learnings, can be incredibly beneficial. It serves as a personal reference and helps track your progress.
What to Include in Your Journal:
- Problem statement
- Your initial approach
- Final solution (with code)
- Time and space complexity analysis
- Key learnings and insights
- Similar problems or patterns observed
20. Stay Updated with Current Trends
The field of computer science and programming is constantly evolving. Stay updated with current trends, new algorithms, and problem-solving techniques.
Ways to Stay Updated:
- Follow tech blogs and websites
- Attend webinars and conferences
- Subscribe to coding newsletters
- Follow thought leaders on social media
Conclusion
Consistent practice in problem-solving is essential for developing strong programming skills and preparing for technical interviews, especially for top tech companies. By following these strategies and maintaining a regular practice routine, you can significantly improve your problem-solving abilities and boost your confidence in tackling complex coding challenges.
Remember, the key to mastering problem-solving is persistence and consistent practice. Don’t get discouraged by difficult problems; view them as opportunities to learn and grow. With time and dedication, you’ll find yourself tackling even the most challenging problems with ease and confidence.
Happy coding and problem-solving!