How to Master Big-O Notation for Coding Interviews
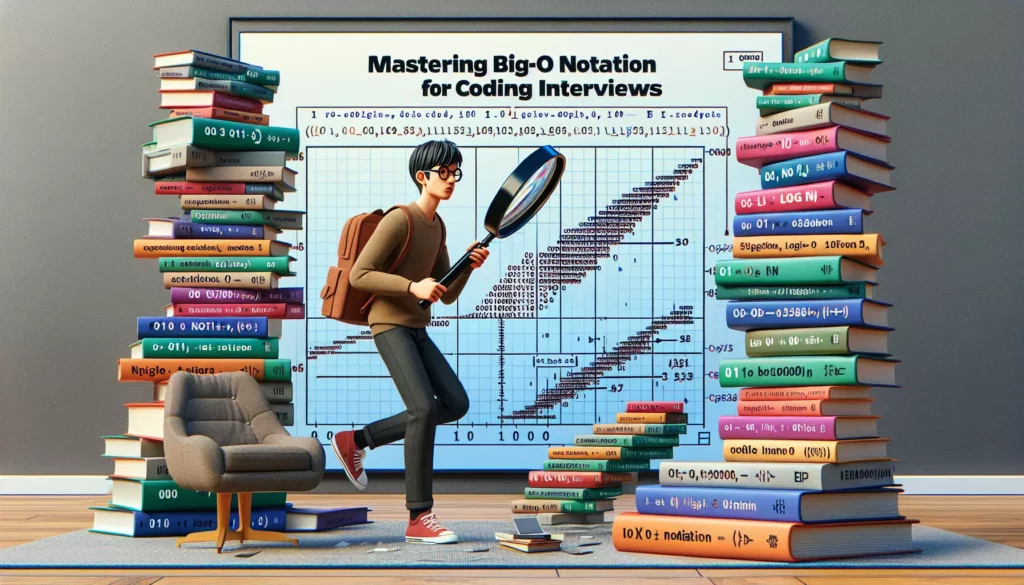
When preparing for coding interviews, especially for top tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google), mastering Big-O notation is crucial. This concept is fundamental to analyzing algorithm efficiency and is frequently asked about in technical interviews. In this comprehensive guide, we’ll dive deep into Big-O notation, its importance, and how to effectively use it to ace your coding interviews.
What is Big-O Notation?
Big-O notation is a mathematical notation used in computer science to describe the performance or complexity of an algorithm. Specifically, it describes the worst-case scenario, or the maximum time it takes to execute as a function of the input size. It’s essential for several reasons:
- It provides a standardized way to talk about algorithm performance
- It allows us to compare different algorithms’ efficiency
- It helps in making decisions about which algorithm to use for a given problem
- It’s a common language used in technical interviews to discuss code efficiency
Common Big-O Complexities
Before diving into how to master Big-O notation, let’s review some common time complexities you’ll encounter:
- O(1) – Constant time
- O(log n) – Logarithmic time
- O(n) – Linear time
- O(n log n) – Linearithmic time
- O(n^2) – Quadratic time
- O(2^n) – Exponential time
- O(n!) – Factorial time
Steps to Master Big-O Notation
1. Understand the Basics
Start by thoroughly understanding what Big-O notation represents. It’s about the rate of growth of the algorithm’s running time as the input size increases. Remember, Big-O notation always represents the worst-case scenario.
2. Learn to Identify Common Patterns
Certain code structures typically correspond to specific Big-O complexities. For example:
- Simple operations (assignment, arithmetic) are usually O(1)
- Single loops are often O(n)
- Nested loops are typically O(n^2)
- Divide and conquer algorithms (like binary search) are often O(log n)
3. Practice Analyzing Code
Regularly practice analyzing different code snippets and determining their time complexity. Here’s an example:
for (int i = 0; i < n; i++) {
for (int j = 0; j < n; j++) {
System.out.println(i + j);
}
}
This nested loop structure has a time complexity of O(n^2) because for each iteration of the outer loop (which runs n times), the inner loop also runs n times.
4. Understand Simplification Rules
Learn the rules for simplifying Big-O notation:
- Drop constants: O(2n) becomes O(n)
- Drop non-dominant terms: O(n^2 + n) becomes O(n^2)
5. Learn About Space Complexity
While time complexity is more commonly discussed, space complexity is also important. It refers to the amount of memory an algorithm uses relative to the input size. The principles are similar to time complexity analysis.
6. Study Different Data Structures
Different data structures have different time complexities for various operations. Familiarize yourself with common data structures and their Big-O complexities:
- Arrays: Access – O(1), Search – O(n), Insertion – O(n), Deletion – O(n)
- Hash Tables: Access – N/A, Search – O(1) average, O(n) worst, Insertion – O(1) average, Deletion – O(1) average
- Binary Search Trees: Access – O(log n), Search – O(log n), Insertion – O(log n), Deletion – O(log n)
7. Understand Amortized Analysis
Some operations might seem expensive but are actually efficient when considered over a sequence of operations. For example, dynamic arrays in many programming languages have an amortized O(1) time complexity for adding an element, even though occasional resizing operations are O(n).
8. Practice with Real Interview Questions
Work through actual coding interview questions and practice determining the time and space complexity of your solutions. This will help you get comfortable applying Big-O analysis in a interview-like setting.
Common Pitfalls to Avoid
When mastering Big-O notation, be aware of these common mistakes:
1. Focusing Only on Time Complexity
While time complexity is crucial, don’t neglect space complexity. Sometimes, an algorithm might be time-efficient but memory-intensive, which could be problematic in certain scenarios.
2. Overlooking Hidden Loops
Be cautious of hidden loops in your code. For example, certain string or array operations in some languages might involve iterating over elements behind the scenes.
3. Misunderstanding Logarithmic Complexity
O(log n) doesn’t always mean the algorithm uses binary search. It could also apply to algorithms that repeatedly divide the problem size, like certain divide-and-conquer algorithms.
4. Ignoring the Input Size
Remember that Big-O notation describes how the runtime grows relative to the input size. An O(n^2) algorithm might be faster than an O(n) algorithm for small inputs, but it will be slower for large inputs.
Advanced Topics in Big-O Notation
Once you’ve mastered the basics, consider exploring these advanced topics:
1. Multi-Variable Time Complexity
Some algorithms have time complexities that depend on multiple variables. For example, an algorithm that operates on a matrix might have a complexity of O(nm), where n and m are the dimensions of the matrix.
2. Big-Ω (Omega) and Big-Θ (Theta) Notation
While Big-O represents the upper bound (worst-case scenario), Big-Ω represents the lower bound (best-case scenario), and Big-Θ represents both the upper and lower bounds (when they’re the same).
3. Recursion and Master Theorem
Understanding how to analyze recursive algorithms is crucial. The Master Theorem is a powerful tool for analyzing the time complexity of many divide-and-conquer algorithms.
4. NP-Completeness
While not directly related to Big-O notation, understanding NP-completeness can give you a deeper appreciation for algorithm complexity and the limits of efficient computation.
Applying Big-O Knowledge in Interviews
During coding interviews, your understanding of Big-O notation will be put to the test. Here’s how to effectively apply your knowledge:
1. Analyze Your Solution
After coding a solution, always analyze and state its time and space complexity. This shows initiative and a deep understanding of your code.
2. Compare Different Approaches
If you can think of multiple solutions, compare their complexities. This demonstrates your ability to evaluate trade-offs between different algorithms.
3. Optimize Your Code
If your initial solution has a high time or space complexity, think about ways to optimize it. Can you use a more efficient data structure? Can you eliminate nested loops?
4. Explain Your Reasoning
When discussing Big-O complexity, explain your thought process. This gives the interviewer insight into your analytical skills and allows them to guide you if you’re on the wrong track.
5. Consider Edge Cases
Remember that Big-O represents worst-case scenarios. Consider what inputs might lead to this worst-case performance.
Practice Problems
To solidify your understanding, try analyzing the time and space complexity of these code snippets:
Problem 1:
def find_sum(arr):
total = 0
for num in arr:
total += num
return total
Time Complexity: O(n) – We iterate through the array once.
Space Complexity: O(1) – We only use a single variable regardless of input size.
Problem 2:
def find_pairs(arr):
for i in range(len(arr)):
for j in range(i+1, len(arr)):
if arr[i] + arr[j] == 0:
print(f"Pair found: {arr[i]}, {arr[j]}")
Time Complexity: O(n^2) – We have nested loops, each potentially iterating through most of the array.
Space Complexity: O(1) – We don’t use any extra space that scales with input size.
Problem 3:
def binary_search(arr, target):
left, right = 0, len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1
Time Complexity: O(log n) – We’re repeatedly dividing our search space in half.
Space Complexity: O(1) – We’re using a constant amount of extra space.
Conclusion
Mastering Big-O notation is a crucial skill for succeeding in coding interviews, especially for top tech companies. It allows you to analyze and communicate about the efficiency of your algorithms effectively. Remember, practice is key. The more you work with Big-O notation, the more intuitive it will become.
As you continue your journey in algorithm analysis, consider using platforms like AlgoCademy, which offer interactive coding tutorials and resources specifically designed to help you progress from beginner-level coding to preparing for technical interviews. With features like AI-powered assistance and step-by-step guidance, you can enhance your algorithmic thinking and problem-solving skills, making Big-O notation and other complex concepts more approachable.
Keep practicing, stay curious, and remember that understanding algorithm efficiency is not just about passing interviews—it’s a fundamental skill that will serve you throughout your career as a software developer. Happy coding!