How to Practice Fundamentals While Building Projects: A Comprehensive Guide
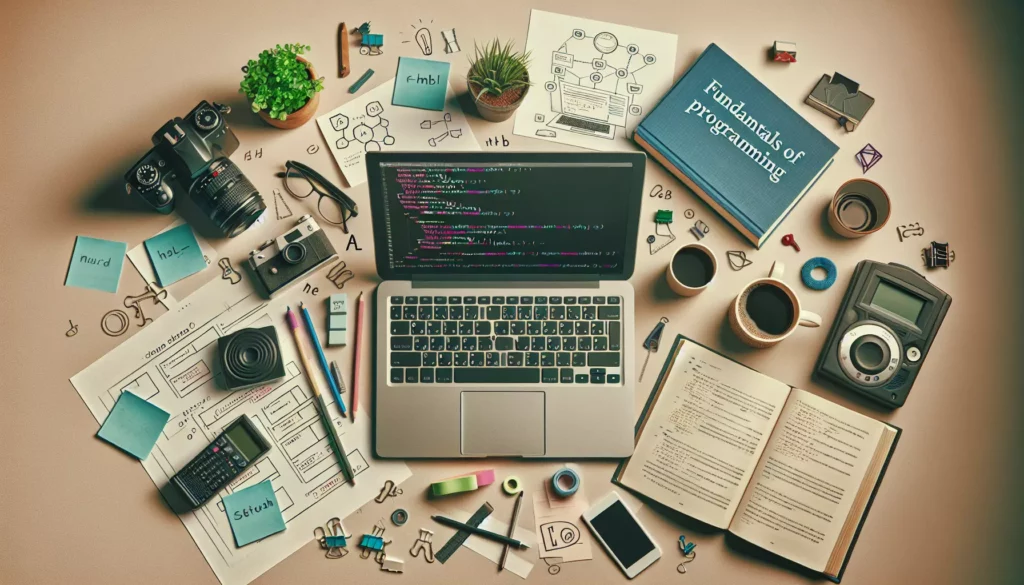
In the world of software development, there’s an ongoing debate about the best way to learn and improve coding skills. Some argue that focusing on fundamentals and theory is crucial, while others advocate for a project-based approach. But what if you could combine both? This comprehensive guide will explore how to practice fundamental programming concepts while simultaneously building real-world projects, helping you become a more well-rounded and effective developer.
The Importance of Balancing Theory and Practice
Before diving into specific strategies, it’s essential to understand why balancing theoretical knowledge with practical application is crucial for your growth as a developer:
- Deeper Understanding: Practicing fundamentals helps you grasp the core concepts that underpin all programming languages and paradigms.
- Problem-Solving Skills: Building projects challenges you to apply your knowledge in real-world scenarios, improving your problem-solving abilities.
- Motivation: Working on projects keeps you engaged and motivated, as you can see tangible results of your efforts.
- Portfolio Building: Completed projects serve as excellent additions to your portfolio, showcasing your skills to potential employers.
Strategies for Practicing Fundamentals in Projects
1. Start with a Solid Foundation
Before embarking on complex projects, ensure you have a strong grasp of programming basics:
- Data types and variables
- Control structures (if/else statements, loops)
- Functions and methods
- Object-oriented programming concepts
- Basic data structures (arrays, lists, dictionaries)
Resources like AlgoCademy offer interactive tutorials and exercises to help you master these fundamentals. Once you feel comfortable with the basics, you can start incorporating them into your projects.
2. Choose Projects That Align with Your Learning Goals
Select projects that allow you to practice specific fundamental concepts. For example:
- To practice data structures: Build a task management application using arrays or linked lists.
- To focus on algorithms: Create a sorting visualization tool that implements various sorting algorithms.
- To improve object-oriented programming skills: Develop a simple game with multiple classes and inheritance.
3. Implement Core Concepts from Scratch
Instead of relying solely on built-in functions or libraries, challenge yourself to implement fundamental concepts from scratch in your projects. This approach deepens your understanding of how things work under the hood. For instance:
- Implement your own sorting algorithm instead of using a language’s built-in sort function.
- Create a custom data structure, like a binary search tree, rather than using a pre-built one.
- Build a simple web server from scratch to understand HTTP protocols better.
4. Refactor and Optimize Your Code
As you build your project, regularly revisit and refactor your code. This practice helps reinforce fundamental concepts and improves your code quality. Consider the following:
- Optimize algorithms for better time and space complexity.
- Refactor repetitive code into reusable functions or classes.
- Apply design patterns to improve code structure and maintainability.
5. Document Your Learning Process
Keep a coding journal or blog where you document the challenges you face and the solutions you discover. This practice helps solidify your understanding of fundamental concepts and serves as a valuable resource for future reference. Include:
- Explanations of key algorithms or data structures used in your project
- Reflections on design decisions and their implications
- Lessons learned from debugging complex issues
6. Incorporate Test-Driven Development (TDD)
Adopting a test-driven development approach in your projects can help reinforce fundamental concepts while ensuring code quality. Follow these steps:
- Write tests for a specific function or feature before implementing it.
- Implement the minimum code necessary to pass the tests.
- Refactor the code while ensuring all tests still pass.
This approach forces you to think critically about edge cases and expected behavior, deepening your understanding of the underlying concepts.
7. Collaborate with Others
Working on projects with other developers can expose you to different approaches and reinforce your understanding of fundamentals. Consider:
- Participating in open-source projects
- Joining coding meetups or hackathons
- Engaging in pair programming sessions
Collaboration challenges you to explain your thought process and defend your design decisions, which further cements your grasp of core concepts.
Practical Examples: Fundamentals in Action
Let’s explore some concrete examples of how you can practice fundamental concepts while building projects:
Example 1: Building a Todo List Application
A todo list app is a classic project that can help you practice several fundamental concepts:
- Data Structures: Use an array or a linked list to store todo items.
- CRUD Operations: Implement functions to create, read, update, and delete todo items.
- Sorting and Filtering: Add features to sort todos by date or priority, and filter completed vs. incomplete items.
- Local Storage: Implement data persistence using browser local storage or a simple file-based system.
Here’s a simple example of how you might structure a Todo class in JavaScript:
<script>
class Todo {
constructor(id, title, completed = false, createdAt = new Date()) {
this.id = id;
this.title = title;
this.completed = completed;
this.createdAt = createdAt;
}
toggle() {
this.completed = !this.completed;
}
}
class TodoList {
constructor() {
this.todos = [];
}
addTodo(title) {
const id = this.todos.length + 1;
const newTodo = new Todo(id, title);
this.todos.push(newTodo);
return newTodo;
}
removeTodo(id) {
this.todos = this.todos.filter(todo => todo.id !== id);
}
getTodos() {
return this.todos;
}
sortByDate() {
this.todos.sort((a, b) => b.createdAt - a.createdAt);
}
}
// Usage example
const myTodoList = new TodoList();
myTodoList.addTodo("Learn JavaScript");
myTodoList.addTodo("Build a todo app");
console.log(myTodoList.getTodos());
myTodoList.sortByDate();
</script>
Example 2: Implementing a Search Algorithm
Creating a search feature for your project allows you to practice fundamental algorithms:
- Linear Search: Implement a basic search for small datasets.
- Binary Search: Optimize search for sorted data.
- Indexing: Create an index to improve search efficiency for larger datasets.
Here’s an example of a binary search implementation in Python:
def binary_search(arr, target):
left, right = 0, len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1 # Target not found
# Usage example
sorted_array = [1, 3, 5, 7, 9, 11, 13, 15]
result = binary_search(sorted_array, 7)
print(f"Element found at index: {result}")
Example 3: Building a Simple Database
Creating a basic database system can help you practice fundamental concepts related to data storage and retrieval:
- File I/O: Implement reading from and writing to files for data persistence.
- Data Serialization: Use JSON or a similar format to store structured data.
- Indexing: Create simple indexes to optimize data retrieval.
- ACID Properties: Implement basic transaction support to ensure data integrity.
Here’s a simple example of a key-value store implemented in Python:
import json
import os
class SimpleDB:
def __init__(self, filename):
self.filename = filename
self.data = {}
self.load()
def load(self):
if os.path.exists(self.filename):
with open(self.filename, 'r') as f:
self.data = json.load(f)
def save(self):
with open(self.filename, 'w') as f:
json.dump(self.data, f)
def set(self, key, value):
self.data[key] = value
self.save()
def get(self, key):
return self.data.get(key)
def delete(self, key):
if key in self.data:
del self.data[key]
self.save()
# Usage example
db = SimpleDB('mydb.json')
db.set('user1', {'name': 'Alice', 'age': 30})
print(db.get('user1'))
db.delete('user1')
Advanced Techniques for Combining Fundamentals and Projects
As you become more comfortable with basic concepts, you can explore more advanced techniques to further enhance your skills:
1. Implement Design Patterns
Design patterns are reusable solutions to common programming problems. Incorporating them into your projects helps you practice advanced object-oriented concepts and improve code structure. Some patterns to explore include:
- Singleton Pattern
- Factory Pattern
- Observer Pattern
- Strategy Pattern
2. Explore Different Programming Paradigms
While building projects, challenge yourself to use different programming paradigms to solve problems. This approach broadens your understanding of fundamental concepts across various styles of coding:
- Functional Programming: Focus on immutability and pure functions.
- Object-Oriented Programming: Emphasize encapsulation, inheritance, and polymorphism.
- Procedural Programming: Organize code into procedures and functions.
3. Implement Core Features of Programming Languages
To deepen your understanding of how programming languages work, try implementing some of their core features in your projects:
- Build a simple interpreter or compiler
- Implement a basic garbage collector
- Create a simple type system
4. Focus on Performance Optimization
As your projects grow in complexity, pay attention to performance optimization. This practice reinforces your understanding of algorithms and data structures:
- Profile your code to identify bottlenecks
- Implement caching mechanisms
- Optimize database queries
- Use appropriate data structures for efficient operations
5. Implement Security Best Practices
Incorporating security considerations into your projects helps you understand fundamental concepts related to data protection and secure coding:
- Implement proper authentication and authorization
- Use encryption for sensitive data
- Protect against common vulnerabilities (e.g., SQL injection, XSS)
Overcoming Challenges and Staying Motivated
Balancing fundamental practice with project building can be challenging. Here are some tips to help you stay on track:
1. Set Realistic Goals
Break down your learning objectives and project milestones into small, achievable tasks. This approach helps maintain motivation and provides a sense of progress.
2. Join a Community
Engage with other developers through online forums, local meetups, or platforms like AlgoCademy. Sharing experiences and seeking advice can provide valuable support and inspiration.
3. Embrace the Learning Process
Remember that making mistakes and encountering challenges are essential parts of the learning process. View each obstacle as an opportunity to deepen your understanding.
4. Regularly Review and Reflect
Set aside time to review your progress, reflect on what you’ve learned, and identify areas for improvement. This practice helps reinforce your knowledge and guides your future learning efforts.
5. Seek Feedback
Share your projects with peers or mentors and ask for constructive feedback. External perspectives can highlight areas where you can improve and provide new insights into fundamental concepts.
Conclusion
Practicing fundamental programming concepts while building projects is a powerful approach to becoming a well-rounded and effective developer. By choosing appropriate projects, implementing core concepts from scratch, and continuously challenging yourself, you can deepen your understanding of essential programming principles while creating meaningful applications.
Remember that the journey of a developer is one of continuous learning. As you progress, you’ll find that your growing knowledge of fundamentals will enable you to tackle increasingly complex projects, while your project experience will give context and depth to your understanding of core concepts.
Platforms like AlgoCademy can be invaluable resources in this journey, offering structured learning paths, interactive coding exercises, and AI-powered assistance to help you master both theoretical concepts and practical skills. By leveraging these resources and applying the strategies outlined in this guide, you’ll be well-equipped to grow as a developer and prepare for the challenges of a career in software development.