How to Pass your Coding Interview, a step by step guide
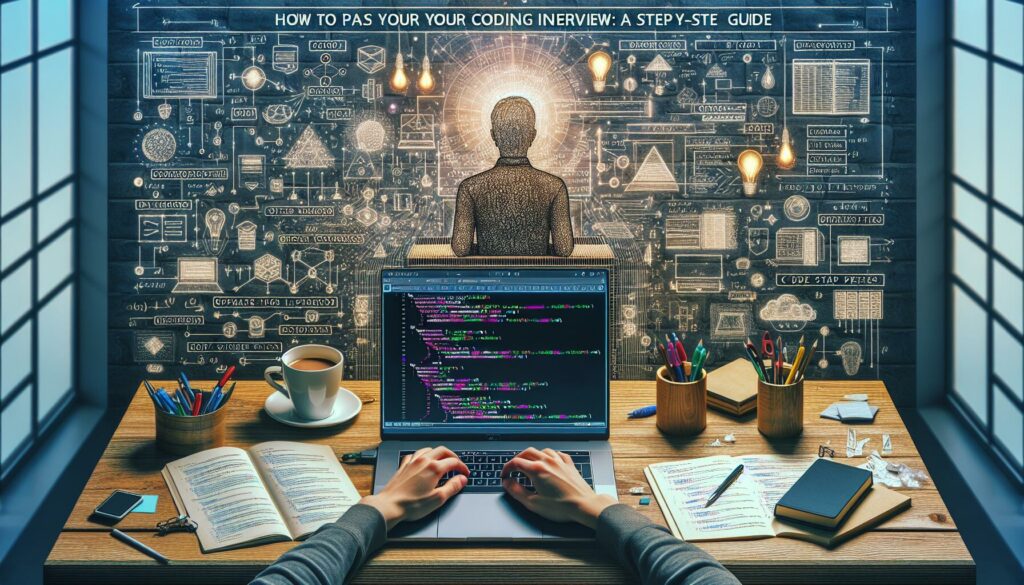
Communication is key to passing your FAANG interview.
Involve the interviewer as if they are your coworker.
Before you code, read the problem statement out loud.
If you’re unsure how the input leads to the output, you don’t understand the problem. Ask the interviewer for clarification.
Give an input test case and expected output.
Ask the interviewer if it’s correct. If it is, you won’t misinterpret the question.
Solving the right problem is essential!
Talk about the algorithm you’re going to use.
Mention out loud what you’re thinking.
Clarify if it’s a brute force method or why it’s optimal or not in terms of space and time complexity.
Ask your interviewer if they want you to code that solution.
They’ll let you know if they want a better approach.
While you’re coding, explain what each line of code is doing.
Mention what part of the algorithm you’re currently addressing.
If your algorithm needs binary search, reference the part of your algorithm you’re at as you’re coding that portion.
After completing a portion of the code, confirm with the interviewer if they understand what you wrote so far.
If they don’t confirm or say anything, dry run your code with a sample test case.
If there’s a mistake, you’ll catch it here.
If they don’t understand what you wrote, you likely wrote overly complicated code.
Talk about optimizations and bottlenecks as you go.
There was already agreement on the algorithm you would write, so you don’t need to optimize on the spot.
Mention as you’re coding if any portion is suboptimal, and let them know you’ll come back to it afterward.
You don’t have to code the perfect solution on the first iteration.
Start your solution with the heavy part of the algorithm.
Point out what you’re going to code later — for instance, an isCellValid function.
It’s not over when you finish writing the algorithm.
We don’t know if it works or not with the test cases we wrote.
We don’t know what the interviewer wants you to do now.
So ask instead of assuming what you should be doing.
Ask the interviewer if they prefer you to dry run your code against the example inputs.
Dry run your code against the example inputs.
Go through your code and reference again what portion of the algorithm your code is covering line by line.
Yes, line by line.
As you’re going through, talk about the state of the output as you go along.
Exactly how a debugger shows you the state of all variables.
And you iterate line by line, watching the variables.
If anything is unexpected, mention you’re going to fix it and work on it.
The rest of the code is invalid if you’ve made a mistake, and you don’t need to discuss it further.
Repeat until you’ve fixed all your bugs.
Do this for all inputs given.
Or even better, try out some edge cases as well.
Optimize your code further.
If there are bottlenecks in your code, point them out.
Describe why the bottleneck is there and what you’re doing to optimize it.
Talk aloud about what you’re thinking and why specific approaches do or don’t work.
When you come up with an improved solution, ask the interviewer if they’re happy with that approach.
They’ll let you know if they want you to implement it or not.
At this point, they’ll probably ask about space and running time analysis.
The mistake everyone makes when asked about time and space complexities is just stating it.
Don’t answer without showing your work.
Iterate over the major components of your algorithm and compute and explain the time complexity.
You can write a comment stating the time complexity for each significant chunk of code.
Add those together and formulate the final time complexity.
As for space, track your data structures, and figure out the total and extra space used.