How to Overcome Analysis Paralysis When Starting a Coding Problem
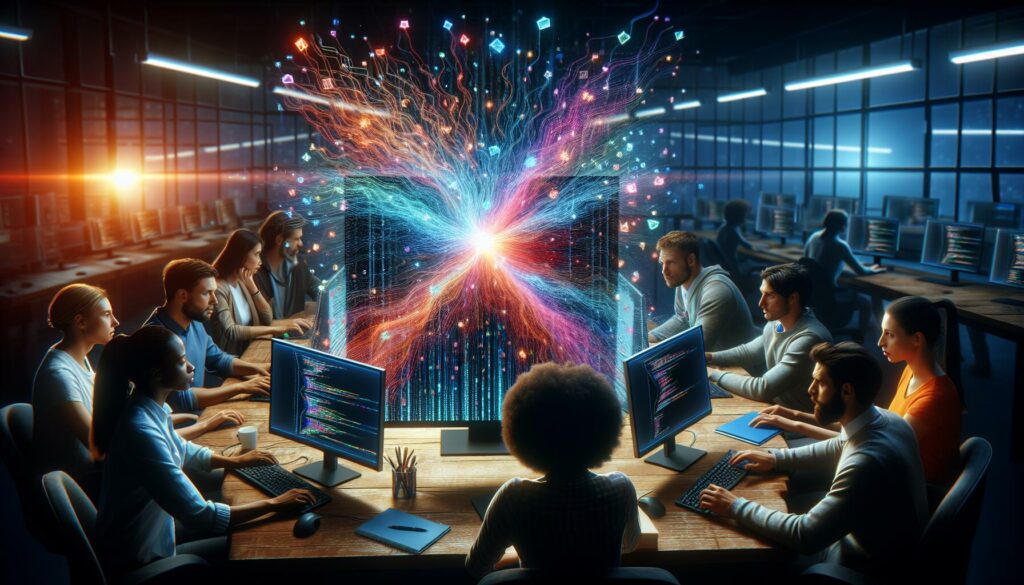
As aspiring programmers and seasoned developers alike, we’ve all encountered that daunting moment when faced with a new coding problem. The cursor blinks mockingly on an empty screen, and our minds race with possibilities, potential solutions, and sometimes, overwhelming doubt. This phenomenon, known as “analysis paralysis,” can be a significant roadblock in our coding journey. But fear not! In this comprehensive guide, we’ll explore effective strategies to overcome this common challenge and jumpstart your problem-solving process.
Understanding Analysis Paralysis in Coding
Before diving into solutions, let’s first understand what analysis paralysis is and why it occurs, especially in the context of coding.
What is Analysis Paralysis?
Analysis paralysis is a state of over-analyzing or over-thinking a situation to the point where a decision or action becomes impossible. In coding, this often manifests as an inability to start writing code due to excessive contemplation of various approaches, fear of choosing the wrong solution, or feeling overwhelmed by the complexity of the problem.
Why Does It Happen in Coding?
Several factors contribute to analysis paralysis in programming:
- The open-ended nature of many coding problems
- The desire to find the “perfect” solution from the start
- Fear of making mistakes or writing inefficient code
- Lack of confidence in one’s abilities
- Overwhelming complexity of certain problems
Recognizing these factors is the first step in overcoming them. Now, let’s explore practical strategies to break through this mental barrier and start coding with confidence.
1. Break Down the Problem
One of the most effective ways to combat analysis paralysis is to break the problem down into smaller, manageable pieces. This approach, often called “divide and conquer,” can make even the most complex problems less intimidating.
Steps to Break Down a Problem:
- Identify the main components or steps of the problem
- Break each component into smaller, actionable tasks
- Prioritize these tasks based on dependencies or complexity
- Focus on solving one small task at a time
For example, if you’re tasked with creating a web scraper, you might break it down like this:
- Set up the development environment
- Choose and install necessary libraries
- Write code to send HTTP requests
- Parse the HTML response
- Extract desired data
- Store or display the results
By focusing on one step at a time, you’ll find it easier to start and maintain momentum throughout the project.
2. Start with Pseudocode
Pseudocode is a plain language description of the steps in an algorithm. It’s an excellent tool for overcoming analysis paralysis because it allows you to outline your approach without getting bogged down in syntax details.
Benefits of Using Pseudocode:
- Helps clarify your thinking
- Provides a roadmap for your actual code
- Allows you to focus on logic without syntax concerns
- Makes it easier to spot potential issues early
Here’s an example of how you might write pseudocode for a simple sorting algorithm:
function sort_array(array):
for each element in array:
compare with next element
if current element > next element:
swap elements
repeat until no swaps are needed
return sorted array
Once you have your pseudocode, translating it into actual code becomes much more straightforward.
3. Embrace the “Just Start” Mentality
Sometimes, the best way to overcome analysis paralysis is to simply start coding, even if you’re not sure it’s the best approach. This “just start” mentality can help you gain momentum and overcome the initial mental hurdle.
Tips for Embracing the “Just Start” Mentality:
- Set a timer for 5-10 minutes and commit to writing code during that time
- Write a simple function or class related to the problem, even if it’s not perfect
- Focus on getting a basic version working before optimizing
- Remember that you can always refactor or improve your code later
The key is to build momentum. Once you’ve started, you’ll often find that ideas flow more easily, and the path forward becomes clearer.
4. Use the Rubber Duck Debugging Technique
Rubber duck debugging is a method of debugging code by explaining it, line-by-line, to an inanimate object (traditionally, a rubber duck). This technique can be adapted to help overcome analysis paralysis by forcing you to articulate your thoughts and approach.
How to Apply Rubber Duck Debugging to Problem-Solving:
- Choose an object (it doesn’t have to be a rubber duck)
- Explain the problem to the object as if it were a person
- Walk through your proposed solution step-by-step
- As you explain, you may naturally identify gaps or issues in your thinking
This process can help clarify your thoughts and often leads to “aha!” moments that break through the paralysis.
5. Time-Box Your Research
While research is crucial for solving coding problems, it can also be a trap that leads to analysis paralysis. To avoid this, set a specific time limit for your initial research phase.
Steps for Time-Boxing Research:
- Set a timer for a fixed duration (e.g., 15-30 minutes)
- During this time, gather relevant information about the problem and potential solutions
- When the timer goes off, stop researching and start coding
- If you need more information later, you can always do focused research on specific issues
This approach ensures that you have enough context to start without getting lost in an endless cycle of research.
6. Implement a Minimal Viable Solution
Instead of aiming for the perfect solution right away, focus on creating a Minimal Viable Solution (MVS). This is the simplest version of your code that solves the core problem, even if it’s not optimized or doesn’t handle all edge cases.
Benefits of the MVS Approach:
- Provides a working foundation to build upon
- Helps identify potential issues early
- Boosts confidence by achieving a quick win
- Makes it easier to iterate and improve
Once you have your MVS, you can gradually refine and optimize it, adding features and handling edge cases as needed.
7. Utilize Test-Driven Development (TDD)
Test-Driven Development is a software development approach where you write tests before writing the actual code. This method can be particularly helpful in overcoming analysis paralysis because it provides a clear starting point and direction.
Steps in TDD:
- Write a failing test that defines a desired improvement or new function
- Write the minimum amount of code to pass the test
- Refactor the code to meet acceptable standards
Here’s a simple example of TDD in Python:
import unittest
class TestStringMethods(unittest.TestCase):
def test_upper(self):
self.assertEqual('foo'.upper(), 'FOO')
if __name__ == '__main__':
unittest.main()
By starting with tests, you create a roadmap for your code and can focus on implementing one small piece at a time.
8. Leverage Pair Programming or Code Reviews
Sometimes, the best way to overcome analysis paralysis is to involve another person. Pair programming or early code reviews can provide fresh perspectives and help you move past mental blocks.
Benefits of Collaboration:
- Immediate feedback on ideas
- Diverse problem-solving approaches
- Accountability and motivation
- Knowledge sharing and learning opportunities
If you don’t have a coding partner readily available, consider joining online coding communities or forums where you can discuss your approach and get feedback.
9. Use Visualization Techniques
Visual representations of your problem or solution can often help clarify your thoughts and provide a new perspective. This can be particularly useful for complex algorithms or data structures.
Visualization Methods:
- Flowcharts
- Mind maps
- Diagrams
- Sketches
For example, if you’re working on a sorting algorithm, drawing out how the array changes with each step can help you understand and implement the algorithm more effectively.
10. Practice Regular Problem-Solving
Like any skill, overcoming analysis paralysis gets easier with practice. Regular engagement with coding problems can help build your confidence and problem-solving abilities.
Ways to Practice:
- Solve daily coding challenges on platforms like LeetCode or HackerRank
- Participate in coding competitions
- Work on personal projects
- Contribute to open-source projects
The more you practice, the more comfortable you’ll become with tackling new and unfamiliar problems.
Conclusion
Analysis paralysis can be a significant obstacle in coding, but it’s not insurmountable. By employing strategies like breaking down problems, using pseudocode, embracing a “just start” mentality, and leveraging techniques like TDD and pair programming, you can overcome this challenge and become a more confident, effective programmer.
Remember, the goal is progress, not perfection. Every line of code you write is a step forward, even if it’s not the final solution. Embrace the iterative nature of coding, and don’t let the fear of imperfection hold you back from starting.
As you apply these strategies and gain more experience, you’ll find that analysis paralysis becomes less of an issue. You’ll develop the ability to quickly assess problems, formulate approaches, and dive into coding with confidence. So the next time you’re faced with a blank screen and a complex problem, take a deep breath, choose a strategy, and start coding. Your future self will thank you for taking that first step.
Happy coding, and may your keystrokes be swift and your solutions elegant!