How to Organize Your Codebase for Scalability and Readability
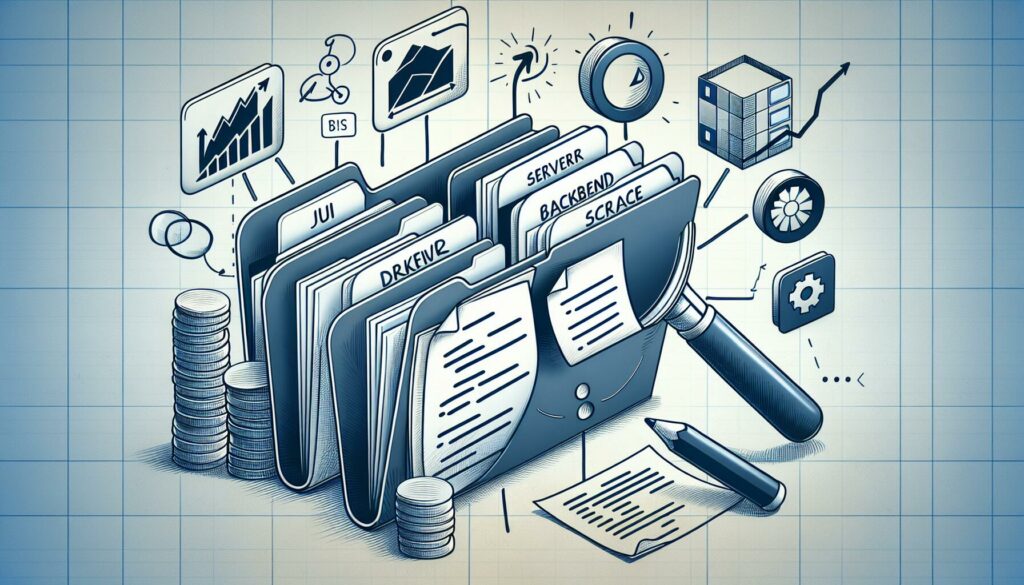
In the world of software development, writing code that works is just the beginning. As projects grow and evolve, the organization of your codebase becomes crucial for maintaining scalability and readability. A well-structured codebase not only makes it easier for you to navigate and update your own code but also facilitates collaboration with other developers. In this comprehensive guide, we’ll explore best practices for organizing your codebase, focusing on folder structure, naming conventions, and modular coding practices that ensure a clean and scalable architecture.
1. The Importance of Code Organization
Before diving into specific techniques, let’s understand why code organization is so critical:
- Maintainability: Well-organized code is easier to maintain and update over time.
- Scalability: A good structure allows your project to grow without becoming unwieldy.
- Collaboration: Clear organization helps team members understand and contribute to the codebase more effectively.
- Debugging: When issues arise, a well-structured codebase makes it easier to locate and fix problems.
- Reusability: Properly organized code promotes the reuse of components and functions across the project.
2. Establishing a Clear Folder Structure
A logical folder structure is the foundation of a well-organized codebase. Here’s a general approach that works for many projects:
project-root/
├── src/
│ ├── components/
│ ├── utils/
│ ├── services/
│ ├── styles/
│ └── pages/
├── tests/
├── docs/
├── config/
├── scripts/
└── public/
Let’s break down each directory:
- src/: Contains the main source code of your application.
- components/: Reusable UI components (for front-end projects).
- utils/: Helper functions and utility classes.
- services/: API calls and business logic.
- styles/: CSS or styling-related files.
- pages/: Page-specific components or routes.
- tests/: Unit tests, integration tests, and other test files.
- docs/: Documentation files.
- config/: Configuration files for different environments.
- scripts/: Build scripts, deployment scripts, etc.
- public/: Static assets like images, fonts, etc.
This structure separates concerns and makes it easy to locate specific parts of your application. As your project grows, you can add subdirectories within these main folders to further organize your code.
3. Naming Conventions
Consistent naming conventions are crucial for readability. Here are some guidelines:
3.1 File Naming
- Use lowercase for folder names:
components/
,utils/
- Use PascalCase for component files:
UserProfile.js
,Button.jsx
- Use camelCase for utility and service files:
apiService.js
,formatDate.js
- Use kebab-case for CSS files:
button-styles.css
3.2 Variable and Function Naming
- Use camelCase for variables and function names:
userName
,calculateTotal()
- Use PascalCase for class names:
class UserProfile {}
- Use UPPER_SNAKE_CASE for constants:
MAX_ITEMS
,API_BASE_URL
3.3 Descriptive Names
Choose names that clearly describe the purpose or functionality:
// Bad
const x = 5;
function doStuff() {}
// Good
const maxRetryAttempts = 5;
function validateUserInput() {}
4. Modular Coding Practices
Modular coding is key to creating a scalable and maintainable codebase. Here are some practices to follow:
4.1 Separating Concerns
Divide your code into modules that each handle a specific functionality. This makes your code easier to understand, test, and maintain.
// userService.js
export function fetchUser(id) {
// API call to fetch user
}
// userProfile.js
import { fetchUser } from './userService';
function UserProfile({ userId }) {
const user = fetchUser(userId);
// Render user profile
}
4.2 Using Classes for Complex Objects
When dealing with complex objects that have both data and behavior, consider using classes:
class ShoppingCart {
constructor() {
this.items = [];
}
addItem(item) {
this.items.push(item);
}
calculateTotal() {
return this.items.reduce((total, item) => total + item.price, 0);
}
}
4.3 Abstracting Logic into Functions
Break down complex operations into smaller, reusable functions:
function calculateTotalPrice(items) {
return items.reduce((total, item) => total + item.price, 0);
}
function applyDiscount(total, discountPercentage) {
return total * (1 - discountPercentage / 100);
}
function calculateFinalPrice(items, discountPercentage) {
const total = calculateTotalPrice(items);
return applyDiscount(total, discountPercentage);
}
5. Implementing Design Patterns
Design patterns are reusable solutions to common problems in software design. Incorporating them can significantly improve your code’s structure and scalability. Here are a few popular patterns:
5.1 Singleton Pattern
Use this pattern when you want to ensure that a class has only one instance throughout the application:
class DatabaseConnection {
constructor() {
if (DatabaseConnection.instance) {
return DatabaseConnection.instance;
}
DatabaseConnection.instance = this;
this.connection = null;
}
connect() {
if (!this.connection) {
// Establish database connection
this.connection = /* connection logic */;
}
return this.connection;
}
}
const db = new DatabaseConnection();
export default db;
5.2 Factory Pattern
This pattern is useful for creating objects without specifying the exact class of object that will be created:
class Car {
constructor(make, model) {
this.make = make;
this.model = model;
}
}
class Truck {
constructor(make, model, payload) {
this.make = make;
this.model = model;
this.payload = payload;
}
}
class VehicleFactory {
createVehicle(type, make, model, payload) {
if (type === 'car') {
return new Car(make, model);
} else if (type === 'truck') {
return new Truck(make, model, payload);
}
}
}
const factory = new VehicleFactory();
const myCar = factory.createVehicle('car', 'Toyota', 'Corolla');
const myTruck = factory.createVehicle('truck', 'Ford', 'F-150', 2000);
5.3 Observer Pattern
This pattern is great for implementing event handling systems:
class Subject {
constructor() {
this.observers = [];
}
addObserver(observer) {
this.observers.push(observer);
}
removeObserver(observer) {
const index = this.observers.indexOf(observer);
if (index > -1) {
this.observers.splice(index, 1);
}
}
notifyObservers(data) {
this.observers.forEach(observer => observer.update(data));
}
}
class Observer {
update(data) {
console.log('Received update:', data);
}
}
const subject = new Subject();
const observer1 = new Observer();
const observer2 = new Observer();
subject.addObserver(observer1);
subject.addObserver(observer2);
subject.notifyObservers('Hello, observers!');
6. Code Documentation
Well-documented code is crucial for maintainability and collaboration. Here are some best practices:
6.1 Inline Comments
Use inline comments to explain complex logic or non-obvious decisions:
function calculateCompoundInterest(principal, rate, time, n) {
// A = P(1 + r/n)^(nt)
// Where: A = final amount, P = principal balance, r = interest rate,
// t = time in years, n = number of times interest is compounded per year
return principal * Math.pow((1 + (rate / n)), (n * time));
}
6.2 JSDoc Comments
For functions and classes, use JSDoc comments to describe parameters, return values, and overall functionality:
/**
* Calculates the compound interest.
* @param {number} principal - The initial investment amount.
* @param {number} rate - The annual interest rate (as a decimal).
* @param {number} time - The time period in years.
* @param {number} n - The number of times interest is compounded per year.
* @returns {number} The final amount after applying compound interest.
*/
function calculateCompoundInterest(principal, rate, time, n) {
// Function implementation...
}
6.3 README Files
Include a README.md file in your project root and important subdirectories. The main README should include:
- Project overview
- Installation instructions
- Usage examples
- Contributing guidelines
- License information
7. Version Control Best Practices
Effective use of version control is crucial for maintaining a clean and organized codebase:
7.1 Branching Strategy
Implement a clear branching strategy, such as GitFlow or GitHub Flow. For example:
main
branch for production-ready codedevelop
branch for ongoing development- Feature branches for new features
- Hotfix branches for critical bug fixes
7.2 Commit Messages
Write clear, concise commit messages that describe the changes made:
feat: add user authentication feature
fix: resolve race condition in data fetching
docs: update API documentation
refactor: improve performance of search algorithm
7.3 Pull Requests
Use pull requests for code reviews before merging changes into main branches. Include:
- A clear description of the changes
- Any related issue numbers
- Steps to test the changes
8. Testing Strategies
Implementing a robust testing strategy is essential for maintaining code quality and preventing regressions:
8.1 Unit Testing
Write unit tests for individual functions and components:
// calculateTotal.js
export function calculateTotal(items) {
return items.reduce((total, item) => total + item.price, 0);
}
// calculateTotal.test.js
import { calculateTotal } from './calculateTotal';
test('calculateTotal returns correct sum', () => {
const items = [
{ name: 'Item 1', price: 10 },
{ name: 'Item 2', price: 20 },
{ name: 'Item 3', price: 30 }
];
expect(calculateTotal(items)).toBe(60);
});
8.2 Integration Testing
Test how different parts of your application work together:
// integration.test.js
import { createUser, getUserProfile } from './userService';
test('create user and fetch profile', async () => {
const userId = await createUser({ name: 'John Doe', email: 'john@example.com' });
const profile = await getUserProfile(userId);
expect(profile.name).toBe('John Doe');
expect(profile.email).toBe('john@example.com');
});
8.3 End-to-End Testing
Use tools like Cypress or Selenium to test your application from a user’s perspective:
// cypress/integration/login.spec.js
describe('Login Flow', () => {
it('successfully logs in a user', () => {
cy.visit('/login');
cy.get('input[name="username"]').type('testuser');
cy.get('input[name="password"]').type('password123');
cy.get('button[type="submit"]').click();
cy.url().should('include', '/dashboard');
cy.contains('Welcome, Test User').should('be.visible');
});
});
9. Continuous Integration and Deployment (CI/CD)
Implementing CI/CD pipelines helps maintain code quality and streamline the deployment process:
9.1 Continuous Integration
Set up a CI system (e.g., Jenkins, Travis CI, GitHub Actions) to automatically run tests and lint your code on each push:
// .github/workflows/ci.yml
name: Continuous Integration
on: [push, pull_request]
jobs:
test:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Use Node.js
uses: actions/setup-node@v2
with:
node-version: '14'
- run: npm ci
- run: npm run lint
- run: npm test
9.2 Continuous Deployment
Automate your deployment process to reduce human error and speed up releases:
// .github/workflows/deploy.yml
name: Deploy to Production
on:
push:
branches: [main]
jobs:
deploy:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Deploy to server
uses: appleboy/ssh-action@master
with:
host: ${{ secrets.HOST }}
username: ${{ secrets.USERNAME }}
key: ${{ secrets.SSH_KEY }}
script: |
cd /path/to/project
git pull origin main
npm install
npm run build
pm2 restart app
10. Performance Optimization
As your codebase grows, performance optimization becomes increasingly important:
10.1 Code Splitting
Use code splitting to load only the necessary code for each page or component:
// React example with React.lazy and Suspense
import React, { Suspense, lazy } from 'react';
const Home = lazy(() => import('./pages/Home'));
const About = lazy(() => import('./pages/About'));
function App() {
return (
<Suspense fallback={<div>Loading...</div>}>
<Switch>
<Route exact path="/" component={Home} />
<Route path="/about" component={About} />
</Switch>
</Suspense>
);
}
10.2 Memoization
Use memoization techniques to cache expensive computations:
import { useMemo } from 'react';
function ExpensiveComponent({ data }) {
const processedData = useMemo(() => {
// Expensive computation here
return data.map(item => /* complex transformation */);
}, [data]);
return <div>{/* Render processed data */}</div>;
}
10.3 Lazy Loading
Implement lazy loading for images and other resources:
<img src="placeholder.jpg" data-src="actual-image.jpg" class="lazy" />
// JavaScript to load images as they enter the viewport
document.addEventListener("DOMContentLoaded", function() {
var lazyImages = [].slice.call(document.querySelectorAll("img.lazy"));
if ("IntersectionObserver" in window) {
let lazyImageObserver = new IntersectionObserver(function(entries, observer) {
entries.forEach(function(entry) {
if (entry.isIntersecting) {
let lazyImage = entry.target;
lazyImage.src = lazyImage.dataset.src;
lazyImage.classList.remove("lazy");
lazyImageObserver.unobserve(lazyImage);
}
});
});
lazyImages.forEach(function(lazyImage) {
lazyImageObserver.observe(lazyImage);
});
}
});
Conclusion
Organizing your codebase for scalability and readability is an ongoing process that requires consistent effort and attention to detail. By implementing clear folder structures, following naming conventions, using modular coding practices, and adopting design patterns, you can create a codebase that is not only easier to maintain but also more scalable as your project grows.
Remember that the best practices outlined in this guide are not one-size-fits-all solutions. Always consider the specific needs of your project and team when deciding how to structure your code. Regular code reviews, refactoring sessions, and open communication within your development team can help ensure that your codebase remains clean, efficient, and scalable over time.
As you continue to develop your coding skills, keep in mind that organizing your code effectively is just as important as writing the code itself. It’s a skill that will serve you well throughout your career, whether you’re working on personal projects, contributing to open-source initiatives, or developing enterprise-level applications.
By mastering these techniques and continuously refining your approach to code organization, you’ll be well-equipped to tackle complex projects and collaborate effectively with other developers. Happy coding!