How to Optimize on the Fly: Writing Efficient Code in Coding Interviews
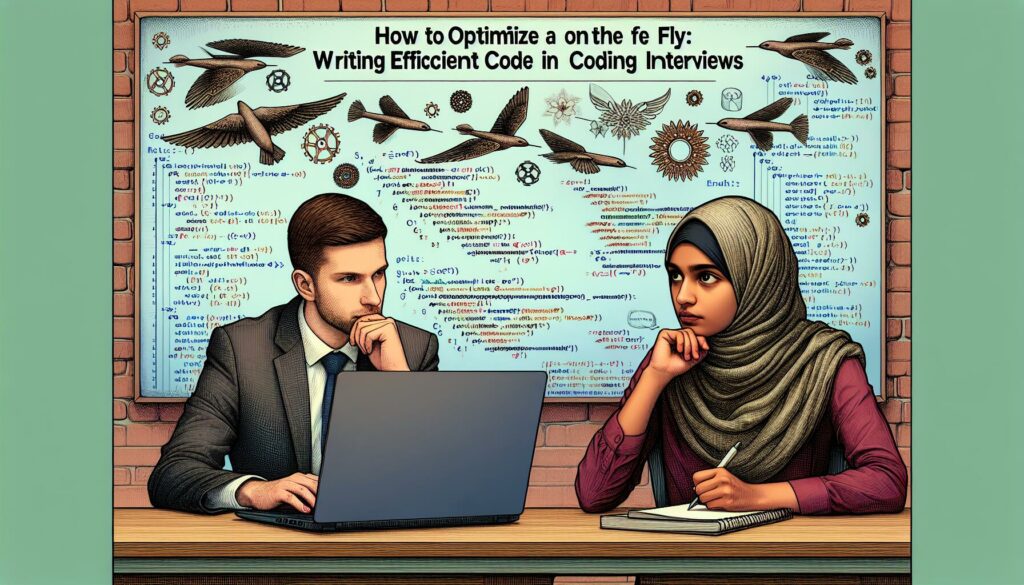
In the high-pressure environment of a coding interview, the ability to write efficient code quickly is a skill that can set you apart from other candidates. It’s not just about solving the problem; it’s about solving it optimally. This article will guide you through the process of optimizing your code on the fly, helping you move from a brute-force solution to an elegant, efficient algorithm during your interview.
Understanding the Importance of Optimization
Before we dive into specific strategies, it’s crucial to understand why optimization matters in coding interviews. When companies like Google, Amazon, or Facebook conduct technical interviews, they’re not just looking for someone who can write code that works. They’re searching for engineers who can write code that works well.
Optimization in coding generally refers to two main aspects:
- Time Complexity: How the execution time of your algorithm grows as the input size increases.
- Space Complexity: How much additional memory your algorithm needs as the input size increases.
By demonstrating your ability to optimize for both time and space, you show that you can:
- Think critically about algorithmic efficiency
- Consider scalability in your solutions
- Balance trade-offs between time and space
- Adapt and improve your code iteratively
These skills are invaluable in real-world software development, where efficiency can mean the difference between a product that scales to millions of users and one that crashes under load.
Starting with a Brute-Force Solution
When faced with a coding problem in an interview, it’s often best to start with a brute-force solution. This approach has several advantages:
- It demonstrates that you can quickly come up with a working solution.
- It provides a baseline for optimization.
- It helps you understand the problem more deeply.
- It gives you something to discuss and improve upon with your interviewer.
Let’s look at an example problem and walk through the process of optimization:
Problem: Given an array of integers, find two numbers such that they add up to a specific target number.
A brute-force solution might look like this:
def two_sum(nums, target):
for i in range(len(nums)):
for j in range(i + 1, len(nums)):
if nums[i] + nums[j] == target:
return [i, j]
return [] # No solution found
This solution has a time complexity of O(n^2) and a space complexity of O(1). It’s straightforward and works, but it’s not efficient for large inputs.
Strategies for On-the-Fly Optimization
Now that we have a working solution, let’s explore strategies to optimize it during the interview:
1. Identify the Bottleneck
The first step in optimization is identifying what’s making your current solution inefficient. In our example, the nested loops are the clear bottleneck, causing the quadratic time complexity.
2. Consider Data Structures
Often, using an appropriate data structure can dramatically improve efficiency. In this case, we can use a hash table (dictionary in Python) to reduce our time complexity:
def two_sum_optimized(nums, target):
num_dict = {}
for i, num in enumerate(nums):
complement = target - num
if complement in num_dict:
return [num_dict[complement], i]
num_dict[num] = i
return [] # No solution found
This solution has a time complexity of O(n) and a space complexity of O(n). We’ve traded some space for a significant improvement in time complexity.
3. Leverage Problem Constraints
Sometimes, the problem constraints can guide you towards optimizations. For instance, if the array is sorted, you could use a two-pointer approach:
def two_sum_sorted(nums, target):
left, right = 0, len(nums) - 1
while left < right:
current_sum = nums[left] + nums[right]
if current_sum == target:
return [left, right]
elif current_sum < target:
left += 1
else:
right -= 1
return [] # No solution found
This solution maintains O(n) time complexity but improves space complexity to O(1).
4. Look for Redundant Work
Identify any calculations or operations that are repeated unnecessarily. In our original brute-force solution, we were checking some pairs of numbers multiple times. The optimized versions eliminate this redundancy.
5. Consider Preprocessing
Sometimes, preprocessing the input can lead to more efficient solutions. For example, if this two-sum problem needed to be solved multiple times for the same array but different targets, sorting the array once could be beneficial:
def two_sum_preprocessed(nums, target):
sorted_nums = sorted((num, i) for i, num in enumerate(nums))
left, right = 0, len(nums) - 1
while left < right:
current_sum = sorted_nums[left][0] + sorted_nums[right][0]
if current_sum == target:
return [sorted_nums[left][1], sorted_nums[right][1]]
elif current_sum < target:
left += 1
else:
right -= 1
return [] # No solution found
This approach has a time complexity of O(n log n) due to sorting, but subsequent queries can be answered in O(n) time.
Communicating Your Optimization Process
In a coding interview, it’s not just about reaching the optimal solution; it’s about demonstrating your problem-solving process. Here’s how to effectively communicate your optimization thoughts:
- Think Aloud: Verbalize your thought process as you work through the problem. This gives the interviewer insight into your reasoning.
- Analyze as You Go: After implementing each solution, discuss its time and space complexity.
- Propose Improvements: Even if you’re not sure how to implement an optimization immediately, suggest potential improvements.
- Discuss Trade-offs: When choosing between different optimizations, explain the trade-offs you’re considering.
For example, you might say something like:
“I’ve implemented a brute-force solution with O(n^2) time complexity. To optimize this, we could use a hash table to store complements, trading some space for improved time complexity. This would bring us down to O(n) time, but increase our space usage to O(n). Alternatively, if we know the array is sorted, we could use a two-pointer approach to achieve O(n) time with O(1) space…”
Common Optimization Techniques
As you practice optimizing code, you’ll start to recognize common patterns and techniques. Here are some frequently used optimization strategies:
1. Memoization and Dynamic Programming
Memoization involves storing the results of expensive function calls and returning the cached result when the same inputs occur again. This is particularly useful in recursive algorithms and can often transform exponential time complexities into polynomial ones.
For example, consider the Fibonacci sequence calculation:
def fib(n):
if n <= 1:
return n
return fib(n-1) + fib(n-2)
This naive recursive implementation has an exponential time complexity. We can optimize it using memoization:
def fib_memoized(n, memo={}):
if n in memo:
return memo[n]
if n <= 1:
return n
memo[n] = fib_memoized(n-1, memo) + fib_memoized(n-2, memo)
return memo[n]
This memoized version has a time complexity of O(n) and space complexity of O(n).
2. Two Pointers Technique
We’ve already seen this technique in action with our sorted two-sum problem. It’s particularly useful for problems involving arrays or linked lists. The basic idea is to use two pointers that move through the data structure in a coordinated way to solve the problem efficiently.
3. Sliding Window
The sliding window technique is useful for problems involving subarrays or substrings. It maintains a “window” that slides over the data, updating the window’s state as it moves.
For example, to find the maximum sum of a subarray of size k:
def max_subarray_sum(arr, k):
n = len(arr)
if n < k:
return None
window_sum = sum(arr[:k])
max_sum = window_sum
for i in range(k, n):
window_sum = window_sum - arr[i-k] + arr[i]
max_sum = max(max_sum, window_sum)
return max_sum
This solution has a time complexity of O(n) and space complexity of O(1).
4. Binary Search
When dealing with sorted arrays, binary search can often reduce time complexity from O(n) to O(log n). It’s not just for searching; many problems can be reframed as binary search problems.
5. Divide and Conquer
This technique involves breaking down a problem into smaller subproblems, solving them, and then combining the results. It’s the basis for efficient algorithms like Merge Sort and Quick Sort.
Balancing Time and Space Complexity
Often, optimizing for time complexity comes at the cost of increased space complexity, and vice versa. The key is to find the right balance based on the specific requirements of the problem and the constraints of the system you’re working with.
Consider these factors when deciding how to balance time and space:
- Input Size: For small inputs, the difference between O(n) and O(n^2) might be negligible, and a simpler solution could be preferable.
- Frequency of Operation: If an operation is performed frequently, optimizing for time might be more important than saving space.
- Memory Constraints: In memory-constrained environments, space complexity might be the primary concern.
- Scalability Requirements: For solutions that need to handle potentially large inputs, prioritize solutions with better asymptotic behavior.
Remember, the goal is not always to achieve the absolute best time complexity at any cost. Sometimes, a slightly less optimal solution that’s easier to understand and maintain is the better choice in a real-world scenario.
Practice Makes Perfect
Optimizing code on the fly is a skill that improves with practice. Here are some ways to hone your optimization skills:
- Solve Problems Regularly: Platforms like LeetCode, HackerRank, and AlgoCademy offer a wide range of problems to practice on.
- Implement Multiple Solutions: For each problem you solve, try to come up with at least two different solutions with different time/space trade-offs.
- Analyze Existing Algorithms: Study well-known algorithms and data structures. Understanding how they work and why they’re efficient can inspire optimizations in your own code.
- Mock Interviews: Practice with a friend or use online platforms that offer mock interview services. This will help you get comfortable with verbalizing your thought process.
- Review and Reflect: After solving a problem, take time to review your solution. Are there any further optimizations you can think of? What did you learn from this problem that you can apply to future problems?
Conclusion
Optimizing code on the fly is a crucial skill for succeeding in coding interviews and in your career as a software developer. By starting with a brute-force solution and systematically improving it, you demonstrate not just your coding ability, but your problem-solving skills and algorithmic thinking.
Remember, the goal in an interview is not just to arrive at the most optimized solution, but to show your thought process and ability to iterate and improve your code. Communicate clearly, consider various approaches, and be prepared to discuss the trade-offs of different solutions.
With practice and a structured approach to optimization, you’ll be well-equipped to tackle complex coding problems and impress your interviewers. Happy coding, and may your algorithms always run in O(1) time!