How to Navigate a Coding Interview with Multiple Interviewers: A Comprehensive Guide
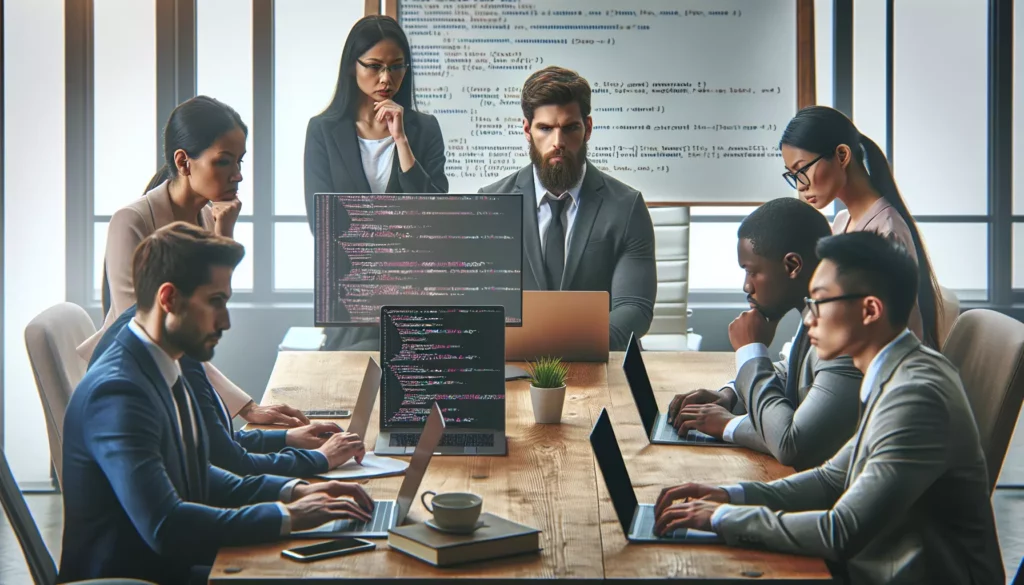
Coding interviews can be nerve-wracking experiences, especially when you’re faced with multiple interviewers. However, with the right preparation and mindset, you can turn this challenging situation into an opportunity to showcase your skills and impress potential employers. In this comprehensive guide, we’ll explore strategies to help you navigate a coding interview with multiple interviewers successfully.
Understanding the Multiple Interviewer Format
Before diving into strategies, it’s essential to understand why companies use multiple interviewers and what to expect in this format.
Why Companies Use Multiple Interviewers
- To get diverse perspectives on a candidate
- To assess different skills and aspects of a candidate’s abilities
- To reduce individual bias in the hiring process
- To simulate team dynamics and observe how candidates interact in a group setting
What to Expect
In a multiple interviewer coding interview, you might encounter:
- A panel of 2-5 interviewers, often with different roles (e.g., software engineer, team lead, HR representative)
- Questions from different interviewers, sometimes in rapid succession
- Collaborative problem-solving scenarios
- Observation of your coding process and problem-solving approach
Preparing for the Interview
Thorough preparation is key to success in any coding interview, but it’s especially crucial when facing multiple interviewers.
1. Brush Up on Core Computer Science Concepts
Review fundamental concepts such as:
- Data structures (arrays, linked lists, trees, graphs, etc.)
- Algorithms (sorting, searching, dynamic programming, etc.)
- Time and space complexity analysis
- Object-oriented programming principles
- Design patterns
2. Practice Coding Problems
Use platforms like AlgoCademy, LeetCode, or HackerRank to practice coding problems. Focus on:
- Solving problems under time pressure
- Explaining your thought process out loud as you code
- Implementing solutions in different programming languages
- Optimizing your solutions for better time and space complexity
3. Improve Your Communication Skills
In a multiple interviewer setting, clear communication is crucial. Practice:
- Explaining technical concepts to non-technical audience
- Articulating your problem-solving approach
- Asking clarifying questions
- Handling interruptions and switching context
4. Research the Company and Interviewers
If possible, try to find out:
- The company’s tech stack and products
- Recent news or developments about the company
- Background information on your interviewers (if their names are provided)
- Common interview questions asked by the company
Strategies for the Interview Day
Now that you’re prepared, let’s look at strategies to help you navigate the interview successfully.
1. Make a Strong First Impression
- Arrive early and dress appropriately
- Greet each interviewer individually and remember their names
- Show enthusiasm and a positive attitude
- Maintain good eye contact with all interviewers
2. Manage Your Attention
With multiple interviewers, it’s important to:
- Address your answers to the person who asked the question, but occasionally make eye contact with other interviewers
- Be prepared for rapid-fire questions from different interviewers
- Stay focused and don’t let interruptions derail your thought process
3. Demonstrate Your Problem-Solving Process
When tackling coding problems:
- Think out loud and explain your approach
- Start with a high-level solution before diving into coding
- Discuss trade-offs between different approaches
- Ask for clarification if needed
4. Handle Collaborative Scenarios
If asked to work on a problem collaboratively:
- Listen actively to others’ ideas
- Build on others’ suggestions
- Be open to feedback and alternative approaches
- Demonstrate leadership by guiding the discussion when appropriate
5. Manage Time Effectively
- Ask about time constraints for each problem
- Prioritize solving the problem over writing perfect code initially
- If you’re stuck, communicate your thoughts and ask for hints
- Be prepared to optimize your solution if time allows
6. Show Adaptability
Different interviewers may have different expectations or areas of focus:
- Be prepared to switch gears quickly between different types of questions
- Demonstrate your ability to learn and adapt on the spot
- If you make a mistake, acknowledge it and show how you’d correct it
Handling Common Challenges
Let’s address some common challenges you might face in a multiple interviewer coding interview and how to handle them.
1. Conflicting Feedback or Instructions
If you receive conflicting feedback or instructions from different interviewers:
- Politely acknowledge the different perspectives
- Ask for clarification on which approach they’d like you to pursue
- If appropriate, suggest a compromise or hybrid approach
2. Interruptions While Coding
If you’re interrupted while in the middle of coding:
- Pause and give your full attention to the interviewer
- Answer their question or address their comment
- Before resuming, briefly recap where you were in your thought process
3. Feeling Overwhelmed
If you start feeling overwhelmed by the multiple interviewers:
- Take a deep breath and pause for a moment if needed
- Focus on one interviewer or problem at a time
- Don’t be afraid to ask for a moment to collect your thoughts
4. Disagreement Among Interviewers
If you notice disagreement among the interviewers:
- Stay neutral and avoid taking sides
- Acknowledge the validity of different viewpoints
- Focus on the problem at hand rather than the disagreement
Demonstrating Your Coding Skills
In a multiple interviewer setting, it’s crucial to showcase your coding skills effectively. Here are some tips:
1. Write Clean, Readable Code
Even under pressure, strive to write code that is:
- Well-organized and properly indented
- Commented where necessary
- Following naming conventions
For example, when writing a function to find the maximum element in an array:
def find_max(arr):
# Check if the array is empty
if not arr:
return None
# Initialize max_value with the first element
max_value = arr[0]
# Iterate through the array to find the maximum value
for num in arr[1:]:
if num > max_value:
max_value = num
return max_value
2. Optimize Your Solutions
After implementing a working solution, discuss potential optimizations:
- Analyze the time and space complexity of your initial solution
- Propose ways to improve efficiency
- Implement the optimized solution if time allows
For instance, if you’ve implemented a bubble sort algorithm, you might discuss how it could be improved:
def bubble_sort(arr):
n = len(arr)
for i in range(n):
for j in range(0, n - i - 1):
if arr[j] > arr[j + 1]:
arr[j], arr[j + 1] = arr[j + 1], arr[j]
return arr
# Optimization discussion:
# 1. Time complexity is O(n^2), which is not efficient for large datasets
# 2. We could improve this by using a more efficient sorting algorithm like Quick Sort or Merge Sort
# 3. Another optimization could be to stop the algorithm early if no swaps are made in a pass
3. Handle Edge Cases
Demonstrate your thoroughness by considering and handling edge cases:
- Empty inputs
- Extremely large or small inputs
- Invalid inputs
Here’s an example of handling edge cases in a function that calculates the average of an array:
def calculate_average(arr):
# Handle empty array
if not arr:
return None
# Handle non-numeric elements
try:
total = sum(arr)
count = len(arr)
return total / count
except TypeError:
return "Error: Array contains non-numeric elements"
4. Use Appropriate Data Structures
Show your knowledge of data structures by choosing the most appropriate one for the problem at hand. For example, when implementing a function to check for balanced parentheses:
def is_balanced(s):
stack = []
opening = set('([{')
closing = set(')]}')
pair = {')': '(', ']': '[', '}': '{'}
for char in s:
if char in opening:
stack.append(char)
elif char in closing:
if not stack or stack[-1] != pair[char]:
return False
stack.pop()
return len(stack) == 0
In this example, using a stack is an efficient way to keep track of opening parentheses and match them with closing ones.
Concluding the Interview
As the interview comes to an end, there are a few key things to remember:
1. Ask Thoughtful Questions
Prepare questions for each interviewer based on their role or expertise. This shows your genuine interest and engagement. Some examples include:
- “What are the biggest technical challenges your team is currently facing?”
- “How does the company support continuous learning and professional development?”
- “Can you tell me about a recent project you found particularly interesting or challenging?”
2. Express Your Interest
If you’re genuinely interested in the position, make sure to express your enthusiasm:
- Summarize why you think you’d be a good fit for the role
- Highlight how your skills align with the company’s needs
- Thank the interviewers for their time and the opportunity
3. Follow Up
After the interview:
- Send a thank-you email to each interviewer within 24 hours
- Reiterate your interest in the position
- If appropriate, connect with the interviewers on professional networking platforms
Conclusion
Navigating a coding interview with multiple interviewers can be challenging, but it’s also an opportunity to showcase your skills, adaptability, and ability to work in a team setting. By thoroughly preparing, staying calm under pressure, and following the strategies outlined in this guide, you can significantly improve your chances of success.
Remember, the key is to demonstrate not just your coding skills, but also your problem-solving approach, communication abilities, and how you handle complex situations. With practice and the right mindset, you can turn a potentially stressful situation into a chance to shine and land your dream job in the tech industry.
Keep honing your skills, stay curious, and approach each interview as a learning experience. Whether you’re using platforms like AlgoCademy to prepare or practicing with peers, every effort you make brings you one step closer to acing that multiple interviewer coding interview and launching your career in tech.