How to Optimize Your Space Complexity: A Comprehensive Guide
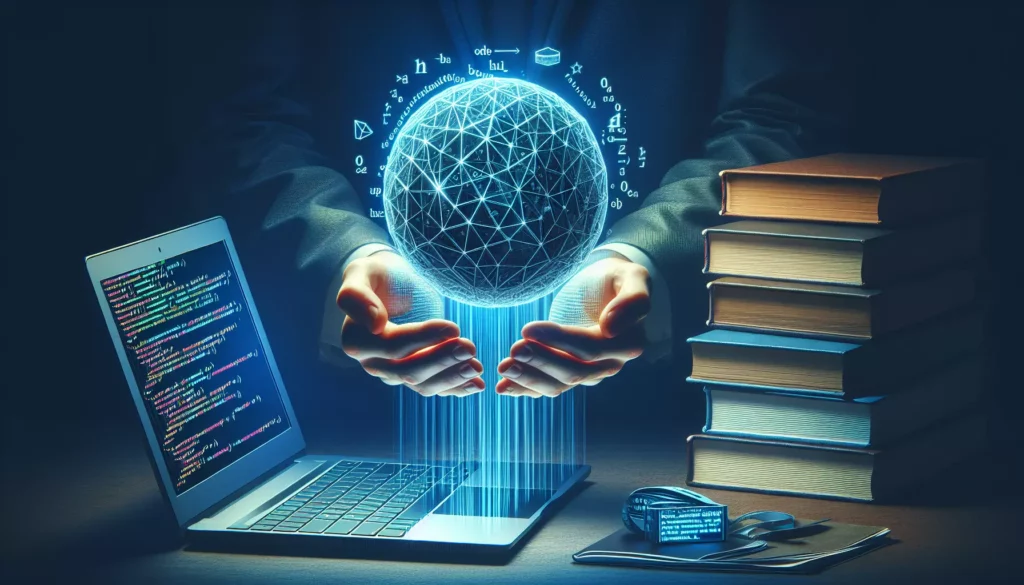
In the world of programming and algorithm design, efficiency is key. While we often focus on optimizing time complexity to make our programs run faster, it’s equally important to consider space complexity. Space complexity refers to the amount of memory an algorithm or program uses in relation to its input size. In this comprehensive guide, we’ll explore various techniques and strategies to optimize your space complexity, helping you write more efficient and scalable code.
Table of Contents
- Understanding Space Complexity
- The Importance of Optimizing Space Complexity
- Common Space Complexity Notations
- Techniques for Optimizing Space Complexity
- Choosing the Right Data Structures
- Algorithmic Approaches for Space Optimization
- Time-Space Trade-offs
- Real-world Examples and Case Studies
- Best Practices for Space Optimization
- Conclusion
1. Understanding Space Complexity
Before diving into optimization techniques, it’s crucial to understand what space complexity is and how it’s measured. Space complexity is a measure of the amount of working storage an algorithm needs as a function of the size of the input data. It includes both the space used by the input data and any additional space the algorithm requires during its execution.
Space complexity is typically expressed using Big O notation, similar to time complexity. For example, an algorithm with O(n) space complexity means its space usage grows linearly with the input size, while O(1) space complexity indicates constant space usage regardless of input size.
2. The Importance of Optimizing Space Complexity
Optimizing space complexity is crucial for several reasons:
- Memory Limitations: Devices have finite memory, and efficient space usage allows your programs to handle larger inputs and datasets.
- Scalability: As your application grows, efficient space usage becomes increasingly important to maintain performance.
- Cost Efficiency: In cloud computing environments, memory usage often directly translates to costs.
- Improved Performance: Reducing memory usage can lead to better cache utilization and fewer page faults, indirectly improving time complexity.
3. Common Space Complexity Notations
Let’s review some common space complexity notations:
- O(1) – Constant Space: The algorithm uses a fixed amount of memory regardless of input size.
- O(n) – Linear Space: Memory usage grows linearly with the input size.
- O(log n) – Logarithmic Space: Memory usage grows logarithmically with the input size.
- O(n^2) – Quadratic Space: Memory usage grows quadratically with the input size.
- O(2^n) – Exponential Space: Memory usage doubles with each increase in input size.
4. Techniques for Optimizing Space Complexity
Now, let’s explore various techniques to optimize space complexity in your algorithms and programs:
4.1. In-place Algorithms
In-place algorithms modify the input data structure directly without using additional data structures. This approach can significantly reduce space complexity.
Example: In-place array reversal
def reverse_array(arr):
left, right = 0, len(arr) - 1
while left < right:
arr[left], arr[right] = arr[right], arr[left]
left += 1
right -= 1
return arr
# Usage
arr = [1, 2, 3, 4, 5]
reversed_arr = reverse_array(arr)
print(reversed_arr) # Output: [5, 4, 3, 2, 1]
This algorithm reverses the array in-place with O(1) space complexity.
4.2. Reusing Variables
Instead of creating new variables for each operation, reuse existing variables when possible.
Example: Calculating the sum of an array
def sum_array(arr):
total = 0
for num in arr:
total += num
return total
# Usage
arr = [1, 2, 3, 4, 5]
result = sum_array(arr)
print(result) # Output: 15
This function uses a single variable ‘total’ to accumulate the sum, maintaining O(1) space complexity.
4.3. Using Generators
Generators in Python allow you to iterate over a sequence of values without storing the entire sequence in memory.
Example: Generating Fibonacci numbers
def fibonacci_generator(n):
a, b = 0, 1
for _ in range(n):
yield a
a, b = b, a + b
# Usage
for num in fibonacci_generator(10):
print(num, end=' ')
# Output: 0 1 1 2 3 5 8 13 21 34
This generator produces Fibonacci numbers on-the-fly, using constant space O(1) regardless of how many numbers are generated.
4.4. Bit Manipulation
Using bit manipulation techniques can help reduce space usage, especially when dealing with boolean flags or sets of small integers.
Example: Checking if a number is a power of 2
def is_power_of_two(n):
return n > 0 and (n & (n - 1)) == 0
# Usage
print(is_power_of_two(16)) # Output: True
print(is_power_of_two(18)) # Output: False
This function uses bitwise operations to check if a number is a power of 2, using constant space O(1).
5. Choosing the Right Data Structures
The choice of data structure can significantly impact space complexity. Let’s compare some common data structures and their space efficiency:
5.1. Arrays vs. Linked Lists
Arrays offer constant-time access but may waste space with pre-allocation. Linked lists use space proportional to the number of elements but require extra space for pointers.
5.2. Hash Tables
Hash tables provide fast lookups but can consume more space due to potential collisions and load factor considerations.
5.3. Tries
Tries are efficient for storing strings with common prefixes, potentially saving space compared to storing each string separately.
5.4. Bloom Filters
Bloom filters provide space-efficient set membership testing at the cost of allowing false positives.
Example: Implementing a simple Bloom filter
import mmh3
class BloomFilter:
def __init__(self, size, hash_count):
self.size = size
self.hash_count = hash_count
self.bit_array = [0] * size
def add(self, item):
for i in range(self.hash_count):
index = mmh3.hash(item, i) % self.size
self.bit_array[index] = 1
def contains(self, item):
for i in range(self.hash_count):
index = mmh3.hash(item, i) % self.size
if self.bit_array[index] == 0:
return False
return True
# Usage
bf = BloomFilter(20, 3)
bf.add("apple")
bf.add("banana")
print(bf.contains("apple")) # Output: True
print(bf.contains("orange")) # Output: False (or True, false positive)
This Bloom filter implementation provides space-efficient set membership testing, using only a fixed-size bit array.
6. Algorithmic Approaches for Space Optimization
Certain algorithmic approaches can help reduce space complexity:
6.1. Two-Pointer Technique
Using two pointers to traverse data structures can often reduce the need for additional space.
Example: Finding the middle of a linked list
class ListNode:
def __init__(self, val=0, next=None):
self.val = val
self.next = next
def find_middle(head):
if not head:
return None
slow = fast = head
while fast and fast.next:
slow = slow.next
fast = fast.next.next
return slow
# Usage
head = ListNode(1, ListNode(2, ListNode(3, ListNode(4, ListNode(5)))))
middle = find_middle(head)
print(middle.val) # Output: 3
This algorithm finds the middle of a linked list using two pointers, maintaining O(1) space complexity.
6.2. Sliding Window
The sliding window technique can be used to process subarrays or substrings efficiently without using additional space.
Example: Finding the maximum sum subarray of size k
def max_sum_subarray(arr, k):
if len(arr) < k:
return None
window_sum = sum(arr[:k])
max_sum = window_sum
for i in range(k, len(arr)):
window_sum = window_sum - arr[i-k] + arr[i]
max_sum = max(max_sum, window_sum)
return max_sum
# Usage
arr = [1, 4, 2, 10, 23, 3, 1, 0, 20]
k = 4
result = max_sum_subarray(arr, k)
print(result) # Output: 39
This algorithm finds the maximum sum subarray of size k using a sliding window approach, maintaining O(1) space complexity.
6.3. Divide and Conquer
Divide and conquer algorithms can sometimes be implemented with reduced space complexity by using recursion carefully.
Example: Merge sort with O(1) auxiliary space
def merge_sort(arr):
def merge(start, mid, end):
left = arr[start:mid+1]
right = arr[mid+1:end+1]
i, j, k = 0, 0, start
while i < len(left) and j < len(right):
if left[i] <= right[j]:
arr[k] = left[i]
i += 1
else:
arr[k] = right[j]
j += 1
k += 1
while i < len(left):
arr[k] = left[i]
i += 1
k += 1
while j < len(right):
arr[k] = right[j]
j += 1
k += 1
def sort(start, end):
if start < end:
mid = (start + end) // 2
sort(start, mid)
sort(mid + 1, end)
merge(start, mid, end)
sort(0, len(arr) - 1)
return arr
# Usage
arr = [64, 34, 25, 12, 22, 11, 90]
sorted_arr = merge_sort(arr)
print(sorted_arr) # Output: [11, 12, 22, 25, 34, 64, 90]
This implementation of merge sort uses in-place merging to reduce the auxiliary space complexity to O(n), which is an improvement over the typical O(n log n) space complexity of merge sort.
7. Time-Space Trade-offs
Often, there’s a trade-off between time and space complexity. Sometimes, using more space can significantly improve time complexity, and vice versa. It’s important to consider the specific requirements of your application when deciding on these trade-offs.
7.1. Memoization
Memoization is a technique that trades space for time by storing the results of expensive function calls and returning the cached result when the same inputs occur again.
Example: Fibonacci sequence with memoization
def fibonacci(n, memo={}):
if n in memo:
return memo[n]
if n <= 1:
return n
memo[n] = fibonacci(n-1, memo) + fibonacci(n-2, memo)
return memo[n]
# Usage
print(fibonacci(100)) # Output: 354224848179261915075
This implementation uses memoization to reduce time complexity from O(2^n) to O(n), but increases space complexity to O(n).
7.2. Dynamic Programming
Dynamic programming often involves using additional space to store intermediate results, trading space for improved time complexity.
Example: 0/1 Knapsack problem
def knapsack(values, weights, capacity):
n = len(values)
dp = [[0 for _ in range(capacity + 1)] for _ in range(n + 1)]
for i in range(1, n + 1):
for w in range(1, capacity + 1):
if weights[i-1] <= w:
dp[i][w] = max(values[i-1] + dp[i-1][w-weights[i-1]], dp[i-1][w])
else:
dp[i][w] = dp[i-1][w]
return dp[n][capacity]
# Usage
values = [60, 100, 120]
weights = [10, 20, 30]
capacity = 50
result = knapsack(values, weights, capacity)
print(result) # Output: 220
This dynamic programming solution to the 0/1 Knapsack problem has a time complexity of O(n*W) and a space complexity of O(n*W), where n is the number of items and W is the knapsack capacity.
8. Real-world Examples and Case Studies
Let’s look at some real-world scenarios where space optimization plays a crucial role:
8.1. Database Indexing
Database systems use various data structures like B-trees and hash indexes to optimize query performance. However, these indexes consume additional space. Database administrators must balance query performance with storage requirements.
8.2. Image Processing
In image processing applications, working with large images can quickly consume available memory. Techniques like tiling (processing the image in smaller chunks) can help manage memory usage.
8.3. Web Servers
Web servers handling numerous concurrent connections must efficiently manage memory to scale effectively. Techniques like connection pooling and efficient session management are crucial for optimizing space usage.
8.4. Mobile Applications
Mobile devices have limited resources, making space optimization critical. Efficient data structures, caching strategies, and resource management are essential for creating responsive mobile applications.
9. Best Practices for Space Optimization
To consistently write space-efficient code, consider the following best practices:
- Profile Your Code: Use profiling tools to identify memory bottlenecks in your applications.
- Choose Appropriate Data Structures: Select data structures that balance the space-time trade-off for your specific use case.
- Avoid Unnecessary Object Creation: Reuse objects when possible, especially in performance-critical sections.
- Use Lazy Evaluation: Compute values only when needed, rather than precomputing and storing everything.
- Consider Compression: For large datasets, consider using compression techniques to reduce memory usage.
- Clean Up Resources: In garbage-collected languages, help the garbage collector by nullifying references to objects no longer needed.
- Use Streams for Large Data Processing: When working with large datasets, use streaming APIs to process data in chunks rather than loading everything into memory.
- Optimize Algorithms: Always look for more space-efficient algorithmic solutions to your problems.
10. Conclusion
Optimizing space complexity is a crucial skill for any programmer aiming to write efficient and scalable code. By understanding space complexity, choosing appropriate data structures, and applying optimization techniques, you can significantly improve the performance and resource utilization of your programs.
Remember that space optimization often involves trade-offs with time complexity or code readability. Always consider the specific requirements and constraints of your project when deciding on optimization strategies. With practice and awareness, you’ll develop an intuition for writing space-efficient code that can handle large-scale problems effectively.
As you continue to develop your programming skills, keep space complexity in mind alongside time complexity. The ability to balance these aspects will set you apart as a thoughtful and efficient programmer, capable of tackling complex challenges in resource-constrained environments.