How to Navigate Pair Programming in a Coding Interview: A Comprehensive Guide
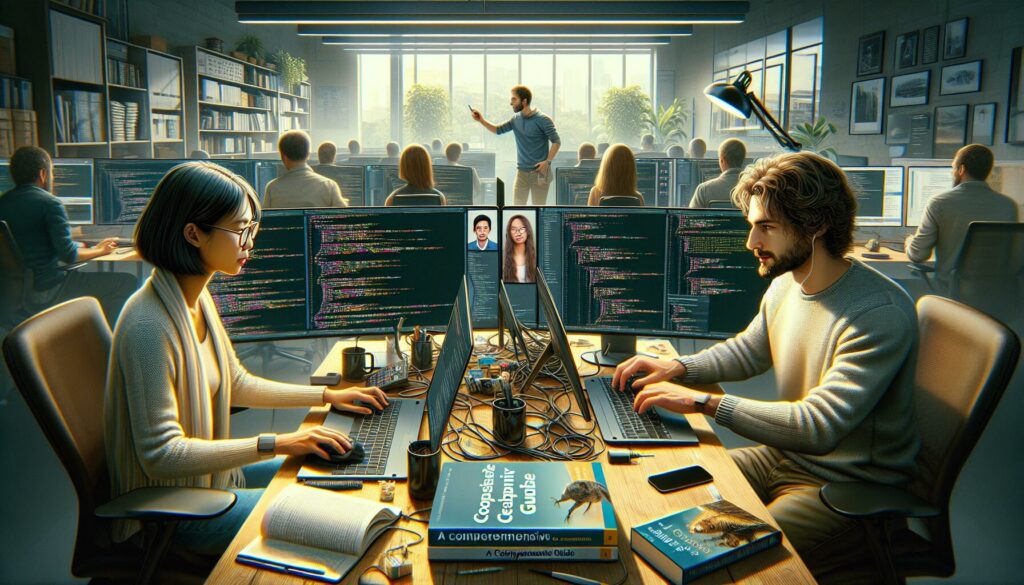
Pair programming interviews have become increasingly popular in the tech industry, especially among major companies like Google, Facebook, and Amazon. These interviews offer a unique opportunity for candidates to showcase their coding skills, problem-solving abilities, and collaboration prowess in real-time. If you’re preparing for a technical interview that includes pair programming, you’ve come to the right place. In this comprehensive guide, we’ll explore what to expect during a pair programming interview and provide valuable tips on how to collaborate effectively with your interviewer.
What is Pair Programming?
Before diving into the specifics of pair programming interviews, let’s first understand what pair programming is. Pair programming is a software development technique where two programmers work together at one workstation. Typically, one person, the “driver,” writes the code, while the other, the “navigator,” reviews each line of code as it’s typed. The two programmers switch roles frequently.
In the context of a coding interview, pair programming allows interviewers to assess not just your technical skills, but also your ability to work collaboratively, communicate effectively, and think critically under pressure.
What to Expect in a Pair Programming Interview
Pair programming interviews can vary depending on the company and the specific role you’re applying for. However, here are some common elements you can expect:
- Problem Introduction: The interviewer will present a coding problem or task.
- Collaborative Coding: You’ll work together with the interviewer to solve the problem.
- Role Switching: You may switch between “driver” and “navigator” roles.
- Discussion and Clarification: There will be ongoing dialogue about the approach and implementation.
- Code Review: You might review and refactor the code together.
- Time Constraint: The interview will typically last 45-60 minutes.
Tips for Effective Collaboration in a Pair Programming Interview
1. Communicate Clearly and Constantly
Clear communication is the cornerstone of successful pair programming. Here’s how you can ensure effective communication:
- Articulate your thoughts and ideas clearly
- Explain your reasoning behind decisions
- Ask questions when you’re unsure about something
- Provide context when introducing new concepts or approaches
Remember, the interviewer is not just evaluating your coding skills, but also your ability to communicate technical concepts effectively.
2. Listen Actively
Active listening is crucial in pair programming. When the interviewer is speaking:
- Give them your full attention
- Ask clarifying questions to ensure you understand their point
- Acknowledge their input, even if you disagree
- Take notes if necessary
3. Be Open to Feedback and Suggestions
Your interviewer may offer suggestions or point out potential issues in your code. Embrace this feedback constructively:
- Thank them for their input
- Consider their suggestions seriously
- If you disagree, explain your reasoning politely
- Be willing to change your approach if a better solution is proposed
4. Think Aloud
Verbalizing your thought process is crucial in a pair programming interview. It helps the interviewer understand your problem-solving approach and reasoning. Here’s how to do it effectively:
- Explain your initial thoughts on approaching the problem
- Discuss potential solutions and their pros and cons
- Walk through your code as you write it
- Mention any assumptions you’re making
5. Strike a Balance Between Listening and Driving the Solution
Finding the right balance between listening to your interviewer and driving the solution forward is crucial. Here are some tips:
- Start by listening carefully to the problem statement and any initial guidance
- Propose your ideas and approach, but be open to alternatives
- Take initiative in writing code, but pause periodically to check in with your interviewer
- If you’re stuck, don’t hesitate to ask for hints or clarification
- Involve your interviewer in the problem-solving process by asking for their opinion
6. Handle Disagreements Gracefully
You and your interviewer might not always agree on the best approach. Here’s how to handle disagreements professionally:
- Listen to their perspective fully before responding
- Acknowledge the merits of their approach
- Explain your reasoning clearly and respectfully
- Be willing to try their suggestion if they insist
- If time allows, you could implement both approaches and compare
7. Stay Calm Under Pressure
Pair programming interviews can be stressful, but maintaining composure is crucial. Here are some strategies:
- Take deep breaths if you feel anxious
- If you’re stuck, verbalize your thought process – this might help you find a solution
- Don’t be afraid to ask for a moment to think
- Remember that the process is as important as the final solution
Common Pair Programming Scenarios and How to Handle Them
Scenario 1: You’re Not Sure How to Start
If you’re unsure how to begin tackling the problem, try this approach:
- Acknowledge that you’re thinking about how to start
- Break down the problem into smaller steps
- Start with what you know – perhaps by writing some pseudocode
- Ask the interviewer if your initial approach seems reasonable
For example:
"I'm considering how to approach this problem. Let's break it down into steps. First, we need to [first step]. Then, we'll need to [second step]. Does this sound like a good starting point?"
Scenario 2: You’ve Made a Mistake
If you realize you’ve made a mistake, here’s how to handle it:
- Acknowledge the mistake calmly
- Explain why you think it’s a mistake
- Propose a solution
- Learn from it and move on
For instance:
"I just realized that this approach won't work because [reason]. Let's modify it by [proposed solution]. What do you think?"
Scenario 3: You Disagree with the Interviewer’s Suggestion
If you disagree with a suggestion from the interviewer, handle it this way:
- Acknowledge their suggestion
- Explain your concerns
- Propose an alternative
- Be open to discussion
You might say:
"I see where you're going with that suggestion. My concern is [explain concern]. What if we tried [your alternative] instead? It might address the issue by [explanation]. What are your thoughts on this approach?"
Preparing for a Pair Programming Interview
To excel in a pair programming interview, preparation is key. Here are some steps you can take:
1. Practice Pair Programming
Find a coding buddy or use online platforms that offer pair programming sessions. The more you practice, the more comfortable you’ll become with the process.
2. Enhance Your Problem-Solving Skills
Work on coding challenges regularly. Platforms like AlgoCademy offer a wide range of problems that can help you improve your algorithmic thinking and problem-solving skills.
3. Improve Your Communication Skills
Practice explaining your code and thought process out loud. You could record yourself solving problems and then review the recording to identify areas for improvement in your communication.
4. Learn to Use Collaborative Tools
Familiarize yourself with common collaborative coding environments like CodePen, JSFiddle, or even simple screen sharing with an editor. Some interviews might use these tools.
5. Review Common Algorithms and Data Structures
Ensure you have a solid understanding of fundamental algorithms and data structures. These often form the basis of interview questions.
6. Practice Active Listening
In your daily interactions, practice active listening. This skill will be invaluable during the interview.
Sample Pair Programming Interview Question
Let’s walk through a sample pair programming interview question to illustrate how you might approach it:
Problem: Write a function that takes a string as input and returns the length of the longest palindromic substring in that string.
Here’s how you might approach this in a pair programming scenario:
// Initial approach verbalization
"Let's start by defining what a palindrome is - a word or phrase that reads the same backward as forward. For this problem, we need to find the longest substring that's a palindrome.
One approach could be to check every possible substring, but that might not be very efficient. What do you think about using a more optimized method, like expanding around the center for each character?"
// After discussion and agreement on the approach
function longestPalindromicSubstring(s) {
if (!s || s.length < 2) {
return s.length;
}
let start = 0;
let maxLength = 1;
function expandAroundCenter(left, right) {
while (left >= 0 && right < s.length && s[left] === s[right]) {
const currentLength = right - left + 1;
if (currentLength > maxLength) {
start = left;
maxLength = currentLength;
}
left--;
right++;
}
}
for (let i = 0; i < s.length; i++) {
expandAroundCenter(i, i); // Odd length palindromes
expandAroundCenter(i, i + 1); // Even length palindromes
}
return maxLength;
}
// Explanation of the solution
"This solution uses the expand around center approach. We iterate through each character in the string and consider it as a potential center of a palindrome. We then expand outwards, comparing characters on both sides.
We do this twice for each position - once considering the current character as the center (for odd-length palindromes), and once considering the current and next character as the center (for even-length palindromes).
We keep track of the start index and length of the longest palindrome found so far. The time complexity of this solution is O(n^2), where n is the length of the string, and the space complexity is O(1).
What do you think about this approach? Is there anything you'd like to modify or any edge cases we should consider?"
Remember, in a real pair programming interview, this would be an interactive process with back-and-forth discussion between you and the interviewer.
Conclusion
Navigating a pair programming interview successfully requires a combination of technical skills, communication abilities, and collaborative spirit. By understanding what to expect, preparing thoroughly, and following the tips outlined in this guide, you’ll be well-equipped to showcase your abilities and make a strong impression.
Remember, the key to success in a pair programming interview is not just solving the problem, but demonstrating your ability to work effectively with others. Show your thought process, be open to feedback, and engage actively with your interviewer. With practice and the right approach, you can turn the pair programming interview into an opportunity to shine and demonstrate why you’re the right fit for the role.
Keep honing your skills, stay curious, and approach each interview as a learning experience. With persistence and the right mindset, you’ll be well on your way to acing your next pair programming interview and landing your dream job in tech.