How to Manage Dependencies in Your Projects: A Comprehensive Guide
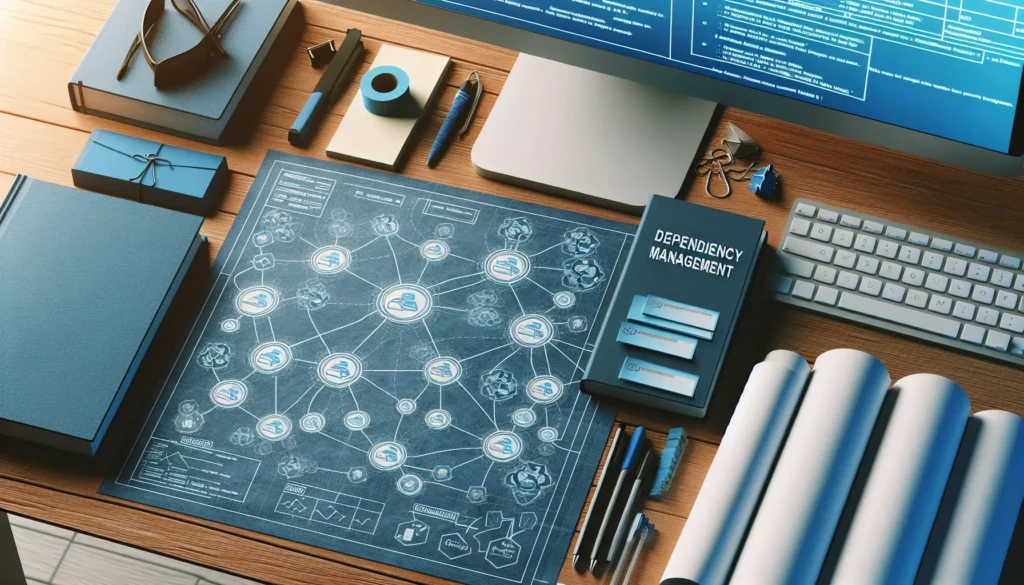
In the world of software development, managing dependencies is a crucial skill that can make or break your project. Whether you’re a beginner just starting out or an experienced developer working on complex systems, understanding how to effectively handle dependencies is essential for creating robust, maintainable, and efficient code. In this comprehensive guide, we’ll explore the ins and outs of dependency management, covering everything from basic concepts to advanced techniques that will help you streamline your development process and improve the overall quality of your projects.
Table of Contents
- What Are Dependencies?
- Why Dependency Management Matters
- Types of Dependencies
- Dependency Management Tools
- Best Practices for Managing Dependencies
- Version Control and Dependencies
- Dealing with Dependency Hell
- Security Considerations in Dependency Management
- Automating Dependency Management
- The Future of Dependency Management
1. What Are Dependencies?
In software development, dependencies are external pieces of code, libraries, or modules that your project relies on to function correctly. These can range from small utility functions to large frameworks that provide essential functionality. Dependencies allow developers to leverage existing solutions and avoid reinventing the wheel, ultimately saving time and effort in the development process.
For example, if you’re building a web application using Python, you might depend on libraries like Flask for web framework functionality, SQLAlchemy for database operations, and Requests for making HTTP requests. Each of these libraries serves a specific purpose and helps you build your application more efficiently.
2. Why Dependency Management Matters
Effective dependency management is crucial for several reasons:
- Consistency: Ensures that all developers working on a project use the same versions of dependencies, reducing conflicts and inconsistencies.
- Reproducibility: Allows for consistent builds and deployments across different environments.
- Maintainability: Makes it easier to update, add, or remove dependencies as needed.
- Security: Helps in identifying and addressing security vulnerabilities in dependencies.
- Performance: Proper management can lead to optimized builds and runtime performance.
- Collaboration: Facilitates easier collaboration among team members and integration with CI/CD pipelines.
3. Types of Dependencies
Understanding the different types of dependencies is crucial for effective management. Here are the main categories:
3.1 Direct Dependencies
These are dependencies that your project explicitly requires to function. They are typically listed in your project’s configuration or manifest file (e.g., package.json for Node.js projects or requirements.txt for Python projects).
3.2 Transitive Dependencies
Transitive dependencies are the dependencies of your direct dependencies. They are not explicitly declared in your project but are required by the libraries you use. Managing transitive dependencies can be challenging, as they can lead to version conflicts and security issues if not handled properly.
3.3 Development Dependencies
These are dependencies required only during development, such as testing frameworks, linters, or build tools. They are not needed for the production deployment of your application.
3.4 Runtime Dependencies
Runtime dependencies are required for your application to run in a production environment. These are the core dependencies that your application needs to function correctly.
4. Dependency Management Tools
Different programming languages and ecosystems have their own dependency management tools. Here are some popular ones:
4.1 npm and Yarn (JavaScript)
npm (Node Package Manager) and Yarn are widely used for managing dependencies in JavaScript projects. They use a package.json file to define project dependencies and provide commands for installing, updating, and removing packages.
Example package.json:
{
"name": "my-project",
"version": "1.0.0",
"dependencies": {
"express": "^4.17.1",
"lodash": "^4.17.21"
},
"devDependencies": {
"jest": "^27.0.6",
"eslint": "^7.32.0"
}
}
4.2 pip and Poetry (Python)
pip is the default package installer for Python, while Poetry is a more modern tool that provides dependency resolution and virtual environment management. They use requirements.txt or pyproject.toml files to specify dependencies.
Example requirements.txt:
Flask==2.0.1
SQLAlchemy==1.4.23
requests==2.26.0
4.3 Maven and Gradle (Java)
Maven and Gradle are popular build automation and dependency management tools for Java projects. They use XML (Maven) or Groovy/Kotlin DSL (Gradle) to define project configurations and dependencies.
Example Maven pom.xml:
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
<version>2.5.4</version>
</dependency>
</dependencies>
4.4 NuGet (.NET)
NuGet is the package manager for .NET, used to manage dependencies in C# and other .NET language projects. It uses a packages.config file or PackageReference elements in project files to specify dependencies.
5. Best Practices for Managing Dependencies
To effectively manage dependencies in your projects, consider the following best practices:
5.1 Use Semantic Versioning
Adopt semantic versioning (SemVer) for your project and dependencies. This helps in understanding the impact of updates and maintaining compatibility. SemVer uses a three-part version number (MAJOR.MINOR.PATCH) where:
- MAJOR version increments indicate incompatible API changes
- MINOR version increments add functionality in a backward-compatible manner
- PATCH version increments make backward-compatible bug fixes
5.2 Lock Dependency Versions
Use lock files (e.g., package-lock.json, Pipfile.lock) to ensure consistent installations across different environments. These files specify exact versions of all dependencies, including transitive ones.
5.3 Regularly Update Dependencies
Keep your dependencies up to date to benefit from bug fixes, security patches, and new features. However, be cautious and test thoroughly after updates, especially for major version changes.
5.4 Minimize Dependencies
Only include dependencies that are absolutely necessary for your project. Each additional dependency increases complexity and potential security risks.
5.5 Use Private Repositories
For proprietary or sensitive code, consider using private package repositories to host your own dependencies securely.
6. Version Control and Dependencies
Proper integration of dependency management with version control systems is crucial for collaborative development. Here are some tips:
6.1 Include Dependency Manifests in Version Control
Always include your dependency manifest files (e.g., package.json, requirements.txt) in version control. This ensures that all developers have the same dependency specifications.
6.2 Lock Files in Version Control
Include lock files in version control to ensure consistent installations across different environments and developer machines.
6.3 Gitignore Dependency Directories
Add dependency directories (e.g., node_modules, venv) to your .gitignore file to avoid cluttering your repository with installed packages.
7. Dealing with Dependency Hell
“Dependency Hell” refers to situations where conflicts between different versions of dependencies make it difficult or impossible to satisfy all requirements simultaneously. Here are strategies to avoid and resolve dependency hell:
7.1 Use a Dependency Resolver
Many modern package managers include dependency resolvers that automatically handle version conflicts. For example, npm and Yarn use sophisticated algorithms to find compatible versions of packages.
7.2 Analyze Dependency Trees
Use tools to visualize and analyze your dependency tree. For npm, you can use the npm list
command or tools like npm-check-updates.
7.3 Upgrade Strategically
When upgrading dependencies, do so incrementally and test thoroughly after each upgrade. Start with patch updates, then minor, and finally major versions if necessary.
7.4 Use Dependency Overrides
In some cases, you may need to override a transitive dependency to resolve conflicts. Many package managers provide ways to do this, but use this feature cautiously as it can lead to unexpected behavior.
8. Security Considerations in Dependency Management
Security is a critical aspect of dependency management. Here are some key considerations:
8.1 Regular Security Audits
Regularly run security audits on your dependencies. Many package managers provide built-in tools for this, such as npm audit
for npm projects.
8.2 Use Trusted Sources
Only use dependencies from trusted sources and reputable package registries. Be cautious when using packages with few downloads or limited community support.
8.3 Keep Dependencies Updated
Regularly update your dependencies to ensure you have the latest security patches. However, always test thoroughly after updates.
8.4 Implement Dependency Scanning in CI/CD
Integrate dependency scanning tools into your CI/CD pipeline to automatically detect and alert on security vulnerabilities.
9. Automating Dependency Management
Automating dependency management can save time and reduce errors. Here are some automation strategies:
9.1 Dependency Update Bots
Use tools like Dependabot or Renovate to automatically create pull requests for dependency updates. These bots can be configured to update dependencies on a schedule or when new versions are released.
9.2 Automated Testing
Implement comprehensive automated tests to ensure that dependency updates don’t break your application. This is crucial for safely automating updates.
9.3 CI/CD Integration
Integrate dependency checks and updates into your CI/CD pipeline. This can include running security audits, checking for outdated packages, and even automatically merging minor updates that pass all tests.
10. The Future of Dependency Management
As software development continues to evolve, so does dependency management. Here are some trends and future directions:
10.1 AI-Assisted Dependency Management
Artificial Intelligence and Machine Learning are being incorporated into dependency management tools to predict compatibility issues, suggest optimal version combinations, and even generate patches for vulnerabilities.
10.2 Improved Security Features
Future dependency management tools are likely to include more advanced security features, such as automated vulnerability patching and real-time threat detection.
10.3 Better Handling of Transitive Dependencies
Expect improvements in how package managers handle transitive dependencies, potentially including more granular control over nested dependencies and better conflict resolution algorithms.
10.4 Integration with Cloud Services
Dependency management is likely to become more tightly integrated with cloud services, offering features like centralized dependency caching and cloud-based security scanning.
Conclusion
Effective dependency management is a critical skill for any developer or development team. By understanding the concepts, tools, and best practices outlined in this guide, you can significantly improve the quality, security, and maintainability of your software projects. Remember that dependency management is an ongoing process that requires regular attention and updates.
As you continue to develop your skills, consider exploring more advanced topics such as monorepo management, dependency injection, and microservices architecture, all of which have their own unique challenges and solutions in the realm of dependency management.
By mastering dependency management, you’ll be better equipped to handle complex projects, collaborate effectively with team members, and create robust, scalable software solutions. Keep learning, stay updated with the latest tools and practices, and don’t hesitate to experiment with different approaches to find what works best for your projects and team.