How to Manage State in Complex Applications: A Comprehensive Guide
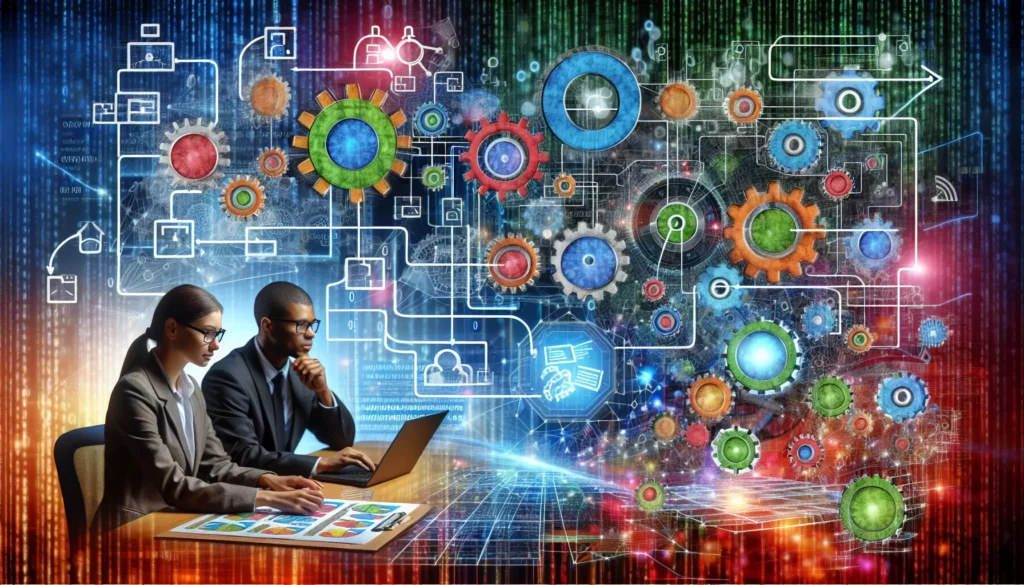
In the world of software development, managing state in complex applications is a crucial skill that can make or break your project. As applications grow in size and complexity, keeping track of data flow and maintaining a consistent state becomes increasingly challenging. This comprehensive guide will explore various techniques and best practices for managing state in complex applications, helping you build more robust, scalable, and maintainable software.
Table of Contents
- Understanding State in Applications
- Challenges in State Management
- State Management Patterns and Techniques
- Popular State Management Libraries
- Best Practices for State Management
- Testing State Management
- Performance Considerations
- Real-World Examples
- Conclusion
1. Understanding State in Applications
Before diving into state management techniques, it’s essential to understand what state is and why it’s crucial in application development.
What is State?
In the context of software applications, state refers to the data that represents the current condition of an application at a given point in time. This includes user input, server responses, UI component properties, and any other data that can change during the application’s lifecycle.
Types of State
There are several types of state in applications:
- Local State: Data that is specific to a single component or module.
- Global State: Data that is shared across multiple components or the entire application.
- Server State: Data that comes from a server and needs to be synchronized with the client-side state.
- URL State: Data that is stored in the URL, often used for navigation and sharing specific application states.
Importance of State Management
Proper state management is crucial for several reasons:
- Ensures data consistency across the application
- Improves application performance
- Facilitates easier debugging and testing
- Enhances user experience by maintaining application coherence
- Enables better code organization and maintainability
2. Challenges in State Management
As applications grow in complexity, managing state becomes increasingly difficult. Here are some common challenges developers face:
State Inconsistency
When multiple components or modules interact with the same piece of state, it can lead to inconsistencies if not managed properly. This is especially problematic in applications with real-time updates or concurrent operations.
Prop Drilling
In component-based architectures, passing state through multiple levels of nested components (known as prop drilling) can make the code harder to maintain and understand.
Unnecessary Re-renders
Poor state management can lead to unnecessary re-renders of components, affecting the application’s performance and user experience.
Complex Data Flows
As applications grow, the flow of data between components and modules can become increasingly complex, making it difficult to track and debug issues.
Scalability Issues
State management solutions that work well for small applications may not scale effectively as the application grows in size and complexity.
3. State Management Patterns and Techniques
To address the challenges of state management, developers have devised various patterns and techniques. Let’s explore some of the most popular ones:
Flux Architecture
Flux is an architectural pattern developed by Facebook to manage the flow of data in applications. It promotes unidirectional data flow, making it easier to track changes and debug issues.
Key components of Flux:
- Actions: Plain JavaScript objects that describe what happened in the application.
- Dispatcher: A central hub that manages all data flow in the application.
- Stores: Containers for application state and logic.
- Views: React components that render the UI based on the current state.
Redux
Redux is a popular state management library that implements the Flux architecture with some modifications. It introduces the concept of a single source of truth for the entire application state.
Key concepts in Redux:
- Store: A single object that holds the entire application state.
- Actions: Plain objects describing what happened in the application.
- Reducers: Pure functions that specify how the application’s state changes in response to actions.
- Middleware: Functions that can intercept and modify actions before they reach the reducer.
Context API (React)
The Context API is a built-in feature in React that allows you to pass data through the component tree without having to pass props manually at every level.
Key components of the Context API:
- React.createContext: Creates a Context object.
- Context.Provider: Wraps components and provides the state to all children.
- Context.Consumer: Allows components to consume the provided state.
MobX
MobX is a state management library that uses observable data structures to automatically track changes and update the UI accordingly.
Key concepts in MobX:
- Observable State: State that can be observed for changes.
- Computed Values: Values derived from the observable state.
- Reactions: Side effects that automatically run when observed data changes.
- Actions: Methods that modify the state.
Recoil (React)
Recoil is a state management library for React applications that introduces the concept of atoms and selectors for managing state.
Key concepts in Recoil:
- Atoms: Units of state that components can subscribe to.
- Selectors: Pure functions that compute derived data based on atoms or other selectors.
- Hooks: useRecoilState, useRecoilValue, and useSetRecoilState for interacting with Recoil state.
4. Popular State Management Libraries
While we’ve touched on some libraries in the previous section, let’s take a closer look at some popular state management libraries and their unique features:
Redux
Redux is one of the most widely used state management libraries, particularly in React applications. It provides a predictable state container and enforces a strict unidirectional data flow.
Pros:
- Predictable state updates
- Easy to debug with Redux DevTools
- Large ecosystem of middleware and extensions
Cons:
- Steep learning curve
- Requires a lot of boilerplate code
- Can be overkill for small applications
MobX
MobX offers a more flexible approach to state management compared to Redux, using observable data structures and automatic tracking of state changes.
Pros:
- Less boilerplate code
- Automatic tracking of state changes
- Works well with object-oriented programming
Cons:
- Less predictable than Redux
- Can be harder to debug complex state changes
- Smaller ecosystem compared to Redux
Recoil
Recoil is a newer state management library developed by Facebook specifically for React applications. It aims to provide a more flexible and performant solution for managing complex application states.
Pros:
- Designed specifically for React
- Easier to manage code splitting and lazy loading
- Good performance for large-scale applications
Cons:
- Relatively new, with a smaller community and ecosystem
- May require more setup for certain use cases
- Limited support for non-React applications
Vuex (Vue.js)
Vuex is the official state management library for Vue.js applications. It implements a centralized store for all components in a Vue application.
Pros:
- Tightly integrated with Vue.js
- Simple and straightforward API
- Good documentation and community support
Cons:
- Limited to Vue.js applications
- Can be verbose for small applications
- Lacks some advanced features found in other libraries
XState
XState is a library for creating, interpreting, and executing finite state machines and statecharts. It provides a different approach to state management by modeling application logic as state machines.
Pros:
- Helps in modeling complex application logic
- Provides visual tools for designing state machines
- Framework-agnostic
Cons:
- Steep learning curve for developers unfamiliar with state machines
- Can be overkill for simple applications
- Requires a different mental model compared to traditional state management
5. Best Practices for State Management
Regardless of the state management solution you choose, following these best practices will help you maintain a clean and efficient state management system:
1. Keep State Minimal and Flat
Avoid storing redundant or derived data in your state. Instead, compute derived values when needed. Also, try to keep your state structure as flat as possible to avoid deep nesting.
2. Use Immutable Updates
When updating state, always create new objects or arrays instead of mutating existing ones. This helps in maintaining predictability and makes it easier to track changes.
Example using JavaScript spread operator:
const updateUser = (state, userId, updates) => {
return {
...state,
users: {
...state.users,
[userId]: {
...state.users[userId],
...updates
}
}
};
};
3. Normalize Complex Data
For complex data structures, consider normalizing your data. This involves storing entities in a flat structure and using references to represent relationships.
4. Use Selectors for Derived Data
Instead of storing derived data in your state, use selector functions to compute it on-demand. This helps keep your state minimal and avoids inconsistencies.
5. Implement Error Handling
Make sure to handle errors gracefully in your state management system. This includes handling network errors, validation errors, and unexpected state transitions.
6. Use Middleware for Side Effects
For asynchronous operations or side effects, use middleware (like Redux Thunk or Redux Saga) to keep your reducers pure and predictable.
7. Follow the Single Responsibility Principle
Each part of your state management system should have a single, well-defined responsibility. This applies to reducers, actions, and selectors.
8. Use TypeScript or PropTypes
Utilize TypeScript or PropTypes to add static typing to your state management code. This helps catch errors early and improves code maintainability.
9. Implement Proper Testing
Write unit tests for your reducers, selectors, and action creators. This ensures that your state management logic works as expected and helps prevent regressions.
6. Testing State Management
Testing is a crucial aspect of maintaining a robust state management system. Here are some strategies for testing different parts of your state management:
Testing Reducers
Reducers are pure functions, making them easy to test. You can write unit tests that provide an initial state and an action, then assert that the resulting state matches the expected output.
Example using Jest:
import { userReducer } from './userReducer';
import { UPDATE_USER } from './actions';
describe('userReducer', () => {
it('should handle UPDATE_USER action', () => {
const initialState = { name: 'John' };
const action = { type: UPDATE_USER, payload: { name: 'Jane' } };
const expectedState = { name: 'Jane' };
expect(userReducer(initialState, action)).toEqual(expectedState);
});
});
Testing Selectors
Selectors can also be tested as pure functions. Provide a mock state and assert that the selector returns the expected derived data.
import { getUserName } from './selectors';
describe('selectors', () => {
it('should select user name', () => {
const state = { user: { name: 'John' } };
expect(getUserName(state)).toBe('John');
});
});
Testing Action Creators
For synchronous action creators, you can test that they return the correct action object. For asynchronous action creators (e.g., those using Redux Thunk), you’ll need to mock the dispatch function and any API calls.
import { updateUser } from './actions';
import { UPDATE_USER } from './actionTypes';
describe('actions', () => {
it('should create an action to update user', () => {
const expectedAction = {
type: UPDATE_USER,
payload: { name: 'Jane' }
};
expect(updateUser({ name: 'Jane' })).toEqual(expectedAction);
});
});
Integration Testing
In addition to unit tests, it’s important to write integration tests that verify the interaction between different parts of your state management system. This can involve testing the entire flow from dispatching an action to updating the UI.
7. Performance Considerations
As applications grow in complexity, performance can become a concern. Here are some tips to optimize the performance of your state management:
1. Use Memoization
Memoize selectors and components to prevent unnecessary recalculations and re-renders. Libraries like Reselect can help with this.
2. Implement Lazy Loading
For large applications, implement code splitting and lazy loading to reduce the initial bundle size and improve load times.
3. Optimize Redux Store
If using Redux, consider using Redux Toolkit, which includes optimizations like memoized selectors and simplified store setup.
4. Use Immutable Data Structures
Consider using immutable data structures (like Immutable.js) for large datasets to improve performance of equality checks and updates.
5. Avoid Excessive Re-renders
Use tools like React DevTools or Vue DevTools to identify and fix unnecessary re-renders caused by state changes.
6. Batch Updates
When making multiple state updates, batch them together to reduce the number of re-renders.
8. Real-World Examples
Let’s look at some real-world examples of state management in complex applications:
E-commerce Platform
An e-commerce platform might use Redux for global state management, handling things like:
- User authentication state
- Shopping cart contents
- Product catalog and inventory
- Order history and tracking
The application might use Redux Thunk for handling asynchronous actions like API calls to fetch product data or process orders.
Social Media Application
A social media application might use a combination of global and local state management:
- Global state (using Redux or MobX) for user profile, friends list, and notifications
- Local state (using React hooks) for UI components like dropdowns or modals
- Server state (using libraries like React Query) for efficiently caching and synchronizing data with the server
Project Management Tool
A project management tool might use a state machine library like XState to manage complex workflows:
- Task states (To Do, In Progress, Review, Done)
- Project lifecycles
- User permissions and role-based access control
This approach can help in modeling and managing complex business logic and state transitions.
9. Conclusion
Managing state in complex applications is a challenging but crucial aspect of modern software development. By understanding the principles of state management, choosing the right tools and patterns, and following best practices, you can build robust and maintainable applications that scale effectively.
Remember that there’s no one-size-fits-all solution for state management. The best approach depends on your specific application requirements, team expertise, and project constraints. Don’t be afraid to experiment with different techniques and libraries to find what works best for your use case.
As you continue to develop your skills in state management, focus on:
- Understanding the underlying principles and patterns
- Staying up-to-date with the latest tools and best practices
- Writing clean, testable code
- Continuously refactoring and optimizing your state management as your application evolves
By mastering state management, you’ll be well-equipped to tackle the challenges of building and maintaining complex applications in today’s fast-paced software development landscape.