How to Perform Unit Testing and Integration Testing: A Comprehensive Guide
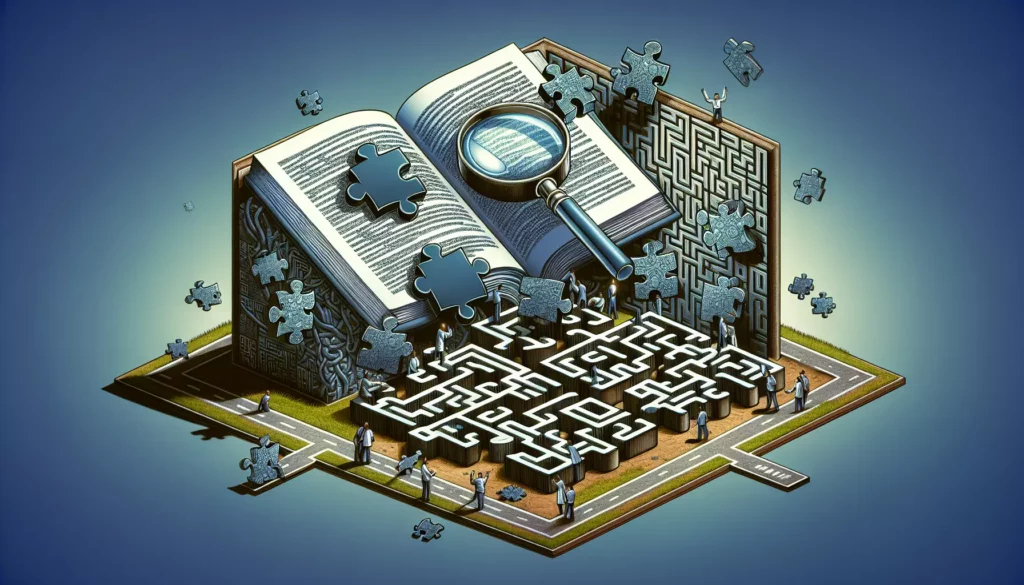
In the world of software development, ensuring the quality and reliability of your code is paramount. Two essential techniques that help achieve this goal are unit testing and integration testing. These testing methodologies play crucial roles in identifying bugs, verifying functionality, and maintaining the overall health of your software projects. In this comprehensive guide, we’ll dive deep into the concepts of unit testing and integration testing, explore their importance, and provide practical examples to help you implement these testing strategies in your own projects.
Table of Contents
- Understanding Unit Testing
- Implementing Unit Tests
- Best Practices for Unit Testing
- Introduction to Integration Testing
- Types of Integration Testing
- Implementing Integration Tests
- Best Practices for Integration Testing
- Tools for Unit and Integration Testing
- Combining Unit and Integration Testing
- Common Challenges and Solutions
- Conclusion
Understanding Unit Testing
Unit testing is a software testing technique where individual units or components of a software are tested in isolation. The primary goal of unit testing is to validate that each unit of the software performs as designed. A unit is the smallest testable part of any software, which is typically a function, method, or class.
Key Characteristics of Unit Testing:
- Isolation: Units are tested independently of other components.
- Automation: Unit tests are typically automated and can be run quickly and frequently.
- Specificity: Each test focuses on a specific functionality or behavior.
- Quick Feedback: Unit tests provide immediate feedback on the correctness of the code.
Benefits of Unit Testing:
- Early bug detection and prevention
- Improved code quality and maintainability
- Facilitates refactoring and code changes
- Serves as documentation for the expected behavior of code units
- Increases confidence in the codebase
Implementing Unit Tests
To implement effective unit tests, you need to follow a structured approach. Let’s walk through the process of creating unit tests using a simple example in Python with the unittest
framework.
Example: Testing a Simple Calculator Class
First, let’s create a simple Calculator class with basic arithmetic operations:
class Calculator:
def add(self, a, b):
return a + b
def subtract(self, a, b):
return a - b
def multiply(self, a, b):
return a * b
def divide(self, a, b):
if b == 0:
raise ValueError("Cannot divide by zero")
return a / b
Now, let’s write unit tests for this Calculator class:
import unittest
from calculator import Calculator
class TestCalculator(unittest.TestCase):
def setUp(self):
self.calc = Calculator()
def test_add(self):
self.assertEqual(self.calc.add(3, 5), 8)
self.assertEqual(self.calc.add(-1, 1), 0)
self.assertEqual(self.calc.add(-1, -1), -2)
def test_subtract(self):
self.assertEqual(self.calc.subtract(5, 3), 2)
self.assertEqual(self.calc.subtract(1, 1), 0)
self.assertEqual(self.calc.subtract(-1, -1), 0)
def test_multiply(self):
self.assertEqual(self.calc.multiply(3, 5), 15)
self.assertEqual(self.calc.multiply(-1, 1), -1)
self.assertEqual(self.calc.multiply(-1, -1), 1)
def test_divide(self):
self.assertEqual(self.calc.divide(6, 3), 2)
self.assertEqual(self.calc.divide(-6, 3), -2)
self.assertEqual(self.calc.divide(5, 2), 2.5)
def test_divide_by_zero(self):
with self.assertRaises(ValueError):
self.calc.divide(5, 0)
if __name__ == '__main__':
unittest.main()
In this example, we’ve created a test class TestCalculator
that inherits from unittest.TestCase
. Each test method starts with test_
and checks a specific functionality of the Calculator class. We use various assertion methods provided by unittest
to verify the expected behavior of each method.
Best Practices for Unit Testing
To make the most of unit testing, follow these best practices:
- Keep tests simple and focused: Each test should verify a single behavior or functionality.
- Use descriptive test names: Test names should clearly indicate what is being tested and the expected outcome.
- Test both positive and negative scenarios: Include tests for expected behavior as well as edge cases and error conditions.
- Maintain test independence: Each test should be able to run independently of others.
- Use setup and teardown methods: Utilize
setUp()
andtearDown()
methods to prepare the test environment and clean up after tests. - Mock external dependencies: Use mocking frameworks to isolate the unit being tested from external dependencies.
- Aim for high code coverage: Strive to cover as much of your code as possible with unit tests, but focus on critical paths and complex logic.
- Keep tests fast: Unit tests should execute quickly to encourage frequent running.
- Refactor tests along with code: As your code evolves, make sure to update and refactor your tests accordingly.
Introduction to Integration Testing
While unit testing focuses on individual components, integration testing is concerned with verifying that different parts of the application work correctly when combined. Integration testing aims to detect issues in the interactions between integrated components or systems.
Key Characteristics of Integration Testing:
- Component Interaction: Tests focus on the communication between different parts of the system.
- Broader Scope: Integration tests cover larger portions of the application compared to unit tests.
- Realistic Environment: Tests are often performed in an environment that closely resembles the production setup.
- Longer Execution Time: Integration tests typically take longer to run than unit tests due to their broader scope.
Benefits of Integration Testing:
- Verifies that components work together as expected
- Identifies interface defects between modules
- Ensures the overall system behaves correctly
- Helps catch issues that may not be apparent in unit testing
- Validates the system’s compliance with functional requirements
Types of Integration Testing
There are several approaches to integration testing, each with its own advantages and use cases:
1. Big Bang Integration Testing
In this approach, all components are integrated simultaneously and tested as a whole. This method is suitable for small systems but can be challenging for larger, more complex applications.
2. Incremental Integration Testing
Components are integrated and tested one at a time. This approach can be further divided into:
- Top-Down Integration: Testing starts with high-level modules and gradually incorporates lower-level modules.
- Bottom-Up Integration: Lower-level modules are tested first, and testing progresses upwards to higher-level modules.
- Sandwich Integration (Hybrid): A combination of top-down and bottom-up approaches.
3. Continuous Integration Testing
This modern approach involves automatically building and testing the entire application whenever changes are committed to the version control system. It helps catch integration issues early in the development process.
Implementing Integration Tests
Let’s look at an example of how to implement integration tests using Python and the pytest
framework. We’ll create a simple order processing system with multiple components and test their integration.
Example: Order Processing System
First, let’s define our components:
class Inventory:
def __init__(self):
self.items = {"apple": 10, "banana": 5, "orange": 7}
def check_availability(self, item, quantity):
return item in self.items and self.items[item] >= quantity
def update_stock(self, item, quantity):
if item in self.items:
self.items[item] -= quantity
class PaymentProcessor:
def process_payment(self, amount):
# Simulate payment processing
return True
class OrderManager:
def __init__(self, inventory, payment_processor):
self.inventory = inventory
self.payment_processor = payment_processor
def place_order(self, item, quantity, payment_amount):
if not self.inventory.check_availability(item, quantity):
return "Item not available in the required quantity"
if not self.payment_processor.process_payment(payment_amount):
return "Payment failed"
self.inventory.update_stock(item, quantity)
return "Order placed successfully"
Now, let’s write integration tests for this system:
import pytest
from order_system import Inventory, PaymentProcessor, OrderManager
@pytest.fixture
def order_system():
inventory = Inventory()
payment_processor = PaymentProcessor()
order_manager = OrderManager(inventory, payment_processor)
return order_manager
def test_successful_order(order_system):
result = order_system.place_order("apple", 2, 10)
assert result == "Order placed successfully"
assert order_system.inventory.items["apple"] == 8
def test_insufficient_stock(order_system):
result = order_system.place_order("banana", 10, 20)
assert result == "Item not available in the required quantity"
assert order_system.inventory.items["banana"] == 5
def test_invalid_item(order_system):
result = order_system.place_order("grape", 1, 5)
assert result == "Item not available in the required quantity"
def test_multiple_orders(order_system):
result1 = order_system.place_order("apple", 3, 15)
result2 = order_system.place_order("orange", 2, 10)
assert result1 == "Order placed successfully"
assert result2 == "Order placed successfully"
assert order_system.inventory.items["apple"] == 7
assert order_system.inventory.items["orange"] == 5
In this example, we’re testing the integration of the Inventory, PaymentProcessor, and OrderManager classes. The tests verify that the components work together correctly for various scenarios, including successful orders, insufficient stock, invalid items, and multiple orders.
Best Practices for Integration Testing
To ensure effective integration testing, consider the following best practices:
- Plan integration tests early: Consider integration scenarios during the design phase of your project.
- Use a staging environment: Perform integration tests in an environment that closely resembles production.
- Start with critical paths: Focus on testing the most important integrations and workflows first.
- Use realistic data: Employ data that represents real-world scenarios to make tests more effective.
- Automate when possible: Automate integration tests to enable frequent execution and faster feedback.
- Monitor and log: Implement proper logging and monitoring to help diagnose integration issues.
- Consider different integration patterns: Choose the appropriate integration testing approach based on your project’s needs.
- Test error handling: Verify that the integrated system handles errors and edge cases gracefully.
- Maintain test data independence: Ensure that tests don’t interfere with each other’s data.
- Regularly review and update tests: Keep integration tests up-to-date as the system evolves.
Tools for Unit and Integration Testing
There are numerous tools available for both unit testing and integration testing across different programming languages and frameworks. Here are some popular options:
Unit Testing Tools:
- Python: unittest, pytest, nose
- Java: JUnit, TestNG
- JavaScript: Jest, Mocha, Jasmine
- C#: NUnit, xUnit.net, MSTest
- Ruby: RSpec, Minitest
Integration Testing Tools:
- Selenium: For web application testing
- Postman: API testing tool
- SoapUI: For testing SOAP and REST APIs
- Jenkins: Continuous integration server that can run integration tests
- Docker: Containerization tool useful for creating consistent test environments
Combining Unit and Integration Testing
While unit testing and integration testing serve different purposes, they are complementary and should be used together for comprehensive test coverage. Here’s how you can effectively combine these testing strategies:
- Start with unit tests: Begin by writing and running unit tests for individual components. This helps catch basic errors and ensures that each unit functions correctly in isolation.
- Move to integration tests: Once unit tests pass, proceed to integration tests to verify that components work together as expected.
- Use the testing pyramid: Follow the testing pyramid principle, where you have many unit tests, fewer integration tests, and even fewer end-to-end tests.
- Automate both levels: Implement continuous integration to automatically run both unit and integration tests whenever code changes are pushed.
- Maintain a balance: Ensure that you have adequate coverage at both the unit and integration levels without unnecessary overlap.
- Use mocking judiciously: While mocks are essential for unit testing, be cautious about overusing them in integration tests, as they may hide real integration issues.
Common Challenges and Solutions
When implementing unit and integration testing, you may encounter several challenges. Here are some common issues and their solutions:
1. Time-consuming test execution
Solution: Optimize test execution by parallelizing tests, using faster testing frameworks, and employing test doubles for external dependencies.
2. Flaky tests
Solution: Identify and fix the root causes of flakiness, such as race conditions, external dependencies, or improper test isolation.
3. Maintaining test code
Solution: Treat test code with the same care as production code. Refactor tests, use descriptive names, and keep them up-to-date with code changes.
4. Dealing with legacy code
Solution: Gradually introduce tests for legacy code by focusing on critical paths and using techniques like the “Strangler Fig” pattern for refactoring.
5. Overreliance on mocks
Solution: Use mocks judiciously, focusing on external dependencies. Consider using real objects for internal components to catch integration issues early.
6. Test data management
Solution: Implement proper test data management strategies, such as using fixtures, factories, or database seeding to ensure consistent and isolated test environments.
Conclusion
Unit testing and integration testing are essential practices in modern software development. They help ensure code quality, catch bugs early, and provide confidence in the reliability of your software. By understanding the principles, implementing best practices, and using appropriate tools, you can create a robust testing strategy that combines both unit and integration testing.
Remember that testing is an ongoing process. As your codebase evolves, so should your tests. Regularly review and update your testing approach to keep pace with your project’s needs and the latest industry best practices.
By mastering unit and integration testing, you’ll be well-equipped to build high-quality, maintainable software that meets the demands of today’s fast-paced development environment. Happy testing!